AbstractLoadBalance
Dubbo 中,所有负载均衡实现类均继承自 AbstractLoadBalance,该类实现了 LoadBalance 接口,并封装了一些公共的逻辑。如下
public <T> Invoker<T> select(List<Invoker<T>> invokers, URL url, Invocation invocation) {
if (invokers != null && invokers.size() != 0) {
return invokers.size() == 1 ? (Invoker)invokers.get(0) : this.doSelect(invokers, url, invocation);
} else {
return null;
}
}
而AbstractLoadBalance实现了, LoadBalance接口
@SPI("random")
public interface LoadBalance {
@Adaptive({"loadbalance"})
<T> Invoker<T> select(List<Invoker<T>> var1, URL var2, Invocation var3) throws RpcException;
}
AbstractLoadBalance除了实现了基本的负载均衡的逻辑之外,还封装了一些公共逻辑,比如服务提供者权重计算逻辑。getWeight()等。
protected int getWeight(Invoker<?> invoker, Invocation invocation) {
// 从 url 中获取权重 weight 配置值
int weight = invoker.getUrl().getMethodParameter(invocation.getMethodName(), Constants.WEIGHT_KEY, Constants.DEFAULT_WEIGHT);
if (weight > 0) {
// 获取服务提供者启动时间戳
long timestamp = invoker.getUrl().getParameter(Constants.REMOTE_TIMESTAMP_KEY, 0L);
if (timestamp > 0L) {
// 计算服务提供者运行时长
int uptime = (int) (System.currentTimeMillis() - timestamp);
// 获取服务预热时间,默认为10分钟
int warmup = invoker.getUrl().getParameter(Constants.WARMUP_KEY, Constants.DEFAULT_WARMUP);
// 如果服务运行时间小于预热时间,则重新计算服务权重,即降权
if (uptime > 0 && uptime < warmup) {
// 重新计算服务权重
weight = calculateWarmupWeight(uptime, warmup, weight);
}
}
}
return weight;
}
static int calculateWarmupWeight(int uptime, int warmup, int weight) {
// 计算权重,下面代码逻辑上形似于 (uptime / warmup) * weight。
// 随着服务运行时间 uptime 增大,权重计算值 ww 会慢慢接近配置值 weight
int ww = (int) ((float) uptime / ((float) warmup / (float) weight));
return ww < 1 ? 1 : (ww > weight ? weight : ww);
}
该过程主要用于保证当服务运行时长小于服务预热时间时,对服务进行降权,避免让服务在启动之初就处于高负载状态。服务预热是一个优化手段,与此类似的还有 JVM 预热。主要目的是让服务启动后“低功率”运行一段时间,使其效率慢慢提升至最佳状态。
RandomLoadBalance
源码如下:
public class RandomLoadBalance extends AbstractLoadBalance {
public static final String NAME = "random";
private final Random random = new Random();
public RandomLoadBalance() {
}
protected <T> Invoker<T> doSelect(List<Invoker<T>> invokers, URL url, Invocation invocation) {
int length = invokers.size();
int totalWeight = 0;
boolean sameWeight = true;
int offset;
for(offset = 0; offset < length; ++offset) {
int weight = this.getWeight((Invoker)invokers.get(offset), invocation);
totalWeight += weight;
if (sameWeight && offset > 0 && weight != this.getWeight((Invoker)invokers.get(offset - 1), invocation)) {
sameWeight = false;
}
}
if (totalWeight > 0 && !sameWeight) {
offset = this.random.nextInt(totalWeight);
Iterator i$ = invokers.iterator();
while(i$.hasNext()) {
Invoker<T> invoker = (Invoker)i$.next();
offset -= this.getWeight(invoker, invocation);
if (offset < 0) {
return invoker;
}
}
}
return (Invoker)invokers.get(this.random.nextInt(length));
}
}
其中计算法权重的使用:
循环让 offset 数减去服务提供者权重值,当 offset 小于0时,返回相应的 Invoker。
- 举例说明一下,我们有 servers = [A, B, C],weights = [5, 3, 2],offset = 7。
- 第一次循环,offset - 5 = 2 > 0,即 offset > 5,
- 表明其不会落在服务器 A 对应的区间上。
- 第二次循环,offset - 3 = -1 < 0,即 5 < offset < 8,
- 表明其会落在服务器 B 对应的区间上
在经过多次请求后,能够将调用请求按照权重值进行“均匀”分配。RandomLoadBalance 也存在一定的缺点,当调用次数比较少时,Random 产生的随机数可能会比较集中,此时多数请求会落到同一台服务器上。这个缺点并不是很严重,多数情况下可以忽略。RandomLoadBalance 是一个简单,高效的负载均衡实现,因此 Dubbo 选择它作为缺省实现.
LeastActiveLoadBalance
LeastActiveLoadBalance 翻译过来是最小活跃数负载均衡。活跃调用数越小,表明该服务提供者效率越高,单位时间内可处理更多的请求。此时应优先将请求分配给该服务提供者。在具体实现中,每个服务提供者对应一个活跃数 active。初始情况下,所有服务提供者活跃数均为0。每收到一个请求,活跃数加1,完成请求后则将活跃数减1。在服务运行一段时间后,性能好的服务提供者处理请求的速度更快,因此活跃数下降的也越快,此时这样的服务提供者能够优先获取到新的服务请求、这就是最小活跃数负载均衡算法的基本思想。除了最小活跃数,LeastActiveLoadBalance 在实现上还引入了权重值。所以准确的来说,LeastActiveLoadBalance 是基于加权最小活跃数算法实现的。举个例子说明一下,在一个服务提供者集群中,有两个性能优异的服务提供者。某一时刻它们的活跃数相同,此时 Dubbo 会根据它们的权重去分配请求,权重越大,获取到新请求的概率就越大。如果两个服务提供者权重相同,此时随机选择一个即可。
public class LeastActiveLoadBalance extends AbstractLoadBalance {
public static final String NAME = "leastactive";
private final Random random = new Random();
@Override
protected <T> Invoker<T> doSelect(List<Invoker<T>> invokers, URL url, Invocation invocation) {
int length = invokers.size();
// 最小的活跃数
int leastActive = -1;
// 具有相同“最小活跃数”的服务者提供者(以下用 Invoker 代称)数量
int leastCount = 0;
// leastIndexs 用于记录具有相同“最小活跃数”的 Invoker 在 invokers 列表中的下标信息
int[] leastIndexs = new int[length];
int totalWeight = 0;
// 第一个最小活跃数的 Invoker 权重值,用于与其他具有相同最小活跃数的 Invoker 的权重进行对比,
// 以检测是否“所有具有相同最小活跃数的 Invoker 的权重”均相等
int firstWeight = 0;
boolean sameWeight = true;
// 遍历 invokers 列表
for (int i = 0; i < length; i++) {
Invoker<T> invoker = invokers.get(i);
// 获取 Invoker 对应的活跃数
int active = RpcStatus.getStatus(invoker.getUrl(), invocation.getMethodName()).getActive();
// 获取权重 - ⭐️
int weight = invoker.getUrl().getMethodParameter(invocation.getMethodName(), Constants.WEIGHT_KEY, Constants.DEFAULT_WEIGHT);
// 发现更小的活跃数,重新开始
if (leastActive == -1 || active < leastActive) {
// 使用当前活跃数 active 更新最小活跃数 leastActive
leastActive = active;
// 更新 leastCount 为 1
leastCount = 1;
// 记录当前下标值到 leastIndexs 中
leastIndexs[0] = i;
totalWeight = weight;
firstWeight = weight;
sameWeight = true;
// 当前 Invoker 的活跃数 active 与最小活跃数 leastActive 相同
} else if (active == leastActive) {
// 在 leastIndexs 中记录下当前 Invoker 在 invokers 集合中的下标
leastIndexs[leastCount++] = i;
// 累加权重
totalWeight += weight;
// 检测当前 Invoker 的权重与 firstWeight 是否相等,
// 不相等则将 sameWeight 置为 false
if (sameWeight && i > 0
&& weight != firstWeight) {
sameWeight = false;
}
}
}
// 当只有一个 Invoker 具有最小活跃数,此时直接返回该 Invoker 即可
if (leastCount == 1) {
return invokers.get(leastIndexs[0]);
}
// 有多个 Invoker 具有相同的最小活跃数,但它们之间的权重不同
if (!sameWeight && totalWeight > 0) {
// 随机生成一个 [0, totalWeight) 之间的数字
int offsetWeight = random.nextInt(totalWeight);
// 循环让随机数减去具有最小活跃数的 Invoker 的权重值,
// 当 offset 小于等于0时,返回相应的 Invoker
for (int i = 0; i < leastCount; i++) {
int leastIndex = leastIndexs[i];
// 获取权重值,并让随机数减去权重值 - ⭐️
offsetWeight -= getWeight(invokers.get(leastIndex), invocation);
if (offsetWeight <= 0)
return invokers.get(leastIndex);
}
}
// 如果权重相同或权重为0时,随机返回一个 Invoker
return invokers.get(leastIndexs[random.nextInt(leastCount)]);
}
}
- 遍历 invokers 列表,寻找活跃数最小的 Invoker
- 如果有多个 Invoker 具有相同的最小活跃数,此时记录下这些 Invoker 在 invokers 集合中的下标,并累加它们的权重,比较它们的权重值是否相等
- 如果只有一个 Invoker 具有最小的活跃数,此时直接返回该 Invoker 即可
- 如果有多个 Invoker 具有最小活跃数,且它们的权重不相等,此时处理方式和 RandomLoadBalance 一致
- 如果有多个 Invoker 具有最小活跃数,但它们的权重相等,此时随机返回一个即可
ConsistentHashLoadBalance
一致性Hash算法实现负载均衡,它的工作过程是这样的,首先根据 ip 或者其他的信息为缓存节点生成一个 hash,并将这个 hash 投射到 [0, 232 - 1] 的圆环上。当有查询或写入请求时,则为缓存项的 key 生成一个 hash 值。然后查找第一个大于或等于该 hash 值的缓存节点,并到这个节点中查询或写入缓存项。如果当前节点挂了,则在下一次查询或写入缓存时,为缓存项查找另一个大于其 hash 值的缓存节点即可。大致效果如下图所示,每个缓存节点在圆环上占据一个位置。如果缓存项的 key 的 hash 值小于缓存节点 hash 值,则到该缓存节点中存储或读取缓存项。比如下面绿色点对应的缓存项将会被存储到 cache-2 节点中。由于 cache-3 挂了,原本应该存到该节点中的缓存项最终会存储到 cache-4 节点中。
我们把上图的缓存节点替换成 Dubbo 的服务提供者,于是得到了下图:
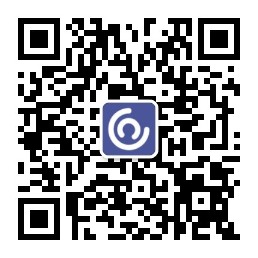
这里相同颜色的节点均属于同一个服务提供者,比如 Invoker1-1,Invoker1-2,……, Invoker1-160。这样做的目的是通过引入虚拟节点,让 Invoker 在圆环上分散开来,避免数据倾斜问题。所谓数据倾斜是指,由于节点不够分散,导致大量请求落到了同一个节点上,而其他节点只会接收到了少量请求的情况.
public class ConsistentHashLoadBalance extends AbstractLoadBalance {
public static final String NAME = "consistenthash";
private final ConcurrentMap<String, ConsistentHashLoadBalance.ConsistentHashSelector<?>> selectors = new ConcurrentHashMap();
private static final int SERVICE_STRATEGY = 1;
private static final int METHOD_STRATEGY = 0;
private static final String PARAM_NAME = "consistenttype";
private static final ThreadLocal<MessageDigest> MD5_THREADLOCAL = new ThreadLocal<MessageDigest>() {
protected MessageDigest initialValue() {
try {
return MessageDigest.getInstance("MD5");
} catch (Exception var2) {
throw new IllegalStateException(var2.getMessage(), var2);
}
}
};
public ConsistentHashLoadBalance() {
}
protected int getConsistentType(URL url, String methodName) {
return KeyWrapperUtils.getMethodParameter(url, methodName, this.keyWrapper, "consistenttype", 0);
}
protected String getHashArguments(URL url, String methodName) {
return KeyWrapperUtils.getMethodParameter(url, methodName, this.keyWrapper, "hash.arguments", "0");
}
protected int getHashNodes(URL url, String methodName) {
return KeyWrapperUtils.getMethodParameter(url, methodName, this.keyWrapper, "hash.nodes", 160);
}
protected <T> Invoker<T> doSelect(List<Invoker<T>> invokers, URL url, Invocation invocation) {
Integer level = this.getConsistentType(url, invocation.getMethodName());
String key;
if (level == 1) {
key = ((Invoker)invokers.get(0)).getUrl().getServiceKey();
} else {
key = ((Invoker)invokers.get(0)).getUrl().getServiceKey() + "." + invocation.getMethodName();
}
int identityHashCode = System.identityHashCode(invokers);
ConsistentHashLoadBalance.ConsistentHashSelector<T> selector = (ConsistentHashLoadBalance.ConsistentHashSelector)this.selectors.get(key);
if (selector == null || selector.identityHashCode != identityHashCode) {
this.selectors.put(key, new ConsistentHashLoadBalance.ConsistentHashSelector(this, invokers, invocation.getMethodName(), identityHashCode));
selector = (ConsistentHashLoadBalance.ConsistentHashSelector)this.selectors.get(key);
}
return selector.select(invocation);
}
private static final class ConsistentHashSelector<T> {
private final TreeMap<Long, Invoker<T>> virtualInvokers = new TreeMap();
public final int identityHashCode;
private final int[] argumentIndex;
public ConsistentHashSelector(ConsistentHashLoadBalance loadBalance, List<Invoker<T>> invokers, String methodName, int identityHashCode) {
this.identityHashCode = identityHashCode;
URL url = ((Invoker)invokers.get(0)).getUrl();
String[] index = Constants.COMMA_SPLIT_PATTERN.split(loadBalance.getHashArguments(url, methodName));
this.argumentIndex = new int[index.length];
int replicaNumber;
for(replicaNumber = 0; replicaNumber < index.length; ++replicaNumber) {
this.argumentIndex[replicaNumber] = Integer.parseInt(index[replicaNumber]);
}
replicaNumber = loadBalance.getHashNodes(url, methodName);
Iterator i$ = invokers.iterator();
while(i$.hasNext()) {
Invoker<T> invoker = (Invoker)i$.next();
String address = invoker.getUrl().getAddress();
for(int i = 0; i < replicaNumber / 4; ++i) {
byte[] digest = this.md5(address + i);
for(int h = 0; h < 4; ++h) {
long m = this.hash(digest, h);
this.virtualInvokers.put(m, invoker);
}
}
}
}
public Invoker<T> select(Invocation invocation) {
String key = this.toKey(invocation.getArguments());
byte[] digest = this.md5(key);
return this.selectForKey(this.hash(digest, 0));
}
private String toKey(Object[] args) {
StringBuilder buf = new StringBuilder();
int[] arr$ = this.argumentIndex;
int len$ = arr$.length;
for(int i$ = 0; i$ < len$; ++i$) {
int i = arr$[i$];
if (i >= 0 && i < args.length) {
buf.append(args[i]);
}
}
return buf.toString();
}
private Invoker<T> selectForKey(long hash) {
Long key = hash;
if (!this.virtualInvokers.containsKey(key)) {
SortedMap<Long, Invoker<T>> tailMap = this.virtualInvokers.tailMap(key);
if (tailMap.isEmpty()) {
key = (Long)this.virtualInvokers.firstKey();
} else {
key = (Long)tailMap.firstKey();
}
}
Invoker<T> invoker = (Invoker)this.virtualInvokers.get(key);
return invoker;
}
private long hash(byte[] digest, int number) {
return ((long)(digest[3 + number * 4] & 255) << 24 | (long)(digest[2 + number * 4] & 255) << 16 | (long)(digest[1 + number * 4] & 255) << 8 | (long)(digest[number * 4] & 255)) & 4294967295L;
}
private byte[] md5(String value) {
MessageDigest md5 = (MessageDigest)ConsistentHashLoadBalance.MD5_THREADLOCAL.get();
md5.reset();
byte[] bytes;
try {
bytes = value.getBytes("UTF-8");
} catch (UnsupportedEncodingException var5) {
throw new IllegalStateException(var5.getMessage(), var5);
}
md5.update(bytes);
return md5.digest();
}
}
}
代码有点多, 总的来说是先试用 ConsistentHashSelector 初始化节点的配置信息,然后在计算节点的时候计算虚拟节点的hash值,TreeMap存储节点信息, 以后每次查找时,对参数进行 md5 以及 hash 运算,得到一个 hash 值。然后再拿这个值到 TreeMap 中查找目标 Invoker 即可。
一致性hash算法使用的场景比较多,最好先自己熟悉这个算法,再来看代码就容易很多了。