单链表是一种非常重要的数据结构,它的优点是采用指针方式来增减结点,非常方便(它只用改变指针的指向即可,不需要移动结点);缺点是不能进行随机访问,只能顺着指针的方向进行顺序访问。
下面,介绍单例表的创建、打印和反转。
1、定义单链表
struct ListNode {
int val;
ListNode *next;
ListNode(int x) : val(x), next(NULL) {
}
};
2、创建单链表
ListNode* createLinkedList(int arr[], int n) {
if (n == 0) {
return NULL;
}
ListNode* head = new ListNode(arr[0]);
ListNode* curNode = head;
for (int i = 1; i < n; i++) {
curNode->next = new ListNode(arr[i]);
curNode = curNode->next;
}
return head;
}
3、打印单链表
void printLinkedList(ListNode* head) {
ListNode* curNode = head;
while (curNode != NULL) {
cout << curNode->val << "->";
curNode = curNode->next;
}
cout << "NULL" << endl;
}
4、反转单链表
//该方法是采用头插法,来反转单链表
class Solution {
public:
//不带头结点,反转单链表(采用头插法实现)
ListNode* reverseList(ListNode* head) {
ListNode* pre = NULL, *next=NULL;
ListNode* cur = head;
while (cur != NULL) {
next = cur->next;
cur->next = pre;
pre = cur;
cur = next;
}
return pre;
}
};
5、删除单链表
void deleteLinkdList(ListNode* head) {
ListNode* curNode = head;
while (curNode != NULL) {
ListNode* delNode = curNode;
curNode = curNode->next;
delete delNode;
delNode = NULL;
}
}
6、 完整代码如下:
//singleLink.cpp
#include <iostream>
#include <vector>
#include <cassert>
using namespace std;
struct ListNode {
int val;
ListNode *next;
ListNode(int x) : val(x), next(NULL) {
}
};
ListNode* createLinkedList(int arr[], int n) {
if (n == 0) {
return NULL;
}
ListNode* head = new ListNode(arr[0]);
ListNode* curNode = head;
for (int i = 1; i < n; i++) {
curNode->next = new ListNode(arr[i]);
curNode = curNode->next;
}
return head;
}
void printLinkedList(ListNode* head) {
ListNode* curNode = head;
while (curNode != NULL) {
cout << curNode->val << "->";
curNode = curNode->next;
}
cout << "NULL" << endl;
}
void deleteLinkdList(ListNode* head) {
ListNode* curNode = head;
while (curNode != NULL) {
ListNode* delNode = curNode;
curNode = curNode->next;
delete delNode;
delNode = NULL;
}
}
class Solution {
public:
//不带头结点,反转单链表(采用头插法实现)
ListNode* reverseList(ListNode* head) {
ListNode* pre = NULL, *next=NULL;
ListNode* cur = head;
while (cur != NULL) {
next = cur->next;
cur->next = pre;
pre = cur;
cur = next;
}
return pre;
}
};
int main() {
int arr[] = {
1, 2, 3, 4, 5 };
int n = sizeof(arr) / sizeof(int);
ListNode* head = createLinkedList(arr, n);
printLinkedList(head);
ListNode* head2 = Solution().reverseList(head);
printLinkedList(head2);
deleteLinkdList(head2);
system("pause");
return 0;
}
7、效果如下:
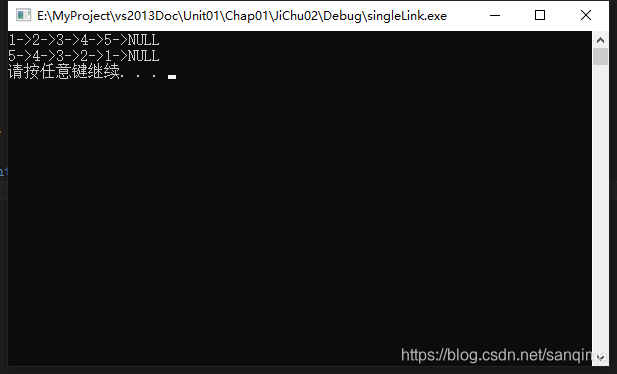