本文翻译自:Get JavaScript object from array of objects by value of property [duplicate]
This question already has an answer here: 这个问题已经在这里有了答案:
Let's say I have an array of four objects: 假设我有四个对象组成的数组:
var jsObjects = [
{a: 1, b: 2},
{a: 3, b: 4},
{a: 5, b: 6},
{a: 7, b: 8}
];
Is there a way that I can get the third object ( {a: 5, b: 6}
) by the value of the property b
for example without a for...in
loop? 有没有一种方法可以通过属性b
的值获取第三个对象( {a: 5, b: 6}
),例如,没有for...in
循环?
#1楼
参考:https://stackoom.com/question/waiJ/通过属性值从对象数组中获取JavaScript对象-重复
#2楼
Filter
array of objects, which property matches value, returns array: Filter
对象数组,其属性与值匹配,返回数组:
var result = jsObjects.filter(obj => {
return obj.b === 6
})
See the MDN Docs on Array.prototype.filter() 请参阅Array.prototype.filter()上的MDN文档
const jsObjects = [ {a: 1, b: 2}, {a: 3, b: 4}, {a: 5, b: 6}, {a: 7, b: 8} ] let result = jsObjects.filter(obj => { return obj.b === 6 }) console.log(result)
Find
the value of the first element/object in the array, otherwise undefined
is returned. Find
数组中第一个元素/对象的值,否则返回undefined
。
var result = jsObjects.find(obj => {
return obj.b === 6
})
See the MDN Docs on Array.prototype.find() 请参阅Array.prototype.find()上的MDN文档
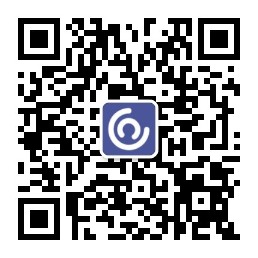
const jsObjects = [ {a: 1, b: 2}, {a: 3, b: 4}, {a: 5, b: 6}, {a: 7, b: 8} ] let result = jsObjects.find(obj => { return obj.b === 6 }) console.log(result)
#3楼
If I understand correctly, you want to find the object in the array whose b
property is 6
? 如果我理解正确,您想在b
属性为6
的数组中找到对象。
var found;
jsObjects.some(function (obj) {
if (obj.b === 6) {
found = obj;
return true;
}
});
Or if you were using underscore: 或者,如果您使用下划线:
var found = _.select(jsObjects, function (obj) {
return obj.b === 6;
});
#4楼
I don't know why you are against a for loop (presumably you meant a for loop, not specifically for..in), they are fast and easy to read. 我不知道您为什么反对for循环(大概是指for循环,而不是专门针对..in),它们快速且易于阅读。 Anyhow, here's some options. 无论如何,这里有一些选择。
For loop: 对于循环:
function getByValue(arr, value) {
for (var i=0, iLen=arr.length; i<iLen; i++) {
if (arr[i].b == value) return arr[i];
}
}
.filter 。过滤
function getByValue2(arr, value) {
var result = arr.filter(function(o){return o.b == value;} );
return result? result[0] : null; // or undefined
}
.forEach .for每个
function getByValue3(arr, value) {
var result = [];
arr.forEach(function(o){if (o.b == value) result.push(o);} );
return result? result[0] : null; // or undefined
}
If, on the other hand you really did mean for..in and want to find an object with any property with a value of 6, then you must use for..in unless you pass the names to check. 另一方面,如果您确实的确想使用..in并希望找到具有值为6的任何属性的对象,那么除非您通过名称进行检查,否则必须使用for..in。 eg 例如
function getByValue4(arr, value) {
var o;
for (var i=0, iLen=arr.length; i<iLen; i++) {
o = arr[i];
for (var p in o) {
if (o.hasOwnProperty(p) && o[p] == value) {
return o;
}
}
}
}
#5楼
It looks like in the ECMAScript 6 proposal there are the Array
methods find()
and findIndex()
. 看起来在ECMAScript 6提案中似乎有Array
方法find()
和findIndex()
。 MDN also offers polyfills which you can include to get the functionality of these across all browsers. MDN还提供了polyfill,您可以将其包括在内以在所有浏览器中获得其功能。
function isPrime(element, index, array) {
var start = 2;
while (start <= Math.sqrt(element)) {
if (element % start++ < 1) return false;
}
return (element > 1);
}
console.log( [4, 6, 8, 12].find(isPrime) ); // undefined, not found
console.log( [4, 5, 8, 12].find(isPrime) ); // 5
function isPrime(element, index, array) {
var start = 2;
while (start <= Math.sqrt(element)) {
if (element % start++ < 1) return false;
}
return (element > 1);
}
console.log( [4, 6, 8, 12].findIndex(isPrime) ); // -1, not found
console.log( [4, 6, 7, 12].findIndex(isPrime) ); // 2
#6楼
var jsObjects = [{a: 1, b: 2}, {a: 3, b: 4}, {a: 5, b: 6}, {a: 7, b: 8}];
to access the third object, use: jsObjects[2];
要访问第三个对象,请使用: jsObjects[2];
to access the third object b value, use: jsObjects[2].b;
要访问第三个对象b值,请使用: jsObjects[2].b;