工具:
- IDEA
- MySQL8.0或之前版本
JDBC是Java访问数据库的标准规范,可以为不同的关系型数据库提供统⼀访问,它由一组用Java编写的接口和类组成。
JDBC需要
连接驱动
,驱动是两个设备要进行通信,满足一定通信数据格式,数据格式由设备提供商规定,设备提供商为设备提供驱动软件,通过软件可以与该设备进行通信。
今天我们使用的是mysql的驱动
mysql-connector-java-8.0.20.jar
(适配MySQL8.0版本),如果是之前版本的MySQL建议使用应用次数较多的
mysql-connector-java-5.1.38.jar
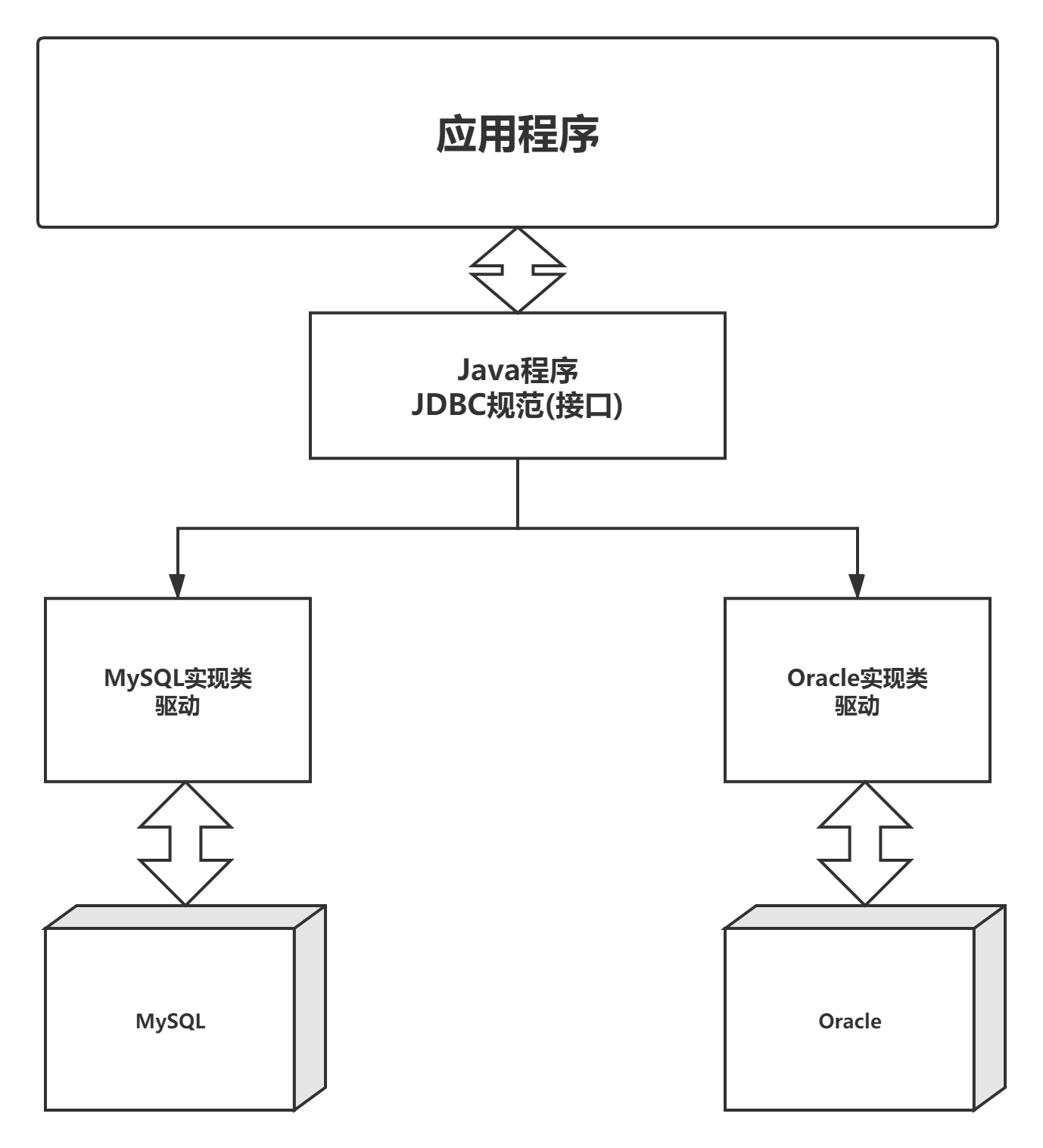
JDBC核心规范:
- DriverManager:⽤于注册驱动
- Connection: 表示与数据库创建的连接
- Statement: 操作数据库sql语句的对象
- ResultSet: 结果集或⼀张虚拟表
JDBC原理
Java提供访问数据库规范称为JDBC
,而生产厂商提供规范的实现类称为驱动
。JDBC是接口,驱动是接口的实现,没有驱动将无法完成数据库连接,从而不能操作数据库!每个数据库厂商都需要提供自己的驱动,用来连接自己公司的数据库,也就是说驱动一般都由数据库生成厂商提供(也就是在我们上边导入的jar包当中)。
开发步骤
- 注册驱动
- 获得连接
- 获得执行sql语句的对象
- 执行sql语句,并返回结果
- 处理结果
- 释放资源
JDBC入门案例
首先熟悉一下JDBC的helloworld,
- 准备MySQL数据:
#创建分类表
create table category(
cid int PRIMARY KEY AUTO_INCREMENT,
cname varchar(100)
);
#初始化数据
insert into category (cname) values('家电');
insert into category (cname) values('服饰');
insert into category (cname) values('化妆品');
建立一个简单的表
2. 导入驱动jar包
在IDEA项目下创建lib目录
在MVN下好jar包并复制,双击新建的lib目录ctrl+v
,jar包就保存到该目录下,之后右键导入的jar包,选择添加到库…
或Add as Library...
,就正式添加完毕了
MySQL8.0连接jdbc方法
package JDBCTest;
import org.junit.Test;
import java.sql.*;
public class JDBCDemo {
public static void main(String[] args) throws ClassNotFoundException, SQLException {
@Test
/**
* 1.注册驱动, 就是把 Driver.class⽂件加载到内存
* mysql8.0之前的版本需要连接的驱动是com.mysql.jdbc.Driver
*/
Class.forName("com.mysql.cj.jdbc.Driver");
/**
* 2.获得连接
* 参数 url : 需要连接数据库的地址 jdbc:mysql://IP地址:端⼝号/要连接的数据库名称
* 参数 user : 连接数据库 使⽤的⽤户名
* 参数 password: 连接数据库 使⽤的密码
*/
String url = "jdbc:mysql://localhost:3306/mydb?characterEncoding=utf8&useSSL=false&serverTimezone=UTC&rewriteBatchedStatments=true";
Connection connection = DriverManager.getConnection(url, "root", "root");
/**
* 3.获取执⾏sql语句的对象
*/
Statement statement = connection.createStatement();
/**
* 4.执行sql语句并返回结果
*/
String sql1 = "select * from category";
ResultSet resultSet = statement.executeQuery(sql1);
/**
* 5.处理结果
*/
while (resultSet.next()) {
int cid = resultSet.getInt("cid");
String cname = resultSet.getString("cname");
System.out.println("cid = " + cid + ", cname = " + cname);
}
/**
* 6.释放资源
*/
resultSet.close();
statement.close();
connection.close();
}
}
JDBC的API详解
- 注册驱动
有两种方法:
①DriverManager.registerDriver(new com.mysql.jdbc.Driver());
②Class.forName("com.mysql.jdbc.Driver");
其中第一种办法不被推荐使用,因为驱动会被注册两次,并且会强烈依赖数据库的驱动jar,通常我们在做开发的时候用的都是第二种方式来注册驱动 - 获得链接
static Connection getConnection(String url, String user, String password)
:试图建立到给定数据库 URL 的连接
使用参数说明:
- url 需要连接数据库的位置(网址)
- user用户名 (要区别于数据库表名)
- password 密码
eg:getConnection("jdbc:mysql://localhost:3306/database", "root", "root");
URL是SUN公司和数据库厂商的一个协议
jdbc:mysql://localhost:3306/database
协议子协议 IP:端口号数据库
- mysql数据库:
jdbc:mysql://localhost:3306/database
或者jdbc:mysql:///database
(默认本机连接)- oracle数据库:
jdbc:oracle:thin:@localhost:1521:sid
-
java.sql.Connection接口:一个连接
接口的实现在数据库驱动中,所有与数据库交互都是基于连接对象的。 -
java.sql.Statement接口: 操作sql语句,并返回相应结果
String sql = "某SQL语句";
获取Statement语句执⾏平台:Statement stmt =con.createStatement();
常用方法:
int executeUpdate(String sql);
--执行insert update delete语句.
ResultSet executeQuery(String sql);
--执行select语句.
boolean execute(String sql);
--仅当执行select并且有结果时才返回true,执行其他的语句返回false.
- 处理结果集(注:执行insert、update、delete无需处理)
ResultSet
实际上就是一张二维的表格,我们可以调用其boolean next()
方法指向某行记录,当第一次调用next()
方法时,便指向第一行记录的位置,这时就可以使用ResultSet
提供的getXXX(int col)
方法来获取指定列的数据:(与数组索引从0开始不同,这里索引从1开始)
rs.next();//指向第⼀⾏
rs.getInt(1);//获取第⼀⾏第⼀列的数据
常用方法:
Object getObject(int index)
/Object getObject(String name)
获得任意对象String getString(int index)
/String getString(String name)
获得字符串int getInt(int index)
/int getInt(String name)
获得整型double getDouble(int index)
/double getDouble(String name)
获得双精度浮点型
- 释放资源
与IO流⼀样,使用后的东西都需要关闭!关闭的顺序是先得到的后关闭,后得到的先关闭。
rs.close();
stmt.close();
con.close();
JDBC增删改查操作
- 增:
@Test
public void testJDBC2() throws SQLException, ClassNotFoundException {
/**
* JDBC 完成 记录的插⼊
* 1.注册驱动
* 2.获得连接
* 3.获得执⾏sql语句的对象
* 4.执⾏sql语句, 并返回结果
* 5.处理结果
* 6.释放资源
*/
Class.forName("com.mysql.jdbc.Driver");
String url = "jdbc:mysql://localhost:3306/mydb";
Connection conn = DriverManager.getConnection(url, "root",
"root");
Statement stat = conn.createStatement();
String sql = "insert into category(cname) values('测试')";
int result = stat.executeUpdate(sql);
System.out.println("result = " + result);
stat.close();
conn.close();
}
- 删:
@Test
public void testJDBC4() throws SQLException, ClassNotFoundException {
/**
* JDBC 完成 记录的删除
* 1.注册驱动
* 2.获得连接
* 3.获得执⾏sql语句的对象
* 4.执⾏sql语句, 并返回结果
* 5.处理结果
* 6.释放资源
*/
Class.forName("com.mysql.jdbc.Driver");
String url = "jdbc:mysql://localhost:3306/mydb";
Connection conn = DriverManager.getConnection(url, "root",
"root");
Statement stat = conn.createStatement();
String sql = "delete from category where cid=4";
int result = stat.executeUpdate(sql);
System.out.println("result = " + result);
stat.close();
conn.close();
}
- 改:
@Test
public void testJDBC3() throws SQLException, ClassNotFoundException {
/**
* JDBC 完成 记录的更新
* 1.注册驱动
* 2.获得连接
* 3.获得执⾏sql语句的对象
* 4.执⾏sql语句, 并返回结果
* 5.处理结果
* 6.释放资源
*/
Class.forName("com.mysql.jdbc.Driver");
String url = "jdbc:mysql://localhost:3306/mydb";
Connection conn = DriverManager.getConnection(url, "root",
"root");
Statement stat = conn.createStatement();
String sql = "update category set cname='测试2' where cid=4";
int result = stat.executeUpdate(sql);
System.out.println("result = " + result);
stat.close();
conn.close();
}
- 查:
@Test
public void testJDBC5() throws SQLException, ClassNotFoundException {
/**
* JDBC 完成 记录的删除
* 1.注册驱动
* 2.获得连接
* 3.获得执⾏sql语句的对象
* 4.执⾏sql语句, 并返回结果
* 5.处理结果
* 6.释放资源
*/
// 通过id 查询数据
Class.forName("com.mysql.jdbc.Driver");
String url = "jdbc:mysql://localhost:3306/mydb";
Connection conn = DriverManager.getConnection(url, "root",
"root");
Statement stat = conn.createStatement();
String sql = "select * from category where cid = 3";
ResultSet rs = stat.executeQuery(sql);
if (rs.next()) {
int cid = rs.getInt("cid");
String cname = rs.getString("cname");
System.out.println("cid = " + cid + ",cname = " +cname);
} else {
System.out.println("数据没有查到");
}
rs.close();
stat.close();
conn.close();
}
JDBC工具类
为啥要用到工具类呢?
JDBC操作中有大量的重复性编码,为了减少不必要的工作量,引入了工具类,获得数据库连接
操作,将在以后的增删改查所有功能中都存在,可以封装工具类JDBCUtils。提供获取连接对象的方法,从而达到代码的重复利用。
该工具类提供方法: public static Connection getConnection()
- 右键src,新建一个文件,随便命名
MySQL8.0用户
jdbc.driver=com.mysql.jdbc.Driver
jdbc:mysql://localhost:3306/mydb?characterEncoding=utf8&useSSL=false&serverTimezone=UTC&rewriteBatchedStatments=true
jdbc.user=root
jdbc.password=root
MySQL8.0以前版本的用户
jdbc.driver=com.mysql.jdbc.Driver
jdbc.url=jdbc:mysql://localhost:3306/mydb
jdbc.user=root
jdbc.password=root
- 建立JDBC工具类
public class JDBCUtils2 {
private static String driver;
private static String url;
private static String user;
private static String password;
static {
try{
//使⽤类加载器, 读取配置⽂件
InputStream is=
JDBCUtils2.class.getClassLoader().getResourceAsStream("jdbc.properties");
Properties prop=new Properties();
prop.load(is);
driver=prop.getProperty("jdbc.driver");
url=prop.getProperty("jdbc.url");
user=prop.getProperty("jdbc.user");
password=prop.getProperty("jdbc.password");
//注册驱动
Class.forName(driver);
}catch(IOException e){
e.printStackTrace();
}catch(ClassNotFoundException e){
e.printStackTrace();
}
}
/**
* 返回连接对象 Connection
*/
public static Connection getConnection()throws SQLException{
Connection conn=DriverManager.getConnection(url,user,password);
return conn;
}
/**
* 释放资源
*/
public static void close(ResultSet rs,Statement stat,Connection
conn)throws SQLException{
if(rs!=null){
rs.close();
}
if(stat!=null){
stat.close();
}
//看Connection来⾃哪⾥, 如果Connection是从连接池⾥⾯获得的, close()⽅法其
实是归还;如果Connection是创建的,就是销毁
if(conn!=null){
conn.close();
}
}
}
- 使用JDBC工具类 完成查询
@Test
public void testJDBC6() throws SQLException {
/**
* 使⽤JDBC⼯具类, 完成查询所有分类
* 1.通过JDBC⼯具类, 获得连接
* 2.获得执⾏sql语句的对象
* 3.执⾏sql语句, 并返回结果
* 4.处理结果
* 5.释放资源
*/
Connection conn = JDBCUtils2.getConnection();
Statement stat = conn.createStatement();
ResultSet rs = stat.executeQuery("select * from category");
while (rs.next()) {
int cid = rs.getInt("cid");
String cname = rs.getString("cname");
System.out.println("cid = " + cid + ",cname = " + cname);
}
JDBCUtils2.close(rs, stat, conn);
}