先搞个表
CREATE TABLE `t_user` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`username` varchar(10) DEFAULT NULL,
`pwd` varchar(10) DEFAULT NULL,
`regTime` date DEFAULT NULL,
`lastLoginTime` timestamp NULL DEFAULT NULL ON UPDATE CURRENT_TIMESTAMP,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=20213 DEFAULT CHARSET=utf8;
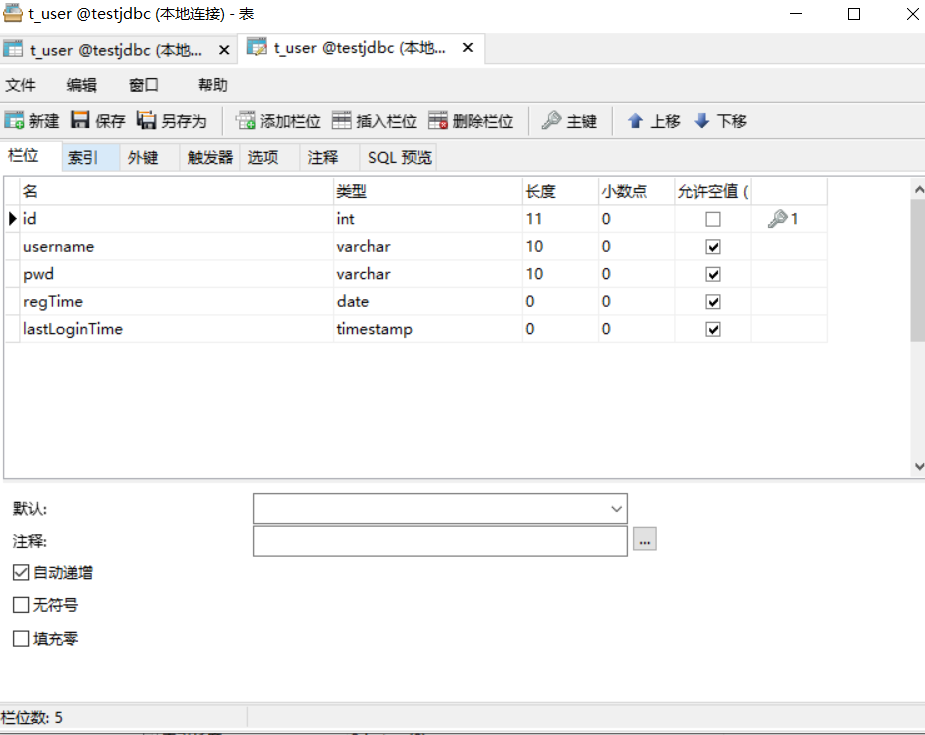
基本操作
// 加载驱动类
Class.forName("com.mysql.jdbc.Driver");
long start = System.currentTimeMillis();
// 创建连接
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/testjdbc", "root", "123456");
long end = System.currentTimeMillis();
System.out.println("建立连接耗时:-->" + (end-start) +" ms");
System.out.println(conn);
Sql注入 Statement
public class Demo02 {
public static void main(String[] args) {
try {
// 加载驱动类
Class.forName("com.mysql.jdbc.Driver");
// 建立连接
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/testjdbc", "root", "123456");
System.out.println(conn);
Statement statement = conn.createStatement();
//statement.execute("insert into t_user(username, pwd, regTime) values('张三','123',now())");
// 测试 SQL 注入
String id = "5 or 1=1";
statement.execute("delete from t_user where id = " + id);
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
}finally {
// 关闭资源
}
}
}
PreparedStatement基本用法
// 加载驱动类
Class.forName("com.mysql.jdbc.Driver");
// 建立连接
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/testjdbc", "root", "123456");
String sql = "insert into t_user (username, pwd, regTime) values(?, ?, ?)";
PreparedStatement ps = conn.prepareStatement(sql);
/*ps.setString(1, "李四");
ps.setString(2, "123456");
ps.setDate(3, new Date(System.currentTimeMillis()));*/
ps.setObject(1, "王五");
ps.setObject(2, "987654");
ps.setObject(3, new Date(System.currentTimeMillis()));
ps.execute();
ResutlSet基本用法
public static void main(String[] args) {
Connection conn = null;
PreparedStatement ps = null;
ResultSet rs = null;
try {
// 加载驱动类
Class.forName("com.mysql.jdbc.Driver");
// 建立连接
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/testjdbc", "root", "123456");
String sql = "select * from t_user where id > ?";
ps = conn.prepareStatement(sql);
ps.setObject(1, 3);
rs = ps.executeQuery();
while(rs.next()) {
System.out.println(rs.getInt(1) + "--" + rs.getString(2) + "--" + rs.getString(3));
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
}finally {
try {
if(rs != null) {
rs.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
try {
if(ps != null) {
ps.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
try {
if(conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}