A.Candies
题意:就是问是否存在一个x,k使得x+2x+4x+⋯+2^(k−1)x=n,并输出x的值
思路:先用等差数列的前n项和公式进行整合公式,然后算出最后的k,n的关系,(2^n-1)x=n,进行打表计算2^n,再循环判断从2^0到2^n是否有满足条件的
题解:

1 #include<iostream> 2 #include<algorithm> 3 #include<cmath> 4 #include<cstring> 5 #include<cstdio> 6 using namespace std; 7 int main(){ 8 long long int a[32]={0}; 9 for(int i=0;i<=30;i++){ 10 a[i]=pow(2,i); 11 } 12 long long int t; 13 scanf("%lld",&t); 14 while(t--){ 15 long long int num; 16 scanf("%lld",&num); 17 for(int i=2;i<=30;i++){ 18 if(num%(a[i]-1)==0){ 19 printf("%lld\n",num/(a[i]-1)); 20 break; 21 } 22 } 23 } 24 }
B - Balanced Array
题意:就是有两组数,元素个数相同,和也相同,并且每组里面的元素奇偶性相同,给出两组数的元素个数总和,找出可能的这两组数
思路:挨着输出2,4,6……,使其成为等差数列,然后第二组输出1,3,5……,然后第一组和第二组总和差n,再把这个所差加到小的一组里面,让第二组的最后一个元素+n
题解:

1 #include<iostream> 2 #include<algorithm> 3 #include<cmath> 4 #include<cstring> 5 #include<cstdio> 6 using namespace std; 7 int main(){ 8 int t; 9 scanf("%d",&t); 10 while(t--){ 11 int n; 12 scanf("%d",&n); 13 if((n/2)%2==1){ 14 printf("NO\n"); 15 }else{ 16 printf("YES\n"); 17 for(int i=1;i<=n/2;i++){ 18 printf("%d ",i+i); 19 } 20 int s=1; 21 for(int i=1;i<n/2;i++){ 22 printf("%d ",s); 23 s+=2; 24 } 25 printf("%d\n",s+n/2); 26 } 27 } 28 }
C - Ichihime and Triangle
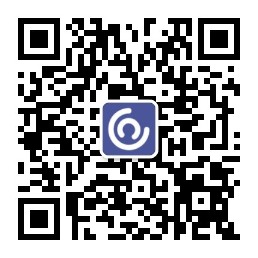
题意:给出a,b,c,d四个数,并且a<=x<=b,b<=y<=c,c<=z<=d,并且x,y,z可以构成三角形,保证xyz的存在,输出xyz
思路:答案存在,所以三角形三个边尽量应该相等就可以,那么x尽量大,z尽量小,那么直接输出b,c,c就可
题解:

1 #include<iostream> 2 #include<algorithm> 3 #include<cmath> 4 #include<cstring> 5 #include<cstdio> 6 using namespace std; 7 int main(){ 8 int t; 9 scanf("%d",&t); 10 while(t--){ 11 int a,b,c,d; 12 scanf("%d %d %d %d",&a,&b,&c,&d); 13 14 printf("%d %d %d\n",b,c,c); 15 16 17 } 18 }
D - Kana and Dragon Quest game
题意:三个数x,n,y,把x变为0,有两种变化形式:1.把h变为[h/2]+10;2.把h变为h-10([h]是四舍五入为整数)
思路:当第一种变成最后的数比第二种小时,即[h/2]+10<h-10,那么h>40,所以先进行第一种方法,让其变化,最后比较h和m*10
题解:

1 #include<iostream> 2 #include<algorithm> 3 #include<cmath> 4 #include<cstring> 5 #include<cstdio> 6 using namespace std; 7 int main(){ 8 int t; 9 scanf("%d",&t); 10 while(t--){ 11 int x,n,m; 12 scanf("%d %d %d",&x,&n,&m); 13 for(int i=1;i<=n&&x>0&&x>x/2+10;i++){ 14 x=x/2+10; 15 } 16 if(x>m*10){ 17 printf("NO\n"); 18 }else{ 19 printf("YES\n"); 20 } 21 } 22 }
E - Candies and Two Sisters
题意:糖果数是n,把它分成两份分别是a,b,保证a>b,问n的分法有多少
思路:直接按奇偶进行分,如果n是奇数则直接输出n/2,如果是偶数就输出n/2-1
题解:

1 #include<iostream> 2 #include<algorithm> 3 #include<cmath> 4 #include<cstring> 5 #include<cstdio> 6 using namespace std; 7 int main(){ 8 int t; 9 scanf("%d",&t); 10 while(t--){ 11 int num; 12 scanf("%d",&num); 13 if(num%2==0){ 14 printf("%d\n",num/2-1); 15 }else{ 16 printf("%d\n",num/2); 17 } 18 } 19 }
F - Construct the String
题意:有一个字符串,长度为n,并且每个字符由小写字母组成,并且a个字符串中由b个不同字符组成
思路:先按规律输出b个字符循环。这样保证了n/a*a个字符满足题目要求,再进行n%a的部分输出刚才循环节
题解:

1 #include<cstdio> 2 #include<cstring> 3 #include<algorithm> 4 #include<iostream> 5 #include<cmath> 6 using namespace std; 7 int main(){ 8 int T; 9 scanf("%d", &T); 10 while (T--){ 11 int a,b,n; 12 scanf("%d %d %d",&n,&a,&b); 13 while (n!=0){ 14 for(int i=0;i<b;i++){ 15 if(n==0){ 16 break; 17 } 18 printf("%c",97+i); 19 n--; 20 } 21 } 22 printf("\n"); 23 } 24 25 26 }
最近其实还是挺忙的,不过忙起来好像并没有想象中的那么累,挺满足的