记录自己参加双周赛的历程,这次是首次参加,AC了第1道,其他三道题是赛后学习了大神们的写法。
历届周赛或双周赛链接:https://leetcode-cn.com/contest/
1、重新格式化字符串
给你一个混合了数字和字母的字符串 s,其中的字母均为小写英文字母。请你将该字符串重新格式化,使得任意两个相邻字符的类型都不同。也就是说,字母后面应该跟着数字,而数字后面应该跟着字母。请你返回 重新格式化后 的字符串;如果无法按要求重新格式化,则返回一个 空字符串 。
输入:s = “a0b1c2”
输出:“0a1b2c”
解释:“0a1b2c” 中任意两个相邻字符的类型都不同。 “a0b1c2”, “0a1b2c”, “0c2a1b” 也是满足题目要求的答案。
来源:力扣(LeetCode)
链接:https://leetcode-cn.com/problems/reformat-the-string
题解:
class Solution:
def reformat(self, s: str) -> str:
lens = len(s)
if lens == 1:
return s
a = "" # 存数字
b = "" # 存字母
c = "" # 存最后的
for i in range(lens):
# for j in range(10):
# if int(s[i]) == j:
if ord('0') <= ord(s[i]) and ord(s[i]) <= ord('9'):
a = a + s[i]
if ord('a') <= ord(s[i]) and ord(s[i]) <= ord('z'):
b = b + s[i]
if b == "" or a == "":
return ""
if len(a) == len(b) - 1:
for i in range(len(a)):
c = c + b[i]
c = c + a[i]
c = c + b[len(b) - 1]
return c
elif len(a) - 1 == len(b):
for i in range(len(b)):
c = c + a[i]
c = c + b[i]
c = c + a[len(a) - 1]
return c
elif len(a) == len(b):
for i in range(len(b)):
c = c + a[i]
c = c + b[i]
return c
else:
return ""
2、点菜展示表
给你一个数组 orders,表示客户在餐厅中完成的订单,确切地说,orders[i]=[customerNamei,tableNumberi,foodItemi] ,其中 customerNamei 是客户的姓名,tableNumberi 是客户所在餐桌的桌号,而 foodItemi 是客户点的餐品名称。请你返回该餐厅的 点菜展示表 。在这张表中,表中第一行为标题,其第一列为餐桌桌号 “Table” ,后面每一列都是按字母顺序排列的餐品名称。接下来每一行中的项则表示每张餐桌订购的相应餐品数量,第一列应当填对应的桌号,后面依次填写下单的餐品数量。
注意:客户姓名不是点菜展示表的一部分。此外,表中的数据行应该按餐桌桌号升序排列。
来源:力扣(LeetCode)
链接:https://leetcode-cn.com/problems/display-table-of-food-orders-in-a-restaurant
输入:orders = [[“David”,“3”,“Ceviche”],[“Corina”,“10”,“Beef Burrito”],[“David”,“3”,“Fried Chicken”],[“Carla”,“5”,“Water”],[“Carla”,“5”,“Ceviche”],[“Rous”,“3”,“Ceviche”]]
输出:[[“Table”,“Beef Burrito”,“Ceviche”,“Fried Chicken”,“Water”],[“3”,“0”,“2”,“1”,“0”],[“5”,“0”,“1”,“0”,“1”],[“10”,“1”,“0”,“0”,“0”]]
解释:
点菜展示表如下所示:
Table,Beef Burrito,Ceviche,Fried Chicken,Water
3 ,0 ,2 ,1 ,0
5 ,0 ,1 ,0 ,1
10 ,1 ,0 ,0 ,0
对于餐桌 3:David 点了 “Ceviche” 和 “Fried Chicken”,而 Rous 点了 “Ceviche”
而餐桌 5:Carla 点了 “Water” 和 “Ceviche”
餐桌 10:Corina 点了 “Beef Burrito”
题解:
class Solution:
def displayTable(self, orders: List[List[str]]) -> List[List[str]]:
table = collections.defaultdict(dict)
tablenum = set()
for c, t, f in orders:
tablenum.add(int(t))
table[f][t] = table[f].get(t, 0) + 1
tablenum = sorted(tablenum)
foodname = sorted(table.keys())
ans = [['Table'] + foodname]
for t in tablenum:
ans.append([str(t)])
for f in foodname:
ans[-1].append(str(table[f].get(str(t), 0)))
return ans
3、数青蛙
给你一个字符串 croakOfFrogs,它表示不同青蛙发出的蛙鸣声(字符串 “croak” )的组合。由于同一时间可以有多只青蛙呱呱作响,所以 croakOfFrogs 中会混合多个 “croak” 。请你返回模拟字符串中所有蛙鸣所需不同青蛙的最少数目。
注意:要想发出蛙鸣 “croak”,青蛙必须 依序 输出 ‘c’, ’r’, ’o’, ’a’, ’k’ 这 5 个字母。如果没有输出全部五个字母,那么它就不会发出声音。如果字符串 croakOfFrogs 不是由若干有效的 “croak” 字符混合而成,请返回 -1 。
来源:力扣(LeetCode)
链接:https://leetcode-cn.com/problems/minimum-number-of-frogs-croaking
输入:croakOfFrogs = “croakcroak”
输出:1
解释:一只青蛙 “呱呱” 两次
输入:croakOfFrogs = “crcoakroak”
输出:2
解释:最少需要两只青蛙,“呱呱” 声用黑体标注
第一只青蛙 “crcoakroak”
第二只青蛙 “crcoakroak”
class Solution:
def minNumberOfFrogs(self, cr: str) -> int:
pre = [0] * 5
res = 0
for c in cr:
if c == 'c':
pre[0] += 1
if c == 'r':
pre[0] -= 1
pre[1] += 1
if c == 'o':
pre[1] -= 1
pre[2] += 1
if c == 'a':
pre[2] -= 1
pre[3] += 1
if c == 'k':
pre[3] -= 1
if any(c < 0 for c in pre):
return -1
res = max(res, sum(pre))
if any(c != 0 for c in pre):
return -1
return res
4、生成数组
给你三个整数 n、m 和 k 。下图描述的算法用于找出正整数数组中最大的元素。
请你生成一个具有下述属性的数组 arr :
arr 中有 n 个整数。
1 <= arr[i] <= m 其中 (0 <= i < n) 。
将上面提到的算法应用于 arr ,search_cost 的值等于 k 。
返回上述条件下生成数组 arr 的 方法数 ,由于答案可能会很大,所以 必须 对 10^9 + 7 取余。
来源:力扣(LeetCode)
链接:https://leetcode-cn.com/problems/build-array-where-you-can-find-the-maximum-exactly-k-comparisons
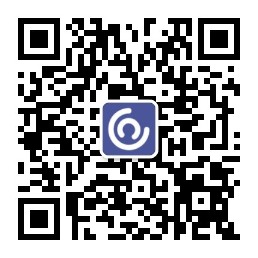
输入:n = 2, m = 3, k = 1
输出:6
解释:可能的数组分别为 [1, 1], [2, 1], [2, 2], [3, 1], [3, 2] [3, 3]
输入:n = 9, m = 1, k = 1
输出:1
解释:可能的数组只有 [1, 1, 1, 1, 1, 1, 1, 1, 1]
输入:n = 50, m = 100, k = 25
输出:34549172
解释:不要忘了对 1000000007 取余
class Solution:
def numOfArrays(self, n: int, m: int, k: int) -> int:
mod = 10 ** 9 + 7
dp = [[[0] * (k + 1) for _ in range(m + 1)] for _ in range(n)]
for i in range(1, m + 1):
dp[0][i][1] = 1
for i in range(1, n):
for j in range(1, m + 1):
for pre in range(1, m + 1):
if pre >= j:
for kk in range(k + 1):
dp[i][pre][kk] += dp[i - 1][pre][kk]
else:
for kk in range(1, k + 1):
dp[i][j][kk] += dp[i - 1][pre][kk - 1]
res = 0
for i in range(m + 1):
res += dp[-1][i][k]
return res % mod