给定一个二叉树,找出其最大深度。
二叉树的深度为根节点到最远叶子节点的最长路径上的节点数。
说明: 叶子节点是指没有子节点的节点。
方法一:递归
====================Python=========================
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution: def maxDepth(self, root: TreeNode) -> int: if not root: return 0 return max(self.maxDepth(root.left),self.maxDepth(root.right)) + 1
====================Java====================
/**
* Definition for a binary tree node.
* public class TreeNode {
* int val;
* TreeNode left;
* TreeNode right;
* TreeNode(int x) { val = x; }
* }
*/
class Solution { public int maxDepth(TreeNode root) { if (root == null) { return 0; } return Math.max(maxDepth(root.left), maxDepth(root.right)) + 1; } }
====================Golang=========================
/**
* Definition for a binary tree node.
* type TreeNode struct {
* Val int
* Left *TreeNode
* Right *TreeNode
* }
*/
func maxDepth(root *TreeNode) int { if root == nil { return 0 } return max(maxDepth(root.Left), maxDepth(root.Right)) + 1 } func max(a, b int) int{ if a > b { return a } else { return b } }
====================PHP==============================
/**
* Definition for a binary tree node.
* class TreeNode {
* public $val = null;
* public $left = null;
* public $right = null;
* function __construct($value) { $this->val = $value; }
* }
*/
class Solution { /** * @param TreeNode $root * @return Integer */ function maxDepth($root) { if (!$root) { return 0; } return max($this->maxDepth($root->left), $this->maxDepth($root->right)) + 1; } }
扫描二维码关注公众号,回复:
11461846 查看本文章
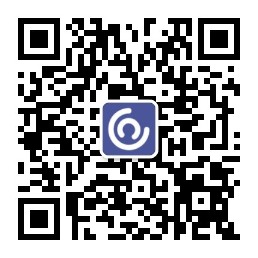
方法二:BFS
====================Python====================
class Solution: def maxDepth(self, root: TreeNode) -> int: if root is None: return 0 from collections import deque q = deque() q.append(root) q_help = deque() res = 0 while q_help or q: while q: x = q.popleft() if x.left: q_help.append(x.left) if x.right: q_help.append(x.right) res += 1 q = q_help q_help = deque() return res
====================Java====================
class Solution { public int maxDepth(TreeNode root) { if (root == null) { return 0; } Queue<TreeNode> queue = new LinkedList<TreeNode>(); queue.offer(root); int ans = 0; while (!queue.isEmpty()) { int size = queue.size(); while (size > 0) { TreeNode node = queue.poll(); if (node.left != null) { queue.offer(node.left); } if (node.right != null) { queue.offer(node.right); } size--; } ans++; } return ans; } }
====================Golang====================
func maxDepth(root *TreeNode) int { if root == nil { return 0 } queue := []*TreeNode{} queue = append(queue, root) ans := 0 for len(queue) > 0 { sz := len(queue) for sz > 0 { node := queue[0] queue = queue[1:] if node.Left != nil { queue = append(queue, node.Left) } if node.Right != nil { queue = append(queue, node.Right) } sz-- } ans++ } return ans }