本文答案,部分参考于C++ Primer 习题集
前面章节的习题答案
8.1
#include<iostream>
#include<stdexcept>
using namespace std;
istream &f(istream &in){
int v;
while(in>>v,!in.eof()){
if(in.bad())
throw runtime_error("IO流错误");
if(in.fail()){
cerr<<"数据错误,请重试"<<endl;
in.clear();
in.ignore(100,'\n');
continue;
}
cout<<v<<endl;
}
in.clear();
return in;
}
int main(){
cout<<"请输入一些参数,按Ctrl+Z结束"<<endl;
f(cin);
return 0;
}
8.2
请输入一些参数,按Ctrl+Z结束
1 2 3
1
2
3
7.6
7
数据错误,请重试
a
数据错误,请重试
4
4
^Z
请按任意键继续. . .
8.3
① 遇到了文件结束符
② 遇到了IO流错误
③ 读入了无效数据
扫描二维码关注公众号,回复:
11525966 查看本文章
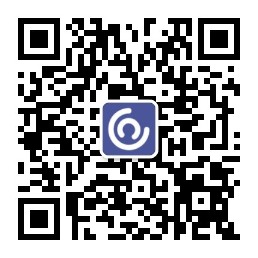
8.4
#include<iostream>
#include<fstream>
#include<string>
#include<vector>
using namespace std;
int main(void){
ifstream in("F:\\Data.txt"); //打开文件
//这里要注意的是,C++里面的文件路径是要用
// \\来写的
if(!in){
cerr<<"无法打开输入文件"<<endl;
return -1;
}
string line;
vector<string> words;
while(getline(in,line)){ //从文件中读取一行
words.push_back(line); //添加到vector中
}
in.close(); //输入完毕,关闭文件
vector<string>::const_iterator it = words.begin(); //迭代器
while(it!=words.end()){
cout<<*it<<endl;
++it;
}
return 0;
}
8.5
#include<iostream>
#include<fstream>
#include<string>
#include<vector>
using namespace std;
int main(void){
ifstream in("F:\\Data.txt"); //打开文件
//这里要注意的是,C++里面的文件路径是要用
// \\来写的
if(!in){
cerr<<"无法打开输入文件"<<endl;
return -1;
}
string line;
vector<string> words;
while(in>>line){ //从文件中读取一行
words.push_back(line); //添加到vector中
}
in.close(); //输入完毕,关闭文件
vector<string>::const_iterator it = words.begin(); //迭代器
while(it!=words.end()){
cout<<*it<<endl;
++it;
}
return 0;
}
8.6
#include<iostream>
#include<fstream>
#include"Sales_data.h"
using namespace std;
int main(int argc,char *argc[]){
if(argc!=2){
cerr<"请给出文件名"<<endl;
return -1;
}
Sales_data total; //保存当前求和结果的变量
if(read(in,total)){ //读入第一笔交易
Sales_data trans; //保存下一条交易数据的变量
while(read(in,trans)){ //读入剩余的交易
if(total.isbn()==trans.isbn()) //检查isbn
total.combine(trans); //更新变量total当前的值
else{
print(cout,total)<<endl; //输出结果
total=trans; //处理下一本书
}
}
print(cout,total)<<endl; //输出最后一条交易
}else{
cerr<<"没有数据"<<endl; //通知用户
}
return 0;
}
8.7
#include<iostream>
#include<fstream>
#include "Sales_data.h"
using namespace std;
int main(int argc,char *argv[]){
if(argc!=3){
cerr<<"请给出输入,输出文件名"<<endl;
return -1;
}
ifstream in(argv[1]);
if(!in){
cerr<<"无法打开输入文件"<<endl;
return -1;
}
ofstream out(argv[2]);
if(!out){
cerr<<"无法打开输入文件"<<endl;
return -1;
}
Sales_data total;
if(read(in,total)){
Sales_data trans;
while(read(in,trans)){
if(total.isbn()==trans.isbn())
total.combine(trans);
else{
print(out,total)<<endl;
total=trans;
}
}
print(out,total)<<endl;
}else{
cerr<<"没有数据"<<endl;
}
return 0;
}
8.8
#include<iostream>
#include<fstream>
#include "Sales_data.h"
using namespace std;
int main(int argc,char *argv[]){
if(argc!=3){
cerr<<"请给出输入,输出文件名"<<endl;
return -1;
}
ifstream in(argv[1]);
if(!in){
cerr<<"无法打开输入文件"<<endl;
return -1;
}
ofstream out(argv[2],ofstream::app);
if(!out){
cerr<<"无法打开输入文件"<<endl;
return -1;
}
Sales_data total;
if(read(in,total)){
Sales_data trans;
while(read(in,trans)){
if(total.isbn()==trans.isbn())
total.combine(trans);
else{
print(out,total)<<endl;
total=trans;
}
}
print(out,total)<<endl;
}else{
cerr<<"没有数据"<<endl;
}
return 0;
}
8.9
#include<iostream>
#include<sstream>
#include<string>
#include<stdexcept>
using namespace std;
istream &f(istream &in){
string v;
while(in>>v,!in.eof()){ //直到遇到文件结束符才停止读取
if(in.bad())
throw runtime_error("IO流错误");
if(in.fail()){
cerr<<"数据错误,请重试:"<<endl;
in.clear();
in.ignore(100,'\n');
continue;
}
cout<<v<<endl;
}
in.clear();
return in;
}
int main(){
ostringstream msg;
msg<<"C++ Primer第五版"<<endl;
istringstream in(msg.str());
f(in);
return 0;
}
8.10
#include<iostream>
#include<fstream>
#include<sstream>
#include<string>
#include<vector>
using namespace std;
int main(){
ifstream in("F:\\Data.txt");
if(!in){
cerr<<"无法打开输入文件"<<endl;
return -1;
}
string line;
vector<string> words;
while(getline(in,line)){
words.push_back(line);
}
in.close();
vector<string>::const_iterator it=words.begin();
while(it!=words.end()){
istringstream line_str(*it);
string word;
while(line_str>>word)
cout<<word<<" ";
cout<<endl;
++it;
}
return 0;
}
8.11
#include<iostream>
#include<sstream>
#include<string>
#include<vector>
using namespace std;
struct PersonInfo{
string name;
vector<string> phones;
};
int main(){
string line,word;
vector<PersonInfo> people;
istringstream record;
while(getline(cin,line)){
PersonInfo info;
record.clear();
record.str(line);
record>>info.name;
while(record>>word)
info.phones.push_back(word);
people.push_back(info);
}
return 0;
}
8.12
由于每个人的电话号码数量不固定,因此更好方式不是通过类内初始化定制人名和所有的电话号码,而是在缺省初始化之后,在程序中设置人名并逐个添加电话号码.
8.13
#include<iostream>
#include<fstream>
#include<sstream>
#include<string>
#include<vector>
using namespace std;
struct PersonInfo{
string name;
vector<string> phones;
};
string format(const string &s){
return s;
}
bool valid(const string &s){
//如何验证电话号码将在第17章介绍
//现在简单返回true
return true;
}
int main(int argc,char *argv[]){
string line,word;
vector<PersonInfo> people;
istringstream record;
if(argc!=2){
cerr<<"请给出文件名"<<endl;
return -1;
}
ifstream in (argv[1]);
if(!in){
cerr<<"无法打开输入文件"<<endl;
return -1;
}
while(getline(in,line)){
PersonInfo info;
record.clear();
record.str(line);
record>>info.name;
while(record>>word)
info.phones.push_back(word);
people.push_back(info);
ostringstream os ;
for(const auto &entry: people){
ostringstream formatted,badNums;
for(const auto &nums:entry.phones){
if(!valid(nums)){
badNums<<" "<<nums;
}
else
formatted<<" "<<format(nums);
}
}
if(badNums.str().empty())
os<<entry.name<<" "<<formatted.str()<<endl;
else
cerr<<"input error: "<<entry.name<<" invalid number(s) "<<badNums.str()<<endl;
}
cout<<os.str()<,endl;
return 0;
}
8.14
这两条语句分别使用范围for语句枚举people中所有项(人)和每项的phones中的所有项(电话号码) 使用const表明在循环中不会改变这些项的值,auto是请求编译器依据vecto元素类型来推断出entry和nums的类型,即简化代码又避免出错.people和phones的元素分别是结构对象和字符串对象,使用引用既可避免对象拷贝.