重点
1.Object
finalize方法、toString方法、equals方法、getClass方法
2.包装类
三种类型(原始类型、包装类、字符串)与6种转换
自动装箱和自动拆箱
3.内部类
练习
1.Object类常用方法
finalize(); 当GC回收没有引用的对象时调用
toString(); hashcode地址码字符串
equals(); 等同于" == "
getClass(); 返回此 Object 的运行时类
2.Object类的toString()和equals()方法
public String toString() {
return getClass().getName() + "@" + Integer.toHexString(hashCode());
}
public boolean equals(Object obj) {
return (this == obj);
}
3.(toString 方法)
class Student{
private int age;
private String name;
public Student(){}
public Student(String name, int age){
this.name = name;
this.age = age;
}
public String toString(){
return name + “ ” + age;
}
}
public class TestStudent{
public static void main(String args[]){
Student stu1 = new Student();
Student stu2 = new Student(“Tom”, 18);
System.out.println(stu1);
System.out.println(stu2);
}
}
null 0
Tom 18
4.(equals)写出下面程序运行的结果
public class TestEquals{
public static void main(String args[]){
String str1 = new String(“Hello”);
String str2 = new String(“Hello”);
System.out.println(str1.equals(str2));
System.out.println(str1 == str2);
}
}
true
false
5.(getClass)写出下面程序运行的结果
class Animal{}
class Dog extends Animal{}
public class TestGetClass{
public static void main(String args[]){
Animal a1 = new Dog();
Animal a2 = new Animal();
System.out.println(a1 instanceof Animal);
System.out.println(a1.getClass() == a2.getClass());
}
}
true
false
6.(包装类,类型转换)
int->Integer: Integer.valueOf();
Integer->int: iNum.intValue(); //intValue是Number类的方法
String->Integer: Integer.valueOf();
Integer->String: String.valueOf();
int->String: String.valueOf();
String->int : Integer.parseInt();
7.(内部类)Java 中的内部类包括
A. 成员内部类
B. 静态内部类
C. 局部内部类
D. 匿名内部类
8.(String)equals()和 " = = "的区别
public class TestString{
public static void main(String args[]){
String str1 = “Hello”;
String str2 = “Hello”;
System.out.println(str1==str2);
System.out.println(str1.equals(str2));
str1 = new String(“Hello”);
str2 = new String(“Hello”);
System.out.println(str1 == str2);
System.out.println(str1.equals(str2));
}
}
true
true
false
true
9.重写equals 和 toString 方法。
@Override
public String toString() {
return name + " " + age;
}
@Override
public boolean equals(Object obj) {
if(obj != null && obj instanceof Worker) {
Workers w = (Worker)obj;
if(this.name == w.getName()) {
return true;
}
}
return false;
}
10.将int类型转化为Long包装类型:
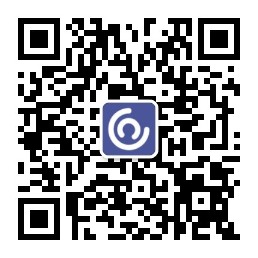
//将int类型转换为Long包装类型
int id = 123;
String s = id + "";
Long lId = Long.valueOf(s);
11.String 直接转换为 int,String间接转换为int
//1.把String直接转换为int
String s1 = "123";
int num1 = Integer.parseInt(s1);
//2.把String间接转换为int
String s2 = "123";
Integer iNum2 = Integer.valueOf(s2);
int num2 = iNum2.intValue();
12.(toString,字符串加法)*基本类型和引用类型进行运算
class Student{
private int age;
private String name;
public Student(){}
public Student(String name, int age){
this.name = name;
this.age = age;
}
public String toString(){
return name + “ ” + age;
}
}
public class TestStudent{
public static void main(String args[]){
Student stu1 = new Student(“tom”, 18);
System.out.println(/1/);
}
}
问:在/1/位置,填入什么代码能编译通过?
A. stu1 + “ ” + 100
B. 100 + “ ” + stu1
C. “ ” + 100 + stu1
13.(Object 类)*
interface IA{
void ma();
}
class MyClass implements IA{
public void ma(){}
public String toString(){
return “MyClass toString()”;
}
}
public class TestMyClass{
public static void main(String args[]){
IA ia = new MyClass();
System.out.println(ia);
}
}
MyClass toString()
14.(匿名内部类,局部内部类)*
interface IA{
void ma();
}
class MyClass {
public static void method(IA ia){
System.out.println(“in method”);
ia.ma();
}
}
public class TestInnerClass{
public static void main(String args[]){
MyClass.method(new IA(){
public void ma(){
System.out.println(“ma in anonymous inner class”);
}
});
class MyMaClass implements IA{
public void ma(){
System.out.println(“ma in local inner class”);
}
}
MyClass.method(new MyMaClass());
}
}
in method
ma in anonymous inner class
in method
ma in local inner class
15.(局部内部类)*有下面代码
class OuterClass{
private int value1 = 100;
private static int value2 = 200;
public void method(int value3){
final int value4 = 400;
class InnerClass{
public void print(){
//1
}
}
}
}
A. System.out.println(value1);
B. System.out.println(value2);
C. System.out.println(value3);
D. System.out.println(value4);
16.已知接口 Light 定义如下:
interface Light{
void shine();
}
定义 Lamp 类:
class Lamp{
public void on(Light light){
light.shine();
}
}
写一个类 TestLamp,部分代码如下:
public class TestLamp{
public static void main(String args[]){
Lamp lamp = new Lamp();
//1
//2
}
}
把 TestLamp 类补充完整,要求: 1) 在//1 处使用局部内部类技术,调用 lamp 的 on 方法要求输出“shine in red” 2) 在//2 处使用匿名内部类技术,调用 lamp 的 on 方法要求输出“shine in yellow”
//1.局部内部类
class MyLamp implements Light{
public void shine(){
System.out.println("shine in red");
}
}
lamp.on(new MyLamp());
//2.匿名内部类
lamp.on(new Light(){
public void shine(){
System.out.println("shine in yellow");
}
});