主界面设计(随意)
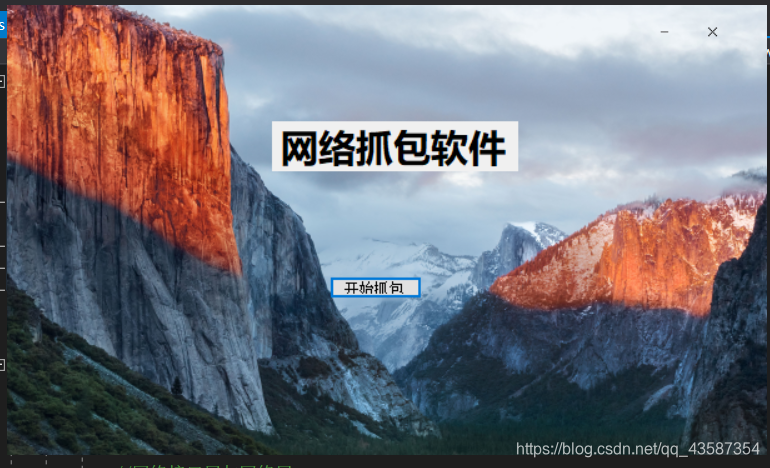
抓包代码
public partial class MainWindow : Form
{
private CaptureDeviceList devices;
public static string[] name = new string[100];
public static int Selectid=-1;
string NowIP="";
public static DeviceMode mode;
public bool State=false;
private BindingSource BS=new BindingSource();
List<ParketAnalysis> listAn = new List<ParketAnalysis>();
private List<ParketStatistics> listSs = new List<ParketStatistics>();
public static Dictionary<string, int> SendCnt = new Dictionary<string, int>();
public static Dictionary<string, int> ReceiveCnt = new Dictionary<string, int>();
int No = 0;
object Lock=new object();
string Filter="";
string FilterIP = "";
public MainWindow()
{
InitializeComponent();
CheckForIllegalCrossThreadCalls = false;
}
private void toolStripButton1_Click(object sender, EventArgs e)
{
devices = CaptureDeviceList.Instance;
if (devices.Count < 1)
{
MessageBox.Show("当前没有活动的网络设备!");
return;
}
Config cf = new Config(devices);
cf.ShowDialog();
if(Selectid==-1)
{
return;
}
else
{
string[] t = devices[Selectid].ToString().Split('\n');
string[] t2 = t[13].Split(':');
NowIP = t2[1];
toolStripLabel1.Text = "准备捕获网络" + name[Selectid] + "的数据包";
}
}
private void toolStripButton2_Click_1(object sender, EventArgs e)
{
if (Selectid == -1)
{
MessageBox.Show("请选择需要捕获的网络!");
return;
}
else if (listAn.Count != 0)
{
#region 是否保存
DialogResult dr1 = MessageBox.Show("重新捕获将清空现有内容,是否继续?", "提示", MessageBoxButtons.OKCancel);
if (dr1 == DialogResult.OK)
{
Reset();
BtnStart.Enabled = false;
btnStop.Enabled = true;
dataGridView.DataSource = BS;
toolStripLabel1.Text = "正在捕获网络" + name[Selectid] + "的数据包";
Start_Capture(devices[Selectid]);
}
else
{
return;
}
#endregion
}
else
{
Reset();
BtnStart.Enabled = false;
btnStop.Enabled = true;
dataGridView.DataSource = BS;
toolStripLabel1.Text = "正在捕获网络" + name[Selectid] + "的数据包";
Start_Capture(devices[Selectid]);
}
}
private void btnStop_Click(object sender, EventArgs e)
{
this.State = false;
BtnStart.Enabled = true;
btnStop.Enabled = false;
toolStripLabel1.Text = "准备捕获网络" + name[Selectid] + "的数据包";
this.Stop();
}
private void Start_Capture(ICaptureDevice dev)
{
this.State = true;
dev.OnPacketArrival += new PacketArrivalEventHandler(Fun_Arrival);
dev.Open(mode);
dev.StartCapture();
}
private void Fun_Arrival(object sender, CaptureEventArgs e)
{
this.BeginInvoke(new MethodInvoker(delegate
{
ParketStatistics temp = new ParketStatistics(No, e.Packet);
lock (Lock)
{
int ID = ConditonType(Filter);
if (ID == 0)
{
BS.Add(temp);
}
else if(ID==1)
{
for (int k = 0; k < temp.ProtocolList.Count; ++k)
{
if (temp.ProtocolList[k] == Filter)
BS.Add(temp);
}
}
else if (ID == 2)
{
if (temp.src == FilterIP)
BS.Add(temp);
}
else if (ID == 3)
{
if (temp.dst == FilterIP)
BS.Add(temp);
}
else if (ID == 4)
{
if (temp.src == FilterIP|| temp.dst == FilterIP)
BS.Add(temp);
}
toolStripLabel1.Text = "正在捕获网络" + name[Selectid] + "的数据包,已捕获:"+(listSs.Count+1)+",已显示:"+BS.Count;
listSs.Add(temp);
ParketAnalysis pa = new ParketAnalysis(temp);
listAn.Add(pa);
if (temp.src == NowIP)
{
if (SendCnt.ContainsKey(temp.dst) == false)
SendCnt.Add(temp.dst, 0);
SendCnt[temp.dst]++;
}
if (temp.dst==NowIP)
{
if (ReceiveCnt.ContainsKey(temp.src) == false)
ReceiveCnt.Add(temp.src, 0);
ReceiveCnt[temp.src]++;
}
No++;
}
}));
}
展示
