大家不是一直吐槽C/C++的指针都要程序员自己管理嘛,不用担心啦 智能指针就可以解决这样的问题【说是智能其实就是一个模板类,声明周期结束析构释放】。
auto_ptr 是c++ 98定义的智能指针模板,其定义了管理指针的对象,可以将new 获得(直接或间接)的地址赋给这种对象。当对象过期时,其析构函数将使用delete 来释放内存!【后面介绍C++11新增的智能指针】
头文件: #include <memory>
用 法: auto_ptr<类型> 变量名(new 类型)
#include <memory>
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
#include <exception>
#include <Windows.h>
using namespace std;
class Temp
{
public:
Temp() {
cout << "构造函数" << endl;
debug = 0;
}
Temp(int val) {
cout << "构造函数" << endl;
debug = val;
}
~Temp() {
cout << "析构函数" << endl;
}
int getDebug()const {
return debug;
}
private:
int debug;
};
void
auto_memery_destroy()
{
auto_ptr<Temp> str_ptr1(new Temp(2));
auto_ptr<Temp> str_ptr2;
cout << str_ptr1->getDebug() << endl;
str_ptr2 = str_ptr1;
cout << "在str_ptr2 = str_ptr1之后" << endl;
cout << "str_ptr1: " << str_ptr1.get() << endl;
cout << "str_ptr2: " << str_ptr2.get() << endl;
return;
}
int
main(int argc, const char* argv[])
{
auto_memery_destroy();
system("pause");
return 0;
}
效果:
我们来看看为什么str_ptr2 = str_ptr1;之后 str_ptr1把它指向的地址交给了 str_ptr2
str_ptr1为什么会变成 NULL ,看看源码就知道了
auto_ptr& operator=(auto_ptr& _Right) noexcept {
reset(_Right.release());
return *this;
}
void reset(_Ty* _Ptr = nullptr) noexcept { // destroy designated object and store new pointer
if (_Ptr != _Myptr) {
delete _Myptr;
}
_Myptr = _Ptr;
}
_Ty* release() noexcept {
_Ty* _Tmp = _Myptr;
_Myptr = nullptr;
return _Tmp;
}
使用建议:
1.尽可能不要将auto_ptr 变量定义为全局变量或指针
扫描二维码关注公众号,回复:
12035944 查看本文章
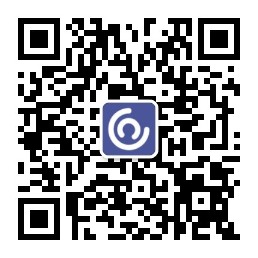
2.除非自己知道后果,不要把auto_ptr 智能指针赋值给同类型的另外一个 智能指针
3.C++11 后auto_ptr 已经被“抛弃”,已使用unique_ptr替代!