题目一:回文判断
[问题描述]
对于一个从键盘输入的字符串,判断其是否为回文。回文即正反序相同。如“abba”是回文,而“abab”不是回文。
[基本要求]
(1)数据从键盘读入;
(2)输出要判断的字符串;
(3)利用栈和队列对给定的字符串判断其是否是回文,若是则输出“Yes”,否则输出“No”。
输入:
123321
123456
zxczxc
zxcxz
输出:
Yes
No
No
Yes
CODE:
#include <iostream>
#include <cstring>
#include <cstdio>
#include <cstdlib>
using namespace std;
const int MAXSIZE=1100;
typedef struct
{
char *base;
char *top;
int l;
} SqStack;
void InitStack(SqStack &s)
{
s.base=new char[MAXSIZE];
if(!s.base)
exit(1);
s.top=s.base;
s.l=MAXSIZE;
return;
}
void Push(SqStack &s,char ch)
{
if(s.top-s.base==s.l)
return;
*s.top++=ch;
return;
}
typedef struct
{
char *base;
int front;
int rear;
} SqQueue;
void InitQueue(SqQueue &q)
{
q.base=new char[MAXSIZE];
if(!q.base)
exit(1);
q.front=q.rear=0;
return;
}
void EnQueue(SqQueue &q,char ch)
{
if(q.rear+1==MAXSIZE)
exit(1);
q.base[q.rear++]=ch;
return;
}
bool Palindrome(SqStack s,SqQueue q)
{
while(s.top!=s.base||q.rear!=q.front)
{
s.top--;
//cout<<*s.top<<" "<<q.base[q.front]<<endl;
if(*s.top!=q.base[q.front])
break;
q.front++;
}
if(s.top!=s.base||q.rear!=q.front)
return false;
else return true;
}
int main()
{
SqStack s;
InitStack(s);
SqQueue q;
InitQueue(q);
char ch;
ch=getchar();
while(ch!='\n')
{
EnQueue(q,ch);
Push(s,ch);
ch=getchar();
}
if(Palindrome(s,q))
cout<<"Yes"<<endl;
else
cout<<"No"<<endl;
return 0;
}
题目二:商品货架管理
[问题描述]
商店货架以栈的方式摆放商品。生产日期越近的越靠近栈底,出货时从栈顶取货。一天营业结束,如果货架不满,则需上货。入货直接将商品摆放到货架上,则会使生产日期越近的商品越靠近栈顶。这样就需要倒货架,使生产日期越近的越靠近栈底。
[基本要求]
设计一个算法,保证每一次上货后始终保持生产日期越近的商品越靠近栈底。
[实现提示]
可以用一个队列和一个临时栈作为周转。
CODE:
#include <iostream>
#include <cstdlib>
#include <cstdio>
#include <cstring>
using namespace std;
const int MAXSIZE=20;
typedef struct
{
char *base;
char *top;
int l;
} SqStack;
void InitStack(SqStack &s)
{
s.base=new char[MAXSIZE];
if(!s.base)
exit(1);
s.top=s.base;
s.l=MAXSIZE;
return;
}
void Push(SqStack &s,char ch)
{
if(s.top-s.base==s.l)
return;
*s.top++=ch;
return;
}
typedef struct
{
char *base;
int front;
int rear;
} SqQueue;
void InitQueue(SqQueue &q)
{
q.base=new char[MAXSIZE];
if(!q.base)
return;
q.front=q.rear=0;
return;
}
int EnQueue(SqQueue &q,char ch)
{
if((q.rear+1)%MAXSIZE==q.front)
{
cout<<"货满,请先上货"<<endl;
return 0;
}
q.base[q.rear]=ch;
q.rear=(q.rear+1)%MAXSIZE;
return 1;
}
void Purchase(SqQueue &q)
{
cout<<"进货:"<<endl;
cout<<"一个字符代表一个商品,以换行结束"<<endl;
char ch[MAXSIZE];
scanf("%s",ch);
int len=strlen(ch),i;
cout<<"进货为:";
for(i=0; i<len; i++)
{
if(EnQueue(q,ch[i])==0)
break;
cout<<ch[i]<<" ";
}
cout<<endl;
return;
}
void Loading(SqStack &s,SqQueue &q)
{
cout<<"上货:"<<endl;
if(q.front==q.rear)
{
cout<<"没货,请先进货"<<endl;
return;
}
else
{
if(s.top-s.base==s.l)
{
cout<<"货架满,请先出货再上货"<<endl;
return;
}
else
{
SqStack s1;
InitStack(s1);
while(s.top!=s.base)
{
Push(s1,*(--s.top));
}
cout<<"上货物品为:";
while(q.rear!=q.front)
{
if(s1.top-s1.base==s1.l)
{
cout<<endl<<"货架已满"<<endl;
break;
}
cout<<q.base[q.front];
Push(s1,q.base[q.front]);
q.front=(q.front+1)%MAXSIZE;
}
if(q.rear==q.front)
{
cout<<endl<<"无货,货架未满"<<endl;
}
while(s1.top!=s1.base)
{
Push(s,*--s1.top);
}
}
}
}
void Shipment(SqStack &s)
{
cout<<"出货:"<<endl;
cout<<"输入出货数目:"<<endl;
int n,sum=0;
cin>>n;
cout<<"出货物品为:";
while(sum!=n)
{
sum++;
if(s.top==s.base)
{
cout<<"货架已空!!"<<endl;
return;
}
else
{
cout<<*--s.top<<" ";
}
}
if(sum==n)
cout<<endl<<"出货完成!!"<<endl;
}
int main()
{
int b;
SqQueue q;
SqStack s;
InitQueue(q);
InitStack(s);
cout<<"1进货"<<endl;
cout<<"2上货"<<endl;
cout<<"3出货"<<endl;
cout<<"其他结束"<<endl;
cin>>b;
while(b==1||b==2||b==3)
{
switch(b)
{
case 1:
Purchase(q);
break;
case 2:
Loading(s,q);
break;
case 3:
Shipment(s);
break;
}
cout<<"1进货"<<endl;
cout<<"2上货"<<endl;
cout<<"3出货"<<endl;
cout<<"其他结束"<<endl;
cin>>b;
}
return 0;
}
题目三:舞伴问题
假设在周末舞会上,男士们和女士们进入舞厅时,各自排成一队。跳舞开始时,依次从男队和女队的队头上各出一人配成舞伴。若两队初始人数不相同,则较长的那一队中未配对者等待下一轮舞曲。现要求写一算法模拟上述舞伴配对问题。
【实验提示】
先入队的男士或女士亦先出队配成舞伴。因此该问题具体有典型的先进先出特性,可用队列作为算法的数据结构。在算法中,假设男士和女士的记录存放在一个数组中作为输入,然后依次扫描该数组的各元素,并根据性别来决定是进入男队还是女队。当这两个队列构造完成之后,依次将两队当前的队头元素出队来配成舞伴,直至某队列变空为止。此时,若某队仍有等待配对者,算法输出此队列中等待者的人数及排在队头的等待者的名字,他(或她)将是下一轮舞曲开始时第一个可获得舞伴的人。
【实验要求】
利用队列实现,存储结构采用顺序或链式均可
输入:
10
Asd F
Qwe M
Wer F
Wqw M
Zxc M
Sdf F
Erf M
Dfg M
Edc F
Rty F
9
Asd F
Qwe M
Wer F
Wqw M
Zxc M
Sdf F
Erf M
Dfg M
Edc F
输出:
F:Asd M:Qwe
F:Wer M:Wqw
F:Sdf M:Zxc
F:Edc M:Erf
F:Rty M:Dfg
The partner matches exactly.
F:Asd M:Qwe
F:Wer M:Wqw
F:Sdf M:Zxc
F:Edc M:Erf
The first man to get a partner is:Dfg
CODE:
#include <iostream>
#include <cstdlib>
using namespace std;
const int MAXSIZE=100;
typedef struct
{
char name[20];
char sex;
}Person;
typedef struct
{
Person *base;
int front;
int rear;
}SqQueue;
SqQueue Mdancers,Fdancers;
void InitQueue(SqQueue &q)
{
q.base=new Person[MAXSIZE];
if(!q.base)
exit(1);
q.front=q.rear=0;
return;
}
void EnQueue(SqQueue &q,Person p)
{
if((q.rear+1)%MAXSIZE==q.front)
{
return;
}
q.base[q.rear]=p;
q.rear=(q.rear+1)%MAXSIZE;
return;
}
void DancePartner(Person dancer[],int num)
{
InitQueue(Mdancers);
InitQueue(Fdancers);
int i;
Person p;
for(i=0;i<num;i++)
{
p=dancer[i];
if(p.sex=='F')
EnQueue(Fdancers,p);
else
EnQueue(Mdancers,p);
}
while(Mdancers.rear!=Mdancers.front&&Fdancers.rear!=Fdancers.front)
{
cout<<"F:"<<Fdancers.base[Fdancers.front].name<<" ";
Fdancers.front=(Fdancers.front+1)%MAXSIZE;
cout<<"M:"<<Mdancers.base[Mdancers.front].name<<endl;
Mdancers.front=(Mdancers.front+1)%MAXSIZE;
}
if(Mdancers.rear!=Mdancers.front)
{
cout<<"The first man to get a partner is:"<<Mdancers.base[Fdancers.front].name<<endl;
}
else if(Fdancers.rear!=Fdancers.front)
{
cout<<"The first woman to get a partner is:"<<Fdancers.base[Fdancers.front].name<<endl;
}
else
{
cout<<"The partner matches exactly."<<endl;
}
}
int main()
{
cout<<"Input the number of dancers:";
int n,i;
cin>>n;
cout<<"Input the dancer's name and gender:"<<endl;
Person dancer[MAXSIZE];
for(i=0;i<n;i++)
{
cin>>dancer[i].name>>dancer[i].sex;
}
DancePartner(dancer,n);
return 0;
}
题目四:Rails
[Description]
There is a famous railway station in PopPush City. Country there is incredibly hilly. The station was built in last century. Unfortunately, funds were extremely limited that time. It was possible to establish only a surface track. Moreover, it turned out that the station could be only a dead-end one (see picture) and due to lack of available space it could have only one track.
The local tradition is that every train arriving from the direction A continues in the direction B with coaches reorganized in some way. Assume that the train arriving from the direction A has N <= 1000 coaches numbered in increasing order 1, 2, …, N. The chief for train reorganizations must know whether it is possible to marshal coaches continuing in the direction B so that their order will be a1, a2, …, aN. Help him and write a program that decides whether it is possible to get the required order of coaches. You can assume that single coaches can be disconnected from the train before they enter the station and that they can move themselves until they are on the track in the direction B. You can also suppose that at any time there can be located as many coaches as necessary in the station. But once a coach has entered the station it cannot return to the track in the direction A and also once it has left the station in the direction B it cannot return back to the station.
[Input]
The input consists of blocks of lines. Each block except the last describes one train and possibly more requirements for its reorganization. In the first line of the block there is the integer N described above. In each of the next lines of the block there is a permutation of 1, 2, …, N. The last line of the block contains just 0.
The last block consists of just one line containing 0.
[Output]
The output contains the lines corresponding to the lines with permutations in the input. A line of the output contains Yes if it is possible to marshal the coaches in the order required on the corresponding line of the input. Otherwise it contains No. In addition, there is one empty line after the lines corresponding to one block of the input. There is no line in the output corresponding to the last ``null’’ block of the input.
[Sample Input]
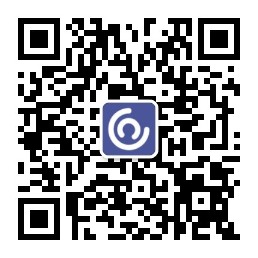
5
1 2 3 4 5
5 4 1 2 3
0
6
6 5 4 3 2 1
0
0
[Sample Output]
Yes
No
Yes
CODE:
#include <iostream>
#include <cstdlib>
using namespace std;
const int MAXSIZE=1100;
typedef struct
{
char *base;
char *top;
int l;
} SqStack;
void InitStack(SqStack &s)
{
s.base=new char[MAXSIZE];
if(!s.base)
exit(1);
s.top=s.base;
s.l=MAXSIZE;
return;
}
void Push(SqStack &s,int ch)
{
if(s.top-s.base==s.l)
return;
*s.top++=ch;
return;
}
typedef struct
{
int *base;
int front;
int rear;
} SqQueue;
void InitQueue(SqQueue &q)
{
q.base=new int[MAXSIZE];
if(!q.base)
exit(1);
q.front=q.rear=0;
return;
}
void EnQueue(SqQueue &q,int ch)
{
if((q.rear+1)%MAXSIZE==q.front)
exit(1);
q.base[q.rear]=ch;
q.rear=(q.rear+1)%MAXSIZE;
return;
}
int main()
{
int n;
while(cin>>n)
{
if(n==0)
break;
while(true)
{
SqQueue q;
InitQueue(q);
int t,i;
for(i=0;i<n;i++)
{
cin>>t;
if(t==0)
break;
EnQueue(q,t);
}
if(i<n)
break;
SqStack s;
InitStack(s);
t=1;
while(q.front!=q.rear)
{
if(s.base==s.top)
{
Push(s,t);
t++;
}
else if(*(s.top-1)!=q.base[q.front])
{
Push(s,t);
t++;
}
else if(*(s.top-1)==q.base[q.front])
{
s.top--;
q.front=(q.front+1)%MAXSIZE;
}
if(t==n+2)
break;
}
if(q.front!=q.rear||t==n+2)
cout<<"No"<<endl;
else
cout<<"Yes"<<endl;
}
}
return 0;
}