书店管理系统正确入口
用户模块(登入账号:admin 密码:123):
界面(丑请见谅):
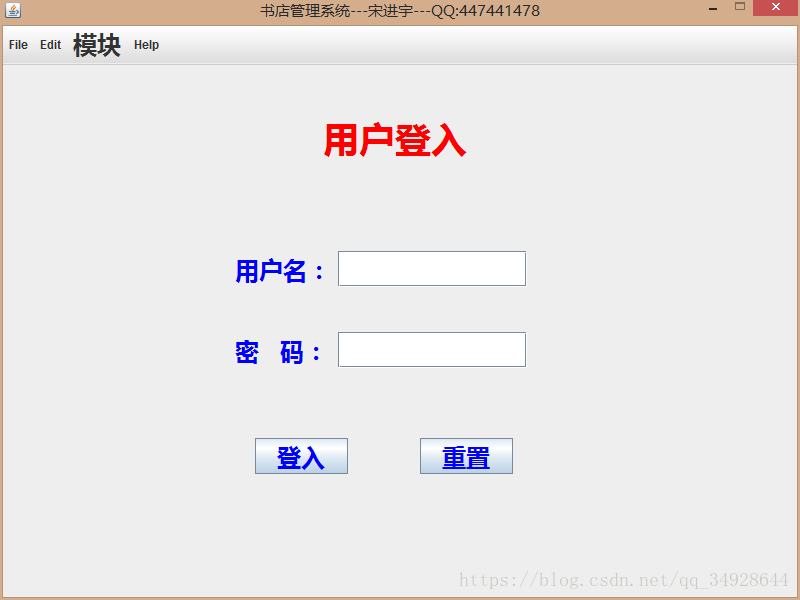
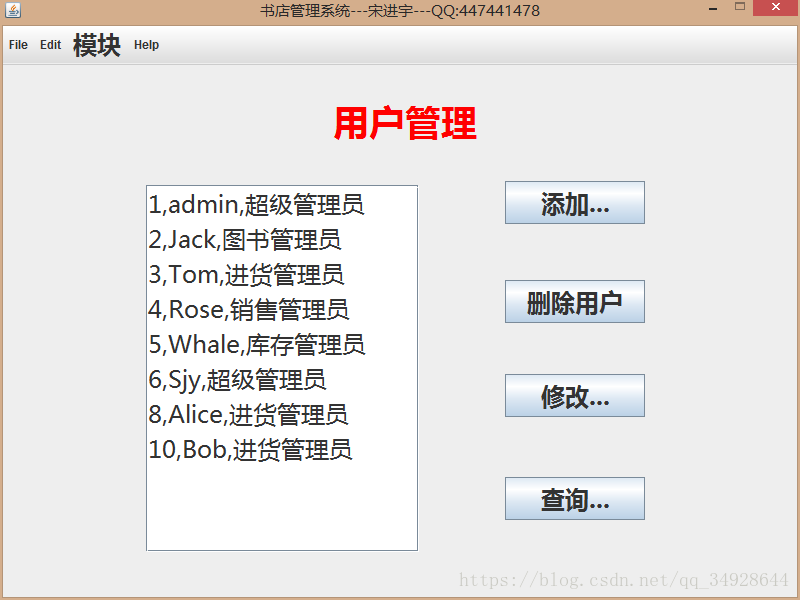
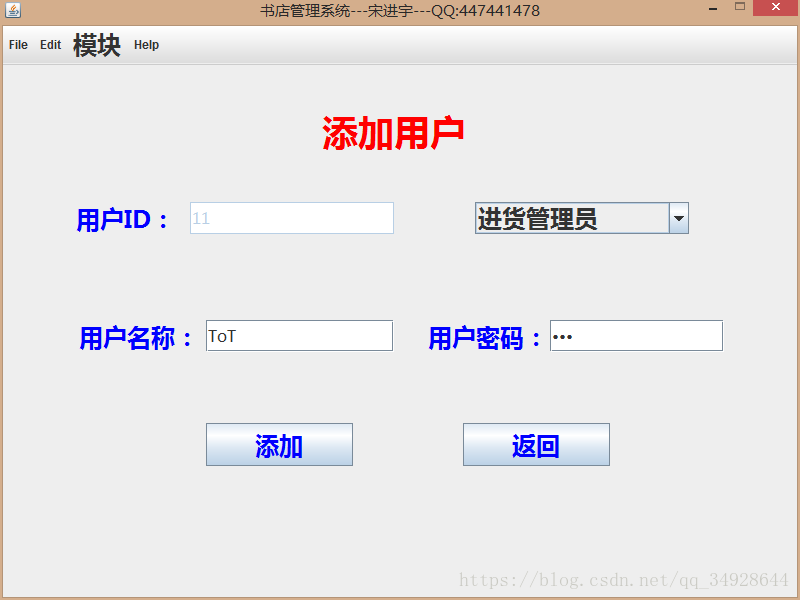
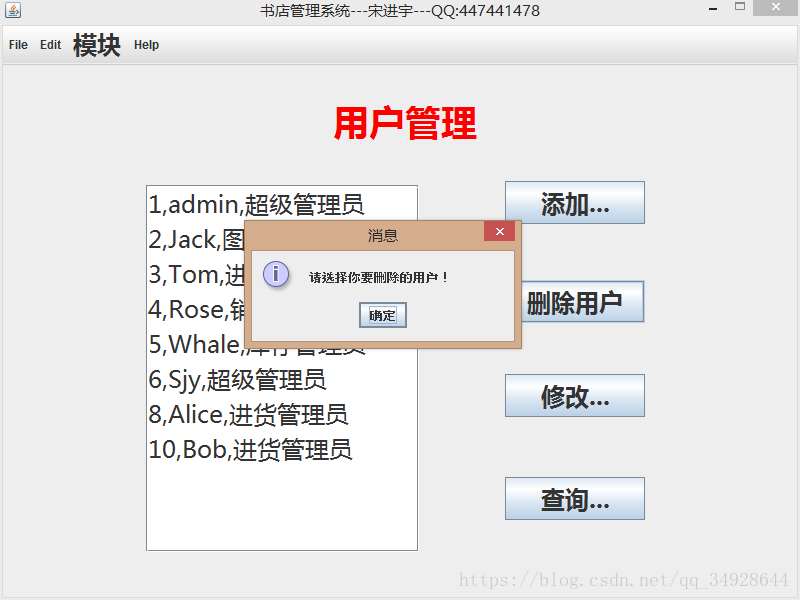
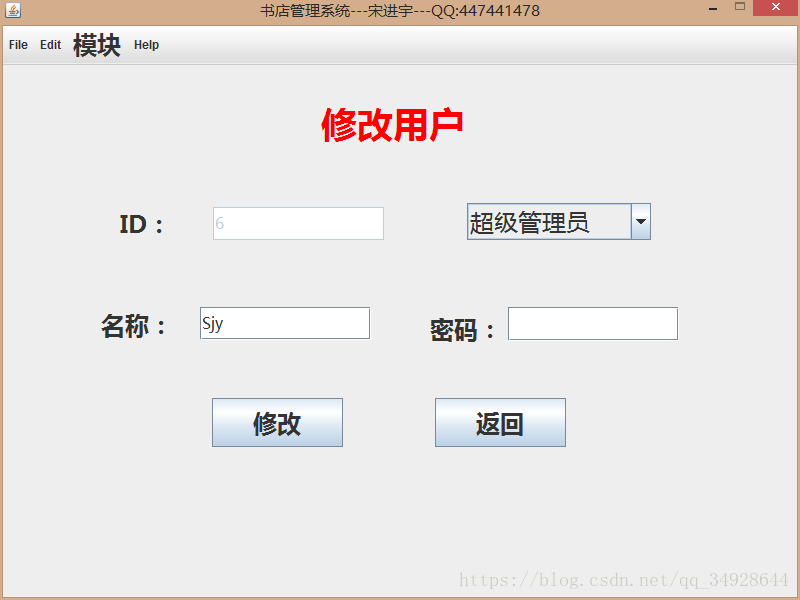
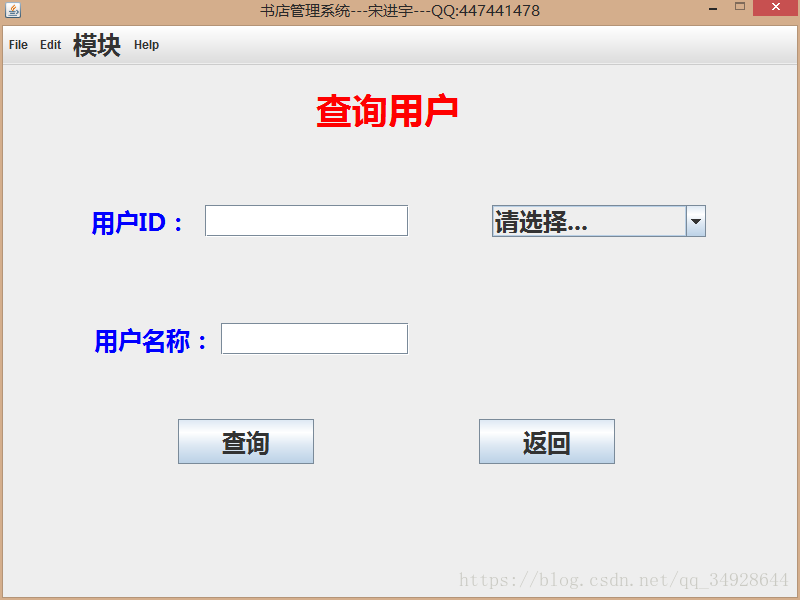
包:
关键代码:
DAO接口:
package cn.hncu.booksManagementSystem.user.dao.dao;
import java.util.Collection;
import cn.hncu.booksManagementSystem.user.vo.UserModel;
import cn.hncu.booksManagementSystem.user.vo.UserQueryModel;
/**
* Time:2018/4/16
* Description:对UserModel类型的数据进行 增、删、改、查(单、全、条件)
* @author 宋进宇
*/
public interface UserDAO {
/**
* 添加一个 UserModel 对象
* @param user 被添加的对象
* @return true:添加成功,false:添加失败
*/
public boolean add(UserModel user);
/**
* 删除一个 UserModel 对象
* @param user 被删除的对象
* @return true:删除成功,false:删除失败
*/
public boolean delete(UserModel user);
/**
* 修改一个 UserModel 对象
* @param user 被修改的对象
* @return true:修改成功,false:修改失败
*/
public boolean update(UserModel user);
/**
* 通过用户id查询
* @param id 被查询对象的编号id
* @return 返回UserModel的实例对象表示查找到,返回null表示没有查找到相应id的用户
*/
public UserModel getUserModelById(String id);
/**
* 查询所有用户
* @return 所有用户的集合
*/
public Collection<UserModel> getAll();
/**
* 通过查询值对象,查询符合条件的用户信息
* @param uqm 查询值对象
* @return 符合条件的用户信息的集合
*/
public Collection<UserModel> getUsersByCondition(UserQueryModel uqm);
}
DAO接口的实现类:
package cn.hncu.booksManagementSystem.user.dao.impl;
import java.util.ArrayList;
import java.util.Collection;
import cn.hncu.booksManagementSystem.common.enums.XxxIdEnum;
import cn.hncu.booksManagementSystem.user.dao.dao.UserDAO;
import cn.hncu.booksManagementSystem.user.vo.UserModel;
import cn.hncu.booksManagementSystem.user.vo.UserQueryModel;
import cn.hncu.booksManagementSystem.utils.FileIoUtil;
import cn.hncu.booksManagementSystem.utils.GeneratorXxxID_Util;
public class UserDAO_FileImpl implements UserDAO {
// 数据源的路径+名称
private final static String FILE_NAME = "./data/user.info";
// 采用单例模式,实现内存中就一个 UserDAO_File 类的实例化对象
private static UserDAO_FileImpl udf = null;
private static Collection<UserModel> col = FileIoUtil.readFromFile(FILE_NAME);
// 初始化一个超级管理员信息
static {
if (col.size()==0) {
Collection<UserModel>cc = new ArrayList<UserModel>();
UserModel user = new UserModel();
user.setId(GeneratorXxxID_Util.getXxxId(XxxIdEnum.USER));
user.setName("admin");
user.setPwd("123");
user.setType(1);
cc.add(user);
FileIoUtil.write2File(cc, FILE_NAME);
}
}
/**
* 通过工厂方式获取UserDAO_File对象,实现单例
* @return UserDAO_File的实例
*/
public synchronized static UserDAO_FileImpl getUserDAO_File() {
if (udf == null) {
udf = new UserDAO_FileImpl();
}
return udf;
}
// 私有化构造函数,保持单例
private UserDAO_FileImpl() {
col=getAll();
}
@Override
public boolean add(UserModel user) {
//如果被添加的对象为空直接返回null;
if (user==null) {
return false;
}
UserModel queryUser = getUserModelById(user.getId());
if (queryUser != null) {// 如果找到了说明该用户已存在
return false;
}
col.add(user);
boolean boo = FileIoUtil.write2File(col, FILE_NAME);
if (boo) {
// 能到这里说明已经成功添加,更新下一个user的Id
GeneratorXxxID_Util.updateXxxId(XxxIdEnum.USER);;
return true;
}
return false;
}
@Override
public boolean delete(UserModel user) {
//如果删除的对象为空,则返回false;
if (user==null) {
return false;
}
boolean boo = col.remove(user);
return boo && FileIoUtil.write2File(col, FILE_NAME);
}
@Override
public boolean update(UserModel user) {
if (user==null) {
return false;
}
ArrayList<UserModel> res = (ArrayList<UserModel>) col;
boolean boo = false;
for(int i = 0 ; i<res.size(); i++) {
if (res.get(i).equals(user)) {
res.set(i, user);
boo = true;
break;
}
}
col = res;
return boo && FileIoUtil.write2File(col, FILE_NAME);
}
@Override
public UserModel getUserModelById(String id) {
if (id==null) {
return null;
}
for (UserModel u : col) {
if (u.getId().equals(id)) {
return u;
}
}
return null;
}
@Override
public Collection<UserModel> getAll() {
if (col.size() > 0) {
return col;
}
return FileIoUtil.readFromFile(FILE_NAME);
}
@Override
public Collection<UserModel> getUsersByCondition(UserQueryModel uqm) {
//如果查询对象为null,则查询所有
if (uqm==null) {
return col;
}
Collection<UserModel> resCol = new ArrayList<UserModel>();
for (UserModel u : col) {
// 卫兵1查id
// 判断是否是有效的查询条件
if (uqm.getId() != null && uqm.getId().trim().length() > 0) {
// 反逻辑
if (!u.getId().equals(uqm.getId().trim())) {
continue;
}
}
// 卫兵2查姓名
// 判断是否是有效的查询条件
if (uqm.getName() != null && uqm.getName().length() > 0) {
// 模糊查询
if (!u.getName().contains(uqm.getName().trim())) {
continue;
}
}
// 卫兵3查类型
// 判断是否是有效的查询条件
if (uqm.getType() != 0) {
if (u.getType() != uqm.getType()) {
continue;
}
}
resCol.add(u);
}
return resCol;
}
}
生产DAO接口的工厂:
package cn.hncu.booksManagementSystem.user.dao.factory;
import cn.hncu.booksManagementSystem.user.dao.dao.UserDAO;
import cn.hncu.booksManagementSystem.user.dao.impl.UserDAO_FileImpl;
public class UserDAO_Factory {
//封掉构造函数
private UserDAO_Factory() {
}
/**
* 通过工厂方法,获得一个 UserDAO 的实现类
* @return 这是 UserDAO 的一个实现类
*/
public static UserDAO getInstancnOfUserDAO() {
return UserDAO_FileImpl.getUserDAO_File();
}
}
user类型的枚举:
package cn.hncu.booksManagementSystem.common.enums;
public enum UserTypeEnum {
ADMIN(1,"超级管理员"),
BOOK(2,"图书管理员"),
IN(3,"进货管理员"),
OUT(4,"销售管理员"),
STOCK(5,"库存管理员")
;
final private int type; //类型
final private String name; //名称
private UserTypeEnum(int type,String name) {
this.type = type;
this.name = name;
}
public int getType() {
return type;
}
public String getName() {
return name;
}
/**
* 通过 type 获取所对应的 name ,如果没有所对应的name则抛出异常
* @param type 枚举中的类型
* @return 该 type 所对应的 name
* @throws IllegalArgumentException 抛出异常,表示没有对应的 name
*/
public static String getNameByType(int type) throws IllegalArgumentException {
//通过 foreach 遍历
for (UserTypeEnum ute : UserTypeEnum.values()) {
if (ute.type==type) {
return ute.name;
}
}
throw new IllegalArgumentException("No name corresponding to this "+type);
}
/**
*
* 通过 name 获取所对应的 type ,如果没有所对应的type则抛出异常
* @param name 枚举中的 name
* @return 该 name 所对应的 type
* @throws IllegalArgumentException 抛出异常,表示没有对应的 type
*/
public static int getTypeByName(String name)throws IllegalArgumentException {
//通过 foreach 遍历
for (UserTypeEnum ute : UserTypeEnum.values()) {
if (ute.name.equals(name)) {
return ute.type;
}
}
throw new IllegalArgumentException("No Type corresponding to this "+name);
}
/**
* 获取所有类型的中文表示方式
* @return 存放所有类型的中文表示方式的字符串数组
*/
public static String[] getAllName() {
String names[] = new String[UserTypeEnum.values().length];
int i = 0;
for (UserTypeEnum ute : UserTypeEnum.values()) {
names[i++] = ute.getName();
}
return names;
}
}