一.简介
RestTemplate 访问服务端时需要明确服务端地址,在服务端宕机、地址变化后则需要大量修改。微服务中使用注册中心对所有微服务管理,微服务启动后把自己注册到注册中心,注册中心以统一的方式暴露服务。
二.定义Eureka服务端
1.统一定义依赖模块
<groupId>com.vincent</groupId>
<artifactId>demo</artifactId>
<packaging>pom</packaging>
<version>1.0-SNAPSHOT</version>
<modules>
<module>eureka</module>
</modules>
<properties>
<jdk.version>1.8</jdk.version>
<spring-boot-dependencies.version>1.5.20.RELEASE</spring-boot-dependencies.version>
<spring-cloud-dependencies.version>Edgware.RELEASE</spring-cloud-dependencies.version>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>${spring-boot-dependencies.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>${spring-cloud-dependencies.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
<dependencyManagement>
2.建立服务端模块
<parent>
<artifactId>demo</artifactId>
<groupId>com.vincent</groupId>
<version>1.0-SNAPSHOT</version>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-server</artifactId>
</dependency>
</dependencies>
3.编写application.yml 配置文件
server:
port: 7001
context-path: /service-eureka
eureka:
client:
#eureka服务端本身也是一个微服务,设置微服务不向eureka注册中心注册服务
register-with-eureka: false
#设置当前的服务不需要从eureka注册中心获取服务注册信息
fetch-registry: false
#注册中心服务地址(不能乱写),其注册中心服务地址为:schema://host-name:port/context-path/eureka,eureka是固定的注册中心访问映射
service-url:
defaultZone: http://localhost:7001/service-eureka/eureka
server:
eviction-interval-timer-in-ms: 60000 #设置对无用微服务清理时间间隔,默认是60s清理一次
当运行清理工作时将有如下输出:
2020-09-05 18:58:22.433 INFO 13108 --- [a-EvictionTimer] c.n.e.registry.AbstractInstanceRegistry : Running the evict task with compensationTime 0ms
4.编写程序启动类并运行
package com.vincent;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer;
@SpringBootApplication
@EnableEurekaServer
public class EureakApp {
public static void main(String[] args) {
SpringApplication.run(EureakApp.class,args);
}
}
5.访问http://localhost:7001/service-eureka
三.注册微服务
1.引入Eureka客户端
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
2.修改application.yml 配置Eureka客户端
server:
port: 8001
context-path: /service-user
spring:
application:
name: service-user #定义微服务名称
eureka:
client:
service-url:
#注册中心地址,Eureka采用HA机制则使用","分割多个注册中心
defaultZone: http://localhost:7001/service-eureka/eureka
instance:
#注册到注册中心的服务依靠心跳机制与Eureka保持联系,默认的心跳时间30s
lease-renewal-interval-in-seconds: 30
3.编写启动类并启动
扫描二维码关注公众号,回复:
11600141 查看本文章
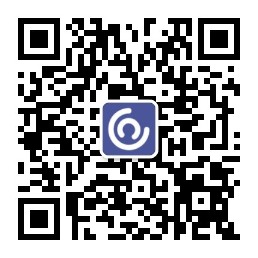
package com.vincent;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
@SpringBootApplication
@EnableEurekaClient
public class ServiceApp {
public static void main(String[] args) {
SpringApplication.run(ServiceApp.class,args);
}
}
4.http://localhost:7001/service-eureka
将能看到注册到注册中心的服务
四.Eureak HA 机制
Eureka 是微服务架构的核心,如果Eureka出现问题则所有服务都将瘫痪,可采用Eureka集群模式实现Eureka的高可用。集群模式需要让任意一台Eureka机器向其他Eureka机器注册。
1.Eureak 模块增加打包配置
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<mainClass>com.vincent.EureakApp</mainClass>
</configuration>
<executions>
<execution>
<goals>
<goal>repackage</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
2.修改application.yml 配置文件
server:
port: 7001
context-path: /service-eureka
eureka:
client:
#eureka服务端本身也是一个微服务,设置微服务不向eureka注册中心注册服务
register-with-eureka: false
#设置当前的服务不需要从eureka注册中心获取服务注册信息
fetch-registry: false
#注册中心服务地址(不能乱写),其注册中心服务地址为:schema://host-name:port/context-path/eureka,eureka是固定的注册中心访问映射
service-url:
#当前的eureka服务向其他的eureka主机注册
defaultZone: http://localhost:7002/service-eureka/eureka,http://localhost:7003/service-eureka/eureka
3.新增配置文件application-7002.yml,application-7003.yml配置文件
server:
port: 7002
eureka:
client:
register-with-eureka: false
fetch-registry: false
service-url:
defaultZone: http://localhost:7001/service-eureka/eureka,http://localhost:7003/service-eureka/eureka
server:
port: 7003
eureka:
client:
register-with-eureka: false
fetch-registry: false
service-url:
defaultZone: http://localhost:7001/service-eureka/eureka,http://localhost:7002/service-eureka/eureka
3.打包并在终端分别运行:
java -jar target\eureka-1.0-SNAPSHOT.jar
java -jar target\eureka-1.0-SNAPSHOT.jar --spring.profiles.active=7002
java -jar target\eureka-1.0-SNAPSHOT.jar --spring.profiles.active=7003