这里写目录标题
1、Scanner
Scanner是一个可以解析基本类型和字符串的简单扫描器
一般用于接收控制台输入
Scanner sc = new Scanner(System.in)
//接收数字
sc.nextInt();
//接收字符串
sc.next();
2、Random
(随机)
生成随机数
Random r = new Random();
int num = r.nextInt();
System.out.println("随机数是:" + num);
//生成0-9随机数
int num2 = r.nextInt(10);
System.out.println(num2);
//生成1-10随机数
int num3 = r.nextInt(10)+1;
System.out.println(num3);
3、 ArrayList
/***
* 当arraylist是空时 为:[]
*/
ArrayList<String> list = new ArrayList();
System.out.println(list);
//添加
list.add("a");
list.add("b");
list.add("c");
//索引获取值
System.out.println(list.get(0));
/***
* 根据索引修改值
* 注意:不能超过索引
*/
list.set(0,"b");
//输出长度
System.out.println(list.size());
//删除
list.remove(1);
4、String
String实际上就是一个字节数组 char[]
看看他的构造方法就懂了
public String();
public String(char[] array)
public String(byte[] array)
直接用双引号赋值的字符串存在于堆字符串常量池内
而new 出来的则存在于对象内
其常用的方法:
public int length() //长度
public String contact() //连接成为一个新的字符串
public char charAt(int index) //获取指定索引位置的字符
public int indexOf(String str) //获取字符串的位置,没有则返回-1
public String substring(int index) //截取字符串,从index开始到结束
public String substring(int begin,int end) //截取字符串,从begin到end[begin,end)
public char[] toCharArray(); //将当前字符串拆分成字节数组
public byte getBytes(); //获得当前字符串底层的字节码
public String replace(); //将某个字符串替换成另一个字符串
public String[] splice(String regex); //切割字符。注意 regex 是一个正则表达式。
String 是final类型意味着它是不可改的
public final class String
但是为什么以下是成立的呢?因为下面的做法不是改变内容,而是改变地址值,到新的内容
String str="A";
str="B";
5、Arrays
Arrays主要是与一个数组相关的工具类,里面提供了大量的静态方法
扫描二维码关注公众号,回复:
11453018 查看本文章
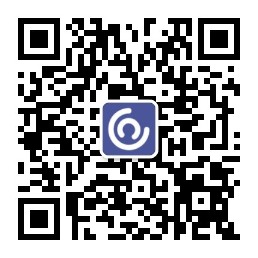
public static void String toString(); //将数组转成字符串,例:[元素1,元素2,...]
public static void sort() //排序
/***
*1.如果数组元素是数字,则默认为升序
*2.如果数组元素为字符,则默认为字母升序
*3.如果是一个自定义类,则他需要Comparable或者Comparator接口的支持
***/
6、Math
Math主要是一个与数学相关的工具类,里面包含大量的静态方法
public static double abs(double num) //获取绝对值
public static double ceil(double num) //向上取整
public static double floor(double num) //向下取整
public static Long round(double num) //四舍五入
7、Date和DateFormate和Calendar
Date类 是表示特定的瞬间,精确到毫秒
DateFormat类 是时间格式化类,我们可以通过这个类完成日期和文本之间的转换
Calendar 日历类 ,里面提供了许多日历字段的方法(year,month等)
//日期转字符串
String format(Date date);
//字符串转日期
Date parse(String date);
//时间格式定义 yyyyMMdd HH:mm:ss
SimpleDateFormate(String pattern)
8、StringBuilder
这是字符串缓冲区,可以提高字符串的操作效率
他的底层是一个数组,但是没有被final修饰,可以改变长度
//添加任意字符串
public StringBuilder append();
//转成String类型
public String toString(String str);
9、==和equal
对于基本来兴来说“==”是数值的比较
对于引用类型来说“==”是地址值的比较
String一般都用equal比较
equal直接比较数值
objiect.equal(object)
注意:前面object不能为空
注意: 这里说一下一个更好的比较方法 Object.equal(str,str);
Object是一个object的工具类,使用这个equal方法的好处是:他不要要注意空值
equalsIgnoreCase 忽略字母大小写
10、Collection
//直接往集合添加多个元素
public static <T> boolean addAll(collection<T> c,T... element);
//打乱集合顺序
public void shuffle(List<?> lsit);
//按照默认规则排序
public static <T> boolean sort(List<T> list);
//按照指定规则排序
public static <T> boolean sort(List<T> list,Comparator<? super T>);