作为一个多年的Android开发的我来说,一个好的网络框架,在一定程度能够提升一个应用的可用性,这样我们就需要找到合适的网络框架,对于比较获得网络框架,前几天比较火的xutils,由于之前工作比较忙,一直没空去好好研究一个新的网络框架,最近闲来无事,了解了最新的比较火的网络框架,那就是okhttp,如果仅仅使用okhttp还不能真正的给自己带来很大的便利,如果加上Retrofit 这样的话就能很大程度的提高代码的可读性。
接下来,咱们来了解写okhttp+Retrofit在项目中我们到底该如何使用。
首先创建请求接口
@Headers({"Content-Type: application/json","Accept: application/json"})//需要添加头 @POST(CommonValue.USER_LOGIN) Call<BaseEntity<String>> bannerCall(@Body RequestBody body);
以上是一个传递json字符串的方法,返回的是call的泛型接口
接下来,配置Retrofit和okhttp
public class RetrofitFactory { private static RetrofitFactory retrofitFactory = null; private Retrofit retrofit; private RetrofitFactory() { retrofit = new Retrofit.Builder().client(OkHttpClientFactory.getInstance().getOkHttpClient()) .addConverterFactory(GsonConverterFactory.create()) .baseUrl(CommonValue.URL) .build(); } public static RetrofitFactory getInstance(){ if(retrofitFactory == null){ retrofitFactory = new RetrofitFactory(); } return retrofitFactory; } public Retrofit getRetrofit(){ return retrofit; } }
public class OkHttpClientFactory { private static OkHttpClientFactory okHttpClientFactory=null; private OkHttpClient okHttpClient; private static final long readTimeOut=15; private static final long connectTimeout=15; private OkHttpClientFactory(){ HttpLoggingInterceptor loggingInterceptor = new HttpLoggingInterceptor(new HttpLoggingInterceptor.Logger() { @Override public void log(String message) { //在这里可以打印请求的具体信息 Log.i("aaa", "log: message == " + message); } }); loggingInterceptor.setLevel(HttpLoggingInterceptor.Level.BODY);//http 数据log 日志中打印出http请求和相应数据 loggingInterceptor.setLevel(HttpLoggingInterceptor.Level.BODY); okHttpClient=new OkHttpClient.Builder().addInterceptor(new CustomerInterceptor()) .addInterceptor(loggingInterceptor) .retryOnConnectionFailure(true)//断网重连 .readTimeout(readTimeOut, TimeUnit.SECONDS) .connectTimeout(connectTimeout, TimeUnit.SECONDS) .build(); } public static OkHttpClientFactory getInstance(){ if (okHttpClientFactory==null){ okHttpClientFactory=new OkHttpClientFactory(); } return okHttpClientFactory; } public OkHttpClient getOkHttpClient() { return okHttpClient; } }
我们还需要传递一些请求头文件之类的东西
public class CustomerInterceptor implements Interceptor { @Override public Response intercept(Chain chain) throws IOException { Request request=chain.request(); HttpUrl httpUrl=request.url().newBuilder() .build(); request=request.newBuilder().url(httpUrl) .header("token",SharedPerferenceUtil.getData(MyApp.getContext(),"token","")+"") .build(); return chain.proceed(request); } }
目前大部分需要的文件已经基本完成,我们还需要写一个回调接口的方法,
扫描二维码关注公众号,回复:
1093401 查看本文章
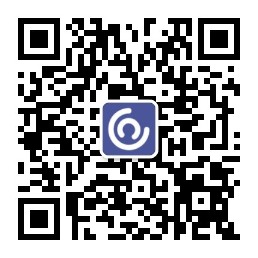
public abstract class RetrofitResultCallBack<T extends BaseEntity> implements Callback<T> { @Override public void onResponse(Call<T> call, Response<T> response) { //定义状态码 根据状态码的不同,给出不同的提示 int code = response.code(); if (response.raw().code() == 200) { if (response.body().getCode().equals("0")) { onSuccess(response.body()); } else if (response.body().getMsg().equals("用户未登录或已失效")) { new android.os.Handler().postDelayed(new Runnable() { @Override public void run() { Intent intent = new Intent(MyApp.getContext(), TestActivity.class); intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK); MyApp.getContext().startActivity(intent); } }, 3000); } else { onFail(response.body().getMsg()); } } else { onFail("网络错误,请检查网络 "); } } @Override public void onFailure(Call<T> call, Throwable t) { onFail("网络错误,请检查网络 "); } public abstract void onSuccess(Object object); public abstract void onFail(String message);}
目前大部分功能已经结束了
由于我们现在要使用的是将map转json字符串,那么我们还需要一个map转json相关的配置
public class RequestBodyUtil { private static RequestBodyUtil instance; public static RequestBodyUtil getInstance(){ if (null==instance){ instance=new RequestBodyUtil(); } return instance; } /** * 将穿入的集合转化为加密的body * @param map 需要转化的参数集合 * @return 加密过后的body对象 */ public RequestBody getRequestBody(Map map){ RequestBody body = null; Gson gson = new Gson(); String json = gson.toJson(map); // String encString = null; try { // encString = DesUtils.encrypt(json); // body = RequestBody.create(MediaType.parse("application/json;charset=UTF-8"), encString); body = RequestBody.create(MediaType.parse("application/json;charset=UTF-8"), json); // Log.e("111", "加密前 == getRequestBody: " + json); // Log.e("111", "加密后 == getRequestBody: " + encString); } catch (Exception e) { e.printStackTrace(); } return body; } }
现在基本工作已经完成。那么接下来我们来看看具体的使用
final RequestBody requestBody= RequestBodyUtil.getInstance().getRequestBody(map); Call<BaseEntity<String>> call= RetrofitFactory.getInstance().getRetrofit().create(ApiService.class).bannerCall(requestBody); call.enqueue(new RetrofitResultCallBack<BaseEntity<String>>() { @Override public void onSuccess(Object object) { listener.onSuccess(object); } @Override public void onFail(String message) { ToastUtils.showToast(message); } });这是一个简单的使用 ,希望能给给你提供帮组,由于不是很会写博客,写的不是很好,不过这些东西复制下来都是可以直接使用的 这种写法,比较适合使用mvp模式,关于mvp模式的构建,网上例子一大堆,这里我就不继续阐述了