题目描述
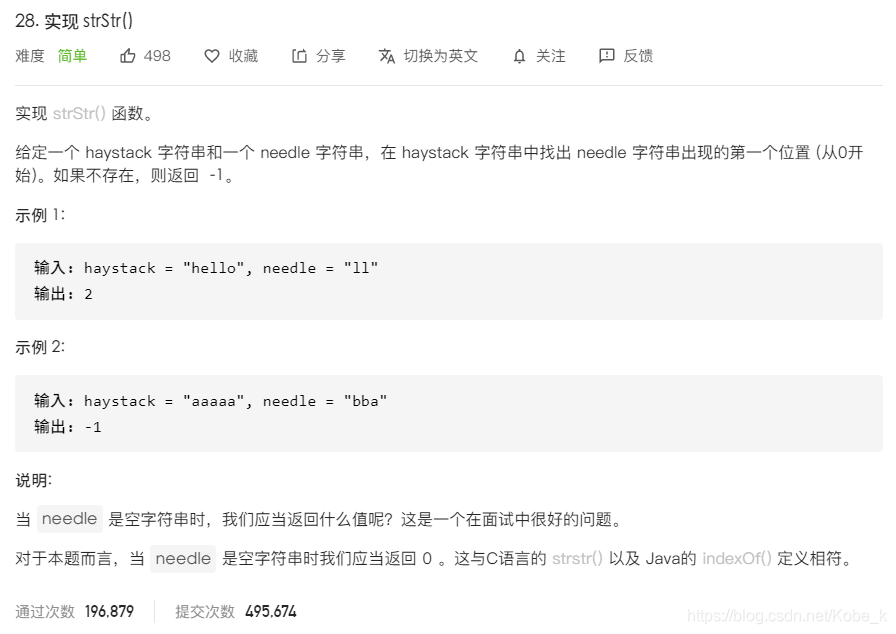
题目分析
- 三种情况,找到、未找到、给定的字符串为空,分别对应的返回结果是:返回第一个字符的索引值、返回-1,返回0
- 难点在于如何匹配字符串
解法分析
- 两个字符串双重嵌套循环,暴力解法,效率不高
- 双指针解法,本质跟上面的解法是一样的,循环遍历两个字符串
- KMP算法或其他字符串匹配算法
代码
class Solution {
public int strStr(String haystack, String needle) {
int h = haystack.length();
int n = needle.length();
if (h<n) return -1;
if (n==0) return 0;
int limit = h-n;
for(int i=0;i<=limit;i++){
if(needle.equals(haystack.substring(i,i+n))) {
return i;
}
}
return -1;
}
}
class Solution {
public int strStr(String haystack, String needle) {
int lh = haystack.length();
int ln = needle.length();
if (ln==0) return 0;
if (lh < ln) return -1;
int[] next = new int[ln];
int len = ln;
next[0] = 0;
for(int i = 1,k=0;i<len;i++){
while (k>0 && needle.charAt(k) != needle.charAt(i)) k=next[k-1];
if (needle.charAt(k) == needle.charAt(i)) k++;
next[i] = k;
}
for(int i = 0;i<len;i++){
System.out.println(next[i]);
}
for(int i=0,j=0;i<lh;i++){
while (j>0 && haystack.charAt(i) != needle.charAt(j)) j = next[j-1];
if ( haystack.charAt(i) == needle.charAt(j)) j++;
if (j==ln) return i-j+1;
}
return -1;
}
}