Android开发的猜拳游戏: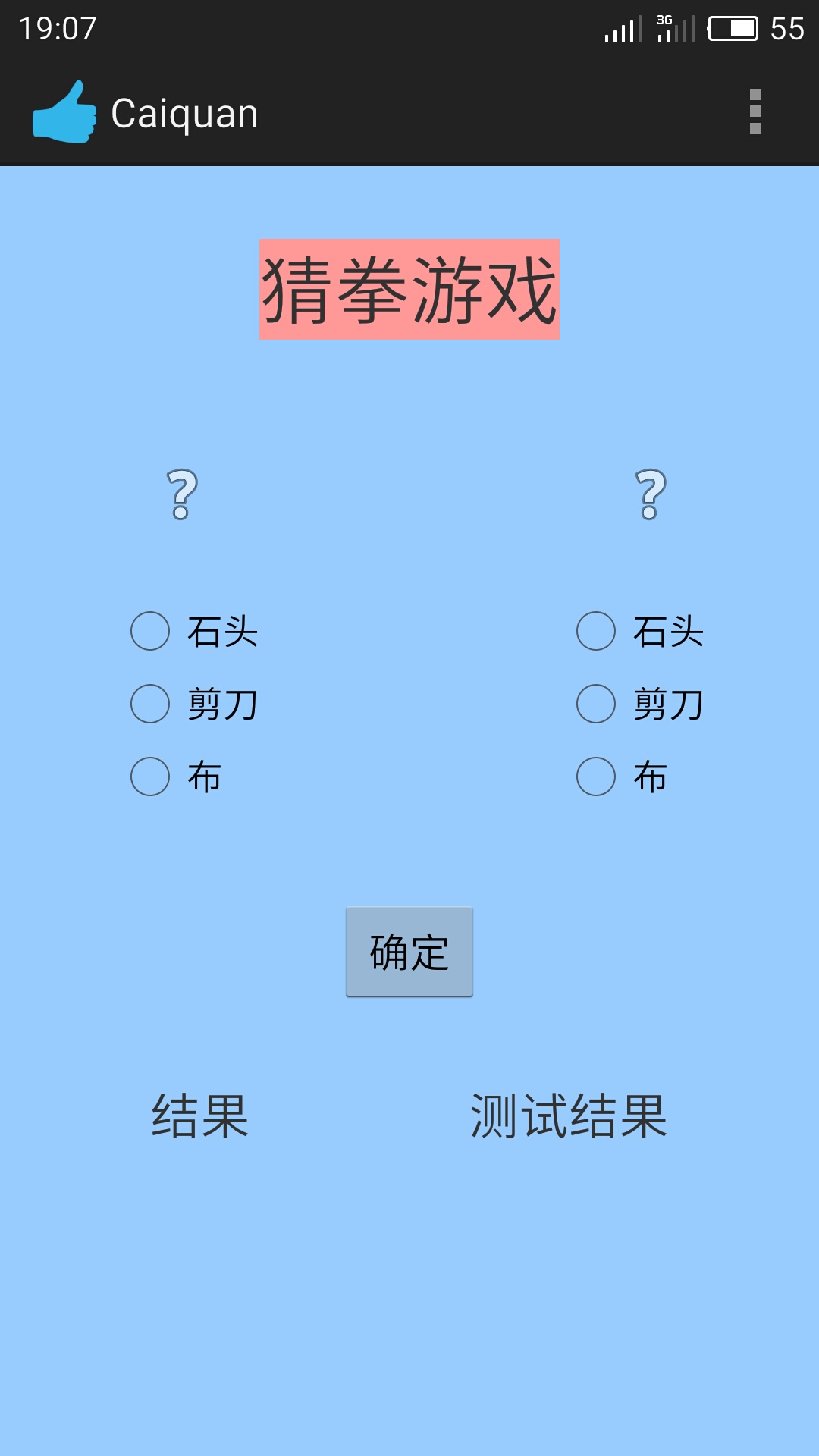
1、XML实现的主UI界面代码
在这里也可以用线性布局来实现,总体为水平线性布局,中间的部分用垂直线性布局
后面两个按钮也可以用垂直线性布局!
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/qianlan"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_vertical_margin"
android:paddingRight="@dimen/activity_vertical_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.Jackson.game.Caiquan" >
<TextView
android:id="@+id/game"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="16dp"
android:background="@color/yellow"
android:text="猜拳游戏"
android:textAlignment="center"
android:textSize="33sp"
tools:ignore="HardcodedText" />
<RadioGroup
android:id="@+id/radioGroupId1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="12dp"
android:layout_below="@+id/imageView1"
android:layout_marginTop="45dp"
android:orientation="vertical" >
<RadioButton
android:id="@+id/radioButton5"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="剪刀" />
<RadioButton
android:id="@+id/radioButton1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_above="@+id/radioButton5"
android:text="石头" />
<RadioButton
android:id="@+id/radioButton3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/radioButton5"
android:text="布" />
</RadioGroup>
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:layout_marginBottom="70dp"
android:text="结果 测试结果"
android:textSize="22sp" />
<Button
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_above="@+id/textView1"
android:layout_marginBottom="36dp"
android:layout_toRightOf="@+id/imageView1"
android:text="确定" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignBaseline="@+id/button1"
android:layout_alignBottom="@+id/button1"
android:layout_alignRight="@+id/game"
android:text="取消" />
<RadioGroup
android:id="@+id/radioGroupId"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/imageView2"
android:layout_alignStart="@+id/imageView2"
android:layout_alignTop="@+id/radioGroupId1"
android:orientation="vertical" >
<RadioButton
android:id="@+id/radioButton6"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignStart="@+id/radioButton5"
android:text="布" />
<RadioButton
android:id="@+id/radioButton2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_above="@+id/radioButton6"
android:layout_alignRight="@+id/radioGroupId"
android:layout_toStartOf="@+id/game"
android:text="剪刀" />
<RadioButton
android:id="@+id/radioButton4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_above="@+id/radioButton2"
android:layout_alignLeft="@+id/radioButton2"
android:text="石头" />
</RadioGroup>
<ImageView
android:id="@+id/imageView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/game"
android:layout_marginEnd="38dp"
android:layout_marginTop="19dp"
android:layout_toLeftOf="@+id/game"
android:layout_toStartOf="@+id/game"
android:src="@android:drawable/ic_menu_help" />
<ImageView
android:id="@+id/imageView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignTop="@+id/imageView1"
android:layout_marginLeft="31dp"
android:layout_marginTop="11dp"
android:layout_toRightOf="@+id/game"
android:src="@android:drawable/ic_menu_help" />
</RelativeLayout>
2、主界面的java代码
package com.Jackson.game;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.CompoundButton;
import android.widget.CompoundButton.OnCheckedChangeListener;
import android.widget.ImageView;
import android.widget.RadioButton;
import android.widget.RadioGroup;
import android.widget.TextView;
public class Caiquan extends Activity {
private RadioGroup radioGroup1,radioGroup;
private RadioButton shitouButton1,buButton1,jiandaoButton1;
private RadioButton jiandaoButton,buButton,shitouButton;
private Button button1,button2;
private TextView textview1,textview;
private ImageView imageview;
private ImageView imageview1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_caiquan);
textview1=(TextView)findViewById(R.id.textView1);
textview=(TextView)findViewById(R.id.game);
button1 = (Button) findViewById(R.id.button1);
button2 = (Button) findViewById(R.id.button2);
button1.setOnClickListener(new ButtonListener());
button2.setOnClickListener(new ButtonListener());
radioGroup=(RadioGroup)findViewById(R.id.radioGroupId);
radioGroup1=(RadioGroup)findViewById(R.id.radioGroupId1);
shitouButton1=(RadioButton)findViewById(R.id.radioButton1);
buButton1=(RadioButton)findViewById(R.id.radioButton3);
jiandaoButton1=(RadioButton)findViewById(R.id.radioButton5);
jiandaoButton=(RadioButton)findViewById(R.id.radioButton2);
shitouButton=(RadioButton)findViewById(R.id.radioButton4);
buButton=(RadioButton)findViewById(R.id.radioButton6);
RadioButtonListener Radiolistener = new RadioButtonListener();
shitouButton1.setOnCheckedChangeListener(Radiolistener);
buButton1.setOnCheckedChangeListener(Radiolistener);
jiandaoButton1.setOnCheckedChangeListener(Radiolistener);
jiandaoButton.setOnCheckedChangeListener(Radiolistener);
shitouButton.setOnCheckedChangeListener(Radiolistener);
buButton.setOnCheckedChangeListener(Radiolistener);
imageview1 = (ImageView) findViewById(R.id.imageView1);
imageview = (ImageView) findViewById(R.id.imageView2);
//在这里也可以为findViewById方法和绑定监听器设置两个方法
}
class RadioButtonListener implements OnCheckedChangeListener{
public void onCheckedChanged(CompoundButton buttonView,
boolean isChecked) {
System.out.println("is Checkede---->" +isChecked);
}
}
class ButtonListener implements OnClickListener{
@Override
public void onClick(View v) {
RadioButton radioButtonleft = (RadioButton) findViewById(radioGroup1
.getCheckedRadioButtonId());
String radioTextleft = radioButtonleft.getText().toString();
RadioButton radioButtonright = (RadioButton) findViewById(radioGroup
.getCheckedRadioButtonId());
String radioTextright = radioButtonright.getText().toString();
Intent intent= new Intent();
intent.setClass(Caiquan.this, Next.class);
//用radioText记录被选中的单选按钮的值,传达到下一个Activity
intent.putExtra("checkedleft", radioTextleft);
intent.putExtra("checkedright", radioTextright);
startActivity(intent);
}
}
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.caiquany, menu);
return true;
}
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return false;
}}
3、点击确定后跳转到的下一个界面
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="174dp"
android:text="TextView"
android:textSize="45sp"/>
<Button
android:id="@+id/button3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/textView1"
android:layout_centerHorizontal="true"
android:layout_marginTop="69dp"
android:text=" " />
</RelativeLayout>
4、下一个界面对应的java代码
package com.Jackson.game;
import android.app.Activity;
import android.content.DialogInterface;
import android.content.DialogInterface.OnClickListener;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class Next extends Activity{
private TextView textView;
private Button button;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.next);
button=(Button)findViewById(R.id.button3);
button.setOnClickListener((android.view.View.OnClickListener) new ButtonLisener());
textView=(TextView)findViewById(R.id.textView1);
}
class ButtonLisener implements OnClickListener{
@Override
public void onClick(DialogInterface dialog, int which) {
Intent intent = getIntent();
String checkedleft = intent.getStringExtra("checkedleft");
String checkedright = intent.getStringExtra("checkedright");
// 设置View为可见
textView.setVisibility(View.VISIBLE);
button.setVisibility(View.VISIBLE);
String msg = "左边:" + checkedleft + "\n" + "右边:" + checkedright
+ "\n";
if (checkedleft.equals(checkedright)) {
textView.setText(msg + "平局");
}
if (checkedright.equals("石头")) {
if (checkedleft.equals("剪刀")) { textView.setText(msg + "右边赢");}
else if (checkedleft.equals("布")) {textView.setText(msg + "左边赢"); }
}
if (checkedright.equals("剪刀")) {
if (checkedleft.equals("布")) {
textView.setText(msg + "右边赢");
} else if (checkedleft.equals("石头")) {
textView.setText(msg + "左边赢");
}
}
if (checkedright.equals("布"))
{
if (checkedleft.equals("石头")) {
textView.setText(msg + "右边赢");
}
else if (checkedleft.equals("剪刀")) {
textView.setText(msg + "左边赢");
}
}
}
}
}
猜拳游戏的基本思路
1、先设置好基本布局界面
2、思考猜拳的基本形式,怎样判断谁胜谁负?
3、怎样用java代码实现这种形式,要用到那些类?哪些方法?
下载资源请点击:Android 猜拳游戏0积分下载