//本博主所写的代码仅为阅读者提供参考;
//若有不足之处请提出,博主会尽所能修改;
//附上课后编程练习题目;
//若是对您有用的话请点赞或分享提供给它人;
//11.13 - 1.c
#include <stdio.h>
#define LEN 10
void getnchar(char str[], int n);
int main(int argc, char *argv[])
{
char input[LEN];
printf("Please enter %d characters:\n", LEN - 1);
getnchar(input, LEN);
printf("Result:\n");
puts(input);
printf("Done.\n");
return 0;
}
void getnchar(char str[], int n)
{
int i = 0;
while (i < n - 1)
{
str[i++] = getchar();
}
str[i] = '\0';
return;
}
//-------------
//11.13 - 2.c
#include <stdio.h>
#include <ctype.h>
#define LEN 10
void getnchar(char str[], int n);
int main(int argc, char *argv[])
{
char input[LEN];
printf("Please enter %d characters:\n", LEN - 1);
getnchar(input, LEN);
printf("Result:\n");
puts(input);
printf("Done.\n");
return 0;
}
void getnchar(char str[], int n)
{
int i = -1;
while (++i < n - 1)
{
str[i] = getchar();
if (isspace(str[i]))
{
break;
}
}
str[i] = '\0';
return;
}
//-------------
//11.13 - 3.c
#include <stdio.h>
#include <ctype.h>
#define LEN 10
char *getword(char *str);
int main(int argc, char *argv[])
{
char input[LEN];
printf("Please enter a word (EOF to quit):\n");
while (getword(input) != NULL)
{
printf("Result:\n");
puts(input);
printf("You can enter a word again (EOF to quit):\n");
}
printf("Done.\n");
return 0;
}
char *getword(char *str)
{
int ch;
int n = 0;
char *pt = str;
while ((ch = getchar()) != EOF && isspace(ch))
continue;
//↑跳过第一个非空白字符前面的所有空白符;
if (ch == EOF)
{
return NULL;
//↑若第一次直接输入Ctrl+Z(Windows)或Ctrl+D(Unix/Linux/Mac os)则返回空指针;
}
else
{
n++;
*str++ = ch;
/*↑把第一个非空白字符赋值给str
所指向的内存空间内并指向下一个
存储空间;*/
}
while ((ch = getchar()) != EOF && !isspace(ch) && (n < LEN - 1))
{
*str++ = ch;
/*↑从第2个字符开始赋值直到
遇见单词后面第一个空白符;*/
n++;
}
*str = '\0';
if (ch == EOF)
{
return NULL;
/*↑输入Ctrl+Z(Windows)或Ctrl+D(Unix/Linux/Mac os)返回空指针;*/
}
else
{
while (getchar() != '\n')
continue;
//↑从单词后面丢弃输入行中的其它字符;
return pt;
}
}
//-------------
//11.13 - 4.c
扫描二维码关注公众号,回复:
12354532 查看本文章
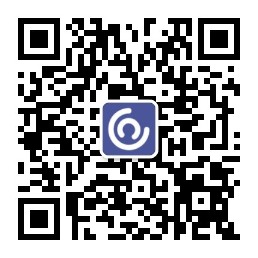
#include <stdio.h>
#include <ctype.h>
#define LEN 10
char *getword(char *str, int len);
int main(int argc, char *argv[])
{
char input[LEN];
printf("Please enter a word (EOF to quit):\n");
while (getword(input, LEN - 1) != NULL)
{
printf("Result:\n");
puts(input);
printf("You can enter a word again (EOF to quit):\n");
}
printf("Done.\n");
return 0;
}
char *getword(char *str, int len)
{
int ch;
int n = 0;
char *pt = str;
while ((ch = getchar()) != EOF && isspace(ch))
continue;
//↑跳过第一个非空白字符前面的所有空白符;
if (ch == EOF)
{
return NULL;
//↑若第一次直接输入Ctrl+Z(Windows)或Ctrl+D(Unix/Linux/Mac os)则返回空指针;
}
else
{
n++;
*str++ = ch;
/*↑把第一个非空白字符赋值给str
所指向的内存空间内并指向下一个
存储空间;*/
}
while ((ch = getchar()) != EOF && !isspace(ch) && n < len)
{
*str++ = ch;
/*↑从第2个字符开始赋值直到
遇见单词后面第一个空白符;*/
n++;
}
*str = '\0';
if (ch == EOF)
{
return NULL;
/*↑输入Ctrl+Z(Windows)或Ctrl+D(Unix/Linux/Mac os)返回空指针;*/
}
else
{
while (getchar() != '\n')
continue;
//↑从单词后面丢弃输入行中的其它字符;
return pt;
}
}
//-------------
//11.13 - 5.c
#include <stdio.h>
#include <string.h>
#define LEN 20
char *s_gets(char *st, int n);
char *mystrchr(char *str, char ch);
int main(int argc, char *argv[])
{
char ch, input[LEN];
printf("Please enter a string (EOF to quit):\n");
while (s_gets(input, LEN) != NULL)
{
printf("Please enter a character: ");
ch = getchar();
while (getchar() != '\n')
continue;
printf("String:\n");
puts(input);
if (mystrchr(input, ch) == NULL)
{
printf("Not exist %c in the string.\n", ch);
}
else
{
printf("Exist %c in the string.\n", ch);
}
printf("You can enter a string again (EOF to quit):\n");
}
printf("Done.\n");
return 0;
}
char *s_gets(char *st, int n)
{
char *ret_val;
char *find;
ret_val = fgets(st, n, stdin);
if (ret_val)
{
find = strchr(st, '\n');
if (find)
{
*find = '\0';
}
else
{
while (getchar() != '\n')
continue;
}
}
return ret_val;
}
char *mystrchr(char *str, char ch)
{
while (*str != '\0')
{
if (*str != ch)
{
++str;
}
else
{
return str;
}
}
return NULL;
}
//-------------
//11.13 - 6.c
#include <stdio.h>
#include <string.h>
#define LEN 20
char *s_gets(char *st, int n);
int is_within(char ch, const char *str);
int main(int argc, char *argv[])
{
char ch, input[LEN];
printf("Please enter a string (EOF to quit):\n");
while (s_gets(input, LEN) != NULL)
{
printf("Please enter a character: ");
ch = getchar();
while (getchar() != '\n')
continue;
printf("String:\n");
puts(input);
if (!is_within(ch, input))
{
printf("Not exist %c in the string.\n", ch);
}
else
{
printf("Exist %c in the string.\n", ch);
}
printf("You can enter a string again (EOF to quit):\n");
}
printf("Done.\n");
return 0;
}
char *s_gets(char *st, int n)
{
char *ret_val;
char *find;
ret_val = fgets(st, n, stdin);
if (ret_val)
{
find = strchr(st, '\n');
if (find)
{
*find = '\0';
}
else
{
while (getchar() != '\n')
continue;
}
}
return ret_val;
}
int is_within(char ch, const char *str)
{
while (*str)
{
if (*str != ch)
{
++str;
}
else
{
return 1;
}
}
return 0;
}
//-------------
//11.13 - 7.c
#include <stdio.h>
#include <string.h>
#define LEN 10
void eatline(void);
char *mystrncpy(char *dest, char *src, int n);
char *s_gets(char *st, int n);
int main(int argc, char *argv[])
{
int len;
char target[LEN];
char source[LEN];
printf("Please enter a string (EOF to quit):\n");
while (s_gets(source, LEN) != NULL)
{
printf("Please enter a number for copy (> 0): ");
while (scanf("%d", &len) != 1 || len <= 0)
{
eatline();
printf("Please enter again: ");
}
eatline();
printf("Source string: %s\n", source);
printf("Target string: %s\n", mystrncpy(target, source, len));
printf("You can enter a string again (EOF to quit):\n");
}
printf("Done.\n");
return 0;
}
char *s_gets(char *st, int n)
{
char *ret_val;
char *find;
ret_val = fgets(st, n, stdin);
if (ret_val)
{
find = strchr(st, '\n');
if (find)
{
*find = '\0';
}
else
{
eatline();
}
}
return ret_val;
}
void eatline(void)
{
while (getchar() != '\n')
continue;
return;
}
char *mystrncpy(char *dest, char *src, int n)
{
int count = 0;
while (*src != '\0' && count < n)
{
*(dest + count) = *src++;
//↑源字符串不为空且长度小于n时复制;
count++;
}
*(dest + count) = '\0';
//↑保证字符串是有效的;
return dest;
}
//-------------
//11.13 - 8.c
#include <stdio.h>
#include <string.h>
#define LEN 10
char *s_gets(char *st, int n);
char *string_in(char *str, char *pt);
int main(int argc, char *argv[])
{
char str1[LEN];
char str2[LEN];
printf("Please enter the first string (EOF to quit):\n");
while (s_gets(str1, LEN) != NULL)
{
printf("Please enter the second string:\n");
if (s_gets(str2, LEN) != NULL)
{
if (string_in(str1, str2) != NULL)
{
printf("String %s exists in string %s\n", str2, str1);
}
else
{
printf("String %s doesn't exist in string %s\n", str2, str1);
}
}
printf("You can enter again (EOF to quit):\n");
}
printf("Done.\n");
return 0;
}
char *s_gets(char *st, int n)
{
char *ret_val;
char *find;
ret_val = fgets(st, n, stdin);
if (ret_val)
{
find = strchr(st, '\n');
if (find)
{
*find = '\0';
}
else
{
while (getchar() != '\n')
continue;
}
}
return ret_val;
}
char *string_in(char *str, char *pt)
{
int i = 0;
int j = 0;
int str_len = strlen(str); //获取主串长度;
int pt_len = strlen(pt); //获取子串长度;
while (i < str_len && j < pt_len)
{
if (str[i] == pt[j])
{
i++;
j++;
}
else //若是主串字符与子串字符不相同则重新匹配;
{
i = i - j + 1;
j = 0;
}
}
return j == pt_len ? str : NULL;
}
//-------------
//11.13 - 9.c
#include <stdio.h>
#include <string.h>
#define LEN 10
char *s_gets(char *st, int n);
void reverse(char *str);
int main(int argc, char *argv[])
{
char input[LEN];
printf("Please enter a string (EOF to quit):\n");
while (s_gets(input, LEN) != NULL)
{
printf("String:\n");
puts(input);
reverse(input);
printf("After reversing:\n");
puts(input);
printf("You can enter a string again (EOF to quit):\n");
}
printf("Done.\n");
return 0;
}
char *s_gets(char *st, int n)
{
char *ret_val;
char *find;
ret_val = fgets(st, n, stdin);
if (ret_val)
{
find = strchr(st, '\n');
if (find)
{
*find = '\0';
}
else
{
while (getchar() != '\n')
continue;
}
}
return ret_val;
}
void reverse(char *str)
{
int i;
char temp;
int len = strlen(str);
for (i = 0; i < len / 2; i++)
{
temp = str[i];
str[i] = str[len - 1 - i];
str[len - 1 - i] = temp;
}
return;
}
//-------------
//11.13 - 10.c
#include <stdio.h>
#include <string.h>
#define LEN 10
#define SPACE ' '
char *s_gets(char *st, int n);
void cancel(char *str);
int main(int argc, char *argv[])
{
char source[LEN];
printf("Please enter a string (EOF or enter to quit):\n");
while (s_gets(source, LEN) != NULL && source[0] != '\0')
{
printf("Source string: %s\n", source);
cancel(source);
printf("Delete space: %s\n", source);
printf("You can enter a string again (EOF or enter to quit):\n");
}
printf("Done.\n");
return 0;
}
char *s_gets(char *st, int n)
{
char *ret_val;
char *find;
ret_val = fgets(st, n, stdin);
if (ret_val)
{
find = strchr(st, '\n');
if (find)
{
*find = '\0';
}
else
{
while (getchar() != '\n')
continue;
}
}
return ret_val;
}
void cancel(char *str)
{
char *pt = str;
while (*str)
{
if (*str != SPACE)
{
*pt++ = *str++;
/*↑修改pt指向的内容就相当
于修改了str所指向的内容;*/
}
else
{
str++;
/*↑若是空格则统计空格数并
指向下一个内存空间;*/
}
}
*pt = '\0';
return;
}
//-------------
//11.13 - 11.c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
#include <stdbool.h>
#define ROWS 10
#define COLUMNS 10
int get_strings(char (*string)[COLUMNS], char **str, int n);
int get_first(void);
int show_menu(void);
int word(char *str);
char *s_gets(char *st, int n);
void origin_output(char (*str)[COLUMNS], int n);
void ascll_output(char **str, int n);
void length_up_output(char **str, int n);
void first_word_output(char **str, int n);
int main(void)
{
int n, choice;
char *str[ROWS];
char strings[ROWS][COLUMNS];
printf("Please enter %d strings (EOF to quit):\n", ROWS);
if ((n = get_strings(strings, str, ROWS)) != 0)
{
while ((choice = show_menu()) != 'q')
{
switch (choice)
{
case 'a':
{
origin_output(strings, n);
break;
}
case 'b':
{
ascll_output(str, n);
break;
}
case 'c':
{
length_up_output(str, n);
break;
}
case 'd':
{
first_word_output(str, n);
break;
}
}
}
}
printf("Done.\n");
return 0;
}
int get_strings(char (*string)[COLUMNS], char **str, int n)
{
int i;
for (i = 0; i < n; i++)
{
if (s_gets(string[i], COLUMNS) != NULL)
{
str[i] = string[i];
}
else
{
break;
}
}
return i;
}
int get_first(void)
{
int ch;
do
{
ch = tolower(getchar());
} while (isspace(ch));
while (getchar() != '\n')
continue;
return ch;
}
int show_menu(void)
{
int ch;
printf("============================================\n");
printf("a) Print source strings\n");
printf("b) Print source strings for ASCII\n");
printf("c) Print source strings for length\n");
printf("d) Print source strings for the first word\n");
printf("q) Quit\n");
printf("============================================\n");
printf("Please you choose: ");
ch = get_first();
while (ch < 'a' || ch > 'd' && ch != 'q')
{
printf("Please enter a, b, c, d or q: ");
ch = get_first();
}
return ch;
}
int word(char *str)
{
int length = 0;
bool inword = false;
/*统计字符串第一个非空白字符的单词
长度并作为返回值传递给调用函数;*/
while (*str)
{
if (!isspace(*str) && !inword)
{
/*↑从第一个非空白字符
开始统计单词长度*/
inword = true;
length++;
}
else if (!isspace(*str) && inword)
{
length++;
}
else if (isspace(*str) && inword)
{
/*↑若遇到第一个单词后的空白符
则退出循环*/
break;
}
str++;
}
return length;
}
char *s_gets(char *st, int n)
{
char *ret_val;
char *find;
ret_val = fgets(st, n, stdin);
if (ret_val)
{
find = strchr(st, '\n');
if (find)
{
*find = '\0';
}
else
{
while (getchar() != '\n')
continue;
}
}
return ret_val;
}
void origin_output(char (*str)[COLUMNS], int n)
{
int i;
printf("Source strings:\n", n);
for (i = 0; i < n; i++)
{
puts(str[i]);
}
putchar('\n');
return;
}
void ascll_output(char **str, int n)
{
int i, j;
char *temp;
for (i = 0; i < n - 1; i++)
{
for (j = i + 1; j < n; j++)
{
//↓按ASCII码顺序进行排序;
if (strcmp(str[i], str[j]) > 0)
{
temp = str[i];
str[i] = str[j];
str[j] = temp;
}
}
}
printf("Print source strings for ASCII:\n", n);
for (i = 0; i < n; i++)
{
puts(str[i]);
}
putchar('\n');
return;
}
void length_up_output(char **str, int n)
{
int i, j;
char *temp;
for (i = 0; i < n - 1; i++)
{
for (j = i + 1; j < n; j++)
{
//↓按字符串中字符数的多少进行排序;
if (strlen(str[i]) > strlen(str[j]))
{
temp = str[i];
str[i] = str[j];
str[j] = temp;
}
}
}
printf("Print source strings for length:\n", n);
for (i = 0; i < n; i++)
{
puts(str[i]);
}
putchar('\n');
return;
}
void first_word_output(char **str, int n)
{
int i, j;
char *temp;
for (i = 0; i < n - 1; i++)
{
for (j = i + 1; j < n; j++)
{
//↓按第一个单词长度进行排序;
if (word(str[i]) > word(str[j]))
{
temp = str[i];
str[i] = str[j];
str[j] = temp;
}
}
}
printf("Print source strings for the first word:\n", n);
for (i = 0; i < n; i++)
{
puts(str[i]);
}
putchar('\n');
return;
}
//-------------
//11.13 - 12.c
#include <stdio.h>
#include <ctype.h>
#include <stdbool.h>
int main(int argc, char *argv[])
{
int ch, lower, upper, digit;
int punct, words;
lower = upper = digit = 0;
punct = words = 0;
bool inword = false;
printf("Please enter some characters (EOF to quit):\n");
while ((ch = getchar()) != EOF)
{
if (islower(ch))
{
lower++;
}
else if (isupper(ch))
{
upper++;
}
else if (isdigit(ch))
{
digit++;
}
else if (ispunct(ch))
{
punct++;
}
if (!isspace(ch) && !inword)
{
inword = true;
words++;
}
if (isspace(ch) && inword)
{
inword = false;
}
}
printf("Words: %d\n", words);
printf("Lower: %d\n", lower);
printf("Upper: %d\n", upper);
printf("Digit: %d\n", digit);
printf("Punct: %d\n", punct);
return 0;
}
//-------------
//11.13 - 13.c
#include <stdio.h>
int main(int argc, char *argv[])
{
int i = 0;
if (argc < 2)
{
printf("Usage: %s words\n", argv[0]);
}
else
{
printf("Words:\n");
for (i = 1; i < argc; i++)
{
printf("%s ", argv[i]);
}
printf("\nReversing printing is:\n");
for (i = argc - 1; i > 0; i--)
{
printf("%s ", argv[i]);
}
putchar('\n');
}
return 0;
}
//-------------
//11.13 - 14.c
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char *argv[])
{
int i, exp;
double num, sum;
sum = 1.0;
if (argc != 3)
{
printf("Usage: %s double_number exp\n", argv[0]);
}
else
{
num = atof(argv[1]);
exp = atoi(argv[2]);
for (i = 1; i <= exp; i++)
{
sum *= num;
}
printf("%g ^ %d is %g.\n", num, exp, sum);
}
return 0;
}
//-------------
//11.13 - 15.c
#include <stdio.h>
#include <string.h>
#include <ctype.h>
#define LEN 10
char *s_gets(char *st, int n);
int myatoi(char *str);
int main(int argc, char *argv[])
{
char input[LEN];
printf("Please enter a string (EOF to quit):\n");
while (s_gets(input, LEN) != NULL)
{
printf("String %s convert number %d\n", input, myatoi(input));
printf("You can enter a string again (EOF to quit):\n");
}
printf("Done.\n");
return 0;
}
char *s_gets(char *st, int n)
{
char *ret_val;
char *find;
ret_val = fgets(st, n, stdin);
if (ret_val)
{
find = strchr(st, '\n');
if (find)
{
*find = '\0';
}
else
{
while (getchar() != '\n')
continue;
}
}
return ret_val;
}
int myatoi(char *str)
{
int i;
int n = 0;
for (i = 0; i < strlen(str); i++)
{
if (!isdigit(str[i]))
{
return 0;
}
else
{
n = n * 10 + (str[i] - '0');
}
}
return n;
}
//-------------
//11.13 - 16.c
#include <stdio.h>
#include <ctype.h>
int main(int argc, char *argv[])
{
int ch;
int flag = 1;
char mode = 'p';
if (argc > 2)
{
printf("Usage: %s [-p | -u | -l]\n", argv[0]);
flag = 0;
}
else if (argc == 2)
{
if (argv[1][0] != '-')
{
printf("Usage: %s [-p | -u | -l]\n", argv[0]);
flag = 0;
}
else
{
switch (argv[1][1])
{
case 'p':
case 'u':
case 'l':
{
mode = argv[1][1];
break;
}
default:
{
printf("The argument isn't p, u or l, the program start to run with -p way.\n");
}
}
}
}
if (flag)
{
printf("Please enter some characters (EOF to quit):\n");
while ((ch = getchar()) != EOF)
{
switch (mode)
{
case 'p':
{
putchar(ch);
break;
}
case 'u':
{
putchar(toupper(ch));
break;
}
case 'l':
{
putchar(tolower(ch));
break;
}
}
}
}
printf("Done.\n");
return 0;
}
//-------------
//----------------------------2020年4月4日 -------------------------------
//致敬英雄,缅怀同胞,愿山河无恙,人间皆安!