1.项目结构搭建
2.相关依赖
<!--web相关-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!--测试相关-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
</dependency>
<!-- 实现mybatis的核心 -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>LATEST</version>
</dependency>
<!-- jdbc连接mysql的支持-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.25</version>
</dependency>
3.配置相关
application.peoperties
<!--web相关-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!--测试相关-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
</dependency>
<!-- 实现mybatis的核心 -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>LATEST</version>
</dependency>
<!-- jdbc连接mysql的支持-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.25</version>
</dependency>
mybatis-config.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<settings>
<!-- 开启驼峰命名 -->
<setting name="mapUnderscoreToCamelCase" value="true"/>
<!-- 打印sql -->
<!--<setting name="logImpl" value="STDOUT_LOGGING"/>-->
</settings>
<!--指定包批量起别名,默认类名(不区分大小写)-->
<typeAliases>
<package name="com.example.demo.bean"/>
</typeAliases>
</configuration>
UserMapper.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" >
<mapper namespace="com.example.demo.dao.UserMapper">
<!-- 自定义封装规则 -->
<resultMap id="rm" type="User">
<id property="id" column="id" javaType="java.lang.Integer" jdbcType="INTEGER"/>
<result property="name" column="name" javaType="java.lang.String" jdbcType="VARCHAR"/>
<result property="age" column="age" javaType="java.lang.Integer" jdbcType="INTEGER"/>
</resultMap>
<!-- 增 -->
<insert id="save" parameterType="User">
insert into user
<trim prefix="(" suffix=")" suffixOverrides=","><!--前后缀重写用于去除多余的内容-->
<if test="id != null">
id,
</if>
<if test="name != null">
name,
</if>
<if test="age != null">
age
</if>
</trim>
<trim prefix="values (" suffix=")" suffixOverrides=",">
<if test="id != null">
#{id,jdbcType=INTEGER},
</if>
<if test="name != null">
#{name,jdbcType=VARCHAR},
</if>
<if test="age != null">
#{age,jdbcType=INTEGER},
</if>
</trim>
</insert>
<!-- 删 -->
<delete id="deleteById">
delete from user where id=#{id}
</delete>
<!-- 改 --><!--#{}中指定jdbcType可以解决插入空值引起的问题-->
<update id="update" parameterType="User">
update user
<set>
<if test="name != null">
name = #{name,jdbcType=VARCHAR},
</if>
<if test="age != null">
age = #{age,jdbcType=INTEGER},
</if>
</set>
where id = #{id,jdbcType=INTEGER}
</update>
<!-- 查 -->
<select id="selectAll" resultMap="rm">
select * from user
</select>
<select id="selectById" resultMap="rm">
select
*
from user
where id = #{id,jdbcType=INTEGER}
</select>
</mapper>
4.代码实现
User
package com.example.demo.bean;
public class User {
private Integer id;
private String name;
private Integer age;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
@Override
public String toString() {
return "User{" +
"id=" + id +
", name='" + name + '\'' +
", age=" + age +
'}';
}
}
MybatisUserController
package com.example.demo.controller;
import com.example.demo.bean.User;
import com.example.demo.dao.UserMapper;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController
public class MybatisUserController {
@Autowired
UserMapper userMapper;
@GetMapping("/save")
public String save(){
System.out.println("[save userMapper]"+userMapper);
User user=new User();
user.setName("东哥");
user.setAge(20);
int num=userMapper.save(user);
System.out.println("[insert]"+num);
return "添加"+num+"条记录";
}
@GetMapping("/getAll")
public List<User> getAll(){
System.out.println("[getAll userMapper]"+userMapper);
List<User> users=userMapper.selectAll();
System.out.println("[select]"+users.size());
return users;
}
@GetMapping("/update")
public int update(){
System.out.println("[update userMapper]"+userMapper);
User update=new User();
update.setName("xqq3");
update.setId(5);
int num = userMapper.update(update);
System.out.println(num>0?"修改成功":"");
return num;
}
@GetMapping("/delete")
public int delete(){
System.out.println("[delete userMapper]"+userMapper);
int num = userMapper.deleteById(1);
System.out.println(num>0?"删除成功":"删除失败");
return num;
}
@GetMapping("/getOne")
public User getOne(){
System.out.println("[getOne userMapper]"+userMapper);
User user = userMapper.selectById(2);
System.out.println(user!=null?"查到1条":"查到0条");
return user;
}
}
UserMapper
扫描二维码关注公众号,回复:
11449490 查看本文章
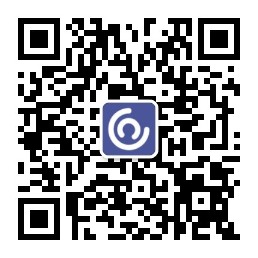
package com.example.demo.dao;
import com.example.demo.bean.User;
import java.util.List;
//@Repository
public interface UserMapper {
int save(User user);//新增用户
int update(User user);//更新用户信息
int deleteById(int id);//根据id删除
User selectById(int id);//根据id查询
List<User> selectAll();//查询所有用户信息
}
DemoApplication
package com.example.demo;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
@MapperScan("com.example.demo.dao") //会注册bean
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
基于注解版的,GitHub:https://github.com/atkgc2019wxd/springboot-learning