REST:即 Representational State Transfer。(资源)表现层状态转化。是目前最流行的一种互联网软件架构。它结构清晰、符合标准、易于理解、扩展方便, 所以正得到越来越多网站的采用。使用 REST 风格的请求方式,可以简化 url,达到使用同一个 url 不同请求方式来执行不同的方法。
REST 风格的请求方式分别对应了以下四种请求,这四种请求有分别对应了四种对资源的操作:
GET -----------> 获取资源
POST ---------> 新建资源
PUT -----------> 更新资源
DELETE ------> 删除资源
GET
GET 请求我们都很熟悉了,比如地址栏直接访问,超链接访问等。
我们创建一个控制器用来接收 GET 请求:
package com.pudding.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
@Controller
public class RestController {
@RequestMapping(value = "/rest/{id}", method = RequestMethod.GET)
public String get(@PathVariable Integer id) {
System.out.println("GET --- 查询数据 --- " + id);
return "success";
}
}
并且使用一个超链接来发出 GET 请求:
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Rest风格请求</title>
</head>
<body>
<a href="rest/1">GET 请求</a>
</body>
</html>
访问页面,点击页面上的超链接,会发现画面跳转到了 success.jsp 并且在控制台上输出了 "GET --- 查询数据 --- 1" 。说明控制器成功的接收到了 GET 请求并且获取到了 url 地址中的参数。
POST
POST 请求最常见的方式就是 form 表单了。
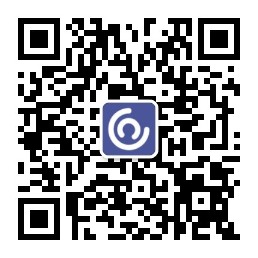
我们创建一个控制器来接收 POST 请求:
package com.pudding.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
@Controller
public class RestController {
@RequestMapping(value = "/rest", method = RequestMethod.POST)
public String post() {
// 接收表单中的各种信息
System.out.println("POST --- 创建数据");
return "success";
}
}
并且使用一个 form 表单来发出 POST 请求:
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<form action="rest" method="post">
<input type="submit" value="POST 请求" />
</form>
</body>
</html>
访问页面,点击页面上表单中的按钮,会发现画面跳转到了 success.jsp 并且在控制台上输出了 "POST --- 创建数据" 。说明控制器成功的接收到了 GET 请求。
PUT 和 DELETE
创建 GET 请求和创建 PUT 请求都很简单,但是 PUT 请求和 DELETE 请求呢?在我们正常的访问当中是创建不出 PUT 请求和 DELETE 请求的,所以我们需要使用 Spring 三大组件之一的过滤器Filter
来发出 PUT 请求和 DELTE 请求。
发送 PUT 和 DELTE 请求的步骤:
- 在 web.xml 中添加
HiddenHttpMethodFilter
过滤器:
<filter>
<filter-name>HiddenHttpMethodFilter</filter-name>
<filter-class>org.springframework.web.filter.HiddenHttpMethodFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>HiddenHttpMethodFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
- 创建一个带 POST 请求的 form 表单:
<form action="rest" method="post">
<input type="submit" value="PUT 请求" />
</form>
- 在表单内添加 name 为 _method 的标签:
<form action="rest" method="post">
<input type="hidden" name="_method" value="put" />
<input type="submit" value="PUT 请求" />
</form>
DELTE 请求同理,只需要将 put 求改为 delte 即可。不区分大小写。
如果按照以上步骤处理完成之后,点击按钮发现 HTTP 405 的错误提示:"消息 JSP 只允许 GET、POST 或 HEAD。Jasper 还允许 OPTIONS"。那么请参 HTTP 405 的错误提示:消息 JSP 只允许 GET、POST 或 HEAD。Jasper 还允许 OPTIONS 的解决方法
章节。