PS:在看到这个题目的同时,你们估计会想,Volley与Glide怎么拿来一块说呢,他们虽然不是一个框架,但有着相同功能,那就是图片处理方面。首先我们先来看一下什么volley,又什么是glide。
Volley是Google官方出的一套小而巧的异步请求库,该框架封装的扩展性很强,支持OkHttp,Volley里面也封装了ImageLoader,自身作为图片加载框架,不过这块功能没有一些专门的图片加载框架强大,对于简单的需求可以使用,对于稍复杂点的需求还是需要用到专门的图片加载框架。Volley也有缺陷,比如不支持post大数据,所以不适合上传文件。不过Volley设计的初衷本身也就是为频繁的、数据量小的网络请求而生!
个人建议:
如果请求的数据比较小的话,建议用volley,因为它代码量小,效果高,但是如果是下载大型文件(视频),那就不要用它了。
首先 AndroidStudio中引入Volley三种方法
- 引入volley.jar文件
- 添加volley到gradle依赖
compile 'com.mcxiaoke.volley:library:1.0.19'
- 通过git下载volley,添加为项目module
1:StringRequest
先热热身,传入一个百度链接,返回一些数据。
1.1简单请求一个网络地址并返回数据,创建队列
RequestQueue queue=Volley.newRequestQueue(context);
1.2在需要的地方创建StringRequest(参数..)
- GET/POST
- url地址
- 响应监听
- 错误监听
String url = "http://www.baidu.com"; StringRequest request = new StringRequest(Request.Method.GET,url,new Response.Listener<String>(){ @Override public void onResponse(String response) {
result = SecuritUtil.aesBase64Decode(response); }
,new ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
} } });
1.3最后处理要加入到队列中
queue.add(request);
我去,这就可以了,我自己都紧张了,记得以前用httpconnect的时候,写的真的是多,还要配置很多的东西,就连retrofit都要写注解什么的。retrofit我之前有些文章,不怎么会用的同志可以去看看。好了,数据是出来了,我没有截图,大家了解,这什么都不传是简单,但如果想传值呢,那就POST方法呗。
2:POST带参数请求
在创建StringRequest方法前,我们先看一下源码方法,4个参数。
/** * Creates a new request with the given method. * * @param method the request {@link Method} to use * @param url URL to fetch the string at * @param listener Listener to receive the String response * @param errorListener Error listener, or null to ignore errors */ public StringRequest(int method, String url, Listener<String> listener, ErrorListener errorListener) { super(method, url, errorListener); mListener = listener; }
2.1:还是一样的写创建一个StringRequest,看注释
StringRequest request = new StringRequest(Request.Method.POST, url, new Response.Listener<String>() { @Override public void onResponse(String response) { //成功后 } }, new Response.ErrorListener() { @Override public void onErrorResponse(VolleyError error) { //失败后 } }) {//传值方法书写位置 @Override protected Map<String, String> getParams() throws AuthFailureError { HashMap<String, String> map = new HashMap<>(); map.put("name", "liu"); map.put("id", "123456789"); return map; } };
//这里需要注意的是getParams()方法是写在StringRequest(内)的,括号标红。
2.2最后要把该对象放在queue中
queue.add(request);
这就完事了,传值直接写上就OK了,都是键值对的形式。到这估计有人觉得这是传普通值,如果我传JSON呢,有有有,下面就是。
3:JSON格式传参和接受数据
这个JSON传值话也是分GET和PSOT方法,GET一般都不传值,直接填""。POST则是用专用类JsonObjectRequest,如果你觉得不过瘾还可以用
/** * Creates a new request. * @param method the HTTP method to use * @param url URL to fetch the JSON from * @param requestBody A {@link String} to post with the request. Null is allowed and * indicates no parameters will be posted along with request. * @param listener Listener to receive the JSON response * @param errorListener Error listener, or null to ignore errors. */ public JsonObjectRequest(int method, String url, String requestBody, Listener<JSONObject> listener, ErrorListener errorListener) { super(method, url, requestBody, listener, errorListener); }
3.1:请求方式GET,无参数传入
JsonObjectRequest json=new JsonObjectRequest(Request.Method.GET, url, "", new Response.Listener<JSONObject>() { @Override public void onResponse(JSONObject response) { } }, new Response.ErrorListener() { @Override public void onErrorResponse(VolleyError error) { } });
3.2:请求方式POST
JSONObject jsonO=new JSONObject(); try { jsonO.put("name",""); jsonO.put("ID",""); } catch (JSONException e) { e.printStackTrace(); }//创建JSONObject对象 JsonObjectRequest json=new JsonObjectRequest(Request.Method.POST, url, jsonO, new Response.Listener<JSONObject>() { @Override public void onResponse(JSONObject response) { //ok } }, new Response.ErrorListener() { @Override public void onErrorResponse(VolleyError error) { //error } });
3.3:最后要把该对象放在queue中
queue.add(request);
到这里volley怎么用来访问网络数据就完事了,到现在还没有说他的图片处理,不过这个框架真心好用,所以就写的多了点。下面咱们来看一下他的图片处理
4:ImageRequest, 图片加载
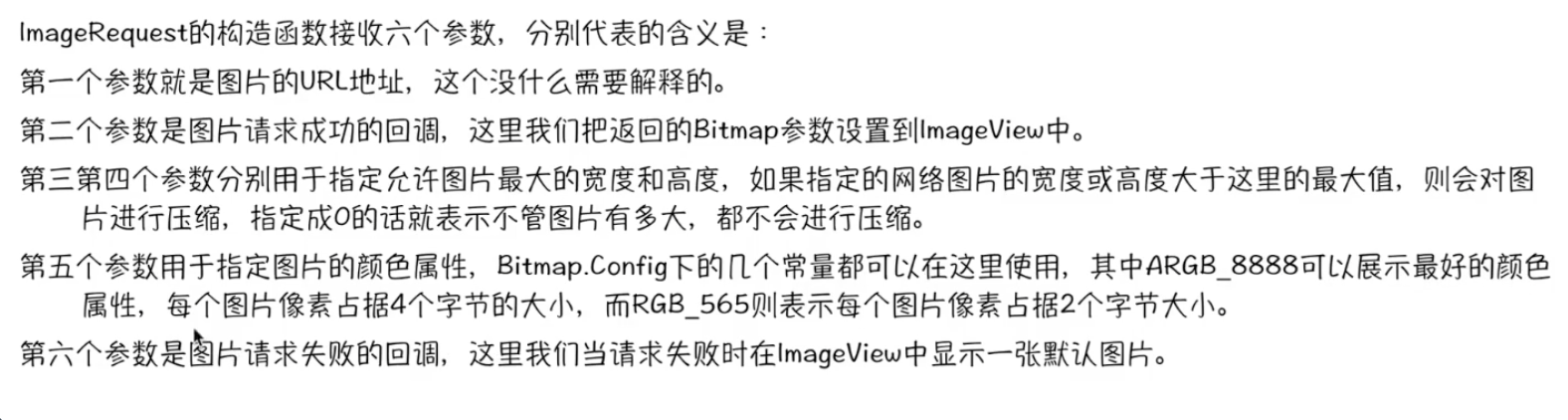
/** * Creates a new image request, decoding to a maximum specified width and * height. If both width and height are zero, the image will be decoded to * its natural size. If one of the two is nonzero, that dimension will be * clamped and the other one will be set to preserve the image's aspect * ratio. If both width and height are nonzero, the image will be decoded to * be fit in the rectangle of dimensions width x height while keeping its * aspect ratio. * * @param url URL of the image * @param listener Listener to receive the decoded bitmap * @param maxWidth Maximum width to decode this bitmap to, or zero for none * @param maxHeight Maximum height to decode this bitmap to, or zero for * none * @param scaleType The ImageViews ScaleType used to calculate the needed image size. * @param decodeConfig Format to decode the bitmap to * @param errorListener Error listener, or null to ignore errors */ public ImageRequest(String url, Response.Listener<Bitmap> listener, int maxWidth, int maxHeight, ScaleType scaleType, Config decodeConfig, Response.ErrorListener errorListener) { super(Method.GET, url, errorListener); setRetryPolicy( new DefaultRetryPolicy(IMAGE_TIMEOUT_MS, IMAGE_MAX_RETRIES, IMAGE_BACKOFF_MULT)); mListener = listener; mDecodeConfig = decodeConfig; mMaxWidth = maxWidth; mMaxHeight = maxHeight; mScaleType = scaleType; }
用法:每个参数是什么我都在上面写好,第几个参数是干什么的,还有源码供大家参考。url为图片地址
ImageRequest request =new ImageRequest(url,Response.Listener<Bitmap>(){ @Override public void onResponse(Bitmap s) { Log.i("aa", "post请求成功" + s); } } ,0,0,Bitmap.config.RGB_565,new Response.ErrorListener() { @Override public void onErrorResponse(VolleyError volleyError) {
Log.i("aa", "post请求失败" + s);
});
5:ImageLoader 图片缓存机制(推荐使用)
在普通版中自身是调用自己的缓存类,这个是我们不能控制的,如果想要控制的就要自己写类来实现ImageLoader.ImageCache,这就相当于我们的自定义View,或者自定义适配器,我们可以更好的去控制我们想要的结果,比如说,我们要它最大缓存量是10M,超过这个值会发出警报等。
下面来说简单用法
ImageLoader imageLoader = new ImageLoader(requestQueue, new ImageLoader.ImageCache() { @Override public Bitmap getBitmap(String url) { //具体操作,主要针对对缓存数据大小、如何缓存。 return null; } @Override public void putBitmap(String url, Bitmap bitmap) { } }); //imgShow是imageview控件。后面参数分类是失败和过程时出现的图片 ImageLoader.ImageListener listener = ImageLoader.getImageListener(imgShow, R.mipmap.ic_launcher, R.drawable.btn_add_true); imageLoader.get(url, listener, 200, 200);
上面这个就可以对图片进行处理,不过还有一个就是定义接口,里面有两个方法,一个放一个是取,重点是标红
public class ImageCache implements ImageLoader.ImageCache{ //LruCache 是专门用于缓存的类,String可以作为缓存入后的名称,Bitmap是位图。 public LruCache<String,Bitmap> lruCache; public int maxCache=10 * 1024 *1024;//最大缓存大小 10M public ImageCache (){ lruCache=new LruCache<>(maxCache);//实例化创建 } @Override public Bitmap getBitmap(String url) {//得到位图 return lruCache.get(url); } @Override public void putBitmap(String url, Bitmap bitmap) {//存入位图 lruCache.put(url,bitmap); } }
6:NetWorkImageView自动适配图片(控件)
netimg = (NetworkImageView) findViewById(R.id.id_net_img); netimg.setErrorImageResId(R.mipmap.ic_launcher);//错误后 netimg.setDefaultImageResId(R.drawable.btn_add_true);//加载中默认 //这里new ImageCache()是上面自己写的类 netimg.setImageUrl(url,new ImageLoader(queue,new ImageCache()));
到这里volley基本用法就已经够用了,原本想写点Glide的用法呢,还有对比,这一篇写的就不少了。大家可以消化一下,下一篇我写Glide的简单用法,然后是Volley对比Glide。
总结:
但是如果有一个listview了,GridView了等加载图片的话,可以用Volley,尤其是最后一个NetWorkImageView,可以自动适配图片大小,然后统一作出判断到底该多大才能更好的呈现给用户。每一个框架都是一些人的心血,肯定是优点爆棚的,对于程序员来讲一个好的工具对以后的开发是多么的重要,一个功能省去了一些代码,功能多了代码就非诚客观了,而且简介明了规范。谢谢大家的支持。