目录
扫描二维码关注公众号,回复:
11363073 查看本文章
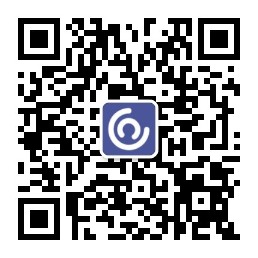
引言:
最近有一个需求需要用到发送邮箱功能,使用的邮箱不一样,自然邮箱的地址也不一样。
下面这篇文章我将简单的描述一下如何发送邮件到企业邮箱。接下来就让我们一起看这个功能如何实现吧!
了解相关概念:
1.我现在用的是腾讯企业邮箱,那么要发送到腾讯企业邮箱上的话,我们首先要有腾讯企业邮箱。腾讯企业邮箱的地址是 smtp.exmail.qq.com
其中可以注意到smtp 这是邮箱的传输协议,
发送邮件用的是SMTP 协议,(处理用户邮箱发送请求的服务器我们通常称之为邮件发送服务器)。
接收邮件用的是POP3协议,(处理用户邮件接收请求的服务器我们称之为邮件接收服务器) 。
准备开发:
(1)我们需要准备一个腾讯企业邮箱的用户名和密码 ,并且需要一个接收方邮箱号。
整个流程如下:
(2)我们需要引入一个Jar包
这是一个为方便Java 开发人员在应用程序中实现邮件发送和接收功能而提供的一套标准开发包,它支持一些常用的邮件协议 比如上面将的SMTP ,POP3 等。
<!-- 发送邮件的maven -->
<dependency>
<groupId>javax.mail</groupId>
<artifactId>mail</artifactId>
<version>1.4.7</version>
</dependency>
(3)编写Controller
package com.test.sendmail;
import io.swagger.annotations.ApiOperation;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
/**
* @Author tanghh
* @Date 2020/4/17 10:05
*/
@RestController
public class SendEmailController {
@Autowired
private SendEmailService sendEmailService;
@ApiOperation(value = "实现企业邮箱发送邮件")
@GetMapping(value = "/sendEmail")
public void sendEmailToCompanyEmail(){
//发送邮件到腾讯企业邮箱
sendEmailService.sendEmail();
}
}
(4)编写业务逻辑类
package com.test.sendmail;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Service;
import javax.mail.*;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
import java.util.Date;
/**
* @Author tanghh
* @Date 2020/4/17 10:06
*/
@Service
public class SendEmailServiceImpl implements SendEmailService {
private Logger logger = LoggerFactory.getLogger(SendEmailServiceImpl.class);
@Override
public void sendEmail() {
try{
//收件人腾讯企业邮箱账号
String receiveAccount = "接收方邮箱账号号";
//发送方账号密码
String userName = "发送方邮箱账号";
String password = "发送方邮箱密码";
//邮箱主题
String theme = "企业邮箱主题";
//邮件内容
String content = "企业邮箱发送内容";
//获取Session对象
Session session = Session.getDefaultInstance(SendMailUtil.setTencentExEmail(),
new Authenticator() {
//此访求返回用户和密码的对象
@Override
protected PasswordAuthentication getPasswordAuthentication() {
PasswordAuthentication pa = new PasswordAuthentication(userName, password);
return pa;
}
});
// for (int i = 0; i <2 ; i++) {
// 有循环的情况下,实现单独发送的功能 收件人方只显示自己的邮箱
MimeMessage mimeMessage = new MimeMessage(session);
mimeMessage.setFrom(new InternetAddress(userName, userName));
mimeMessage.addRecipient(Message.RecipientType.TO, new InternetAddress(receiveAccount));
//设置主题
mimeMessage.setSubject(theme);
mimeMessage.setSentDate(new Date());
//设置内容
mimeMessage.setText(content);
mimeMessage.saveChanges();
try {
//具体发送邮件的方法
Transport.send(mimeMessage);
} catch (MessagingException e) {
logger.error(e.getMessage());
// continue;
}
// }
}catch (Exception e){
logger.error("发送邮箱失败",e);
}
}
}
(5)工具类
package com.test.sendmail;
import com.sun.mail.util.MailSSLSocketFactory;
import java.security.GeneralSecurityException;
import java.util.Properties;
/**
* @Author tanghh
* @Date 2020/4/17 10:08
*/
public class SendMailUtil {
/**
* 邮箱协议
*/
private static String MAIL_TRANSPORT_PROTOCOL = "smtp";
/**
* 发件服务器地址(以下是腾讯企业邮箱)
*/
private static String MAIL_SMTP_HOST = "smtp.exmail.qq.com";
/**
* 端口
*/
private static String MAIL_SMTP_PORT = "465";
/**
* 使用smtp身份验证
*/
private static String MAIL_SMTP_AUTH = "true";
/**
* 邮箱配置
*/
public static Properties setTencentExEmail (){
Properties prop = new Properties();
//协议
prop.setProperty("mail.transport.protocol", MAIL_TRANSPORT_PROTOCOL);
//服务器
prop.setProperty("mail.smtp.host", MAIL_SMTP_HOST);
//端口
prop.setProperty("mail.smtp.port", MAIL_SMTP_PORT);
//使用smtp身份验证
prop.setProperty("mail.smtp.auth", MAIL_SMTP_AUTH);
//开启安全协议 使用SSL,企业邮箱必需!
MailSSLSocketFactory sf = null;
try {
sf = new MailSSLSocketFactory();
sf.setTrustAllHosts(true);
} catch (GeneralSecurityException e1) {
e1.printStackTrace();
}
prop.put("mail.smtp.ssl.enable", "true");
prop.put("mail.smtp.ssl.socketFactory", sf);
return prop;
}
}
(6)测试
在浏览器上访问:http://localhost:8006/sendEmail
如果需要发送多个邮件的话,可以将我注释的for循环放开。
参考文章:
https://blog.csdn.net/qq_41151659/article/details/96475739
如果觉得小编写的不错的话,可以给小编一个赞喔,