一、使用 Spring Data JPA 中接口定义的方法
在继承 JpaRepository,和 JpaRepository 接口后,我们就可以使用接口中定义的方法进行查询
- 继承 JpaRepository 后的方法列表
- 继承 JpaSpecificationExecutor 的方法列表
例如:
/**
* 测试统计查询:查询客户的总数量
* count:统计总条数
*/
@Test
public void testCount() {
long count = customerDao.count();//查询全部的客户数量
System.out.println(count);
}
/**
* 测试:判断id为4的客户是否存在
* 1. 可以查询以下id为4的客户
* 如果值为空,代表不存在,如果不为空,代表存在
* 2. 判断数据库中id为4的客户的数量
* 如果数量为0,代表不存在,如果大于0,代表存在
*/
@Test
public void testExists() {
boolean exists = customerDao.exists(2l);
System.out.println("id为4的客户 是否存在:"+exists);
}
/**
* 根据id从数据库查询
* @Transactional : 保证getOne正常运行
*
* findOne:
* em.find() :立即加载
* getOne:
* em.getReference :延迟加载
* * 返回的是一个客户的动态代理对象
* * 什么时候用,什么时候查询
*/
@Test
@Transactional
public void testGetOne() {
Customer customer = customerDao.getOne(2l);
System.out.println(customer);
}
二、使用JPQL的方式查询
已经学习过:一篇文章带你搞定 JPA 中的复杂查询(JPQL)
这里我们来学习下 SpringDataJPA 中的JPQL 的用法
使用 Spring Data JPA 提供的查询方法已经可以解决大部分的应用场景,但是对于某些业务来说,我们还需要灵活的构造查询条件,这时就可以使用@Query
注解,结合 JPQL 的语句方式完成查询
(1)根据客户名称查询客户
public interface CustomerDao extends JpaRepository<Customer, Long>, JpaSpecificationExecutor<Customer> {
/**
* 案例:根据客户名称查询客户
* 使用jpql的形式查询
* jpql:from Customer where custName = ?
* <p>
* 配置jpql语句,使用的@Query注解
*/
@Query(value = "from Customer where custName = ? ")
public Customer findJpql(String custName);
}
测试:
(2)根据客户名称和客户 id 查询客户
public interface CustomerDao extends JpaRepository<Customer, Long>, JpaSpecificationExecutor<Customer> {
/**
* 案例:根据客户名称和客户id查询客户
* jpql: from Customer where custName = ? and custId = ?
* <p>
* 对于多个占位符参数
* 赋值的时候,默认的情况下,占位符的位置需要和方法参数中的位置保持一致
* <p>
* 可以指定占位符参数的位置
* ? 索引的方式,指定此占位的取值来源
*/
@Query(value = "from Customer where custName = ?2 and custId = ?1")
public Customer findCustNameAndId(Long id, String name);
}
注意对于占位符的顺序,可以使用默认的顺序依次对应,也可以自己如上述指定对应顺序
测试:
(3)根据id更新,客户的名称
public interface CustomerDao extends JpaRepository<Customer, Long>, JpaSpecificationExecutor<Customer> {
/**
* 使用jpql完成更新操作
* 案例 : 根据id更新,客户的名称
* 更新4号客户的名称,将名称改为“NLCs”
* <p>
* sql :update cst_customer set cust_name = ? where cust_id = ?
* jpql : update Customer set custName = ? where custId = ?
*
* @Query : 代表的是进行查询
* * 声明此方法是用来进行更新操作
* @Modifying * 当前执行的是一个更新操作
*/
@Query(value = " update Customer set custName = ?2 where custId = ?1 ")
@Modifying
public void updateCustomer(long custId, String custName);
}
测试:注意更新操作,需要添加事务支持,而且手动配置事务,将自动回滚设置为 false
/**
* 测试jpql的更新操作
* * springDataJpa中使用jpql完成 更新/删除操作
* * 需要手动添加事务的支持
* * 默认会执行结束之后,回滚事务
* @Rollback : 设置是否自动回滚
* false | true
*/
@Test
@Transactional //添加事务的支持
@Rollback(value = false)
public void testUpdateCustomer() {
customerDao.updateCustomer(4l,"NLCs");
}
三、使用 SQL 语句查询
Spring Data JPA同样也支持sql语句的查询,如下:
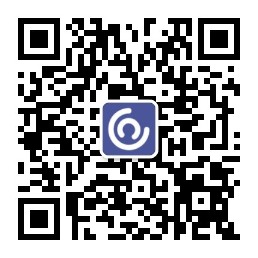
特有的查询:需要在dao接口上配置方法
* 在新添加的方法上,使用注解的形式配置sql查询语句
* 注解 : @Query
value :jpql语句 | sql语句
nativeQuery :false(使用jpql查询) | true(使用本地查询:sql查询)
是否使用本地查询
public interface CustomerDao extends JpaRepository<Customer, Long>, JpaSpecificationExecutor<Customer> {
/**
* 使用sql的形式查询:
* 查询全部的客户
* sql : select * from cst_customer;
* Query : 配置sql查询
* value : sql语句
* nativeQuery : 查询方式
* true : sql查询
* false:jpql查询
*/
//@Query(value = " select * from cst_customer" ,nativeQuery = true)
@Query(value = "select * from cst_customer where cust_name like ?1", nativeQuery = true)
public List<Object[]> findSql(String name);
}
测试:
四、方法命名规则查询
顾名思义,方法命名规则查询就是根据方法的名字,就能创建查询。只需要按照Spring Data JPA提供的方法命名规则定义方法的名称,就可以完成查询工作。Spring Data JPA在程序执行的时候会根据方法名称进行解析,并自动生成查询语句进行查询
按照 Spring Data JPA 定义的规则,查询方法以 findBy 开头,涉及条件查询时,条件的属性用条件关键字连接,要注意的是:条件属性首字母需大写。
框架在进行方法名解析时,会先把方法名多余的前缀截取掉,然后对剩下部分进行解析。
方法名的约定:
* findBy : 查询
* 对象中的属性名(首字母大写) : 查询的条件
* CustName
* 默认情况 : 使用等于的方式查询
* findByCustName -- 根据客户名称查询
在 springdataJpa 的运行阶段
* 会根据方法名称进行解析 findBy from xxx(实体类)
* 属性名称 where custName =
* (1)findBy + 属性名称 (根据属性名称进行完成匹配的查询=)
* (2)findBy + 属性名称 + “查询方式(Like | isnull)”
* findByCustNameLike
* (3)多条件查询
* findBy + 属性名 + “查询方式” + “多条件的连接符(and|or)” + 属性名 + “查询方式”
(1)findBy + 属性名称 (根据属性名称进行完成匹配的查询=)
public interface CustomerDao extends JpaRepository<Customer, Long>, JpaSpecificationExecutor<Customer> {
public Customer findByCustName(String custName);
}
测试:
(2)findBy + 属性名称 + “查询方式(Like | isnull)”
public interface CustomerDao extends JpaRepository<Customer, Long>, JpaSpecificationExecutor<Customer> {
public List<Customer> findByCustNameLike(String custName);
}
(3)多条件查询:注意这里参数位置的对应,因为没有占位符,所以一定要位置对应
public interface CustomerDao extends JpaRepository<Customer, Long>, JpaSpecificationExecutor<Customer> {
//使用客户名称模糊匹配和客户所属行业精准匹配的查询
public Customer findByCustNameLikeAndCustIndustry(String custName, String custIndustry);
}
测试:
具体的关键字,使用方法和生产成SQL如下表所示:
Keyword | Sample | JPQL |
And | findByLastnameAndFirstname | … where x.lastname = ?1 and x.firstname = ?2 |
Or | findByLastnameOrFirstname | … where x.lastname = ?1 or x.firstname = ?2 |
Is,Equals | findByFirstnameIs,findByFirstnameEquals | … where x.firstname = ?1 |
Between | findByStartDateBetween | … where x.startDate between ?1 and ?2 |
LessThan | findByAgeLessThan | … where x.age < ?1 |
LessThanEqual | findByAgeLessThanEqual | … where x.age ⇐ ?1 |
GreaterThan | findByAgeGreaterThan | … where x.age > ?1 |
GreaterThanEqual | findByAgeGreaterThanEqual | … where x.age >= ?1 |
After | findByStartDateAfter | … where x.startDate > ?1 |
Before | findByStartDateBefore | … where x.startDate < ?1 |
IsNull | findByAgeIsNull | … where x.age is null |
IsNotNull,NotNull | findByAge(Is)NotNull | … where x.age not null |
Like | findByFirstnameLike | … where x.firstname like ?1 |
NotLike | findByFirstnameNotLike | … where x.firstname not like ?1 |
StartingWith | findByFirstnameStartingWith | … where x.firstname like ?1 (parameter bound with appended %) |
EndingWith | findByFirstnameEndingWith | … where x.firstname like ?1 (parameter bound with prepended %) |
Containing | findByFirstnameContaining | … where x.firstname like ?1 (parameter bound wrapped in %) |
OrderBy | findByAgeOrderByLastnameDesc | … where x.age = ?1 order by x.lastname desc |
Not | findByLastnameNot | … where x.lastname <> ?1 |
In | findByAgeIn(Collection ages) | … where x.age in ?1 |
NotIn | findByAgeNotIn(Collection age) | … where x.age not in ?1 |
TRUE | findByActiveTrue() | … where x.active = true |
FALSE | findByActiveFalse() | … where x.active = false |
IgnoreCase | findByFirstnameIgnoreCase | … where UPPER(x.firstame) = UPPER(?1) |