1.python中的注释
Python中的注释有单行注释和多行注释:
Python中单行注释以 #
开头
多行注释用三个单引号 ‘’’ 或者三个双引号 “”" 将注释括起来
print('hello world')
# this is a comment
print('hello python') #this also a comment
"""
they are all
comment
"""
print('hello westos')
执行结果:
2.基本数据类型
2.1 类型转换
1. 4.0 == 4
2. "4.0" == 4
3. bool("1")
4. bool("0")
5. str(32)
6. int(6.26)
7. float(32)
8. float("3.21")
9. int("434")
10. int("3.42")
11. bool(-1)
12. bool("")
13. bool(0)
14. "wrqq" > "acd"
15. "ttt" == "ttt "
16. "sd"*3
17. "wer" + "2322"
执行结果为:
- True
- False
- True
- True
- '32'
- 6
- 32.0
- 3.21
- 434
- 会报错
- True
- False
- False
- True
- False
- "sdsdsd"
- 'wer2322'
- bool函数转换规则:
bool函数进行转换时,其结果取决于传入参数与True和False的等价关系,只需记住一点即可
0 , 空字符串, None在条件判断语句中等价于False, 其他数值都等价于True
bool函数在做数据类型转换时遵循该原则 - 字符串大小比较规则:
两个字符串在比较大小时,比的不是长度,而是内容。字符串左对齐后,逐个字符依次比较,直到可以分出胜负
2.2 数据类型考察
请说出下面表达式结果的类型:
1. "True"
2. "Flase"
3. 4 >= 5
4. 5
5. 5.0
6. True
1. str
2. str
3. bool
4. int
5. float
6. bool
3.格式化输出
%s #str
%d #int
%f #float
%% #%
name = 'westos'
age = 11
print('%s age is %d' %(name,age))
执行结果为:
westos age is 11
money = 8234.123545
print('%s salary is %f' %(name,money))
执行结果为:
westos salary is 8234.123545
money = 8234
print('%s salary is %f' %(name,money))
执行结果为:
westos salary is 8234.000000
print('%s salary is %.2f' %(name,money))
执行结果为:
westos salary is 8234.00
studentid = 1
print('%s studentid is 031%d' %(name,studentid))
执行结果为:
westos studentid is 0311
print('%s studentid is 031%.3d' %(name,studentid))
执行结果为:
westos studentid is 0310001
scale = 0.1
print('The num is %.2f' %(scale * 100) )
执行结果为:
The num is 10.00
扫描二维码关注公众号,回复:
10955179 查看本文章
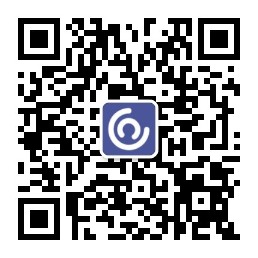
print('The num is %.2f%%' %(scale * 100) )
执行结果为:
The num is 10.00%
示例:
"""
- 输入学生姓名;
- 依次输入学生的三门科目成绩;
- 计算该学生的平均成绩, 并打印;
- 平均成绩保留一位小数点;
- 计算该学生语文成绩占总成绩的百分之多少?并打印。eg: 78%;
"""
name = input('Student Name:')
Chinese = float(input('Chinese:'))
Math = float(input('Math:'))
English = float(input('English:'))
#SumScore
SumScore = Chinese + Math + English
#AvgScore
AvgScore = SumScore / 3
#ChinesePercent
ChinesePercent = (Chinese / SumScore) * 100
print('%s avgscore is %.1f' %(name,AvgScore))
print('ChinesePercent is %.2f%%' %(ChinesePercent))
4. if语句
示例1:
age = 12
if age >= 18:
print('welcome to netbar')
print('--------------')
示例2:
age = 18
if age >= 18:
print('Welcome to netbar')
else:
print('Please go home and do homework')
示例3:
value = input('Value:')
if not value:
print('Check your input!')
示例4:
holiday_name = 'a1'
if holiday_name == 'a2':
print('a2')
elif holiday_name == 'a3':
print('a3')
else:
print('a4')
示例5:
have_ticket = True
knife_length = 18
if have_ticket:
print('Please come in')
if knife_length > 20:
print('length:%d ;too long,not permitted!' %knife_length)
else:
print('length: %d;acceptable long,come in' %knife_length)
else:
print('Please buy ticket')
示例6:
"""
在python中,要使用随机数,首先需要导入随即数模块 -- ‘工具包’
导入模块后,可以直接在模块名称后面敲一个.然后Tab键,会提示该模块中>包含的所有函数
random.randint(a,b),返回[a b]之间的整数,包含a和b
eg: random.randint(12,20):生成随机数n: 12 <= n <= 20
random.randint(20,20): 结果永远是20
random.randint(20,12):结果会报错:下限必须小于上限
"""
"""
1.从控制台输入要出的拳 ---石头(1)/剪刀(2)/布(3)
2.电脑随即出拳--先假定电脑只会出石头,完成整体代码功能
3.比较胜负
石头 胜 剪刀
剪刀 胜 布
布 胜 石头
"""
"""
# 导入随即工具包
# 注意,在导入工具包的时候,应该将导入的语句,放在文件的顶部
# 因为,这样可以方便下方的代码,在任何需要的时候,使用工具包中的工>具
"""
import random
#1.player
player = int(input('Your Choice:'))
#2.computer
computer = random.randint(1,3)
print(computer)
#3.compare
if((player == 1 and computer == 2) or (player == 2 and computer == 3)
or (player == 3 and computer == 1)):
print('player win')
elif player == computer:
print('no winner')
else:
print('computer win')
5. range
>>> range(5)
[0, 1, 2, 3, 4]
>>> range(10)
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
>>>
>>> range(1,10)
[1, 2, 3, 4, 5, 6, 7, 8, 9]
>>> range(1,11)
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
>>> range(1,11,2)
[1, 3, 5, 7, 9]
>>> range(2,11,2)
[2, 4, 6, 8, 10]
5. 跳出循环相关
break:跳出整个循环,不会再循环后面的内容
continue:跳出本次循环,continue后面的代码不再执行,但是循环依然继续
exit():结束程序的运行
示例1:
for i in range(10):
if i == 5:
break
print('hello python')
print(i)
print('hello world')
示例2:
for i in range(10):
if i == 5:
continue
print('hello python')
print(i)
print('hello world')
示例3:
for i in range(10):
if i == 5:
exit()
print('hello python')
print(i)
print('hello world')
6. for循环
示例1:累加器
sum = 0
for i in range(1,101,2):
sum += i
print(sum)
示例2:
"""
有1,2,3,4四个数字
求这四个数字能生成多少个互不相同且无重复数字的三位数(不能含有122,
133这种)
"""
count = 0
for i in range(1,5):
for j in range(1,5):
for x in range(1,5):
if i != j and j != x and i != x:
print(i * 100 + j * 10 + x)
count += 1
print('The count is: %d' %count)
示例3:
"""
用户登陆程序需求:
1. 输入用户名和密码;
2. 判断用户名和密码是否正确? (name='root', passwd='westos')
3. 为了防止暴力破解, 登陆仅有三次机会, 如果超过三次机会,
报错提示;
"""
for i in range(3):
name = input('UserName:')
passwd = input('Password:')
if name == 'root' and passwd == 'westos':
print('Login success')
break
else:
print('Login failed')
print('%d chance last' %(2 - i))
else:
print('Please try later!')
示例4:模拟shell中的命令行
import os
for i in range(1000):
cmd = input('[kiosk@python test]$ ')
if cmd:
if cmd == 'exit':
print('logout')
break
else:
print('run %s' %(cmd))
os.system(cmd)
else:
continue
示例5:
"""
输入两个数值:
求两个数的最大公约数和最小公倍数.
最小公倍数=(num1*num2)/最大公约数
"""
a = input("Please input num1:")
b = input("Please input num2:")
n = []
m = 0
k = 0
for i in range(1,int(a)+1):
if int(a) % i ==0:
n.append(i)
for j in n:
if int(b) % j ==0:
m = j
print(m)
k = int(a) * int(b) / m
print(k)
7. while循环
while 条件:
条件满足时,做的事情1
条件满足时,做的事情2
示例1:
i = 1
while i <= 5:
print('hello python')
i += 1
示例2:死循环
while True:
print('hello python')
示例3:用while循环完成上述for循环中的示例3
trycount = 0
# for i in range(3):
while trycount < 3:
name = input('UserName:')
passwd = input('Password:')
if name == 'root' and passwd == 'westos':
print('Login success')
break
else:
print('Login failed')
print('%d chance last' %(2 - trycount))
trycount += 1
#
else:
print('Please try later!')
示例4:
"""
*
**
***
****
*****
"""
row = 1
while row <= 5:
col = 1
while col <= row:
print('*',end='')
col += 1
print('')
row += 1
示例5:
"""
猜数字游戏
1. 系统随机生成一个1~100的数字;
2. 用户总共有5次猜数字的机会;
3. 如果用户猜测的数字大于系统给出的数字,打印“too big”;
4. 如果用户猜测的数字小于系统给出的数字,打印"too small";
5. 如果用户猜测的数字等于系统给出的数字,打印"恭喜",并且退
出循环;
"""
import random
trycount = 0
computer = random.randint(1,100)
print(computer)
while trycount < 5:
player = int(input('Num:'))
if player > computer:
print('too big')
trycount += 1
elif player < computer:
print('too small')
trycount += 1
else:
print('Congratulation!')
break
8. str字符型
a = 'hello'
b = 'westos'
c = 'what\'s up'
e = """
用户管理系统
1.添加用户
2.删除用户
3.显示用户
"""
print(c)
print(e)
print(type(e))
执行结果为:
8.1 索引
s = 'hello'
print(s[0])
print(s[1])
print(s[2])
8.2 切片
s = 'wangdandan'
print(s[0:3]) #s[start:end-1]
print(s[0:4:2])
print(s[:])
print(s[:3])
print(s[1:])
print(s[::-1])
8.3 重复
s = 'wang'
print(s * 2)
8.4 连接
print('hello' + ' world')
8.5 成员操作符
s = 'hello world'
print('h' in s)
print('f' in s)
示例1:判断一个数是否为回文数
"""
示例 1:
输入: 121
输出: true
示例 2:
输入: -121
输出: false
解释: 从左向右读, 为 -121 。 从右向左读, 为 121- 。因此它不
是一个回文数。
示例 3:
输入: 10
输出: false
解释: 从右向左读, 为 01 。因此它不是一个回文数。
"""
num = input('Num:')
if num == num[::-1]:
print('ok')
else:
print('failed')
判断字符串是否为数字:
print('123'.isdigit())
print('123abc'.isdigit())
判断字符串是否为标题(只有第一个字母为大写,其他均为小写):
print('Hello'.istitle())
print('HelLo'.istitle())
将小写字母转为大写字母
print('hello'.upper())
将大写字母转为小写字母
print('HeLlO'.lower())
判断字符串是否为小写
print('hello'.islower())
判断字符串是否为大写
print('HELLO'.isupper())
判断字符串是否由字母和数字组成
print('HELL1'.isalnum())
判断字符串是否为字母
print('123'.isalpha())
判断字符串是否以固定的字符结尾
filename = 'hello.loggg'
if filename.endswith('.log'):
print(filename)
else:
print('error file')
示例2:
"""
变量名是否合法:
1.变量名可以由字母,数字或者下划线组成
2.变量名只能以字母或者下划线开头
s = 'hello@'
1.判断变量名的第一个元素是否为字母或者下划线 s[0]
2.如果第一个元素符合条件,判断除了第一个元素之外的其他元素s[1:]
"""
s = 'hh77_hhduq99_'
if s[0].isalpha() or s[0] == '_':
for i in s[1:]:
if i.isalpha() or i.isdigit() or i == '_':
continue
else:
print('no')
break
print("ok")
else:
print("no,no.no")