迭代法
思路:
当L1和L2都不是空链表时,判断L1和L2哪一个链表的头节点的值更小,将较小值的节点添加到结果里。当一个节点添加到结果里后,将对应链表中的节点向后移一位。
代码:
# Definition for singly-linked list. # class ListNode: # def __init__(self, x): # self.val = x # self.next = None class Solution: def mergeTwoLists(self, l1: ListNode, l2: ListNode) -> ListNode: newList = ListNode(0) first = newList while l1 and l2: if l1.val < l2.val: first.next = l1 l1 = l1.next else: first.next = l2 l2 = l2.next first = first.next else: first.next = l1 if l1 else l2 return newList.next
递归法
思路:
考虑到从头开始每个节点后面都是有序的,所以可以采用递归的方法对后面的节点进行有序拼接。
代码:
扫描二维码关注公众号,回复:
11172971 查看本文章
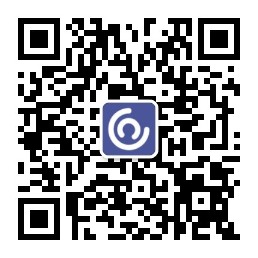
# Definition for singly-linked list. # class ListNode: # def __init__(self, x): # self.val = x # self.next = None class Solution: def mergeTwoLists(self, l1: ListNode, l2: ListNode) -> ListNode: if not l1: return l2 elif not l2: return l1 elif l1.val < l2.val: l1.next = self.mergeTwoLists(l1.next,l2) return l1 else: l2.next = self.mergeTwoLists(l1,l2.next) return l2