一.Spring IOC容器
IOC是控制反转的意思,就是和传统的对象调用另一个对象不同,IOC容器会根据你的需求分配你需要的对象。例如,一个订单管理组件需要信用卡的认证组件,但他不需要自己创建信用卡认证组件的实例,它只需表明自己没有它,容器就会主动赋予它一个信用卡认证组件。
Spring Application Framework的核心就是其IOC容器,该容器的工作包括对应用程序的对象(Bean)的实例化,初始化,装配以及在对象的整个生命周期中提供其他的Spring功能。
过程图如下:
二.使用基于XML的配置创建和使用Spring容器
1.编写Bean对象文件:
package com.yanjiadou.spring.study;
public class HelloWorld {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public void hello() {
System.out.println("hello:"+name);
}
}
2.在xml中配置它
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<!-- 配置bean -->
<bean id="helloworld" class="com.yanjiadou.spring.study.HelloWorld" >
<property name="name" value="Spring"></property>
</bean>
</beans>
3.在Main.java中使用它:
package com.yanjiadou.spring.study;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Main {
public static void main(String[] args) {
//加载应用上下文
ApplicationContext ct = new ClassPathXmlApplicationContext("applicatioinContext.xml");
//获取bean对象
HelloWorld helloworld=(HelloWorld)ct.getBean("helloworld");
//调用方法
helloworld.hello();
}
}
结果:
我们可以看出关键在于xml文件的配置,下面我们解析xml文件中的bean配置:
<!-- 配置bean -->
<bean id="helloworld" class="com.yanjiadou.spring.study.HelloWorld" >
<property name="name" value="Spring"></property>
</bean>
id表示我们为要装配入的bean命名。
class的值就是我们要装配的Bean。
< property >中的name的值就是我们装配的Bean中的一个属性,后面的value的值就是我们要赋给它的值(调用Setter()方法注入)。
从上面我们可以看出元素的注入交给Spring容器实现了,我们其他类中只需向容器申请调用就可以了。
三.Spring注入方式
1.Setter注入
Setter注入是在Bean实例创建完毕后执行,通过调用与Bean的配置元数据定义的属性相对应的Setter方法注入这些属性。
< property name=“属性名” value=“属性值”>< /property >
还可以通过下面的方式指定对其他Bean的引用:
扫描二维码关注公众号,回复:
11501384 查看本文章
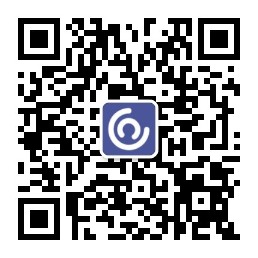
< property name=“定义的Bean类型的名” ref=“要注入的Bean”>< /property >
2.构造函数注入
比如:
package com.yanjiadou.spring.study;
public class HelloWorld {
private World world;
private String name;
public HelloWorld(World world)
{
this.world=world;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public void hello() {
System.out.println("hello:"+name);
}
}
那么我们可以这么配置:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<!-- 配置bean -->
<bean id="helloworld" class="com.yanjiadou.spring.study.HelloWorld" >
<property name="name" value="Spring"></property>
<constructor-arg ref="World"/>
</bean>
</beans>
构造函数的其中一个缺点是无法处理循环依赖。