一.redis.php
<?php //实例化 $redis = new Redis(); //连接服务器 $redis->connect("localhost"); //授权 $redis->auth("lamplijie"); //相关操作 $redis->set("name","lampbrother"); $data = $redis->key("*"); var_dump($data);
页面显示结果:
array(1) { [0]=> string(4) "name" }
二.简单回顾redis的四种数据类型
a.string:最简单的数据类型
set user:001:name lijie set user:001:age 20
b.hash:可以当做表,hash table,比string速度快
hset user:001 name lamp age 20 hset user:001 sex nan hset user:002 name lijie age 20 hgetall user:001
c.list:栈、队列
d.set:并集、交集、差集
e.zset:set升级版,多了一个顺序
三.小型的用户管理系统(用户的增删改查、分页、登陆退出、加关注)
扫描二维码关注公众号,回复:
1192031 查看本文章
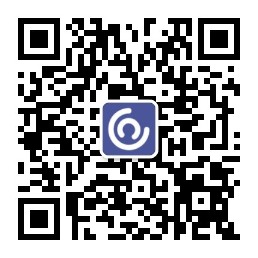
redis.php
<?php //实例化 $redis = new Redis(); //连接服务器 $redis->connect("localhost"); //授权 $redis->auth("lamplijie");
add.php
<form action="reg.php" method="post"> 用户名:<input type="text" name="username"/><br/> 密码:<input type="password" name="password"/><br/> 年龄:<input type="text" name="age"/><br/> <input type="submit" value="注册"/> <input type="reset" value="重新填写"/> </form>
reg.php
<?php require("redis.php"); $username = $_POST['username']; $password = md5($_POST['password']); $age = $_POST['age']; echo $uid = $redis->incr("userid"); $redis->hmset("user:".$uid,array("uid"=>$uid,"username"=>$username,"password"=>$password,"age"=>$age)); $redis->rpush("uid",$uid); $redis->set("username:".$username,$uid); header("location:list.php");list.php
<a href="add.php">注册</a> <?php require("redis.php"); if(!empty($_COOKIE['auth'])){ $id = $redis->get("auth:".$COOKIE['auth']); $name = $redis->hget("user:".$id,"username"); ?> 欢迎您,<?php echo $name?>,<a href="logout.php">退出</a> <?php }else{ ?> <a href="login.php">登陆</a> <?php } //用户总数 $count = $redis->lsize("uid"); //页大小 $page_size = 3; //当前页码 $page_num = (!empty($_GET['page']))?$_GET['page']:1; //页总数 $page_count = ceil($count/$page_size); $ids = $redis->lrange("uid",($page_num-1)*$page_size,(($page_num-1)*$page_size+$page_size-1)); //var_dump($ids); /* for($i=1;$i<=($redis->get("userid"));$i++) { $data[] = $redis->hgetall("user:".$i); }*/ foreach($ids as $v){ $data[] = $redis->hgetall("user:".$v); } //var_dump($data); //$data = array_filter($data); ?> <table border="1"> <tr> <th>uid</th> <th>username</th> <th>age</th> <th>操作</th> </tr> <?php foreach($data as $v){?> <tr> <td><?php echo $v['uid']?></td> <td><?php echo $v['username']?></td> <td><?php echo $v['age']?></td> <td> <a href="del.php?id=<?php echo $v['uid']?>">删除</a> <a href="mod.php?id=<?php echo $v['uid']?>">编辑</a> <?php if(!empty($_COOKIE['auth']) && $id!=$v['uid']){?> <a href="addfans.php?id=<?php echo $v['uid']?>&uid=<?php echo $id?>">加关注</a> <?php } ?> </td> </tr> <?php}?> <tr> <td colspan="4"> <a href="?page=<?php echo(($page_num-1)<=1)?1:($page_num-1) ?>">上一页</a> <a href="?page=<?php echo(($page_num+1)>=$page_count)?$page_count:($page_num+1) ?>">下一页</a> <a href="?page=1">首页</a> <a href="?page=<?php echo $page_count ?>">尾页</a> 当前<?php echo $page_num ?>页 总共<?php echo $page_count ?>页 总共<?php echo $count ?>个用户 </td> </tr> </table> <table border=1> <caption>我关注了谁</caption> <?php $data = $redis->smembers("user:".$id.":following");?> foreach($data as $v) { $row = $redis->hgetall("user:".$v); <tr> <td><?php echo $row['uid']?></td> <td><?php echo $row['username']?></td> <td><?php echo $row['age']?></td> </tr> <?php } ?> </table> <table border=1> <caption>我的粉丝</caption> <?php $data = $redis->smembers("user:".$id.":followers"); foreach($data as $v) { $row = $redis->hgetall("user:".$v); ?> <tr> <td><?php echo $row['uid']?></td> <td><?php echo $row['username']?></td> <td><?php echo $row['age']?></td> </tr> <?php } ?> </table>
del.php
<?php require("redis.php"); $uid = $_GET['id']; $redis->del("user:".$uid); $redis->lrem("uid",$uid); header("localhost:list.php");mod.php
<?php require("redis.php"); $uid = $_GET['id']; $data = $redis->hgetall("user:".$uid); ?> <form action="doedit.php" method="post"> <input type="hidden" value="<?php echo $data['uid']?>" name="uid"/> 用户名:<input type="text" name="username" value="<?php echo $data['username']?>"/><br/> 年龄:<input type="text" name="age" value="?php echo $[data['age']?>"/><br/> <input type="submit" value="修改"/> <input type="reset" value="重新填写"/> </form>
doedit.php
<?php $uid = $_POST['uid']; $username = $_POST['username']; $age = $_POST['age']; $a = $redis->hmset("user:".$uid,array("username"=>$username,"age"=>$age)); if($a) { header("location:list.php"); }else { header(location:mod.php?id=".$uid); }
login.php
<?php require("redis.php"); $username = $_POST['username']; $pass = $_POST['password']; $id = $redis->get("username:".$username); if(!empty($id)) { $password = $redis->hget("user:".$id,"password"); if(md5($pass) == $password) { $auth = md5(time().$username.rand()); $redis->set("auth:".$auth,$id); setcookie("auth", $auth, time() + 86400); header("location:list.php"); } } ?> <form action="" method="post"> 用户名:<input type="text" name="username"/><br/> 密码:<input type="password" name="password"/><br/> <input type="submit" value="登陆"/> </form>
logout.php
<?php setcookie("auth","",time()-1); header("location:list.php");
addfans.php
<?php $id = $_GET['id']; $uid = $_GET['uid']; require("redis.php"); $redis->sadd("user:".$uid.":following",$id); $redis->sadd("user:".$id.":followers",$uid); header("location:list.php");当然,采用sdiff user:1:following user:2:following语句,用户1可以向用户2推荐关注(即用户1的关注与用户2的关注的差集)。 说明:本文是我网上学习LAMP兄弟连李捷老师《NoSQL数据库之Redis数据库管理》的学习笔记。