今天学习了利用数组方式的栈的C++实现,这种方式跟指针实现有很多不一样的地方:
栈的指针实现,栈的创建申请头结点,push需要申请新的结点,pop释放结点,这些结点都放在第一个位置,top时,S->next->data即可。
栈的数组实现,只申请一个结点,该结点的结构体内包含,数组的最大容量、栈顶元素下标、指向整形数组的指针(用于存放和删除新的元素)。
S->topOfStack == -1,空栈;
S->topOfStack == S->capacity - 1,满栈;
1、声明结点
1 struct Node
2 {
3 int capacity; //数组的最大容量
4 int topOfStack; //栈顶下标为-1表示空栈,每次添加新的元素,栈顶元素下标加1
5 int *Array; //指向整形数组的指针
6 };
7 typedef struct Node stack;
2、空栈,满栈判断
1 int stackArray::isEmpty(stack *S)
2 {
3 return S->topOfStack == emptyTOS;
4 }
5 int stackArray::isFull(stack *S)
6 {
7 return S->topOfStack == S->capacity - 1;
8 }
3、创建栈
1 stackArray::stack *stackArray::createStack(int maxElements)
2 {
3 if (maxElements < minStackSize)
4 cout << "the space of stack is too short,please increase value of maxElements!" << endl;
5
6 stack *S;
7 S = (stack*)new(stack);
8 if (S == NULL)
9 cout << "Out of space! " << '\n';
10
11 S->Array = new int[maxElements];
12 if (S->Array == NULL)
13 cout << "Out of space! " << '\n';
14
15 S->topOfStack = emptyTOS; //栈顶下标置-1表示空栈
16 S->capacity = maxElements; //数组最大容量赋值
17 makeEmpty(S);
18 return S;
19 }
5、push,top,pop
1 stackArray::stack *stackArray::push(stack *S)
2 {
3 if (isFull(S))
4 {
5 cout << "stack is full!" << endl;
6 return 0;
7 }
8 int x = 0;
9 cout << "Please input the data to push: " << endl;
10 scanf_s("%d", &x);
11 S->Array[++S->topOfStack] = x;
12 return S;
13 }
14 int stackArray::top(stack *S)
15 {
16 if (isEmpty(S)) //非空判断
17 {
18 cout << "empty stack! " << endl;
19 return -1;
20 }
21 else
22 return S->Array[S->topOfStack];
23 }
24 stackArray::stack *stackArray::pop(stack *S)
25 {
26 if (isEmpty(S)) //非空判断
27 {
28 cout << "empty stack! " << endl;
29 return 0;
30 }
31 else
32 {
33 S->topOfStack--;
34 return S;
35 }
36 }
6、主函数
1 int main(int argc, char * argv[])
2 {
3 cout << '\n' << "***************************************" << '\n' << '\n';
4 cout << "Welcome to the stackArray world! " << '\n';
5 cout << '\n' << "***************************************" << '\n' << '\n';
6
7 int i = 1;
8 int j = 0;
9 int topElement = 0;
10 stackArray *a = new stackArray;
11 stackArray::stack *S = NULL;
12 //int x = 0;
13 while (i)
14 {
15 cout << '\n' << "***************************************" << '\n';
16 cout << " 0 : end the stack " << '\n';
17 cout << " 1 : creat a stack " << '\n';
18 cout << " 2 : display the top element of stack " << '\n';
19 cout << " 3 : push a node in the stack " << '\n';
20 cout << " 4 : pop a node from the stack " << '\n';
21 cout << "***************************************" << '\n';
22 cout << "Please input the function your want with the number above : " << '\n';
23 scanf_s("%d", &j);
24
25 switch (j)
26 {
27 case 1:
28 cout << "CreatStack now begin : ";
29 S = a->createStack(5);
30 break;
31 case 2:
32 topElement = a->top(S);
33 cout << "The top element of stack is : " << topElement;
34 break;
35 case 3:
36 cout << "push now begin : ";
37 S = a->push(S);
38 break;
39 case 4:
40 cout << "pop now begin : ";
41 S = a->pop(S);
42 break;
43 default:
44 cout << "End the stack. ";
45 a->disposeStack(S);
46 i = 0;
47 break;
48 }
49
50 }
51
52 return 0;
53 }
效果同指针,不再赘述。
扫描二维码关注公众号,回复:
10978684 查看本文章
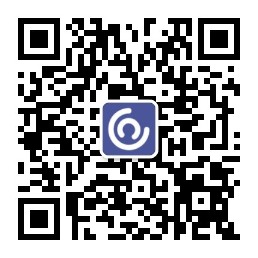