一、英雄类
这是游戏的主角,我们通过操作英雄在地图上的走动来获得道具,开门与战斗操作。对于英雄我们有等级,血量,攻击,防御,金币数量,红、黄、蓝钥匙数量以及经验值的属性
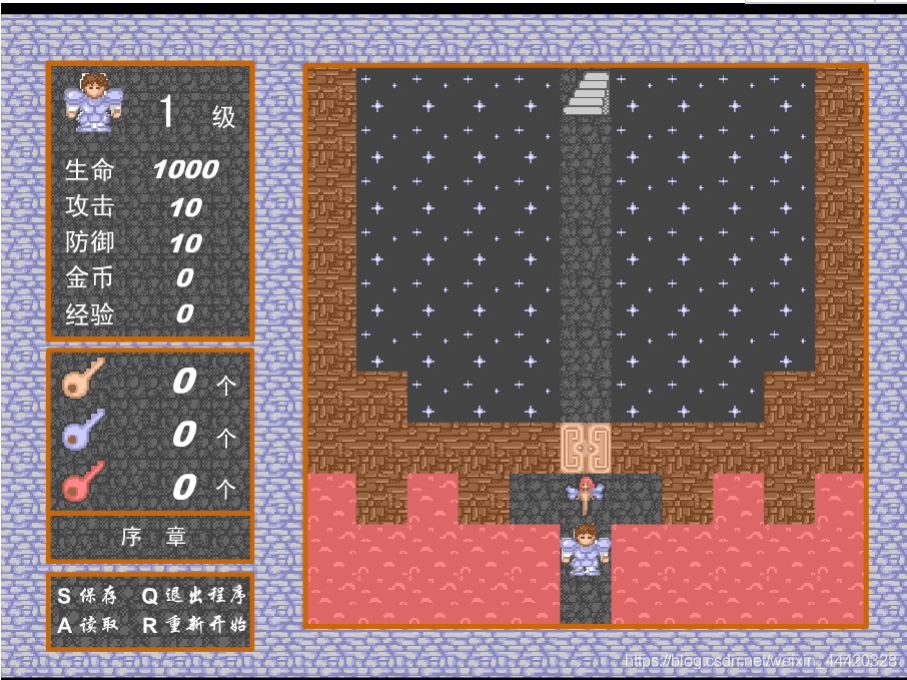
下面展示一些 内联代码片
。
// An highlighted block
package com.yc.opp3.hc0701;
public class Hero {
private int level;
private int blood;
private int att;
private int def;
private int gold;
private int rKey;
private int yKey;
private int bKey;
private int exp;
public Hero() {
this.level = 1;
this.blood = 1000;
this.att = 10;
this.def = 10;
this.gold = 0;
this.rKey = 1;
this.yKey = 1;
this.bKey = 1;
this.exp = 0;
}
public int getExp() {
return exp;
}
public void setExp(int exp) {
this.exp = exp;
}
@Override
public String toString() {
return "勇士等级为:" + level + ", 血量为:" + blood + ", 攻击为:" + att + ", 防御为:" + def + ", 金币为:" + gold
+ ", 红钥匙:" + rKey + ", 黄钥匙:" + yKey + ", 蓝钥匙:" + bKey + ", 经验:" + exp ;
}
public int getLevel() {
return level;
}
public void setLevel(int level) {
this.level = level;
}
public int getBlood() {
return blood;
}
public void setBlood(int blood) {
this.blood = blood;
}
public int getAtt() {
return att;
}
public void setAtt(int att) {
this.att = att;
}
public int getDef() {
return def;
}
public void setDef(int def) {
this.def = def;
}
public int getGold() {
return gold;
}
public void setGold(int gold) {
this.gold = gold;
}
public int getrKey() {
return rKey;
}
public void setrKey(int rKey) {
this.rKey = rKey;
}
public int getyKey() {
return yKey;
}
public void setyKey(int yKey) {
this.yKey = yKey;
}
public int getbKey() {
return bKey;
}
public void setbKey(int bKey) {
this.bKey = bKey;
}
}
二、怪物类
怪物类有怪物名,血量,攻击,防御,击败后获得金币数和经验值的属性
下面展示一些 `内联代码片`。
// An highlighted block
package com.yc.opp3.hc0701;
public class Monster {
private String name;
private int blood;
private int att;
private int def;
private int gold;
private int exp;
public Monster(String name, int blood, int att, int def, int gold, int exp) {
super();
this.name = name;
this.blood = blood;
this.att = att;
this.def = def;
this.gold = gold;
this.exp = exp;
}
@Override
public String toString() {
return "怪物名:" + name + ", 血量:" + blood + ", 攻击:" + att + ", 防御:" + def + ", 金币:" + gold
+ ", 经验:" + exp ;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getBlood() {
return blood;
}
public void setBlood(int blood) {
this.blood = blood;
}
public int getAtt() {
return att;
}
public void setAtt(int att) {
this.att = att;
}
public int getDef() {
return def;
}
public void setDef(int def) {
this.def = def;
}
public int getGold() {
return gold;
}
public void setGold(int gold) {
this.gold = gold;
}
public int getExp() {
return exp;
}
public void setExp(int exp) {
this.exp = exp;
}
}
三、地图类
地图类用一个二维数组来存储地图,第一个下标表示塔的层数,第二个下标表示具体地图
下面展示一些 内联代码片
。
// An highlighted block
package com.yc.opp3.hc0701;
public class Maps {
//这个地图20层,每层10*10
public static String[][] maps = new String[20][100];
static {
// TODO Auto-generated constructor stub
//创建地图
//x表示强 ,h 勇士,a红宝石 d蓝宝石,r红血瓶 b 蓝雪平 ,1-9 表示怪物
//空格表示空地 ijk三种钥匙 IJK三种门
//n 下一层 p上一层
maps[0] = new String[] {
"x","x","x","x","x","x","x","x","x","x",
"x","a","d","x","a","x","d","3","n","x",
"x","x","1","x","1"," ","2","x","J","x",
"x","b"," ","3"," "," "," ","1","a","x",
"x","x","2","x","i","x","x"," ","1","x",
"x","r","a","J"," ","x","a","x"," ","x",
"x","x","I","x"," ","I","d"," ","2","x",
"x","a","1","x","h","1"," ","x","1","x",
"x","r","d","x"," ","x","x","1","a","x",
"x","x","x","x","x","x","x","x","x","x"
};
}
}
四、怪物集合
我们把怪物放在一个集合里面
下面展示一些 内联代码片
。
package com.yc.opp3.hc0701;
public class Monsters {
static Monster m1 = new Monster("绿色史莱姆",50,20,1,1,1);
static Monster m2 = new Monster("红色史莱姆",50,20,8,2,1);
static Monster m3 = new Monster("小蝙蝠",60,25,15,3,3);
static Monster m4 = new Monster("初级魔法师",100,30,10,4,4);
static Monster m5 = new Monster("骷髅人",70,70,0,5,5);
static Monster m6 = new Monster("大蝙蝠",120,50,30,6,6);
public static Monster[] ms = new Monster[] {m1,m2,m3,m4,m5,m6};
}
五、游戏类
游戏类用来对英雄进行操作,开始游戏,创建地图,英雄的移动,遇到不同的对象执行的不同操作。
下面展示一些 内联代码片
。
package com.yc.opp3.hc0701;
import java.util.Scanner;
public class Game {
public Hero hero;
public int mindex = 1;
public int hindex = 74;
public boolean isGameOver = false;
public Scanner sc = new Scanner(System.in);
public void startGame() {
hero = new Hero(); // 实例化对象
//创建地图 显示地图
createMap(mindex);
System.out.println("\n开始游戏");
System.out.println(hero.toString());
System.out.println("请按wsad键进行移动:");
//开始游戏 ,移动勇士
while(!isGameOver) {
String chiose = sc.nextLine();
//开始移动,无视大小写
if("w".equalsIgnoreCase(chiose)) {
walk(-10);
}else if ("s".equalsIgnoreCase(chiose)) {
walk(10);
}else if("a".equalsIgnoreCase(chiose)) {
walk(-1);
}else {
walk(1);
}
}
}
private void createMap(int mindex) {
// TODO Auto-generated method stub
String[] maps = Maps.maps[mindex -1];
for (int i = 0; i < maps.length; i++) {
System.out.print(maps[i] + " ");
//10个换行
if((i + 1) %10 == 0)
System.out.println();
}
}
//移动
public void walk(int step) {
//移动过程中遇到不同对象的判断
//碰到墙壁禁止移动
if("x".equals(Maps.maps[mindex-1][hindex + step])) {
System.out.println();
createMap(mindex);
return;
}
//红血瓶
else if("r".equals(Maps.maps[mindex-1][hindex + step])) {
System.out.println("勇士获得红血瓶,血量+100!");
hero.setBlood(hero.getBlood() + 100);
System.out.println(hero.toString());
}
//蓝血瓶
else if("r".equals(Maps.maps[mindex-1][hindex + step])) {
System.out.println("勇士获得蓝血瓶,血量+500!");
hero.setBlood(hero.getBlood() + 500);
System.out.println(hero.toString());
}
//攻击力
else if("a".equals(Maps.maps[mindex-1][hindex + step])) {
System.out.println("勇士获得红宝石,攻击力+3!");
hero.setAtt(hero.getAtt() + 3);
System.out.println(hero.toString());
}
//防御力
else if("d".equals(Maps.maps[mindex-1][hindex + step])) {
System.out.println("勇士获得蓝宝石,防御力+3!");
hero.setDef(hero.getDef()+3);
System.out.println(hero.toString());
}
//黄钥匙
else if("i".equals(Maps.maps[mindex-1][hindex + step])) {
System.out.println("勇士获得黄钥匙!");
hero.setyKey(hero.getyKey()+1);
System.out.println(hero.toString());
}
//红钥匙
else if("j".equals(Maps.maps[mindex-1][hindex + step])) {
System.out.println("勇士获得红钥匙!");
hero.setrKey(hero.getrKey()+1);
System.out.println(hero.toString());
}
//蓝钥匙
else if("k".equals(Maps.maps[mindex-1][hindex + step])) {
System.out.println("勇士获得蓝钥匙!");
hero.setbKey(hero.getbKey()+1);
System.out.println(hero.toString());
}
//开门
else if ("I".equals(Maps.maps[mindex-1][hindex + step])) {
System.out.println("勇士遇到了黄门,是否开门?Y/N");
String op = sc.nextLine();
if("n".equalsIgnoreCase(op)) {
//不开门,不移动,显示地图
createMap(mindex);
return;
}
//开门判断是否有钥匙
if(hero.getyKey()<=0) {
System.out.println("钥匙不足,不可开门!");
createMap(mindex);
return;
}
//开门,钥匙-1
hero.setyKey(hero.getyKey() - 1);
System.out.println("黄门开启,黄钥匙 - 1");
System.out.println(hero.toString());
}
//红色
else if ("J".equals(Maps.maps[mindex-1][hindex + step])) {
System.out.println("勇士遇到了红门,是否开门?Y/N");
String op = sc.nextLine();
if("n".equalsIgnoreCase(op)) {
//不开门,不移动,显示地图
createMap(mindex);
return;
}
//开门判断是否有钥匙
if(hero.getrKey()<=0) {
System.out.println("钥匙不足,不可开门!");
createMap(mindex);
return;
}
//开门,钥匙-1
hero.setrKey(hero.getrKey() - 1);
System.out.println("红门开启,红钥匙 - 1");
System.out.println(hero.toString());
}
// 蓝色
else if ("K".equals(Maps.maps[mindex-1][hindex + step])) {
System.out.println("勇士遇到了蓝门,是否开门?Y/N");
String op = sc.nextLine();
if("n".equalsIgnoreCase(op)) {
//不开门,不移动,显示地图
createMap(mindex);
return;
}
//开门判断是否有钥匙
if(hero.getbKey()<=0) {
System.out.println("钥匙不足,不可开门!");
createMap(mindex);
return;
}
//开门,钥匙-1
hero.setbKey(hero.getbKey() - 1);
System.out.println("蓝门开启,蓝钥匙 - 1");
System.out.println(hero.toString());
}
else if(" ".equals(Maps.maps[mindex-1][hindex + step])) {
//空格不管
}else {
//剩下的全是数字
String strNum = Maps.maps[mindex - 1][hindex + step];
int mNum = Integer.parseInt(strNum);
System.out.println("遇到怪物:" + Monsters.ms[mNum - 1].toString());
fight(mNum);
}
//移动前位置置空,移动后的位置标记位h
Maps.maps[mindex - 1][hindex] = " ";
Maps.maps[mindex - 1][hindex +step ] = "h";
//改变英雄坐标
hindex += step;
//重新显示地图
System.out.println();
createMap(mindex);
}
//战斗
public void fight(int mNum) {
//先获得原始数据对象
Monster om = Monsters.ms[mNum - 1];
//原始数据不能改变,所以每次战斗都要重新实例化一个对象
Monster m = new Monster(om.getName(), om.getBlood(), om.getAtt(), om.getDef(), om.getGold(), om.getExp());
//战斗逻辑 扣血量 = 攻击 - 防御
int h2m = hero.getAtt() - m.getDef();
int m2h = m.getAtt() - hero.getDef();
if (m2h <= 0) {
System.out.println("怪物防御高于英雄攻击,英雄阵亡!");
isGameOver = true;
return;
}
//避免bug 血量越打越高
m2h = m2h < 0 ? 0 : m2h;
//对砍
do {
//开始 ,勇士攻击怪物
m.setBlood(m.getBlood() - h2m);
if(m.getBlood() <= 0) {
System.out.println("战斗胜利,勇士获得" + m.getGold() + "金币," + m.getExp() + "经验!");
hero.setGold(hero.getGold() + m.getGold());
hero.setExp(hero.getExp() + m.getExp());
break;
}
//怪物反击
hero.setBlood(hero.getBlood() - m2h);
if(hero.getBlood() <= 0) {
System.out.println("战斗失败,英雄死亡!请重新开始游戏");
isGameOver = true;
return;
}
}while(hero.getBlood() < 0 && m.getBlood() > 0);
System.out.println(hero.toString());
}
}
六、测试类,进行游戏的测试
下面展示一些 内联代码片
。
package com.yc.opp3.hc0701;
public class Test {
public static void main(String[] args) {
Game g = new Game();
g.startGame();
}
}
这就是一个简单的魔塔案例,是对于类与对象的简单运用。