smart_ptr库
boost.smart_ptr库提供了六种智能指针,包括scope_ptr、scoped_array、shared_ptr、shared_array、weak_ptr和intrusive_ptr。它们是轻量级的对象,速度和原始指针相差无几,都是异常安全的。
需要包含头文件
#include<boost/smart_ptr.hpp>
using namespace boost;
scoped_ptr
类似于auto_prt/unique_ptr的智能指针,它的所有权更加严格,不能转让,一旦scoped_ptr获取了对象的管理权,我们就无法再从它那里取回来。
①拷贝构造函数和赋值操作符都声明为私有,保证被它管理的指针不能被转让所有权。
②reset()重置scoped_ptr,删除原来保存的指针,再保存新的指针值p。
③用operator *()和operator ->()重载解引用操作符和箭头操作符,以模仿被代理的原始指针的行为。
④提供了一个bool语境中自动转换成bool值的功能,用来测试scoped_ptr是否持有一个有效的指针。
⑤swap()交换两个scoped_ptr保存的原始指针。
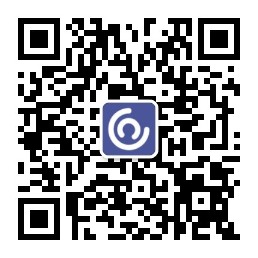
⑥get()返回scoped_ptr内部保存的原始指针。
用法:
#include <iostream>
#include <string>
#include <boost/smart_ptr.hpp>
using namespace std;
using namespace boost;
int main()
{
//构造对象
scoped_ptr<string> sp(new string("text"));
//使用显式bool转型
assert(sp);
//空指针比较
assert(sp != nullptr);
//输出
cout << *sp << endl;
cout << sp->size() << endl;
//错误,不能拷贝或赋值
//scoped_ptr<string> sp2 = sp;
//错误不能使用++或者--等操作符,只能使用 *和->
//sp++;
getchar();
return 0;
}
由于scoped_ptr类不能拷贝和赋值,所以一个类持有scoped_ptr成员变量,那么这个类也是不可拷贝和赋值的。
对比unique_ptr
unique_ptr是C++11标准中的智能指针,它不仅能够代理new 创建的单个对象,也能够代理new[ ]创建的数组对象,也就是说它结合了scoped_ptr和scoped_array两者的能力。
scoped_array
它很像 scoped_ptr,它包装了new[ ]操作符在堆上分配的动态数组。
scoped_array的接口和功能几乎与scoped_ptr是相同的。
①构造函数接受的指针p必须是new[ ]的结果,而不能是new表达式的结果。
②没有*、->操作符重载,因为scoped_array持有的不是一个普通指针。
③提供operator[ ]操作符重载,可以像普通数组一组下标访问元素。
④没有begin()、end()等类似容器的迭代器操作函数。
用法:
#include <iostream>
#include <string>
#include <boost/smart_ptr.hpp>
using namespace std;
using namespace boost;
int main()
{
//构造对象
scoped_array<int> sa(new int[100]);
//使用标准库算法赋值数据
fill_n(&sa[0], 100, 5);
//用起来就像普通数组
sa[10] = sa[20] + sa[30];
//错误用法,不能通过编译
//*(sa + 1) = 20;
getchar();
return 0;
}
对比unique_ptr
unique_ptr用法与scoped_array基本相同,但模板参数中需要声明为数组类型。
unique_ptr<int []> up(new int[10])
使用建议
scoped_array的功能有限,不能动态增长,没有边界检查,也没有迭代器支持,不能搭配STL算法。
在需要动态数组的情况下我们应该使用std::vector,不推荐使用scoped_array。