import re
x1='I'
x2='she'
x='I will meet you.'
print(re.search(x1,x)) #在x中寻找x1
print(re.search(x2,x))

print(re.search(r"m[A-Z]t", "I met you"))
print(re.search(r"m[a-z]t", "I meet you"))
print(re.search(r"m[0-9]t", "I m0t you"))
print(re.search(r"m[0-9a-z]t", "I met you"))

- \d : 任何数字
- \D : 不是数字
- \s : 任何 white space, 如 [\t\n\r\f\v]
- \S : 不是 white space
- \w : 任何大小写字母, 数字和 “” [a-zA-Z0-9]
- \W : 不是 \w
- \b : 空白字符 (只在某个字的开头或结尾)
- \B : 空白字符 (不在某个字的开头或结尾)
- \\ : 匹配 \
- . : 匹配任何字符 (除了 \n)
- ^ : 匹配开头
- $ : 匹配结尾
- ? : 前面的字符可有可无
# \d : decimal digit
print(re.search(r"r\dn", "run r4n")) # <_sre.SRE_Match object; span=(4, 7), match='r4n'>
# \D : any non-decimal digit
print(re.search(r"r\Dn", "run r4n")) # <_sre.SRE_Match object; span=(0, 3), match='run'>
# \s : any white space [\t\n\r\f\v]
print(re.search(r"r\sn", "r\nn r4n")) # <_sre.SRE_Match object; span=(0, 3), match='r\nn'>
# \S : opposite to \s, any non-white space
print(re.search(r"r\Sn", "r\nn r4n")) # <_sre.SRE_Match object; span=(4, 7), match='r4n'>
# \w : [a-zA-Z0-9_]
print(re.search(r"r\wn", "r\nn r4n")) # <_sre.SRE_Match object; span=(4, 7), match='r4n'>
# \W : opposite to \w
print(re.search(r"r\Wn", "r\nn r4n")) # <_sre.SRE_Match object; span=(0, 3), match='r\nn'>
# \b : empty string (only at the start or end of the word)
print(re.search(r"\bruns\b", "dogruns runs to cat runs")) # <_sre.SRE_Match object; span=(8, 12), match='runs'>
# \B : empty string (but not at the start or end of a word)
print(re.search(r"\Bruns\B", "dogrunso runs to cat runs")) #<_sre.SRE_Match object; span=(3, 7), match='runs'>
print(re.search(r"\B runs \B", "dog runs to cat")) # <_sre.SRE_Match object; span=(8, 14), match=' runs '>
# \\ : match \
print(re.search(r"runs\\", "runs\ to me")) # <_sre.SRE_Match object; span=(0, 5), match='runs\\'>
# . : match anything (except \n)
print(re.search(r"r.n", "r[ns to me")) # <_sre.SRE_Match object; span=(0, 3), match='r[n'>
# ^ : match line beginning
print(re.search(r"^dog", "dog runs to cat")) # <_sre.SRE_Match object; span=(0, 3), match='dog'>
print(re.search(r"^dog", "ccdog runs to cat")) # None
# $ : match line ending
print(re.search(r"cat$", "dog runs to cat")) # <_sre.SRE_Match object; span=(12, 15), match='cat'>
print(re.search(r"cat$", "dog runs to cat.")) # None
# ? : may or may not occur
print(re.search(r"Mon(day)?", "Monday")) # <_sre.SRE_Match object; span=(0, 6), match='Monday'>
print(re.search(r"Mon(day)?", "Mon")) # <_sre.SRE_Match object; span=(0, 3), match='Mon'>
- 匹配非第一行的字符串:flags=re.MULTILINE或者flags=re.M
*
: 重复零次或多次
+
: 重复一次或多次
{n, m}
: 重复 n 至 m 次
{n}
: 重复 n 次
string="""
People ran to square.
SShhhhout and cheer.
She was also very exited.
"""
print(re.search(r"^Sh*",string,flags=re.MULTILINE)) #<_sre.SRE_Match object; span=(23, 24), match='S'>
print(re.search(r"^Sh+",string,flags=re.MULTILINE)) #<_sre.SRE_Match object; span=(44, 46), match='Sh'>
- match.group() :如下例,匹配有2个group,分别对应re.search中打括号的2个地方,即"(\d+)"和"(.+)"
match = re.search(r"(\d+), Date: (.+)", "ID: 021523, Date: Feb/12/2017")
print(match.group())
print(match.group(1))
print(match.group(2))

- match.group() :
?P<名字>
给这个组定义了一个名字. 然后就能用这个名字找到这个组的内容.
match = re.search(r"(?P<id>\d+), Date: (?P<date>.+)", "ID: 021523, Date: Feb/12/2017")
print(match.group('id'))
print(match.group('date'))

- re.findall():上述都只匹配第一个出现的项,findall可以匹配所有出现的项:
# findall
print(re.findall(r"me+t", "met meet meat"))
# | : or
print(re.findall(r"(met|meet)", "met meet meat"))

- re.sub():功能是先匹配,再替换,比string.replace()更灵活:
print(re.sub(r"^[Nn]ongfu", "Wahaha", "Nongfu Spring"))

- re.split():分割,注意分割的结果不包括分割项:
print(re.split(r"[,;\.]", "a;b,c.d;e"))
print(re.split(r"[sS][h]", "Shout,cheer,she,sheet"))

compiled=re.compile(r"m[e]+t")
print(compiled.search("I will meet you."))
print(compiled.search("I met you yesterday."))

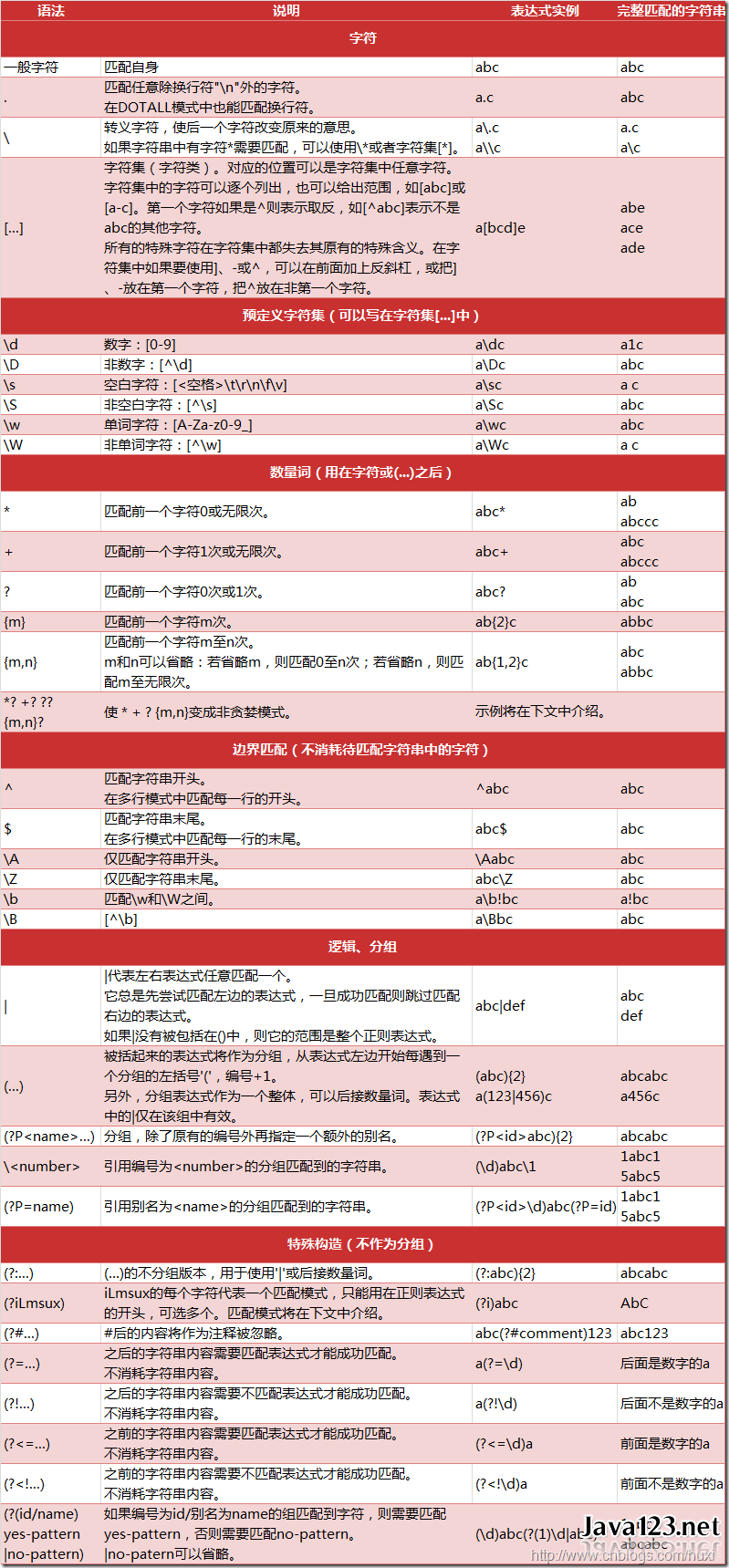