简介
Redis是当前比较热门的NOSQL系统之一,它是一个开源的使用ANSI c语言编写的key-value存储系统(区别于MySQL的二维表格的形式存储。)。
Redis它会周期性的把更新的数据写入磁盘或者把修改操作写入追加的记录文件,实现数据的持久化。
主要特点
优点:
-
执行效率高,Redis读取的速度是110000次/s,写的速度是81000次/s;
-
支持多种数据结构:string(字符串);list(列表);hash(哈希),set(集合);zset(有序集合)
-
持久化,集群部署
-
支持过期时间,支持事务,消息订阅
-
所有Redis操作是原子的,这保证了如果两个客户端同时访问的Redis服务器将获得更新后的值。
缺点:
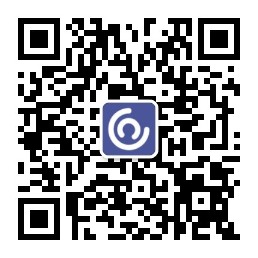
- 数据无结构化,通常只被当作字符串或者二进制数据
Redis的安装
Redis的安装常用有两种,一种是通过虚拟机在Linux系统安装,一种是在Windows直接安装,第一种公司开发比较常用,为了操作方便,我这里演示第二种,有需要第一种的私信。
-
下载地址:
https://github.com/ServiceStack/redis-windows
找到自己想要的版本下载。 -
解压下载后的压缩包,解压后页面如下
Redis的使用
引入相关依赖
<!-- redis -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<!--spring2.X集成redis所需common-pool2-->
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-pool2</artifactId>
<version>2.6.0</version>
</dependency>
创建配置类
配置类基本都是固定的,不需要我们敲,大家可以直接复制
package com.athk.servicebase;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.PropertyAccessor;
import com.fasterxml.jackson.databind.ObjectMapper;
import org.springframework.cache.CacheManager;
import org.springframework.cache.annotation.CachingConfigurerSupport;
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.cache.RedisCacheConfiguration;
import org.springframework.data.redis.cache.RedisCacheManager;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.serializer.Jackson2JsonRedisSerializer;
import org.springframework.data.redis.serializer.RedisSerializationContext;
import org.springframework.data.redis.serializer.RedisSerializer;
import org.springframework.data.redis.serializer.StringRedisSerializer;
import java.time.Duration;
@EnableCaching
@Configuration
public class RedisConfig extends CachingConfigurerSupport {
@Bean
public RedisTemplate<String, Object> redisTemplate(RedisConnectionFactory factory) {
RedisTemplate<String, Object> template = new RedisTemplate<>();
RedisSerializer<String> redisSerializer = new StringRedisSerializer();
Jackson2JsonRedisSerializer jackson2JsonRedisSerializer = new Jackson2JsonRedisSerializer(Object.class);
ObjectMapper om = new ObjectMapper();
om.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY);
om.enableDefaultTyping(ObjectMapper.DefaultTyping.NON_FINAL);
jackson2JsonRedisSerializer.setObjectMapper(om);
template.setConnectionFactory(factory);
//key序列化方式
template.setKeySerializer(redisSerializer);
//value序列化
template.setValueSerializer(jackson2JsonRedisSerializer);
//value hashmap序列化
template.setHashValueSerializer(jackson2JsonRedisSerializer);
return template;
}
@Bean
public CacheManager cacheManager(RedisConnectionFactory factory) {
RedisSerializer<String> redisSerializer = new StringRedisSerializer();
Jackson2JsonRedisSerializer jackson2JsonRedisSerializer = new Jackson2JsonRedisSerializer(Object.class);
//解决查询缓存转换异常的问题
ObjectMapper om = new ObjectMapper();
om.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY);
om.enableDefaultTyping(ObjectMapper.DefaultTyping.NON_FINAL);
jackson2JsonRedisSerializer.setObjectMapper(om);
// 配置序列化(解决乱码的问题),过期时间600秒
RedisCacheConfiguration config = RedisCacheConfiguration.defaultCacheConfig()
.entryTtl(Duration.ofSeconds(600))
.serializeKeysWith(RedisSerializationContext.SerializationPair.fromSerializer(redisSerializer))
.serializeValuesWith(RedisSerializationContext.SerializationPair.fromSerializer(jackson2JsonRedisSerializer))
.disableCachingNullValues();
RedisCacheManager cacheManager = RedisCacheManager.builder(factory)
.cacheDefaults(config)
.build();
return cacheManager;
}
}
在接口中添加Redis缓存
-
@Cacheable
一般用在查询方法上。
第一次请求时会请求数据库,后面再次请求,则会在缓存中查找,若缓存有数据,直接获取数据;若缓存没数据,则到数据查询数据,并添加到缓存中。 -
@CachePut
一般用在新增方法上。
每次都会执行,并将结果存入指定的缓存中。其他方法可以直接从响应的缓存中读取缓存数据,而不需要再去查询数据库。
对于上述两个注解的属性有三个
1、value:缓存名,必填,它指定了你的缓存存放在哪块命名空间
2、cacheNames:与 value 差不多,二选一即可
3、key:可选属性,可以使用 SpEL 标签自定义缓存的key(**注:**若注解添加该属性,需要在双引号中再加个单引号才能正常显示,如"'selectIndexList'"
)
@CacheEvict
一般用在更新或者删除方法上。
会清空指定的缓存。
上面注解有多个属性,除了上面的三个属性还额外加了两个
4、allEntries:是否清空所有缓存,默认为 false。如果指定为 true,则方法调用后将立即清空所有的缓存
5、beforeInvocation:是否在方法执行前就清空,默认为 false。如果指定为 true,则在方法执行前就会清空缓存
总的来说,当我们添加缓存后,需要获取数据,或者填充数据的时候,先会通过缓存,若缓存中没有,则在数据库进行操作,然后把结果返回给缓存,减少数据库压力和提高访问速度。
配置Redis
若不配置,则使用默认配置
#在application.properties文件中进行配置
spring.redis.host=127.0.0.1
spring.redis.port=6379
spring.redis.database= 0
spring.redis.timeout=180000
spring.redis.lettuce.pool.max-active=20
spring.redis.lettuce.pool.max-wait=-1
#最大阻塞等待时间(负数表示没限制)
spring.redis.lettuce.pool.max-idle=5
spring.redis.lettuce.pool.min-idle=0
启动Redis
在上述解压完成的页面进入cmd窗口,输入redis-server.exe
命令,既可以成功启动
验证缓存
当我们第一次点击获取数据时,控制台会打印出sql查询语句
我们也可以通过Redis Desktop Manager工具查看我们缓存的数据
我们把控制台清空后,再次获取数据看看效果
此时控制台不再打印sql语句,说明我们的数据是从缓存中获取的,没有操作数据库查找,而且可以发现获取数据的速度比之前快,使用Redis可以减少数据库压力和提高访问速度。
使用场景
并非所有的场景我们都可以使用Redis,当我们遇到被高频访问,且数据不经常被修改,数据重要程度一般的时候我们可以使用Redis帮我们存储数据,达到高效的开发;
但是如果数据经常被修改,或者很重要,则不用Redis,如财务系统;
希望该文章对你们有帮助哈,有帮到你们的麻烦点个赞哈,有兴趣的朋友可以关注一下公众号,公众号上会发布一些最近行业常用的技术,还有一些常见的开发错误,想要一些Java资源的话也可以公众号留言,小编会尽力为你搜索,同时也会发表一些自己见解的文章。