问题描述
练习 2-8 编写一个函数rightrot(x, n),该函数返回将x循环右移(即从最右端移出的位将从最左端移入)n(二进制)位后所得到的值。
Write a function rightrot(x,n)
that returns the value of the integer x
rotated to the right by n
bit positions.
解题思路
这个题目我感觉可以有两种理解方式
第一种
就是默认二进制位数,前面没有多余的0来补位,比如111的二进制是1101 111,我就认为题目的函数对1101 111进行循环右移,就是把最右端的数字放到最左端,即 1101 111
第二种
这一种理解方式是比较符合实际的,就是计算机中二进制数位是有规定的,比如有的计算机是32位
那么111的二进制就不是1101 111了,而是
扫描二维码关注公众号,回复:
11199922 查看本文章
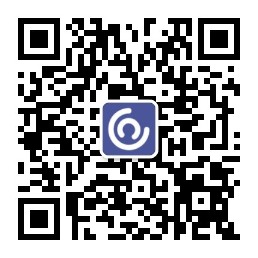
0000 0000 0000 0000 0000 0000 0110 1111(在前面补了25位0,对数值大小无影响)
那么我们把最右端的一个数字放到最左端就是1000 0000 0000 0000 0000 0000 0110 111 结果显然与第一种不同
思路一代码:
#include<stdio.h> int rightrot(unsigned int x , int n) { int k,i; int ribits; k = x; for ( i = 0; k!=0; i++)//算出x二进制形式有多少位 { k=k>>1; } ribits = (~(~0<<n) & x)<<(i-n); x = (x>>n) | ribits; return x; } int main() { printf("%d",rightrot(111,3)); return 0; }
运行输出
思路二代码
#include<stdio.h> unsigned rightrot(unsigned x, unsigned n) { while (n > 0) { if ((x & 1) == 1) x = (x >> 1) | ~(~0U >> 1);//U表示无符号,相当于unsigned else x = (x >> 1); n--; } return x; } int main() { printf("%d",rightrot(111,3)); return 0; }
运行结果