参考自
os模块和shutil模块
import os
import shutil
目录操作
1.取得当前目录
s = os.getcwd()
eg:将abc.py放入A文件夹,并且希望不管将A文件夹放在硬盘的哪个位置,都可以在A文件夹内生成一个新文件夹。且文件夹的名字根据时间自动生成。
import os
import time
folder = time.strftime(r"%Y-%m-%d_%H-%M-%S",time.localtime())
os.makedirs(r'%s/%s'%(os.getcwd(),folder))
2.更改当前目录
os.chdir( "C:\123")
tip: 当指定的目录不存在时,引发异常WindowsError。
3.创建目录
os.mkdir("file") 创建单个目录
os.makedirs( r“c:\python\test” ) 创建多级目录
4.删除目录
os.rmdir( path ) 只能删除空目录
shutil.rmtree("dir") #空目录、有内容的目录都可以删
5.目录改名
os.rename("oldname","newname") 文件或目录都是使用这条命令
6.移动文件(目录)
shutil.move("oldpos","newpos")
7.获取某目录中的文件及子目录的列表
L = os.listdir( "你要判断的路径")
L = os.listdir( "c:/" )
print L
显示 :
['1.avi', '1.jpg', '1.txt', 'CONFIG.SYS', 'Inetpub', 'IO.SYS', 'ntldr', 'pagefile.sys', 'PDOXUSRS.NET', 'Program Files', 'QQVideo.Cache', 'RECYCLER', 'test.txt', 'WINDOWS']
- 获取某指定目录下的所有子目录的列表
def getDirList( p ):
p = str( p )
if p=="":
return [ ]
p = p.replace( "/","\\") //将文件目录表示方式统一转化使用\\
if p[ -1] != "\\": //如果实参中没有添加路径最后的\\,统一添加
p = p+"\\"
a = os.listdir( p )
b = [ x for x in a if os.path.isdir( p + x ) ]
return b
print (getDirList( "C:\\" ))
结果:
['Documents and Settings', 'Downloads', 'HTdzh', 'KCBJGDJC' , 'QQVideo.Cache', 'RECYCLER', 'System Volume Information', 'TDDOWNLOAD', 'WINDOWS']
- 获取某指定目录下的所有文件的列表
def getFileList( p ):
p = str( p )
if p=="":
return [ ]
p = p.replace( "/","\\")
if p[ -1] != "\\":
p = p+"\\"
a = os.listdir( p )
b = [ x for x in a if os.path.isfile( p + x ) ]
return b
print getFileList( "C:\\" )
结果:
['1.avi', '1.jpg', '1.txt', '123.txt', '12345.txt', '2.avi', 'a.py', 'AUTOEXEC.BAT', 'boot.ini', 'bootfont.bin', 'MSDOS.SYS', 'NTDETECT.COM', 'pagefile.sys', 'PDOXUSRS.NET', 'test.txt']
文件操作
1.创建空文件
os.mknod("test.txt")
2.删除文件
os.remove( filename ) # filename: "要删除的文件名"
4.文件改名
os.name( oldfileName, newFilename)
os.rename("oldname","newname") 文件或目录都是使用这条命令
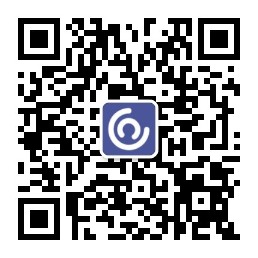
5.移动文件
shutil.move("oldpos","newpos")
6.复制文件
shutil.copyfile("oldfile","newfile") oldfile和newfile都只能是文件
shutil.copy("oldfile","newfile") oldfile只能是文件夹,newfile可以是文件,也可以是目标目录
对路径的操作(文件/文件夹)
1.判断路径
- 判断路径( 目录或文件)是否存在
b = os.path.exists( "你要判断的路径")
返回值b: True 或 False
- 判断一个路径是否文件
b = os.path.isfile( "你要判断的路径")
返回值b: True 或 False
- 判断一个路径是否目录
b = os.path.isdir( "你要判断的路径")
返回值b: True 或 False
2.分解路径名
- 分解路径名中文件名的扩展名
fpathandname , fext = os.path.splitext( "你要分解的路径")
a, b = os.path.splitext( "c:\\123\\456\\test.txt" )
print a
print b
显示:
c:\123\456\test
.txt
- 返回一个路径的目录名和文件名:os.path.split()
os.path.split('/home/swaroop/byte/code/poem.txt')
结果:('/home/swaroop/byte/code', 'poem.txt')
-
获取路径名:os.path.dirname()
-
获取文件名:os.path.basename()
3.路径拼接
os.path.join()函数 连接两个或更多的路径名组件
- 如果各组件名首字母不包含’/’,则函数会自动加上
- 如果有一个组件是一个绝对路径,则在它之前的所有组件均会被舍弃
- 如果最后一个组件为空,则生成的路径以一个’/’分隔符结尾
import os
Path1 = '/home'
Path2 = 'develop'
Path3 = 'code'
Path10 = Path1 + Path2 + Path3
Path20 = os.path.join(Path1,Path2,Path3)
print ('Path10 = ',Path10)
print ('Path20 = ',Path20)
#输出
#Path10 = /homedevelopcode
#Path20 = /home\develop\code
例子:将文件夹下所有图片名称加上'_fc'
# -*- coding:utf-8 -*-
import re
import os
import time
#str.split(string)分割字符串
#'连接符'.join(list) 将列表组成字符串
def change_name(path):
global i
if not os.path.isdir(path) and not os.path.isfile(path):
return False
if os.path.isfile(path):
file_path = os.path.split(path) #分割出目录与文件
lists = file_path[1].split('.') #分割出文件与文件扩展名
file_ext = lists[-1] #取出后缀名(列表切片操作)
img_ext = ['bmp','jpeg','gif','psd','png','jpg']
if file_ext in img_ext:
os.rename(path,file_path[0]+'/'+lists[0]+'_fc.'+file_ext)
i+=1 #注意这里的i是一个陷阱
#或者
#img_ext = 'bmp|jpeg|gif|psd|png|jpg'
#if file_ext in img_ext:
# print('ok---'+file_ext)
elif os.path.isdir(path):
for x in os.listdir(path):
change_name(os.path.join(path,x)) #os.path.join()在路径处理上很有用
img_dir = 'D:\\xx\\xx\\images'
img_dir = img_dir.replace('\\','/')
start = time.time()
i = 0
change_name(img_dir)
c = time.time() - start
print('程序运行耗时:%0.2f'%(c))
print('总共处理了 %s 张图片'%(i))