学习笔记,仅供参考
数据库的迁移
我在学习一对多映射时,由于操作不慎,导致报错频频,现在,我就来解决这个问题,顺便学习一下迁移操作。
现在,我在第7次迁移时出错了,它的错误是这样的:
pymysql.err.InternalError: (1054, "Unknown column 'pub' in 'china_publisher'")
报错信息显示,pub字段不在china_publisher表中。
我修改之后,还有一个报错,它是这样的:
django.db.utils.InternalError: (1366, "Incorrect integer value: '清华大学出版社' for column 'pub_id' at row 1")
错误太多,我也不知道该怎么办,所以我选择回到前几次迁移文件,重新开始。
看一下项目目录,方便后续理解:
目前,我在第7次迁移的位置,我想回到第1次迁移,那该咋整呢?
我们可以在cmd中敲入如下代码:
python manage.py migrate bookstore 0001
输出:
扫描二维码关注公众号,回复:
11341733 查看本文章
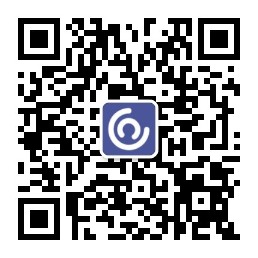
Operations to perform:
Target specific migration: 0001_initial, from bookstore
Running migrations:
Rendering model states... DONE
Unapplying bookstore.0002_author... OK
这里,我从0007退到0005,发现Django还是可能会报错,就再退到0002,发现Django依然可能会报错,最后退到0001,也就是说我敲入了3次退回代码。上面显示的输出,是我从0002退回到0001的输出。
我们查看一下数据库:
mysql> show tables;
+----------------------------+
| Tables_in_mywebdb |
+----------------------------+
| auth_group |
| auth_group_permissions |
| auth_permission |
| auth_user |
| auth_user_groups |
| auth_user_user_permissions |
| bookstore_book |
| china_publisher |
| django_admin_log |
| django_content_type |
| django_migrations |
| django_session |
+----------------------------+
12 rows in set (0.00 sec)
此时的数据库应该没有china_publisher数据表,不知道哪里出错了,这种情况和Django在0001迁移文件里记录的情况完全不同,所以我果断将其删除!
mysql> drop table china_publisher;
Query OK, 0 rows affected (0.03 sec)
一般情况下,还是不要随便删表,要不然很容易出现混乱。
现在,我们把0001以上的记录文件全部删除:
并再次进行迁移操作
F:\MyStudio\PythonStudio\goatbishop.project01\Django\mywebsite_db>python manage.py ma
kemigrations
Migrations for 'bookstore':
bookstore\migrations\0002_auto_20200622_0213.py
- Create model Author
- Create model Publisher
- Remove field pub from book
- Add field exfacPrice to book
- Add field price to book
- Create model Partner
F:\MyStudio\PythonStudio\goatbishop.project01\Django\mywebsite_db>python manage.py mi
grate
Operations to perform:
Apply all migrations: admin, auth, bookstore, contenttypes, sessions
Running migrations:
Applying bookstore.0002_auto_20200622_0213... OK
迁移成功!
查看一下数据表:
mysql> show tables;
+----------------------------+
| Tables_in_mywebdb |
+----------------------------+
| auth_group |
| auth_group_permissions |
| auth_permission |
| auth_user |
| auth_user_groups |
| auth_user_user_permissions |
| bookstore_author |
| bookstore_book |
| bookstore_partner |
| china_publisher |
| django_admin_log |
| django_content_type |
| django_migrations |
| django_session |
+----------------------------+
14 rows in set (0.00 sec)
mysql> select * from china_publisher;
Empty set (0.00 sec)
mysql> select * from bookstore_book;
+----+-------------------+------------+-------+
| id | title | exfacPrice | price |
+----+-------------------+------------+-------+
| 1 | Djangoweb开发实战 | 0.00 | 0.00 |
| 2 | python | 0.00 | 0.00 |
| 3 | R | 0.00 | 0.00 |
| 5 | 算法 | 0.00 | 0.00 |
| 6 | 集体智慧编程 | 0.00 | 0.00 |
+----+-------------------+------------+-------+
5 rows in set (0.00 sec)
这时,我们在Book模型类中再加入一个字段pub,并与Publisher模型类进行一对多关联:
from django.db import models
# Create your models here.
class Publisher(models.Model):
name = models.CharField("出版社名", max_length = 50,null = True)
booknumber = models.PositiveIntegerField("初版书籍总量", default = 0)
tele = models.CharField("联系电话", max_length = 11, null = False)
class Meta:
db_table = "china_publisher"
verbose_name = "ChinaPublisher"
verbose_name_plural = "ChinaPublishers"
def __str__(self):
string = "出版社:%s" % (self.name)
return string
class Book(models.Model):
title = models.CharField("书名", max_length = 30)
exfacPrice = models.DecimalField("出厂价",
max_digits = 6, decimal_places = 2,
default = 0)
price = models.DecimalField("售价",
max_digits = 6, decimal_places = 2,
default = 0)
pub = models.ForeignKey(Publisher, on_delete = models.CASCADE , null=True)
def __str__(self):
string = "书名:%s" % (self.title)
return string
class Author(models.Model):
name = models.CharField("姓名", max_length = 30, null = False,
unique = True, db_index = True)
age = models.IntegerField("年龄", null = False,
default = 1)
email = models.EmailField("邮箱", null = True)
def __str__(self):
string = "姓名:{}, 年龄:{}".format(self.name, self.age)
return string
class Partner(models.Model):
'''作家伴侣模型类'''
name = models.CharField("姓名", max_length=50)
age = models.IntegerField("年龄", null = False,
default = 1)
author = models.OneToOneField(Author, on_delete = models.CASCADE)
再次执行迁移操作:
F:\MyStudio\PythonStudio\goatbishop.project01\Django\mywebsite_db>python manage.py ma
kemigrations
Migrations for 'bookstore':
bookstore\migrations\0003_book_pub.py
- Add field pub to book
F:\MyStudio\PythonStudio\goatbishop.project01\Django\mywebsite_db>python manage.py mi
grate
Operations to perform:
Apply all migrations: admin, auth, bookstore, contenttypes, sessions
Running migrations:
Applying bookstore.0003_book_pub... OK
迁移成功!
再次查看bookstore_book数据表:
mysql> select * from bookstore_book;
+----+-------------------+------------+-------+--------+
| id | title | exfacPrice | price | pub_id |
+----+-------------------+------------+-------+--------+
| 1 | Djangoweb开发实战 | 0.00 | 0.00 | NULL |
| 2 | python | 0.00 | 0.00 | NULL |
| 3 | R | 0.00 | 0.00 | NULL |
| 5 | 算法 | 0.00 | 0.00 | NULL |
| 6 | 集体智慧编程 | 0.00 | 0.00 | NULL |
+----+-------------------+------------+-------+--------+
5 rows in set (0.00 sec)