编写Android程序从网络中获取图片资源,练习AsyncHttpClient、SmartImageView等开源框架的使用。
1.编写APP程序,导入AsyncHttpClient、HttpClient、SmartImageView、Gson四个开源框架的Jar文件包。
2.搭建Tomcat网络服务器,将imgInfo.json文件部署到服务器中并测试运行效果。
3.利用AsyncHttpClient访问网络服务器,获取json文件并解析其内容。
4.利用SmartImageView通过json文件中解析出的网络地址下载图片,并在界面列表中显示图片与相应文字,界面如图所示
这次写的有些坎坷,最后还没写完,最后有一个小问题没解决,因为时间不够了,就先放上来,慢慢修改吧
先给上xml文件
homework_12.xml
扫描二维码关注公众号,回复:
11327610 查看本文章
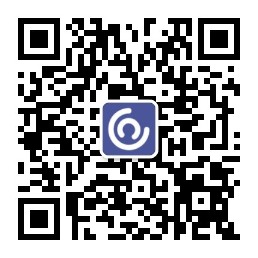
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <ListView android:id="@+id/lv_news" android:layout_width="wrap_content" android:layout_height="wrap_content" /> </LinearLayout>
homework_12_2.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="65dp"> <LinearLayout android:orientation="horizontal" android:layout_width="match_parent" android:layout_height="wrap_content"> <com.loopj.android.image.SmartImageView android:id="@+id/siv_icon" android:layout_weight="4" android:layout_width="0dp" android:layout_height="180dp"/> <TextView android:id="@+id/tv_description" android:layout_gravity="center" android:textSize="24sp" android:layout_weight="6" android:layout_width="0dp" android:layout_height="wrap_content"/> </LinearLayout> </LinearLayout>
然后写java文件
mainactivity.java
package com.example.app; import androidx.appcompat.app.AppCompatActivity; import android.annotation.SuppressLint; import android.content.ContentValues; import android.content.Intent; import android.database.Cursor; import android.database.sqlite.SQLiteDatabase; import android.graphics.Bitmap; import android.graphics.BitmapFactory; import android.graphics.Color; import android.net.Uri; import android.os.AsyncTask; import android.os.Bundle; import android.util.Log; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.*; import com.google.gson.*; import com.loopj.android.http.AsyncHttpClient; import com.loopj.android.http.AsyncHttpResponseHandler; import com.loopj.android.image.SmartImage; import com.loopj.android.image.SmartImageView; import org.apache.http.Header; import java.io.IOException; import java.io.UnsupportedEncodingException; import java.net.URLEncoder; import java.util.ArrayList; import java.util.List; public class MainActivity extends AppCompatActivity { private ListView lvNews; private List<NewsInfo> newsInfos; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.homework_12); fillData(); lvNews = (ListView) this.findViewById(R.id.lv_news); } private void fillData(){ AsyncHttpClient client = new AsyncHttpClient(); //get请求 client.get(getString(R.string.serverurl), new AsyncHttpResponseHandler() { @Override public void onSuccess(int i, Header[] headers, byte[] bytes) { //请求成功 try { newsInfos = JsonParse.getNewsInfo(bytes); if (newsInfos == null){ Toast.makeText(MainActivity.this,"解析失败",Toast.LENGTH_SHORT).show(); }else { MyBaseAdapter myAdapter = new MyBaseAdapter(); lvNews.setAdapter(myAdapter); Toast.makeText(MainActivity.this,"success",Toast.LENGTH_SHORT).show(); } } catch (IOException e) { e.printStackTrace(); Toast.makeText(MainActivity.this,"连接失败",Toast.LENGTH_SHORT).show(); } } @Override public void onFailure(int i, Header[] headers, byte[] bytes, Throwable throwable) { } }); } class MyBaseAdapter extends BaseAdapter{ @Override public int getCount() { return newsInfos.size(); } @Override public Object getItem(int position) { return newsInfos.get(position); } @Override public long getItemId(int position) { return position; } @Override public View getView(int position, View convertView, ViewGroup parent) { ViewHolder holder; if(convertView == null){ convertView = LayoutInflater.from(getApplicationContext()).inflate(R.layout.homework_12_2,parent,false); holder = new ViewHolder(); holder.mTextView = (TextView) convertView.findViewById(R.id.tv_description); holder.imageView = (SmartImageView) convertView.findViewById(R.id.siv_icon); convertView.setTag(holder); }else { holder = (ViewHolder) convertView.getTag(); } holder.mTextView.setText(newsInfos.get(position).getMessage()); holder.imageView.setImageUrl(newsInfos.get(position).getImg()); return convertView; } class ViewHolder { TextView mTextView; SmartImageView imageView; } } }
然后是JsonParse.java
package com.example.app; import com.google.gson.*; import com.google.gson.reflect.TypeToken; import java.io.IOException; import java.lang.reflect.Type; import java.util.ArrayList; import java.util.List; public class JsonParse { public static List<NewsInfo> getNewsInfo(byte[] bytes) throws IOException{ String JsonParse = new String(bytes,"GBK"); Gson gson = new Gson(); List<NewsInfo> list = new ArrayList<>(); Type listType = new TypeToken<List<NewsInfo>>() { }.getType(); System.out.println(JsonParse); list = gson.fromJson(JsonParse,listType); return list; } }
还有NewsInfo.java
package com.example.app; import android.net.Uri; class NewsInfo { public String message; public String img; public void setMessage(String message){ this.message=message; } public void setImg(String img){ this.img = img; } public String getMessage(){ return message; } public String getImg(){ return img; } }
经过重重调试,到这步卡死了,实在是解决不了了。你们可以试试,也许就行了
第一步安装tomcat就不说了,我是安装在我的虚拟机上,上个本机访问的图
还有一些点,我的url是写在了配置文件里,如果不想写在这里,可以自己手动写到activity里
我大致照模板写一下
private String Port = "8080"; // 端口 private String Host = "192.168.0.1"; // 主机(本地IP) private String Path = "/android/imginfo.json"; // 文件路径 private String serverUrl = "http://" + Host + ":" + Port + Path; // 网址
然后就是AndroidManifest.xml
这里我直接放图片了
应该是这几个,有点忘了
就先写到这儿,继续解决这个问题去了。