Next的作用
我们在定义express中间件函数的时候都会将第三个参数定义为next,
这个next就是我们今天的主角,next函数主要负责将控制权交给下一个中间件,
如果当前中间件没有终结请求,并且next没有被调用,
那么请求将被挂起,后边定义的中间件将得不到被执行的机会。
何时使用Next()
从上边的描述我们已经知道,next函数主要是用来确保所有注册的中间件
被一个接一个的执行,那么我们就应该在所有的中间件中调用next函数,
但有一个特例,如果我们定义的中间件终结了本次请求,那就不应该
再调用next函数,否则就可能会出问题,我们来看段代码:
let express=require('express')
let app=new express()
app.use('/',function(req,res,next){
res.send('Hello World');
console.log('第一个')
next()
})
app.use('/',function(req,res,next){
console.log('第二个')
res.send('rld');
next()
})
app.use('/',function(req,res,next){
console.log('第三个')
res.send('Herld');
next()
})
app.use('/',function(req,res,next){
console.log('第四个')
res.send('Helld');
})
app.listen(8888,function(){
console.log('running.....')
})
执行上面的代码我们在控制台可以得到4条数据打印如下:
第一个
第二个
第三个
第四个
而当我们将next()去掉时只会打印第一个,从而我们就知道了next()的作用就是执行完当前的中间件后,放行允许下一个中间件执行
Next的内部机制
function next(err) {
... //此处源码省略
// find next matching layer
var layer;
var match;
var route;
while (match !== true && idx < stack.length) {
layer = stack[idx++];
match = matchLayer(layer, path);
route = layer.route;
if (typeof match !== 'boolean') {
// hold on to layerError
layerError = layerError || match;
}
if (match !== true) {
continue;
}
... //此处源码省略
}
... //此处源码省略
// this should be done for the layer
if (err) {
layer.handle_error(err, req, res, next);
} else {
layer.handle_request(req, res, next);
}
}
上边就是express中next的源码,从上边的源码可以发现,next函数内部有个while循环,每次循环都会从stack中拿出一个layer,这个layer中包含了路由和中间件信息,然后就会用layer和请求的path就行匹配,如果匹配成功就会执行layer.handle_request,调用中间件函数。但如果匹配失败,就会循环下一个layer(即中间件)。
body-parser中间件
这是node.js的消息体解析中间件,但是这个中间件不会解析multipart body,由于这种消息体很复杂而且也很大。这种multipart的消息体可以用multer,formidable等来完成(mulpipart表示表单的enctype属性)
四个不同的处理方法:
这四个处理方法分别对body的内容采用不同的处理方法:分别是处理json数据、Buffer流数据、文本数据、UTF-8的编码的数据。
- bodyParser.json(options)
- bodyParser.raw(options)
- bodyParser.text(options)
- bodyParser.urlencoded(options)
以下是它的三种用法:
1、底层中间件用法:这将拦截和解析所有的请求;也即这种用法是全局的。
var express = require('express')
var bodyParser = require('body-parser')
var app = express()
// parse application/x-www-form-urlencoded
app.use(bodyParser.urlencoded({ extended: false }))
// parse application/json
app.use(bodyParser.json())
app.use(function (req, res) {
res.setHeader('Content-Type', 'text/plain')
res.write('you posted:\n')
res.end(JSON.stringify(req.body, null, 2))
})
express的use方法调用body-parser实例;且use方法没有设置路由路径;这样的body-parser实例就会对该app所有的请求进行拦截和解析。
2、特定路由下的中间件用法:这种用法是针对特定路由下的特定请求的,只有请求该路由时,中间件才会拦截和解析该请求;也即这种用法是局部的;也是最常用的一个方式。
var express = require('express')
var bodyParser = require('body-parser')
var app = express()
// create application/json parser
var jsonParser = bodyParser.json()
// create application/x-www-form-urlencoded parser
var urlencodedParser = bodyParser.urlencoded({ extended: false })
// POST /login gets urlencoded bodies
app.post('/login', urlencodedParser, function (req, res) {
if (!req.body) return res.sendStatus(400)
res.send('welcome, ' + req.body.username)
})
// POST /api/users gets JSON bodies
app.post('/api/users', jsonParser, function (req, res) {
if (!req.body) return res.sendStatus(400)
// create user in req.body
})
express的post(或者get)方法调用body-parser实例;且该方法有设置路由路径;这样的body-parser实例就会对该post(或者get)的请求进行拦截和解析。
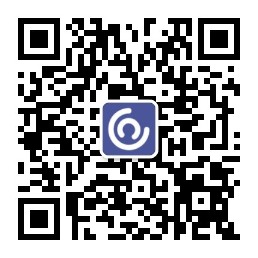
3、设置Content-Type 属性;用于修改和设定中间件解析的body类容类型。
// parse various different custom JSON types as JSON
app.use(bodyParser.json({ type: 'application/*+json' });
// parse some custom thing into a Buffer
app.use(bodyParser.raw({ type: 'application/vnd.custom-type' }));
// parse an HTML body into a string
app.use(bodyParser.text({ type: 'text/html' }));