一、XmlDocument类
参考资料:
1.visual studio documentation:XmlDocument Class
//继承关系
public class XmlDocument : System.Xml.XmlNode
XmlDocument类是XML文档的内存表示。它实现了W3CXML文档对象模型(DOM)的第1级核心和第2级核心
DOM代表文档对象模型document object model
可以使用XmlDocument类将XML加载到DOM中,然后以编程方式读取、修改和删除文档中的XML。
示例部分:
1.将XML加载到文档对象模型中
<?xml version="1.0" encoding="utf-8"?>
<books xmlns="http://www.contoso.com/books">
<book genre="novel" ISBN="1-861001-57-8" publicationdate="1823-01-28">
<title>Pride And Prejudice</title>
<price>24.95</price>
</book>
<book genre="novel" ISBN="1-861002-30-1" publicationdate="1985-01-01">
<title>The Handmaid's Tale</title>
<price>29.95</price>
</book>
<book genre="novel" ISBN="1-861001-45-3" publicationdate="1811-01-01">
<title>Sense and Sensibility</title>
<price>19.95</price>
</book>
</books>
*******************从文件中加载xml,如果文件不存在,则先创建再加载***************
XmlDocument doc = new XmlDocument();
doc.PreserveWhitespace = true;
try { doc.Load("booksData.xml"); }
catch (System.IO.FileNotFoundException)
{
doc.LoadXml("<?xml version=\"1.0\"?> \n" +
"<books xmlns=\"http://www.contoso.com/books\"> \n" +
" <book genre=\"novel\" ISBN=\"1-861001-57-8\" publicationdate=\"1823-01-28\"> \n" +
" <title>Pride And Prejudice</title> \n" +
" <price>24.95</price> \n" +
" </book> \n" +
" <book genre=\"novel\" ISBN=\"1-861002-30-1\" publicationdate=\"1985-01-01\"> \n" +
" <title>The Handmaid's Tale</title> \n" +
" <price>29.95</price> \n" +
" </book> \n" +
"</books>");
}
2.导航文档树Navigate the document tree
xml文档树结构基本介绍:
文档由节点(Node)组成。
每个节点的正上方都有一个父节点(Parent Node)。
唯一没有父节点的节点是文档根节点(root node),因为它是顶级节点。
大多数节点都可以有子节点(child node),子节点是它们下面的节点。
处于同一级别的节点是同级节点(siblings)。
*******************获取根节点,并输出整个文档内容****************
using System;
using System.IO;
using System.Xml;
public class Sample
{
public static void Main()
{
//Create the XmlDocument.
XmlDocument doc = new XmlDocument();
doc.LoadXml("<?xml version='1.0' ?>" +
"<book genre='novel' ISBN='1-861001-57-5'>" +
"<title>Pride And Prejudice</title>" +
"</book>");
//Display the document element.
Console.WriteLine(doc.DocumentElement.OuterXml);
}
}
*******************获取根节点的第一个子节点,并遍历该节点的子节点(如果有)**************
using System;
using System.IO;
using System.Xml;
public class Sample
{
public static void Main() {
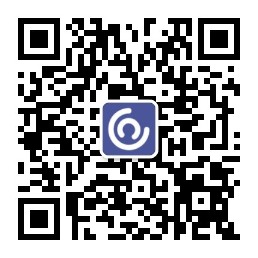
XmlDocument doc = new XmlDocument();
doc.LoadXml("<book ISBN='1-861001-57-5'>" +
"<title>Pride And Prejudice</title>" +
"<price>19.95</price>" +
"</book>");
XmlNode root = doc.FirstChild;
//Display the contents of the child nodes.
if (root.HasChildNodes)
{
for (int i=0; i<root.ChildNodes.Count; i++)
{
Console.WriteLine(root.ChildNodes[i].InnerText);
}
}
}
}
*******************获取节点的父节点*****************
使用ParentNode属性
*******************获取节点最后一个子节点*****************
using System;
using System.IO;
using System.Xml;
public class Sample
{
public static void Main() {
XmlDocument doc = new XmlDocument();
doc.LoadXml("<book ISBN='1-861001-57-5'>" +
"<title>Pride And Prejudice</title>" +
"<price>19.95</price>" +
"</book>");
XmlNode root = doc.FirstChild;
Console.WriteLine("Display the price element...");
Console.WriteLine(root.LastChild.OuterXml);
}
}
******************在兄弟节点中向前导航 Navigate forward across siblings******************
using System;
using System.Xml;
public class Sample {
public static void Main() {
XmlDocument doc = new XmlDocument();
doc.Load("books.xml");
XmlNode currNode = doc.DocumentElement.FirstChild;
Console.WriteLine("First book...");
Console.WriteLine(currNode.OuterXml);
XmlNode nextNode = currNode.NextSibling;
Console.WriteLine("\r\nSecond book...");
Console.WriteLine(nextNode.OuterXml);
}
}
******************在兄弟节点中向后导航 Navigate backwards across siblings******************
using System;
using System.Xml;
public class Sample {
public static void Main() {
XmlDocument doc = new XmlDocument();
doc.Load("books.xml");
XmlNode lastNode = doc.DocumentElement.LastChild;
Console.WriteLine("Last book...");
Console.WriteLine(lastNode.OuterXml);
XmlNode prevNode = lastNode.PreviousSibling;
Console.WriteLine("\r\nPrevious book...");
Console.WriteLine(prevNode.OuterXml);
}
}
找到节点:查找一个或多个数据节点的最常用方法是使用XPath查询字符串
******************获取单个节点*****************
public XmlNode GetBook(string uniqueAttribute, XmlDocument doc)
{
XmlNamespaceManager nsmgr = new XmlNamespaceManager(doc.NameTable);
nsmgr.AddNamespace("bk", "http://www.contoso.com/books");
string xPathString = "//bk:books/bk:book[@ISBN='" + uniqueAttribute + "']";
XmlNode xmlNode = doc.DocumentElement.SelectSingleNode(xPathString, nsmgr);
return xmlNode;
}
******************获取单个节点的属性和子节点的值*****************
public void GetBookInformation(ref string title, ref string ISBN, ref string publicationDate,
ref string price, ref string genre, XmlNode book)
{
XmlElement bookElement = (XmlElement)book;
// Get the attributes of a book.
XmlAttribute attr = bookElement.GetAttributeNode("ISBN");
ISBN = attr.InnerXml;
attr = bookElement.GetAttributeNode("genre");
genre = attr.InnerXml;
attr = bookElement.GetAttributeNode("publicationdate");
publicationDate = attr.InnerXml;
// Get the values of child elements of a book.
title = bookElement["title"].InnerText;
price = bookElement["price"].InnerText;
}
******************获取节点集合*****************
//This example selects all books where the author's last name is Austen,
//and then changes the price of those books.
using System;
using System.IO;
using System.Xml;
public class Sample {
public static void Main() {
XmlDocument doc = new XmlDocument();
doc.Load("booksort.xml");
XmlNodeList nodeList;
XmlNode root = doc.DocumentElement;
//SelectNodes方法的参数是一个XPath表达式
nodeList=root.SelectNodes("descendant::book[author/last-name='Austen']");
//Change the price on the books.
foreach (XmlNode book in nodeList)
{
book.LastChild.InnerText="15.95";
}
Console.WriteLine("Display the modified XML document....");
doc.Save(Console.Out);
}
}
//使用节点名获取节点集合
using System;
using System.IO;
using System.Xml;
public class Sample
{
public static void Main()
{
//Create the XmlDocument.
XmlDocument doc = new XmlDocument();
doc.Load("books.xml");
//Display all the book titles.
XmlNodeList elemList = doc.GetElementsByTagName("title");
for (int i=0; i < elemList.Count; i++)
{
Console.WriteLine(elemList[i].InnerXml);
}
}
}
******************编辑节点*****************
//This example edits a book node and its attributes.编辑单个节点
public void editBook(string title, string ISBN, string publicationDate,
string genre, string price, XmlNode book, bool validateNode, bool generateSchema)
{
XmlElement bookElement = (XmlElement)book;
// Get the attributes of a book.
bookElement.SetAttribute("ISBN", ISBN);
bookElement.SetAttribute("genre", genre);
bookElement.SetAttribute("publicationdate", publicationDate);
// Get the values of child elements of a book.
bookElement["title"].InnerText = title;
bookElement["price"].InnerText = price;
if (validateNode)
{
validateXML(generateSchema, bookElement.OwnerDocument);
}
}
******************添加节点*****************
//要添加节点,请使用CreateElement方法或CreateNode方法;
//要添加一个数据节点,比如一本书,使用CreateElement方法。
//对于任何其他类型的节点,如注释、空白节点或CDATA节点,请使用CreateNode方法
//本例创建一个book节点,向该节点添加属性,然后将该节点添加到文档中
public XmlElement AddNewBook(string genre, string ISBN, string misc,
string title, string price, XmlDocument doc)
{
// Create a new book element.
XmlElement bookElement = doc.CreateElement("book", "http://www.contoso.com/books");
// Create attributes for book and append them to the book element.
XmlAttribute attribute = doc.CreateAttribute("genre");
attribute.Value = genre;
bookElement.Attributes.Append(attribute);
attribute = doc.CreateAttribute("ISBN");
attribute.Value = ISBN;
bookElement.Attributes.Append(attribute);
attribute = doc.CreateAttribute("publicationdate");
attribute.Value = misc;
bookElement.Attributes.Append(attribute);
// Create and append a child element for the title of the book.
XmlElement titleElement = doc.CreateElement("title");
titleElement.InnerText = title;
bookElement.AppendChild(titleElement);
// Introduce a newline character so that XML is nicely formatted.
bookElement.InnerXml =
bookElement.InnerXml.Replace(titleElement.OuterXml,
"\n " + titleElement.OuterXml + " \n ");
// Create and append a child element for the price of the book.
XmlElement priceElement = doc.CreateElement("price");
priceElement.InnerText= price;
bookElement.AppendChild(priceElement);
// Introduce a newline character so that XML is nicely formatted.
bookElement.InnerXml =
bookElement.InnerXml.Replace(priceElement.OuterXml, priceElement.OuterXml + " \n ");
return bookElement;
}
******************移除节点*****************
//移除节点使用RemoveChild方法
//This example removes a book from the document and any whitespace that appears just before the book node.
public void deleteBook(XmlNode book)
{
XmlNode prevNode = book.PreviousSibling;
book.OwnerDocument.DocumentElement.RemoveChild(book);
if (prevNode.NodeType == XmlNodeType.Whitespace ||
prevNode.NodeType == XmlNodeType.SignificantWhitespace)
{
prevNode.OwnerDocument.DocumentElement.RemoveChild(prevNode);
}
}
******************节点位置*****************
可以使用InsertBefore和InsertAfter方法选择要在文档中显示节点的位置。
这个例子显示了两个辅助方法。其中一个向上移动列表中的一个节点。另一个向下移动一个节点。
这些方法可用于允许用户在图书列表中上下移动图书的应用程序。
当用户选择了一本书并按下向上或向下按钮时,您的代码可以调用这样的方法来定位相应的书节点在其他书节点之前或之后的位置
//************************************************************************************
//
// Summary: Move elements up in the XML.
//
//
//************************************************************************************
public void MoveElementUp(XmlNode book)
{
XmlNode previousNode = book.PreviousSibling;
while (previousNode != null && (previousNode.NodeType != XmlNodeType.Element))
{
previousNode = previousNode.PreviousSibling;
}
if (previousNode != null)
{
XmlNode newLineNode = book.NextSibling;
book.OwnerDocument.DocumentElement.RemoveChild(book);
if (newLineNode.NodeType == XmlNodeType.Whitespace |
newLineNode.NodeType == XmlNodeType.SignificantWhitespace)
{
newLineNode.OwnerDocument.DocumentElement.RemoveChild(newLineNode);
}
InsertBookElement((XmlElement)book, Constants.positionAbove,
previousNode, false, false);
}
}
//************************************************************************************
//
// Summary: Move elements down in the XML.
//
//
//************************************************************************************
public void MoveElementDown(XmlNode book)
{
// Walk backwards until we find an element - ignore text nodes
XmlNode NextNode = book.NextSibling;
while (NextNode != null && (NextNode.NodeType != XmlNodeType.Element))
{
NextNode = NextNode.NextSibling;
}
if (NextNode != null)
{
XmlNode newLineNode = book.PreviousSibling;
book.OwnerDocument.DocumentElement.RemoveChild(book);
if (newLineNode.NodeType == XmlNodeType.Whitespace |
newLineNode.NodeType == XmlNodeType.SignificantWhitespace)
{
newLineNode.OwnerDocument.DocumentElement.RemoveChild(newLineNode);
}
InsertBookElement((XmlElement)book, Constants.positionBelow,
NextNode, false, false);
}
}
微软官方XML文档介绍:https://docs.microsoft.com/zh-cn/dotnet/standard/data/xml/