文章目录
013 unity工程结构
014 封装C#微信中间件
14.1、封装中间件简介
14.2、WeChatComponent.cs文件的编写
using System.Collections;
using System.Collections.Generic;
using System.Runtime.InteropServices;
using UnityEngine;
public class WeChatComponent : MonoBehaviour
{
public string WXAppID = "wxc0d38c38f13506d4";
public string WXAppSecret = "9c789be0809b1353f2fdd08e6fbaae95";
//是否已经注册微信app (appid)
public bool isRegisterToWechat = false;
//用于与安卓层交互的对象
AndroidJavaClass javaClass;
AndroidJavaObject javaActive;
//表示当前游戏运行的activity
public string javaClassStr = "com.unity3d.player.UnityPlayer";
public string javaActiveStr = "currentActivity";
public string WeChatCallObjName = "WeChatComponent";
//事件原型
public delegate void WeChatLogonCallback(WeChatUserData userData);//微信登录回调 userData:null表示登陆了失败 否则表示成功,携带用户公开的信息
public delegate void WeChatShareCallback(string result);//分享结果 result:ERR_OK(由于业务调整,现在始终返回分享成功)
public delegate void WeChatPayCallback(int state);//微信充值回调 result 0:成功 -1失败 -2取消
//用委托定义的变量 可以赋值 注册绑定相应的方法 当委托调用的时候 实际上就是调用注册的方法
public WeChatLogonCallback weChatLogonCallback;
public WeChatShareCallback weChatShareTextCallback, weChatShareImageCallback, weChatShareWebPageCallback;
public WeChatPayCallback weChatPayCallback;
//宏定义
#if UNITY_EDITOR
#elif UNITY_ANDROID
#elif UNITY_IPHONE
#endif
void Start()
{
#if UNITY_EDITOR
#elif UNITY_ANDROID
//初始化 获得项目对应的MainActivity
javaClass = new AndroidJavaClass(javaClassStr);
javaActive = javaClass.GetStatic<AndroidJavaObject>(javaActiveStr);
#elif UNITY_IPHONE
#endif
RegisterAppWechat();
}
/// <summary> 微信初始化:注册ID </summary>
public void RegisterAppWechat()
{
#if UNITY_EDITOR
#elif UNITY_ANDROID
//安卓端已经在java层做了 这里忽略
if (!isRegisterToWechat)
{
javaActive.Call("WechatInit", WXAppID);
}
isRegisterToWechat=true;
#elif UNITY_IPHONE
#else
return;
#endif
}
/// <summary> 是否安装了微信 </summary>
public bool IsWechatInstalled()
{
#if UNITY_EDITOR
return false;
#elif UNITY_ANDROID
return javaActive.Call<bool>("IsWechatInstalled");
#elif UNITY_IPHONE
#else
return false;
#endif
}
/// <summary>微信登录 </summary>
public void WeChatLogon(string state)
{
#if UNITY_EDITOR
#elif UNITY_ANDROID
object[] objs = new object[] { WXAppID, state, WeChatCallObjName, "LogonCallback" };
javaActive.Call("LoginWechat", objs);
#elif UNITY_IPHONE
#endif
}
/// <summary>微信充值</summary>
public void WeChatPay(string appid, string mchid, string prepayid, string noncestr, string timestamp, string sign)
{
#if UNITY_EDITOR
#elif UNITY_ANDROID
//将服务器返回的参数 封装到object数组里 分别是:会话ID,随机字符串,时间戳,签名,支付结果通知回调的物体,物体上的某个回调函数名称
object[] objs = new object[] { appid, mchid, prepayid, noncestr, timestamp, sign, WeChatCallObjName, "WechatPayCallback" };
//调用安卓层的WeiChatPayReq方法 进行支付
javaActive.Call("WeChatPayReq", objs);
#elif UNITY_IPHONE
#endif
}
/// <summary>微信分享网页 </summary>
public void WeChatShare_WebPage(int scene, string url, string title, string content, byte[] thumb)
{
#if UNITY_EDITOR
#elif UNITY_ANDROID
// ShareWebPage(scene, @"http://www.baidu.com/", "这个参数是标题", "这个参数是身体部分",thumb是缩略图);
object[] objs = new object[] { scene, url, title,content, thumb,WeChatCallObjName,"ShareWebPage_Callback"};
javaActive.Call("WXShareWebPage", objs);
#elif UNITY_IPHONE
#endif
}
/// <summary>微信分享图片 场景:0 = 好友列表 1 = 朋友圈 2 = 收藏 图片 大小 </summary>
public void WeChatShare_Image(int scene, byte[] imgData, byte[] thumbData)
{
#if UNITY_EDITOR
#elif UNITY_ANDROID
object[] objs = new object[] { scene, imgData, thumbData, WeChatCallObjName, "ShareImage_Callback" };
javaActive.Call("WXShareImage", objs);
#elif UNITY_IPHONE
#endif
}
//--------------------------回调接口---------------------------------//
/// <summary> 登录回调 </summary>
public void LogonCallback(string str)
{
if (str != "用户拒绝授权" && str != "用户取消授权")
{
Debug.Log("微信登录,用户已授权:" + str);
StartCoroutine(GetWeChatUserData(WXAppID, WXAppSecret, str));
}
if (str == "用户拒绝授权" || str == "用户取消授权")
{
Debug.Log("微信登录," + str);
weChatLogonCallback(null);
}
}
//微信SDK的业务调整 现在的分享是无法获取分享成功还是失败:
//现在的分享业务始终返回success(成功)状态
//公告链接:https://open.weixin.qq.com/cgi-bin/announce?action=getannouncement&key=11534138374cE6li&version=&lang=zh_CN&token=
/// <summary> 分享图片回调 </summary>
public void ShareImage_Callback(string code)
{
//ERR_OK = 0(用户同意) ERR_AUTH_DENIED = -4(用户拒绝授权) ERR_USER_CANCEL = -2(用户取消)
switch (code)
{
case "ERR_OK":
Debug.Log("分享成功");
break;
case "ERR_AUTH_DENIED":
case "ERR_USER_CANCEL":
Debug.Log("用户取消分享");
break;
default:
break;
}
weChatShareImageCallback(code);
}
public void ShareWebPage_Callback(string code)
{
switch (code)
{
case "ERR_OK":
Debug.Log("wx:网页分享成功");
break;
case "ERR_AUTH_DENIED":
case "ERR_USER_CANCEL":
Debug.Log("wx:用户取消分享网页");
break;
default:
break;
}
weChatShareWebPageCallback(code);
}
/// <summary> 微信支付回调 </summary>
public void WechatPayCallback(string retCode)
{
int state = int.Parse(retCode);
switch (state)
{
case -2:
Debug.Log("支付取消");
break;
case -1:
Debug.Log("支付失败");
break;
case 0:
Debug.Log("支付成功");
break;
}
weChatPayCallback(state);
}
/// <summary>
/// 请求微信用户公开的信息
/// </summary>
/// <param name="appid">应用id</param>
/// <param name="secret">应用秘密指令</param>
/// <param name="code">微信客户端返回的code</param>
/// <returns></returns>
IEnumerator GetWeChatUserData(string appid, string secret, string code)
{
//第一步:通过appid、secret、code去获取请求令牌以及openid;
//code是用户在微信登录界面授权后返回的
string url = "https://api.weixin.qq.com/sns/oauth2/access_token?appid="
+ appid + "&secret=" + secret + "&code=" + code + "&grant_type=authorization_code";
WWW www = new WWW(url);
yield return www;
if (www.error != null)
{
Debug.Log("微信登录请求令牌失败:" + www.error);
}
else
{
Debug.Log("微信登录请求令牌成功:" + www.text);
WeChatData weChatData = JsonUtility.FromJson<WeChatData>(www.text);
if (weChatData == null)
{
yield break;
}
else
{
//第二步:请求个人微信公开的信息
string getuserurl = "https://api.weixin.qq.com/sns/userinfo?access_token="
+ weChatData.access_token + "&openid=" + weChatData.openid;
WWW getuser = new WWW(getuserurl);
yield return getuser;
if (getuser.error != null)
{
Debug.Log("向微信请求用户公开的信息,出现异常:" + getuser.error);
}
else
{
//从json格式的数据中反序列化获取
WeChatUserData weChatUserData = JsonUtility.FromJson<WeChatUserData>(getuser.text);
if (weChatUserData == null)
{
Debug.Log("error:" + "用户信息反序列化异常");
yield break;
}
else
{
Debug.Log("用户信息获取成功:" + getuser.text);
Debug.Log("openid:" + weChatUserData.openid + ";nickname:" + weChatUserData.nickname);
//获取到微信的openid与昵称
string wxOpenID = weChatUserData.openid;
string wxNickname = weChatUserData.nickname;
int sex = weChatUserData.sex;//0-女 1-男 //sex 普通用户性别,1为男性,2为女性
//微信登录 外部要处理的事件
weChatLogonCallback(weChatUserData);
}
}
}
}
}
}
/// <summary> 用户授权后 微信返回的数据 </summary>
[System.Serializable]
public class WeChatData
{
//使用token进一步获取个人公开的资料
public string access_token;
public string expires_in;
public string refresh_token;
public string openid;
public string scope;
}
[System.Serializable]
public class WeChatUserData
{
public string openid;//用户唯一ID
public string nickname;//昵称
public int sex;//性别
public string province;//省份
public string city;//城市
public string country;//县级
public string headimgurl;//头像Url
public string[] privilege;//用户特权
public string unionid;//会员
}
015 支付宝中间件的封装
15.1、AliComponent.cs文件的编写
using System.Collections;
using System.Collections.Generic;
using System.Runtime.InteropServices;
using UnityEngine;
public class AliComponent : MonoBehaviour
{
string aliSDKCallObjName = "AliComponent";
//是java里的类,一些静态方法可以直接通过这个调用。
//androidjavaobject 调用的话,会生成一个对象,就和java里new一个对象一样,可以通过对象去调用里面的方法以及属性。
AndroidJavaClass javaClass;
AndroidJavaObject javaActive;
//"com.mafeng.alinewsdk.AliSDKActivity"是2018.11.01日更新的版本 对应安卓工程中的alinewsdk Module
//而"com.mafeng.aliopensdk.AliSDKActivity"是之前的版本 对应安卓工程中的aliopensdk Module
string javaClassStr = "com.mafeng.alinewsdk.AliSDKActivity"; //"com.mafeng.aliopensdk.AliSDKActivity";
string javaActiveStr = "currentActivity";
#if UNITY_EDITOR
#elif UNITY_ANDROID
#elif UNITY_IPHONE
#endif
void Start()
{
#if UNITY_EDITOR
#elif UNITY_ANDROID
javaActive = new AndroidJavaObject(javaClassStr);
//初始化 获得项目对应的MainActivity
//javaClass = new AndroidJavaClass(javaClassStr);
//javaActive = javaClass.GetStatic<AndroidJavaObject>(javaActiveStr);
#elif UNITY_IPHONE
#endif
}
/// <summary>
/// 支付宝支付
/// </summary>
public void AliPay(string OrderInfo)
{
#if UNITY_EDITOR
#elif UNITY_ANDROID
object[] objs = new object[] { OrderInfo,aliSDKCallObjName, "AliPayCallback" };
javaActive.Call("AliPay", objs);
#elif UNITY_IPHONE
#endif
}
public delegate void AliSDKAction(string result);
public AliSDKAction aliPayCallBack;
/// <summary>支付宝支付回调</summary>
public void AliPayCallback(string result)
{
aliPayCallBack(result);
//告诉服务器已经支付 等待返回结果
//再监听结果 进行发放奖励 实际上都是独立的
if (result == "支付成功")
{
Debug.Log("支付宝支付成功");
}
else
{
Debug.Log("支付宝支付失败");
}
}
}
016 完成demo入口场景
16.1、ToDemoScene.cs文件的编写
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
using UnityEngine.UI;
public class ToDemoScene : MonoBehaviour
{
void Start()
{
var btns = transform.GetComponentsInChildren<Button>();
for (int i = 0; i < btns.Length; i++)
{
string btnName = btns[i].name;
btns[i].onClick.AddListener(() =>
{
switch (btnName)
{
case "ToWeChatLogon":
SceneManager.LoadScene("微信登录");
break;
case "ToWeChatShare":
SceneManager.LoadScene("微信分享");
break;
case "ToWeChatPay":
SceneManager.LoadScene("微信支付功能");
break;
case "ToAliPay":
SceneManager.LoadScene("支付宝支付功能");
break;
default:
break;
}
});
}
}
}
017 微信登录
17.1、插入EventSystem响应单击事件
17.2、将WeChatComponent放入场景编写WeChatLoginTest.cs文件
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
using UnityEngine.UI;
public class WeChatLogonTest : MonoBehaviour
{
//微信交互的中间件
WeChatComponent weChatComponent;
// Use this for initialization
void Start()
{
//初始化
weChatComponent = GameObject.Find("WeChatComponent").GetComponent<WeChatComponent>();
//登录按钮 注册点击事件 TestWechatLogon
transform.Find("WechatLogonBtn").GetComponent<Button>().onClick.AddListener(TestWechatLogon);
//返回按钮 匿名委托
transform.Find("ReturnBtn").GetComponent<Button>().onClick.AddListener(() =>
{
SceneManager.LoadScene("这是demo入口");
});
}
private void TestWechatLogon()
{
//绑定登录的回调
weChatComponent.weChatLogonCallback += WeChatLogonCallback;
if (weChatComponent.IsWechatInstalled())
{
//wechat logon
weChatComponent.WeChatLogon("mafeng");
}
else
{
//未安装微信 进行相应的提示即可
}
}
private void WeChatLogonCallback(WeChatUserData userData)
{
if (userData != null)
{
transform.Find("tipsWindow/content").GetComponent<Text>().text = "登录成功,用户的OpenID:" + userData.openid + '\n'
+ "昵称:" + userData.nickname + '\n'
+ "性别:" + userData.sex;
Debug.Log("头像链接:" + userData.headimgurl);
}
else
{
transform.Find("tipsWindow/content").GetComponent<Text>().text = "用户拒绝授权或者取消登录";
}
transform.Find("tipsWindow").gameObject.SetActive(true);
weChatComponent.weChatLogonCallback -= WeChatLogonCallback;
}
}
17.3、将WeChatLoginTest文件挂载到camera
018 微信分享
18.1、编写FileComponent.cs文件
using System.Collections;
using System.Collections.Generic;
using System.IO;
using UnityEngine;
public class FileComponent
{
//获取StreamingAssets文件夹的路径
/// <summary>
///
/// </summary>
/// <param name="path">文件的子路径</param>
/// <param name="ifwwwLoad">是否www加载</param>
/// <returns></returns>
public static string GetStreamingAssets(string path, bool ifwwwLoad)
{
string tempPath = "";
if (ifwwwLoad)
{
//当前运行的平台是否等于Iphone
if (Application.platform == RuntimePlatform.IPhonePlayer)
{
tempPath = "file://" + Application.streamingAssetsPath + "/" + path;
}
else
{
tempPath = Application.streamingAssetsPath + "/" + path;
}
}
else
{
Debug.Log("现在并不支持通过File类去访问 如果发现可以的同学,请告诉我哦");
}
return tempPath;
}
//获取Persistentdatapath的文件夹路径
public static string GetPersistentdatapath(string path, bool ifwwwLoad)
{
string temppath = "";
if (ifwwwLoad)
{
temppath = "file://" + Application.persistentDataPath + "/" + path;
}
else
{
temppath = Application.persistentDataPath + "/" + path;
}
return temppath;
}
}
18.2、编写WeChatShareTest.cs文件
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
using UnityEngine.UI;
public class WeChatShareTest : MonoBehaviour
{
WeChatComponent weChatComponent;
void Start()
{
weChatComponent = GameObject.Find("WeChatComponent").GetComponent<WeChatComponent>();
transform.Find("ShareImageBtn").GetComponent<Button>().onClick.AddListener(ShareImageToWeChat);
transform.Find("ShareWebPageBtn").GetComponent<Button>().onClick.AddListener(ShareWebPageToWeChat);
//注册场景中返回按钮的事件
transform.Find("ReturnBtn").GetComponent<Button>().onClick.AddListener(() => {
SceneManager.LoadScene("这是demo入口");
});
}
//分享网页结果
private void WeChatShareWebPageCallback(string result)
{
transform.Find("contentText").GetComponent<Text>().text = "分享结果网页结果:" + result;
weChatComponent.weChatShareWebPageCallback -= WeChatShareWebPageCallback;
}
//分享图片的
private void WeChatShareImageCallback(string result)
{
transform.Find("contentText").GetComponent<Text>().text = "分享结果图片结果:" + result;
weChatComponent.weChatShareImageCallback -= WeChatShareImageCallback;
}
/// <summary> 分享图片 </summary>
public void ShareImageToWeChat()
{
//分享图片的回调
weChatComponent.weChatShareImageCallback += WeChatShareImageCallback;
//需要缩略图 以及 分享的目标:场景:0 = 好友列表 1 = 朋友圈 2 = 收藏
StartCoroutine(ShareImageToWeChat(0));
}
/// <summary> 分享网页 </summary>
public void ShareWebPageToWeChat()
{
Debug.Log("分享网页");
//分享网页的回调
weChatComponent.weChatShareWebPageCallback += WeChatShareWebPageCallback;
//0 = 好友列表 1 = 朋友圈 2 = 收藏
StartCoroutine(ShareWebPageToWeChat(0, @"https://www.baidu.com/", "百度一下,你就知道了",
"全球最大的中文搜索引擎、致力于让网民更便捷地获取信息."));
}
Texture2D miniTexture, mainTexture;
byte[] thumbData, mainImageData;
private IEnumerator ShareImageToWeChat(int scene)
{
if (miniTexture == null)
{
Debug.Log("分享图片");
string miniImagePath = FileComponent.GetStreamingAssets("shareIcon.png",true);
WWW loadMini = new WWW(miniImagePath);
yield return loadMini;
if (loadMini != null && string.IsNullOrEmpty(loadMini.error))
{
//获取缩略图
miniTexture = loadMini.texture;
thumbData = miniTexture.EncodeToPNG();
}
else
{
Debug.Log("error 获取不到分享的缩略图:" + loadMini.error);
}
}
if (mainTexture == null)
{
string imagePath = FileComponent.GetStreamingAssets("shareImage.jpg",true);
WWW loadMainImage = new WWW(imagePath);
yield return loadMainImage;
if (loadMainImage != null && string.IsNullOrEmpty(loadMainImage.error))
{
//获取缩略图
mainTexture = loadMainImage.texture;
mainImageData = mainTexture.EncodeToJPG();
}
else
{
Debug.Log("error 获取不到分享的图片:" + loadMainImage.error);
}
}
weChatComponent.WeChatShare_Image(scene, mainImageData, thumbData);
}
private IEnumerator ShareWebPageToWeChat(int scene, string url, string title, string content)
{
if (miniTexture == null)
{
string miniImagePath = FileComponent.GetStreamingAssets("shareIcon.png",true);
WWW loadMini = new WWW(miniImagePath);
yield return loadMini;
if (loadMini != null && string.IsNullOrEmpty(loadMini.error))
{
//获取缩略图
miniTexture = loadMini.texture;
thumbData = miniTexture.EncodeToPNG();
}
else
{
Debug.Log("error 获取不到分享的缩略图:" + loadMini.error);
}
}
weChatComponent.WeChatShare_WebPage(scene, url, title, content, thumbData);
}
}
019 实现微信支付
19.1、camera挂载WeChatPayTest.cs文件
19.2、编写WeChatPayTest.cs文件
using System;
using System.Collections;
using System.Collections.Generic;
using System.Net;
using System.Net.Sockets;
using System.Text;
using System.Threading;
using UnityEngine;
using UnityEngine.SceneManagement;
using UnityEngine.UI;
public class WeChatPayTest : MonoBehaviour
{
//服务器IP与端口
private EndPoint remoteEndPoint;
//Socket实例
Socket tcpSocket;
public string serverIP = "193.112.30.89";//要连接的服务器IP 公网IP
public int serverPort = 7577;//游戏服务器程序的端口
//接收消息 发送消息的线程
Thread receiveThread, sendThread;
//获取参数
public string appid;
public string mch_id;
public string prepayid;
public string noncestr;
public string timestamp;
public string packageValue;
public string sign;
//调起支付的参数
public string aliPreOrder;
//网络协议的类型 WeChatPay 表示微信支付
public string sendMsgType = "WeChatPay";
WeChatComponent weChatComponent;
//缓存网络消息的队列
Queue<string> netMsg = new Queue<string>();
void Start()
{
//注册微信组件的事件:同步回调通知
weChatComponent = GameObject.Find("WeChatComponent").GetComponent<WeChatComponent>();
weChatComponent.weChatPayCallback += WeChatPayCallback;
//注册场景中返回按钮的事件
transform.Find("ReturnBtn").GetComponent<Button>().onClick.AddListener(() =>
{
SceneManager.LoadScene("这是demo入口");
});
//注册所有购买按钮的事件
transform.Find("PayBtn").GetComponent<Button>().onClick.AddListener(()=> {
RequestPayment(sendMsgType, "1001", 1);
}
);
//初始化连接服务器
tcpSocket = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
remoteEndPoint = new IPEndPoint(IPAddress.Parse(serverIP), serverPort);
tcpSocket.Connect(remoteEndPoint);
//接收服务器消息
receiveThread = new Thread(Receivemsg);
receiveThread.Start();
receiveThread.IsBackground = true;
}
/// <summary> 微信支付回调 0成功 -1失败 -2取消 </summary>
private void WeChatPayCallback(int state)
{
string result = "";
switch (state)
{
case 0:
result = "成功";
break;
case 1:
result = "失败";
break;
case 2:
result = "取消";
break;
default:
break;
}
transform.Find("PayResult/clientText").GetComponent<Text>().text += "...收到微信客户端的支付结果:" + result;
transform.Find("PayResult").gameObject.SetActive(true);
}
/// <summary> 第一步:向服务器请求调起支付SDK所需要的参数 </summary>
public void RequestPayment(string msgType, string goodsID, int buyCount)
{
//与服务器请求的时候,至少包含3个参数,甚至更多
//支付的类型、购买的物品ID、购买的物品数量
SendToServer(msgType + "," + goodsID + "," + buyCount);
}
/// <summary> 第二步:调用安卓层的支付方法 从而发起支付 </summary>
public void Update()
{
//处理网络消息 根据不同的协议 调用不同的消息
if (netMsg.Count > 0)
{
string msg = netMsg.Dequeue();
string[] msgList = msg.Split(',');
switch (msgList[0])
{
case "WeChatPay":
Debug.Log("WeChatPay");
WechatPay(msgList);
break;
//case "AliPay":
// break;
case "sendProps":
transform.Find("PayResult/serverText").GetComponent<Text>().text += "...现在收到服务器发放的道具";
transform.Find("PayResult").gameObject.SetActive(true);
//消息协议 SendProps,会话ID/订单号,道具ID,数量...(道具实体)
string sendMsg = "getProps" + msgList[1] + "," + msgList[2] + "," + msgList[3];
SendToServer(sendMsg);//告诉服务器 我已经收到道具
break;
default:
break;
}
}
}
/// <summary> 将参数传递给安卓层 调起微信支付 </summary>
private void WechatPay(string[] parameter)
{
Debug.Log("获取到微信支付参数:");
appid = parameter[1];
mch_id = parameter[2];
prepayid = parameter[3];
noncestr = parameter[4];
timestamp = parameter[5];
packageValue = parameter[6];
sign = parameter[7];
Debug.Log("执行微信支付");
weChatComponent.WeChatPay(appid, mch_id, prepayid, noncestr, timestamp, sign);
}
/// <summary>接收消息到服务器</summary>
private void Receivemsg()
{
//存储网络消息的容器
byte[] data = new byte[1024];
while (true)
{
//将接收到的消息进行解码 byte[]->string
int length = tcpSocket.ReceiveFrom(data, ref remoteEndPoint);
if (length > 0)
{
//字节数组
string message = Encoding.UTF8.GetString(data, 0, length);
netMsg.Enqueue(message);
Debug.Log("收到的服务器消息是:" + message);
}
}
}
/// <summary>发送消息到服务器</summary>
public void SendToServer(string str)
{
string sendMsg = str;
sendThread = new Thread(() => {
byte[] data = Encoding.UTF8.GetBytes(sendMsg);//封装发送数据 转化为byte[]字节数组
tcpSocket.SendTo(data, remoteEndPoint);//发送到服务器
sendThread.Abort();//中止线程
});
sendThread.Start();
}
void Close()
{
//释放连接
if (tcpSocket != null)
{
tcpSocket.Close();
}
sendThread.Abort();
receiveThread.Abort();
}
/// <summary> 当应用程序正常退出的时候 释放网络连接和中止发送线程 </summary>
private void OnApplicationQuit()
{
Close();
}
private void OnDestroy()
{
Close();
}
}
020 实现支付宝支付
20.1、camera挂载AliPayTest.cs文件
扫描二维码关注公众号,回复:
11534864 查看本文章
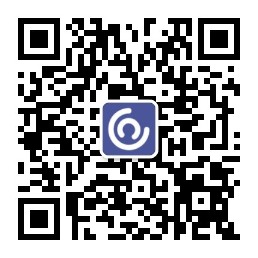
20.2、工程内挂载AliComponent预置体
20.3、编写AliPayTest.cs文件
using System;
using System.Collections;
using System.Collections.Generic;
using System.Net;
using System.Net.Sockets;
using System.Text;
using System.Threading;
using UnityEngine;
using UnityEngine.SceneManagement;
using UnityEngine.UI;
public class AliPayTest : MonoBehaviour
{
//服务器IP与端口
private EndPoint remoteEndPoint;
//Socket实例
Socket tcpSocket;
//远程主机 端口->游戏服务器程序
public string serverIP = "193.112.30.89";
public int serverPort = 7577;
//接收消息 发送消息的线程
Thread receiveThread, sendThread;
//AliPay 协议的头部 :请求调起阿里支付的参数:
public string sendMsgType = "AliPay";
AliComponent aliComponent;
//缓存网络消息的队列
Queue<string> netMsg = new Queue<string>();
void Start()
{
//注册微信组件的事件:同步回调通知
aliComponent = GameObject.Find("AliComponent").GetComponent<AliComponent>();
aliComponent.aliPayCallBack += AliPayCallback;
//场景中返回按钮的事件
transform.Find("ReturnBtn").GetComponent<Button>().onClick.AddListener(() =>
{
SceneManager.LoadScene("这是demo入口");
});
//注册所有购买按钮的事件
//注册所有购买按钮的事件
transform.Find("PayBtn").GetComponent<Button>().onClick.AddListener(() => {
RequestPayment(sendMsgType, "1001", 1);
}
);
//初始化连接服务器
tcpSocket = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
remoteEndPoint = new IPEndPoint(IPAddress.Parse(serverIP), serverPort);
tcpSocket.Connect(remoteEndPoint);
//接收服务器消息
receiveThread = new Thread(Receivemsg);
receiveThread.Start();
receiveThread.IsBackground = true;
}
/// <summary> 支付宝回调处理事件 </summary>
private void AliPayCallback(string result)
{
if (result == "支付成功")
{
Debug.Log("支付宝支付成功");
}
else
{
Debug.Log("支付宝支付失败");
}
transform.Find("PayResult/clientText").GetComponent<Text>().text += "...收到支付宝支付结果:" + result;
transform.Find("PayResult").gameObject.SetActive(true);
}
/// <summary> 第一步:向服务器请求调起支付SDK所需要的参数 </summary>
public void RequestPayment(string msgType, string goodsID, int buyCount)
{
//与服务器请求的时候,至少包含3个参数,甚至更多
//支付的类型、购买的物品ID、购买的物品数量
SendToServer(msgType + "," + goodsID + "," + buyCount);
}
/// <summary> 第二步:调用安卓层的支付方法 从而发起支付 </summary>
public void Update()
{
//处理网络消息 根据不同的协议 调用不同的消息
if (netMsg.Count > 0)
{
string msg = netMsg.Dequeue();
string[] msgList = msg.Split(',');
switch (msgList[0])
{
case "AliPay":
Debug.Log("AliPay");
AliPay(msgList);
break;
case "sendProps":
transform.Find("PayResult/serverText").GetComponent<Text>().text += "...现在收到服务器发放的道具";
transform.Find("PayResult").gameObject.SetActive(true);
//消息协议 SendProps,会话ID/订单号,道具ID,数量...(道具实体)
string sendMsg = "getProps" + msgList[1] + "," + msgList[2] + "," + msgList[3];
SendToServer(sendMsg);
break;
default:
break;
}
}
}
/// <summary> 将参数传递给安卓层 调起微信支付 </summary>
private void AliPay(string[] parameter)
{
Debug.Log("执行支付宝支付");
aliComponent.AliPay(parameter[1]);//索引从0开始 表示第一个位置 如果是1就是第二个位置
}
/// <summary>接收到服务器的消息</summary>
private void Receivemsg()
{
//存储网络消息的容器
byte[] data = new byte[1024];
while (true)
{
//将接收到的消息进行解码 byte[]->string
int length = tcpSocket.ReceiveFrom(data, ref remoteEndPoint);
if (length > 0)
{
string message = Encoding.UTF8.GetString(data, 0, length);
netMsg.Enqueue(message);
Debug.Log("收到的服务器消息是:" + message);
}
}
}
/// <summary>发送消息到服务器</summary>
public void SendToServer(string str)
{
string sendMsg = str;
sendThread = new Thread(() => {
byte[] data = Encoding.UTF8.GetBytes(sendMsg);//封装发送数据 转化为byte[]字节数组
tcpSocket.SendTo(data, remoteEndPoint);//发送到服务器
sendThread.Abort();//中止线程
});
sendThread.Start();
}
void Close()
{
//释放连接
if (tcpSocket != null)
{
tcpSocket.Close();
}
sendThread.Abort();
receiveThread.Abort();
}
/// <summary> 当应用程序正常退出的时候 释放网络连接和中止发送线程 </summary>
private void OnApplicationQuit()
{
Close();
}
private void OnDestroy()
{
Close();
}
}