一、jQuery 设置宽度和高度
高度操作:
```javascript
$(selector).height(); //不带参数表示获取高度
$(selector).height(200); //带参数表示设置高度
```
宽度操作:
```javascript
$(selector).width(); //不带参数表示获取宽度
$(selector).width(200); //带参数表示设置高宽度
```
jQuery的css()获取高度,和jQuery的height获取高度,二者的区别?
```javascript
$("div").css(); //返回的是string类型,例如:30px
$("div").height(); //返回得失number类型,例如:30。常用于数学计算。
```
如上方代码所示,`$("div").height();`返回的是number类型,常用于数学计算。
二、jQuery 的坐标操作
1、offset()方法
```javascript
$(selector).offset();
$(selector).offset({left:100, top: 150});
```
作用:获取或设置元素相对于 document 文档的位置。参数解释:
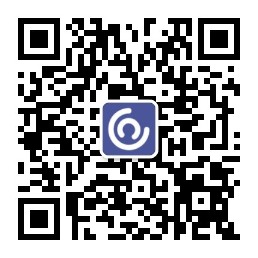
- 无参数:表示获取。返回值为:{left:num, top:num}。返回值是相对于document的位置。
- 有参数:表示设置。参数建议使用 number 数值类型。
注意:设置offset后,如果元素没有定位(默认值:static),则被修改为relative。
2、position()方法
```javascript
$(selector).position();
```
作用:获取相对于其最近的**带有定位**的父元素的位置。返回值为对象:`{left:num, top:num}`。
注意:只能获取,不能设置。
3、scrollTop()方法
```javascript
scrollTop();
$(selector).scrollTop(100);
```
作用:获取或者设置元素被卷去的头部的距离。参数解释:
- 无参数:表示获取偏移。
- 有参数:表示设置偏移,参数为数值类型。
4、scrollLeft()方法
```javascript
scrollLeft();
$(selector).scrollLeft(100);
```
作用:获取或者设置元素水平方向滚动的位置。参数解释:
- 无参数:表示获取偏移。
- 有参数:表示设置偏移,参数为数值类型。
代码示范:
```html
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8">
<title></title>
<style>
body {
height: 5000px;
}
.box1 {
width: 300px;
height: 300px;
position: relative;
margin: 10px;
overflow: auto;
background-color: pink;
}
.box2 {
width: 200px;
height: 400px;
position: absolute;
top: 50px;
left: 50px;
background-color: yellow;
}
</style>
<script src="jquery-1.11.1.js"></script>
<script>
$(function () {
//距离页面最顶端或者最左侧的距离和有没有定位没有关系
$("button").eq(0).click(function () {
alert($(".box2").offset().top);
})
//距离页面最顶端或者最左侧的距离和有没有定位没有关系
$("button").eq(1).click(function () {
$(".box2").offset({"left": 1000, "top": 1000});
})
//距离父系盒子中带有定位的盒子的距离(获取的就是定位值,和margin/padding无关)
$("button").eq(2).click(function () {
alert($(".box2").position().top);
})
//距离父系盒子中带有定位的盒子的距离(获取的就是定位值,和margin/padding无关)
$("button").eq(3).click(function () {
$(".box2").position().top = "100px";
})
//获取被选取的头部
$("button").eq(4).click(function () {
alert($(window).scrollTop());
})
//获取被选取的头部
$("button").eq(5).click(function () {
$(window).scrollTop(100);
})
})
</script>
</head>
<body>
<div class="box1">
<div class="box2"></div>
</div>
<button>offset().top获取</button>
<button>offset().top设置</button>
<button>position().top获取</button>
<button>position().top设置</button>
<button>scrollTop()</button>
<button>scrollTop()</button>
</body>
</html>
```