字符串
package com.example.wxhy.util;
import java.util.ArrayList;
import java.util.List;
import java.util.UUID;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* 项目名称:
* 类名称:StringUtil.java
* 类描述:字符串工具类
* 创建人:huangchenyang
* 创建时间:2014-09-22 15:22
* 修改人:huangchenyang
* 修改时间:2014-09-22 15:22
* 修改备注:
*/
public class StringUtil
{
/**
* 判断字符串是否为空
*
* @param str
* @return
*/
public static Boolean isNullOrWhiteSpace(String str)
{
if (str == null || "".equals(str) || str.trim().length() == 0)
{
return true;
}
return false;
}
/**
* 返回字符串,如果为空和null返回空字符串
*
* @param str
* @return
*/
public static String getString(String str)
{
if (StringUtil.isNullOrWhiteSpace(str))
{
return "";
}
else
{
return str;
}
}
/**
* 两个字符串相加
*
* @param str1
* @param str2
* @return
*/
public static String appendString(String str1, String str2)
{
if (StringUtil.isNullOrWhiteSpace(str1))
{
str1 = "";
}
if (StringUtil.isNullOrWhiteSpace(str2))
{
str2 = "";
}
return str1 + str2;
}
/**
* 判断字符串的长度
*
* @param str
* @param minLength
* @param maxLength
* @return
*/
public static Boolean validateLength(String str, Integer minLength, Integer maxLength)
{
if (str.length() >= minLength && str.length() <= maxLength)
{
return true;
}
return false;
}
/**
* 判断字符串是否是数字(整数或浮点数)
*
* @param str
* @return
*/
public static Boolean isNum(String str)
{
Pattern pattern = Pattern.compile("^(-)?(([1-9]{1}\\d*)|([0]{1}))(\\.(\\d){1,2})?$");
Matcher matcher = pattern.matcher(str);
return matcher.matches();
}
/**
* 检查整数
*
* @param num
* @param type "0+":非负整数 "+":正整数 "-0":非正整数 "-":负整数 "":整数
* @return
*/
public static boolean checkNumber(String num, String type)
{
String eL = "";
if (type.equals("0+"))
{
eL = "^\\d+$";//非负整数
}
else if (type.equals("+"))
{
eL = "^\\d*[1-9]\\d*$";//正整数
}
else if (type.equals("-0"))
{
eL = "^((-\\d+)|(0+))$";//非正整数
}
else if (type.equals("-"))
{
eL = "^-\\d*[1-9]\\d*$";//负整数
}
else
{
eL = "^-?\\d+$";//整数
}
Pattern p = Pattern.compile(eL);
Matcher m = p.matcher(num);
boolean b = m.matches();
return b;
}
public static String object2String(Object value)
{
if (value == null)
{
return null;
}
return String.valueOf(value);
}
public static String getUUID()
{
return getGUID().replace("-","");
}
public static String getGUID()
{
return UUID.randomUUID().toString().toUpperCase();
}
/**
* 不带横线 长度32个字符
*
* @return
*/
public static String getUuidNoneHyphen()
{
return UUID.randomUUID().toString().toUpperCase().replace("-", "");
}
public static String removeSpecialChar(String value)
{
if (isNullOrWhiteSpace(value))
{
return null;
}
value = value.replace(" ", "");
value = value.replace(" ", "");
return value;
}
public static String[] toStrings(String srcString, String separator)
{
List<String> stringList = new ArrayList<String>();
if (!StringUtil.isNullOrWhiteSpace(srcString))
{
String[] temps = srcString.split(separator);
if (temps != null && temps.length > 0)
{
for (String str : temps)
{
if (StringUtil.isNullOrWhiteSpace(str))
{
continue;
}
stringList.add(str);
}
}
}
if (stringList.size() > 0)
{
String[] array = new String[stringList.size()];
return stringList.toArray(array);
}
else
{
return null;
}
}
}
日期时间`
package com.example.wxhy.util;
import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
/**
* 项目名称:DAGL
* 类名称:DateUtil.java
* 类描述:日期工具类
* 创建人:huangchenyang
* 创建时间:2014-09-22 15:22
* 修改人:leeyunjiao
* 修改时间:2014-09-22 15:22
* 修改备注:
*/
public class DateUtil
{
//showType 的取值:例如“yyyy年MM月dd日hh时mm分ss秒”,“yyyy-MM-dd HH:mm:ss” 等
public static Calendar cal = new GregorianCalendar();
public static int YEAR = cal.get(Calendar.YEAR);
public static int MONTH = cal.get(Calendar.MONTH) + 1;
public static int DAY_OF_MONTH = cal.get(Calendar.DAY_OF_MONTH);
public static int DAY_OF_WEEK = cal.get(Calendar.DAY_OF_WEEK) - 1;
public static Date getNowTime()
{
return new Date();
}
//以showType的格式,返回String类型的当前时间
public static String getNowTime(String showType)
{
Date date = new Date();
SimpleDateFormat sdf = new SimpleDateFormat(showType);
return sdf.format(date);
}
//获取昨天String
public static String getYesterdayToString(String showType){
Calendar cal=Calendar.getInstance();
cal.add(Calendar.DATE,-1);
Date d=cal.getTime();
SimpleDateFormat sp=new SimpleDateFormat(showType);
return sp.format(d);
}
public static String getNowLongTimeStringTs()
{
Date date = new Date();
SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMddHHmmssSSS");
return sdf.format(date);
}
public static String getNowDateString()
{
Date date = new Date();
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
return sdf.format(date);
}
public static String getNowDateTimeString()
{
Date date = new Date();
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
return sdf.format(date);
}
public static String getNowDateTimeString(String showType)
{
Date date = new Date();
DateFormat df = new SimpleDateFormat(showType);
return df.format(date);
}
//将date按照showType转换成string
public static String turnDateToString(Date dDate, String showType)
{
DateFormat df = new SimpleDateFormat(showType);
return df.format(dDate);
}
public static String turnDateToString(Date dDate)
{
return turnDateToString(dDate, "yyyy-MM-dd HH:mm:ss");
}
public static String turnDateToStringNYR(Date dDate)
{
return turnDateToString(dDate, "yyyy-MM-dd");
}
//返回将string转换成date类型
public static Date turnStringToDate(String sDate, String showType)
{
//sDate的格式要和showType相符
DateFormat df = new SimpleDateFormat(showType);
Date date = null;
try
{
date = df.parse(sDate);
}
catch (ParseException e)
{
System.out.println("格式不一致,返回null");
}
return date;
}
public static Date turnStringToDate(String sDate)
{
return turnStringToDate(sDate, "yyyy-MM-dd HH:mm:ss");
}
//获得时间差
public static boolean getTimeDifference(Long timeBegin, Long timeEnd, Long timeStanding)
{
//大于指定时间返回true,否则返回false
Long difference = timeEnd - timeBegin;
if (difference >= timeStanding)
{
return true;
}
else
{
return false;
}
}
//获得时间差
public static boolean getTimeDifference(Date timeBegin, Date timeEnd, Long timeStanding)
{
return getTimeDifference(timeBegin.getTime(), timeEnd.getTime(), timeStanding);
}
//计算两个日期相隔的天数
public static int getTimeDifferenceForDays(Date firstDate, Date secondDate)
{
int nDay = (int) ((firstDate.getTime() - secondDate.getTime()) / (24 * 60 * 60 * 1000));
return nDay;
}
//计算两个日期相隔的天数
public static int getTimeDifferenceForSeconds(Date firstDate, Date secondDate)
{
int nDay = (int) ((firstDate.getTime() - secondDate.getTime()) / 1000);
return nDay;
}
/**
* 判断当前时间是否在[startTime, endTime]区间,注意时间格式要一致
*
* @param nowTime 当前时间
* @param startTime 开始时间
* @param endTime 结束时间
* @return
*/
public static boolean isBetweenDate(Date nowTime, Date startTime, Date endTime)
{
if (nowTime.getTime() == startTime.getTime()
|| nowTime.getTime() == endTime.getTime())
{
return true;
}
Calendar date = Calendar.getInstance();
date.setTime(nowTime);
Calendar begin = Calendar.getInstance();
begin.setTime(startTime);
Calendar end = Calendar.getInstance();
end.setTime(endTime);
if (date.after(begin) && date.before(end))
{
return true;
}
else
{
return false;
}
}
/**
* 获取一年中的第几周
* 时间格式'yyyy-MM-dd'
*
* @param sDate
* @return
*/
public static int getWeek(String sDate)
{
int year = new Integer(sDate.substring(0, 4));
int month = new Integer(sDate.substring(5, 7)) - 1;
int day = new Integer(sDate.substring(8, 10));
Calendar cal = Calendar.getInstance();
cal.set(Calendar.YEAR, year);
cal.set(Calendar.MONTH, month);
cal.set(Calendar.DAY_OF_MONTH, day);
int weekno = cal.get(Calendar.WEEK_OF_YEAR);
return weekno;
}
/**
* 获取一年中的第几季度
* 时间格式'yyyy-MM-dd'
*
* @param sDate
* @return
*/
public static int getQuarter(String sDate)
{
int month = new Integer(sDate.substring(5, 7));
switch (month)
{
case 1:
return 1;
case 2:
return 1;
case 3:
return 1;
case 4:
return 2;
case 5:
return 2;
case 6:
return 2;
case 7:
return 3;
case 8:
return 3;
case 9:
return 3;
case 10:
return 4;
case 11:
return 4;
case 12:
return 4;
default:
return 1;
}
}
/**
* 获取半年,上半年0,下半年1
* 时间格式'yyyy-MM-dd'
*
* @param sDate
* @return
*/
public static int getHYear(String sDate)
{
int month = new Integer(sDate.substring(5, 7));
if (month >= 1 && month <= 6)
{
return 0;
}
else
{
return 1;
}
}
public static String getYesterday(String sDate)
{
if (sDate.equals(""))
{
sDate = getNowTime("yyyy-MM-dd");
}
int year = new Integer(sDate.substring(0, 4));
int month = new Integer(sDate.substring(5, 7)) - 1;
int day = new Integer(sDate.substring(8, 10));
Calendar cal = Calendar.getInstance();
cal.set(Calendar.YEAR, year);
cal.set(Calendar.MONTH, month);
cal.set(Calendar.DAY_OF_MONTH, day - 1);
return DateUtil.turnDateToString(cal.getTime(), "yyyy-MM-dd");
}
public static String getTomorrow(String sDate)
{
if (sDate.equals(""))
{
sDate = getNowTime("yyyy-MM-dd");
}
int year = new Integer(sDate.substring(0, 4));
int month = new Integer(sDate.substring(5, 7)) - 1;
int day = new Integer(sDate.substring(8, 10));
Calendar cal = Calendar.getInstance();
cal.set(Calendar.YEAR, year);
cal.set(Calendar.MONTH, month);
cal.set(Calendar.DAY_OF_MONTH, day + 1);
return DateUtil.turnDateToString(cal.getTime(), "yyyy-MM-dd");
}
public static String getOneWeekAgo(String sDate)
{
if (sDate.equals(""))
{
sDate = getNowTime("yyyy-MM-dd");
}
int year = new Integer(sDate.substring(0, 4));
int month = new Integer(sDate.substring(5, 7)) - 1;
int day = new Integer(sDate.substring(8, 10));
Calendar cal = Calendar.getInstance();
cal.set(Calendar.YEAR, year);
cal.set(Calendar.MONTH, month);
cal.set(Calendar.DAY_OF_MONTH, day - 7);
return DateUtil.turnDateToString(cal.getTime(), "yyyy-MM-dd");
}
public static String getOneMonthAgo(String sDate)
{
if (sDate.equals(""))
{
sDate = getNowTime("yyyy-MM-dd");
}
int year = new Integer(sDate.substring(0, 4));
int month = new Integer(sDate.substring(5, 7)) - 1;
int day = new Integer(sDate.substring(8, 10));
Calendar cal = Calendar.getInstance();
cal.set(Calendar.YEAR, year);
cal.set(Calendar.MONTH, month - 1);
cal.set(Calendar.DAY_OF_MONTH, day);
return DateUtil.turnDateToString(cal.getTime(), "yyyy-MM-dd");
}
public static String getMonthChange(Date date, int months, String format)
{
Calendar cal = Calendar.getInstance();
cal.setTime(date == null ? new Date() : date);
cal.add(Calendar.MONTH, months);
return DateUtil.turnDateToString(cal.getTime(), format == null || format.equals("") ? "yyyy-MM-dd" : format);
}
public static String getSomeDaysAgo(String sDate, int days)
{
if (sDate.equals(""))
{
sDate = getNowTime("yyyy-MM-dd");
}
int year = new Integer(sDate.substring(0, 4));
int month = new Integer(sDate.substring(5, 7)) - 1;
int day = new Integer(sDate.substring(8, 10));
Calendar cal = Calendar.getInstance();
cal.set(Calendar.YEAR, year);
cal.set(Calendar.MONTH, month);
cal.set(Calendar.DAY_OF_MONTH, day - days);
return DateUtil.turnDateToString(cal.getTime(), "yyyy-MM-dd");
}
public static String getSomeDaysAfter(String sDate, int days)
{
if (sDate.equals(""))
{
sDate = getNowTime("yyyy-MM-dd");
}
int year = new Integer(sDate.substring(0, 4));
int month = new Integer(sDate.substring(5, 7)) - 1;
int day = new Integer(sDate.substring(8, 10));
Calendar cal = Calendar.getInstance();
cal.set(Calendar.YEAR, year);
cal.set(Calendar.MONTH, month);
cal.set(Calendar.DAY_OF_MONTH, day + days);
return DateUtil.turnDateToString(cal.getTime(), "yyyy-MM-dd");
}
public static String getSomeSecondsAgo(String sDate, int seconds)
{
if (sDate.equals(""))
{
sDate = getNowTime("yyyy-MM-dd HH:mm:ss");
}
int year = new Integer(sDate.substring(0, 4));
int month = new Integer(sDate.substring(5, 7)) - 1;
int day = new Integer(sDate.substring(8, 10));
int hour = new Integer(sDate.substring(11, 13));
int minute = new Integer(sDate.substring(14, 16));
int second = new Integer(sDate.substring(17, 19));
Calendar cal = Calendar.getInstance();
cal.set(Calendar.YEAR, year);
cal.set(Calendar.MONTH, month);
cal.set(Calendar.DAY_OF_MONTH, day);
cal.set(Calendar.HOUR_OF_DAY, hour);
cal.set(Calendar.MINUTE, minute);
cal.set(Calendar.SECOND, second - seconds);
return DateUtil.turnDateToString(cal.getTime(), "yyyy-MM-dd HH:mm:ss");
}
public static String getSomeSecondsAfter(String sDate, int seconds)
{
if (sDate.equals(""))
{
sDate = getNowTime("yyyy-MM-dd HH:mm:ss");
}
int year = new Integer(sDate.substring(0, 4));
int month = new Integer(sDate.substring(5, 7)) - 1;
int day = new Integer(sDate.substring(8, 10));
int hour = new Integer(sDate.substring(11, 13));
int minute = new Integer(sDate.substring(14, 16));
int second = new Integer(sDate.substring(17, 19));
//logger.debug("year:"+year+" month:"+month+" day:"+day+" hour:"+hour+" minute:"+minute+" second:"+second);
Calendar cal = Calendar.getInstance();
cal.set(Calendar.YEAR, year);
cal.set(Calendar.MONTH, month);
cal.set(Calendar.DAY_OF_MONTH, day);
cal.set(Calendar.HOUR_OF_DAY, hour);
cal.set(Calendar.MINUTE, minute);
cal.set(Calendar.SECOND, second + seconds);
return DateUtil.turnDateToString(cal.getTime(), "yyyy-MM-dd HH:mm:ss");
}
/**
* 获取指定日期后几天的日期
*
* @param date
* @param days
* @return
*/
public static Date getAfterDate(Date date, int days)
{
Calendar calendar = Calendar.getInstance();
calendar.setTime(date);
calendar.set(Calendar.DAY_OF_YEAR, calendar.get(Calendar.DAY_OF_YEAR) + days);
return calendar.getTime();
}
/**
* 获取指定日期前几天的日期
*
* @param date
* @param days
* @return
*/
public static Date getBeforeDate(Date date, int days)
{
Calendar calendar = Calendar.getInstance();
calendar.setTime(date);
calendar.set(Calendar.DAY_OF_YEAR, calendar.get(Calendar.DAY_OF_YEAR) - days);
return calendar.getTime();
}
/**
* 取得当前日期所在周的第一天
*
* @param date
* @return
*/
public static Date getFirstDayOfWeek(Date date)
{
Calendar calendar = Calendar.getInstance();
calendar.setFirstDayOfWeek(Calendar.SUNDAY);
calendar.setTime(date);
// Sunday
calendar.set(Calendar.DAY_OF_WEEK, calendar.getFirstDayOfWeek());
return calendar.getTime();
}
/**
* 取得当前日期所在周的最后一天
*
* @param date
* @return
*/
public static Date getLastDayOfWeek(Date date)
{
Calendar calendar = Calendar.getInstance();
calendar.setFirstDayOfWeek(Calendar.SUNDAY);
calendar.setTime(date);
// Saturday
calendar.set(Calendar.DAY_OF_WEEK, calendar.getFirstDayOfWeek() + 6);
return calendar.getTime();
}
/**
* 返回指定日期的月的第一天
*
* @param date
* @return
*/
public static Date getFirstDayOfMonth(Date date)
{
Calendar calendar = Calendar.getInstance();
calendar.setTime(date);
calendar.set(calendar.get(Calendar.YEAR), calendar.get(Calendar.MONTH), 1);
return calendar.getTime();
}
/**
* 返回指定日期的月的最后一天
*
* @param date
* @return
*/
public static Date getLastDayOfMonth(Date date)
{
Calendar calendar = Calendar.getInstance();
calendar.setTime(date);
calendar.set(calendar.get(Calendar.YEAR), calendar.get(Calendar.MONTH), 1);
calendar.roll(Calendar.DATE, -1);
return calendar.getTime();
}
/**
* 返回指定日期的季的第一天
*
* @param date
* @return
*/
public static Date getFirstDayOfQuarter(Date date)
{
Calendar calendar = Calendar.getInstance();
calendar.setTime(date);
return getFirstDayOfQuarter(calendar.get(Calendar.YEAR), getQuarterOfYear(date));
}
/**
* 返回指定日期的季的最后一天
*
* @param date
* @return
*/
public static Date getLastDayOfQuarter(Date date)
{
Calendar calendar = Calendar.getInstance();
calendar.setTime(date);
return getLastDayOfQuarter(calendar.get(Calendar.YEAR), getQuarterOfYear(date));
}
/**
* 返回指定年月的月的第一天
*
* @param year
* @param month
* @return
*/
public static Date getFirstDayOfMonth(Integer year, Integer month)
{
Calendar calendar = Calendar.getInstance();
if (year == null)
{
year = calendar.get(Calendar.YEAR);
}
if (month == null)
{
month = calendar.get(Calendar.MONTH);
}
calendar.set(year, month, 1);
return calendar.getTime();
}
/**
* 返回指定年月的月的最后一天
*
* @param year
* @param month
* @return
*/
public static Date getLastDayOfMonth(Integer year, Integer month)
{
Calendar calendar = Calendar.getInstance();
if (year == null)
{
year = calendar.get(Calendar.YEAR);
}
if (month == null)
{
month = calendar.get(Calendar.MONTH);
}
calendar.set(year, month, 1);
calendar.roll(Calendar.DATE, -1);
return calendar.getTime();
}
/**
* 返回指定月的日期
*
* @param date
* @param month,负数之前,正数之后
* @return
*/
public static Date getDateBeforeMonth(Date date, Integer month)
{
Calendar calendar = Calendar.getInstance();
calendar.setTime(date);
//-1表示前一个月等
calendar.add(Calendar.MONTH, month);
return calendar.getTime();
}
/**
* 返回指定年季的季的第一天
*
* @param year
* @param quarter
* @return
*/
public static Date getFirstDayOfQuarter(Integer year, Integer quarter)
{
Calendar calendar = Calendar.getInstance();
Integer month = new Integer(0);
if (quarter == 1)
{
month = 1 - 1;
}
else if (quarter == 2)
{
month = 4 - 1;
}
else if (quarter == 3)
{
month = 7 - 1;
}
else if (quarter == 4)
{
month = 10 - 1;
}
else
{
month = calendar.get(Calendar.MONTH);
}
return getFirstDayOfMonth(year, month);
}
/**
* 返回指定年季的季的最后一天
*
* @param year
* @param quarter
* @return
*/
public static Date getLastDayOfQuarter(Integer year, Integer quarter)
{
Calendar calendar = Calendar.getInstance();
Integer month = new Integer(0);
if (quarter == 1)
{
month = 3 - 1;
}
else if (quarter == 2)
{
month = 6 - 1;
}
else if (quarter == 3)
{
month = 9 - 1;
}
else if (quarter == 4)
{
month = 12 - 1;
}
else
{
month = calendar.get(Calendar.MONTH);
}
return getLastDayOfMonth(year, month);
}
/**
* 返回指定年季的上一季的最后一天
*
* @param year
* @param quarter
* @return
*/
public static Date getLastDayOfLastQuarter(Integer year, Integer quarter)
{
Calendar calendar = Calendar.getInstance();
Integer month = new Integer(0);
if (quarter == 1)
{
month = 12 - 1;
}
else if (quarter == 2)
{
month = 3 - 1;
}
else if (quarter == 3)
{
month = 6 - 1;
}
else if (quarter == 4)
{
month = 9 - 1;
}
else
{
month = calendar.get(Calendar.MONTH);
}
return getLastDayOfMonth(year, month);
}
/**
* 返回指定日期的季度
*
* @param date
* @return
*/
public static int getQuarterOfYear(Date date)
{
Calendar calendar = Calendar.getInstance();
calendar.setTime(date);
return calendar.get(Calendar.MONTH) / 3 + 1;
}
/**
* 把日期和时间组合成一个日期完整形式
*
* @param date yyyy-MM-dd
* @param time HH:mm:ss
* @return 拼接好的日期形式
*/
public static Date getDateTime(Date date, Date time)
{
SimpleDateFormat sdfD = new SimpleDateFormat("yyyy-MM-dd");
SimpleDateFormat sdfT = new SimpleDateFormat("HH:mm:ss");
DateFormat df = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
String formatDate = sdfD.format(date);
String formatTime = sdfT.format(time);
try
{
Date parse = df.parse(formatDate + " " + formatTime);
return parse;
}
catch (ParseException e)
{
e.printStackTrace();
}
return null;
}
/**
* 格式化所有格式的日期字符串
*
* @param dateString
* @return
*/
public static String getAllFormatDate(String dateString)
{
if (StringUtil.isNullOrWhiteSpace(dateString))
{
return null;
}
if (dateString.indexOf("/") > 0)
{
dateString.replace("/", "-");
}
if (dateString.indexOf(".") > 0)
{
dateString.replace(".", "-");
}
int year = 0;
int month = 0;
int day = 0;
String[] strs = dateString.split("-");
if (strs.length != 3)
{
return null;
}
try
{
year = Integer.parseInt(strs[0]);
month = Integer.parseInt(strs[1]);
day = Integer.parseInt(strs[2]);
}
catch (Exception ex)
{
}
if (year < 1900 || year > 2050)
{
return null;
}
if (month < 1 || month > 12)
{
return null;
}
if (day < 1 || day > 31)
{
return null;
}
String formatDate = String.format("%d-%02d-%02d", year, month, day);
return formatDate;
}
public static void main(String[] args)
{
System.out.println(getMonthChange(null, -12, "yyyy-M-d H:m:s"));
}
//计算两个日期相隔的分钟
public static int getTimeDifferenceFormins(Date firstDate, Date secondDate)
{
int nDay = (int) ((firstDate.getTime() - secondDate.getTime()) / (60 * 1000));
return nDay;
}
}
json
package com.example.wxhy.util;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.serializer.SerializerFeature;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
/**
* 项目名称:DAGL
* 类 名 称 :JsonUtil.java
* 类 描 述 :
* 创 建 人 :huangchenyang
* 创建时间:2015-12-08 10:18:00
* 修 改 人 :huangchenyang
* 修改时间:
* 修改备注:
* 文件说明:
* 文件版本:
*/
public class JsonUtil
{
public static Map<String, Object> json2Map(String json)
{
return (Map) JSON.parse(json);
}
public static final <T> T json2Object(String json, Class<T> c)
{
return (T) JSON.parseObject(json, c);
}
public static String warpJson2ListJson(String json)
{
String jsonStr = json;
if ((!StringUtil.isNullOrWhiteSpace(json)) && (!json.startsWith("[")))
{
jsonStr = "[" + json + "]";
}
return jsonStr;
}
public static final <T> List<T> json2List(String json, Class<T> c)
{
String jsonStr = json;
if (!StringUtil.isNullOrWhiteSpace(json))
{
if (!json.startsWith("["))
{
jsonStr = "[" + json + "]";
}
return JSON.parseArray(jsonStr, c);
}
return new ArrayList<>();
}
public static final String object2Json(Object entity)
{
return JSON.toJSONString(entity, SerializerFeature.WriteNonStringValueAsString);
}
public static final String list2Json(List list)
{
return JSON.toJSONString(list);
}
public static final String map2Json(Map<String, Object> map)
{
return JSON.toJSONString(map);
}
}
一个get请求的工具,虽然有点老但是很好用,忘了是哪位写的了
package com.example.wxhy.util;
import java.io.IOException;
import net.sf.json.JSONObject;
import org.apache.http.HttpEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
public class HttpClientUtil {
public static JSONObject doGetJson(String url){
JSONObject jsonObject = null;
CloseableHttpClient client = HttpClients.createDefault();
HttpGet httpGet = new HttpGet(url);
try {
CloseableHttpResponse response = client.execute(httpGet);
HttpEntity entity = response.getEntity();
if(entity != null){
String result = EntityUtils.toString(entity,"UTF-8");
jsonObject = JSONObject.fromObject(result);
}
httpGet.releaseConnection();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
client.close();
} catch (IOException e) {
e.printStackTrace();
}
}
return jsonObject;
}
}
ftp
扫描二维码关注公众号,回复:
10783247 查看本文章
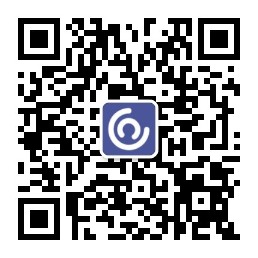
package com.szsxd.dagl.utils;
import org.apache.commons.net.ftp.FTPClient;
import org.apache.commons.net.ftp.FTPFile;
import org.apache.commons.net.ftp.FTPReply;
import java.io.*;
import java.net.MalformedURLException;
public class FtpUtil
{
//ftp服务器地址
public static String hostname = "192.168.0.107";
//ftp服务器端口号默认为21
public static Integer port = 21 ;
//ftp登录账号
public static String username = "ftp";
//ftp登录密码
public static String password = "123456";
public static FTPClient ftpClient = null;
/**
* 初始化ftp服务器
*/
public static void initFtpClient() {
ftpClient = new FTPClient();
ftpClient.setControlEncoding("utf-8");
try {
System.out.println("connecting...ftp服务器:"+hostname+":"+port);
ftpClient.connect(hostname, port); //连接ftp服务器
ftpClient.login(username, password); //登录ftp服务器
int replyCode = ftpClient.getReplyCode(); //是否成功登录服务器
if(!FTPReply.isPositiveCompletion(replyCode)){
System.out.println("connect failed...ftp服务器:"+hostname+":"+port);
}
System.out.println("connect successfu...ftp服务器:"+hostname+":"+port);
}catch (MalformedURLException e) {
e.printStackTrace();
}catch (IOException e) {
e.printStackTrace();
}
}
/**
* 上传文件
* @param pathname ftp服务保存地址
* @param fileName 上传到ftp的文件名
* @param originfilename 待上传文件的名称(绝对地址) *
* @return
*/
public static boolean uploadFile( String pathname, String fileName,String originfilename){
boolean flag = false;
InputStream inputStream = null;
try{
System.out.println("开始上传文件");
inputStream = new FileInputStream(new File(originfilename));
initFtpClient();
ftpClient.setFileType(ftpClient.BINARY_FILE_TYPE);
CreateDirecroty(pathname);
ftpClient.makeDirectory(pathname);
ftpClient.changeWorkingDirectory(pathname);
ftpClient.storeFile(fileName, inputStream);
inputStream.close();
ftpClient.logout();
flag = true;
System.out.println("上传文件成功");
}catch (Exception e) {
System.out.println("上传文件失败");
e.printStackTrace();
}finally{
if(ftpClient.isConnected()){
try{
ftpClient.disconnect();
}catch(IOException e){
e.printStackTrace();
}
}
if(null != inputStream){
try {
inputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
return true;
}
/**
* 上传文件
* @param pathname ftp服务保存地址
* @param fileName 上传到ftp的文件名
* @param inputStream 输入文件流
* @return
*/
public static boolean uploadFileInput( String pathname, String fileName,InputStream inputStream){
boolean flag = false;
try{
System.out.println("开始上传文件");
initFtpClient();
ftpClient.setFileType(ftpClient.BINARY_FILE_TYPE);
CreateDirecroty(pathname);
ftpClient.makeDirectory(pathname);
ftpClient.changeWorkingDirectory(pathname);
ftpClient.storeFile(fileName, inputStream);
inputStream.close();
ftpClient.logout();
flag = true;
System.out.println("上传文件成功");
}catch (Exception e) {
System.out.println("上传文件失败");
e.printStackTrace();
}finally{
if(ftpClient.isConnected()){
try{
ftpClient.disconnect();
}catch(IOException e){
e.printStackTrace();
}
}
if(null != inputStream){
try {
inputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
return true;
}
//改变目录路径
public static boolean changeWorkingDirectory(String directory) {
boolean flag = true;
try {
flag = ftpClient.changeWorkingDirectory(directory);
if (flag) {
System.out.println("进入文件夹" + directory + " 成功!");
} else {
System.out.println("进入文件夹" + directory + " 失败!开始创建文件夹");
}
} catch (IOException ioe) {
ioe.printStackTrace();
}
return flag;
}
//创建多层目录文件,如果有ftp服务器已存在该文件,则不创建,如果无,则创建
public static boolean CreateDirecroty(String remote) throws IOException {
boolean success = true;
String directory = remote + "/";
// 如果远程目录不存在,则递归创建远程服务器目录
if (!directory.equalsIgnoreCase("/") && !changeWorkingDirectory(new String(directory))) {
int start = 0;
int end = 0;
if (directory.startsWith("/")) {
start = 1;
} else {
start = 0;
}
end = directory.indexOf("/", start);
String path = "";
String paths = "";
while (true) {
String subDirectory = new String(remote.substring(start, end).getBytes("UTF-8"), "iso-8859-1");
path = path + "/" + subDirectory;
if (!existFile(path)) {
if (makeDirectory(subDirectory)) {
changeWorkingDirectory(subDirectory);
} else {
System.out.println("创建目录[" + subDirectory + "]失败");
changeWorkingDirectory(subDirectory);
}
} else {
changeWorkingDirectory(subDirectory);
}
paths = paths + "/" + subDirectory;
start = end + 1;
end = directory.indexOf("/", start);
// 检查所有目录是否创建完毕
if (end <= start) {
break;
}
}
}
return success;
}
//判断ftp服务器文件是否存在
public static boolean existFile(String path) throws IOException {
boolean flag = false;
FTPFile[] ftpFileArr = ftpClient.listFiles(path);
if (ftpFileArr.length > 0) {
flag = true;
}
return flag;
}
//创建目录
public static boolean makeDirectory(String dir) {
boolean flag = true;
try {
flag = ftpClient.makeDirectory(dir);
if (flag) {
System.out.println("创建文件夹" + dir + " 成功!");
} else {
System.out.println("创建文件夹" + dir + " 失败!");
}
} catch (Exception e) {
e.printStackTrace();
}
return flag;
}
/** * 下载文件 *
* @param pathname FTP服务器文件目录 *
* @param filename 文件名称 *
* @param localpath 下载后的文件路径 *
* @return */
public static boolean downloadFile(String pathname, String filename, String localpath){
boolean flag = false;
OutputStream os=null;
try {
System.out.println("开始下载文件");
initFtpClient();
//切换FTP目录
ftpClient.changeWorkingDirectory(pathname);
FTPFile[] ftpFiles = ftpClient.listFiles();
for(FTPFile file : ftpFiles){
if(filename.equalsIgnoreCase(file.getName())){
File localFile = new File(localpath + "/" + file.getName());
os = new FileOutputStream(localFile);
ftpClient.retrieveFile(file.getName(), os);
os.close();
}
}
ftpClient.logout();
flag = true;
System.out.println("下载文件成功");
} catch (Exception e) {
System.out.println("下载文件失败");
e.printStackTrace();
} finally{
if(ftpClient.isConnected()){
try{
ftpClient.disconnect();
}catch(IOException e){
e.printStackTrace();
}
}
if(null != os){
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
return flag;
}
/** * 删除文件 *
* @param pathname FTP服务器保存目录 *
* @param filename 要删除的文件名称 *
* @return */
public static boolean deleteFile(String pathname, String filename){
boolean flag = false;
try {
System.out.println("开始删除文件");
initFtpClient();
//切换FTP目录
ftpClient.changeWorkingDirectory(pathname);
ftpClient.dele(filename);
ftpClient.logout();
flag = true;
System.out.println("删除文件成功");
} catch (Exception e) {
System.out.println("删除文件失败");
e.printStackTrace();
} finally {
if(ftpClient.isConnected()){
try{
ftpClient.disconnect();
}catch(IOException e){
e.printStackTrace();
}
}
}
return flag;
}
public static void main(String[] args) {
FtpUtil ftp =new FtpUtil();
ftp.uploadFile("ftptest", "ftp1.txt", "E://ftp1.txt");
//ftp.downloadFile("ftptest", "ftp1.txt", "E://");
//ftp.deleteFile("", "ftptest");
System.out.println("ok");
}
}
二维码
package com.example.wxhy.util;
import com.example.wxhy.entity.QRcode.BufferedImageLuminanceSource;
import com.google.zxing.*;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.common.HybridBinarizer;
import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel;
import javax.imageio.ImageIO;
import java.awt.*;
import java.awt.geom.RoundRectangle2D;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.OutputStream;
import java.util.Hashtable;
import java.util.Random;
/**
* 二维码工具类
*
*/
public class QRCodeUtil {
private static final String CHARSET = "utf-8";
private static final String FORMAT_NAME = "JPG";
// 二维码尺寸
private static final int QRCODE_SIZE = 300;
// LOGO宽度
private static final int WIDTH = 60;
// LOGO高度
private static final int HEIGHT = 60;
private static BufferedImage createImage(String content, String imgPath,
boolean needCompress) throws Exception {
Hashtable<EncodeHintType, Object> hints = new Hashtable<EncodeHintType, Object>();
hints.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.H);
hints.put(EncodeHintType.CHARACTER_SET, CHARSET);
hints.put(EncodeHintType.MARGIN, 1);
BitMatrix bitMatrix = new MultiFormatWriter().encode(content,
BarcodeFormat.QR_CODE, QRCODE_SIZE, QRCODE_SIZE, hints);
int width = bitMatrix.getWidth();
int height = bitMatrix.getHeight();
BufferedImage image = new BufferedImage(width, height,
BufferedImage.TYPE_INT_RGB);
for (int x = 0; x < width; x++) {
for (int y = 0; y < height; y++) {
image.setRGB(x, y, bitMatrix.get(x, y) ? 0xFF000000
: 0xFFFFFFFF);
}
}
if (imgPath == null || "".equals(imgPath)) {
return image;
}
// 插入图片
QRCodeUtil.insertImage(image, imgPath, needCompress);
return image;
}
/**
* 插入LOGO
*
* @param source
* 二维码图片
* @param imgPath
* LOGO图片地址
* @param needCompress
* 是否压缩
* @throws Exception
*/
private static void insertImage(BufferedImage source, String imgPath,
boolean needCompress) throws Exception {
File file = new File(imgPath);
if (!file.exists()) {
System.err.println(""+imgPath+" 该文件不存在!");
return;
}
Image src = ImageIO.read(new File(imgPath));
int width = src.getWidth(null);
int height = src.getHeight(null);
if (needCompress) { // 压缩LOGO
if (width > WIDTH) {
width = WIDTH;
}
if (height > HEIGHT) {
height = HEIGHT;
}
Image image = src.getScaledInstance(width, height,
Image.SCALE_SMOOTH);
BufferedImage tag = new BufferedImage(width, height,
BufferedImage.TYPE_INT_RGB);
Graphics g = tag.getGraphics();
g.drawImage(image, 0, 0, null); // 绘制缩小后的图
g.dispose();
src = image;
}
// 插入LOGO
Graphics2D graph = source.createGraphics();
int x = (QRCODE_SIZE - width) / 2;
int y = (QRCODE_SIZE - height) / 2;
graph.drawImage(src, x, y, width, height, null);
Shape shape = new RoundRectangle2D.Float(x, y, width, width, 6, 6);
graph.setStroke(new BasicStroke(3f));
graph.draw(shape);
graph.dispose();
}
/**
* 生成二维码(内嵌LOGO)
*
* @param content
* 内容
* @param imgPath
* LOGO地址
* @param destPath
* 存放目录
* @param needCompress
* 是否压缩LOGO
* @throws Exception
*/
public static String encode(String content, String imgPath, String destPath,
boolean needCompress) throws Exception {
BufferedImage image = QRCodeUtil.createImage(content, imgPath,
needCompress);
mkdirs(destPath);
String file = new Random().nextInt(99999999)+".jpg";
ImageIO.write(image, FORMAT_NAME, new File(destPath+"/"+file));
return file;
}
/**
* 当文件夹不存在时,mkdirs会自动创建多层目录,区别于mkdir.(mkdir如果父目录不存在则会抛出异常)
* @author lanyuan
* Email: [email protected]
* @date 2013-12-11 上午10:16:36
* @param destPath 存放目录
*/
public static void mkdirs(String destPath) {
File file =new File(destPath);
//当文件夹不存在时,mkdirs会自动创建多层目录,区别于mkdir.(mkdir如果父目录不存在则会抛出异常)
if (!file.exists() && !file.isDirectory()) {
file.mkdirs();
}
}
/**
* 生成二维码(内嵌LOGO)
*
* @param content
* 内容
* @param imgPath
* LOGO地址
* @param destPath
* 存储地址
* @throws Exception
*/
public static void encode(String content, String imgPath, String destPath)
throws Exception {
QRCodeUtil.encode(content, imgPath, destPath, false);
}
/**
* 生成二维码
*
* @param content
* 内容
* @param destPath
* 存储地址
* @param needCompress
* 是否压缩LOGO
* @throws Exception
*/
public static void encode(String content, String destPath,
boolean needCompress) throws Exception {
QRCodeUtil.encode(content, null, destPath, needCompress);
}
/**
* 生成二维码
*
* @param content
* 内容
* @param destPath
* 存储地址
* @throws Exception
*/
public static void encode(String content, String destPath) throws Exception {
QRCodeUtil.encode(content, null, destPath, false);
}
/**
* 生成二维码(内嵌LOGO)
*
* @param content
* 内容
* @param imgPath
* LOGO地址
* @param output
* 输出流
* @param needCompress
* 是否压缩LOGO
* @throws Exception
*/
public static void encode(String content, String imgPath,
OutputStream output, boolean needCompress) throws Exception {
BufferedImage image = QRCodeUtil.createImage(content, imgPath,
needCompress);
ImageIO.write(image, FORMAT_NAME, output);
}
/**
* 生成二维码
*
* @param content
* 内容
* @param output
* 输出流
* @throws Exception
*/
public static void encode(String content, OutputStream output)
throws Exception {
QRCodeUtil.encode(content, null, output, false);
}
/**
* 解析二维码
*
* @param file
* 二维码图片
* @return
* @throws Exception
*/
public static String decode(File file) throws Exception {
BufferedImage image;
image = ImageIO.read(file);
if (image == null) {
return null;
}
BufferedImageLuminanceSource source = new BufferedImageLuminanceSource(
image);
BinaryBitmap bitmap = new BinaryBitmap(new HybridBinarizer(source));
Result result;
Hashtable<DecodeHintType, Object> hints = new Hashtable<DecodeHintType, Object>();
hints.put(DecodeHintType.CHARACTER_SET, CHARSET);
result = new MultiFormatReader().decode(bitmap, hints);
String resultStr = result.getText();
return resultStr;
}
/**
* 解析二维码
*
* @param path
* 二维码图片地址
* @return
* @throws Exception
*/
public static String decode(String path) throws Exception {
return QRCodeUtil.decode(new File(path));
}
// public static void main(String[] args) throws Exception {
// String text = "www.baidu.com";
// QRCodeUtil.encode(text, "D:/1.png", "D:/code/barcode", true);
// }
}
md5
/**
* 项目名称:museum
* 类 名 称 :Md5Utils.java
* 类 描 述 :
* 创 建 人 :leeyunjiao
* 创建时间:2018-01-11 15:00:00
* 修 改 人 :leeyunjiao
* 修改时间:
* 修改备注:
* 文件说明:
* 文件版本:
*/
package com.szsxd.dagl.utils;
import java.security.MessageDigest;
/**
* MD5加密工具(是基于hash算法实现,不可逆)
*/
public class Md5Utils
{
/**
* 16进制的字符数组
*/
private final static String[] hexDigits = {"0", "1", "2", "3", "4", "5", "6", "7", "8", "9", "a", "b", "c", "d", "e", "f"};
/**
* @param source 需要加密的原字符串
* @return
*/
public static String encode(String source)
{
return encode(source, "utf-8", false);
}
/**
* @param source 需要加密的原字符串
* @param encoding 指定编码类型
* @param uppercase 是否转为大写字符串
* @return
*/
public static String encode(String source, String encoding, boolean uppercase)
{
String result = null;
try
{
result = source;
// 获得MD5摘要对象
MessageDigest messageDigest = MessageDigest.getInstance("MD5");
// 使用指定的字节数组更新摘要信息
messageDigest.update(result.getBytes(encoding));
// messageDigest.digest()获得16位长度
result = byteArrayToHexString(messageDigest.digest());
}
catch (Exception e)
{
e.printStackTrace();
}
return uppercase ? result.toUpperCase() : result;
}
/**
* 转换字节数组为16进制字符串
*
* @param bytes 字节数组
* @return
*/
private static String byteArrayToHexString(byte[] bytes)
{
StringBuilder stringBuilder = new StringBuilder();
for (byte tem : bytes)
{
stringBuilder.append(byteToHexString(tem));
}
return stringBuilder.toString();
}
/**
* 转换byte到16进制
*
* @param b 要转换的byte
* @return 16进制对应的字符
*/
private static String byteToHexString(byte b)
{
int n = b;
if (n < 0)
{
n = 256 + n;
}
int d1 = n / 16;
int d2 = n % 16;
return hexDigits[d1] + hexDigits[d2];
}
}
http
package com.example.wxhy.util;
import org.apache.http.client.config.RequestConfig;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.impl.conn.PoolingHttpClientConnectionManager;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.nio.charset.Charset;
/**
* @author dataochen
* @Description
* @date: 2017/11/7 17:49
*/
public class HttpUtil<main> {
private static CloseableHttpClient httpClient;
static {
PoolingHttpClientConnectionManager cm = new PoolingHttpClientConnectionManager();
cm.setMaxTotal(100);
cm.setDefaultMaxPerRoute(20);
cm.setDefaultMaxPerRoute(50);
httpClient = HttpClients.custom().setConnectionManager(cm).build();
}
public static String get(String url) {
CloseableHttpResponse response = null;
BufferedReader in = null;
String result = "";
try {
HttpGet httpGet = new HttpGet(url);
RequestConfig requestConfig = RequestConfig.custom().setConnectTimeout(30000).setConnectionRequestTimeout(30000).setSocketTimeout(30000).build();
httpGet.setConfig(requestConfig);
httpGet.setConfig(requestConfig);
httpGet.addHeader("Content-type", "application/json; charset=utf-8");
httpGet.setHeader("Accept", "application/json");
response = httpClient.execute(httpGet);
in = new BufferedReader(new InputStreamReader(response.getEntity().getContent()));
StringBuffer sb = new StringBuffer("");
String line = "";
String NL = System.getProperty("line.separator");
while ((line = in.readLine()) != null) {
sb.append(line + NL);
}
in.close();
result = sb.toString();
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (null != response) {
response.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
return result;
}
public static String post(String url, String jsonString) {
CloseableHttpResponse response = null;
BufferedReader in = null;
String result = "";
try {
HttpPost httpPost = new HttpPost(url);
RequestConfig requestConfig = RequestConfig.custom().setConnectTimeout(30000).setConnectionRequestTimeout(30000).setSocketTimeout(30000).build();
httpPost.setConfig(requestConfig);
httpPost.setConfig(requestConfig);
httpPost.addHeader("Content-type", "application/json; charset=utf-8");
httpPost.setHeader("Accept", "application/json");
httpPost.setEntity(new StringEntity(jsonString, Charset.forName("UTF-8")));
response = httpClient.execute(httpPost);
in = new BufferedReader(new InputStreamReader(response.getEntity().getContent()));
StringBuffer sb = new StringBuffer("");
String line = "";
String NL = System.getProperty("line.separator");
while ((line = in.readLine()) != null) {
sb.append(line + NL);
}
in.close();
result = sb.toString();
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (null != response) {
response.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
return result;
}
}
http下载文件
package com.example.springbootssm.util;
import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.URLConnection;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
/**
* Java原生的API可用于发送HTTP请求,即java.net.URL、java.net.URLConnection,这些API很好用、很常用,
* 但不够简便;
*
* 1.通过统一资源定位器(java.net.URL)获取连接器(java.net.URLConnection) 2.设置请求的参数 3.发送请求
* 4.以输入流的形式获取返回内容 5.关闭输入流
*
* @author H__D
*
*/
public class HttpDownUtil {
/**
*
* @param urlPath
* 下载路径
* @param downloadDir
* 下载存放目录
* @return 返回下载文件
*/
public static File downloadFile(String urlPath, String downloadDir,String ssh) {
File file = null;
try {
// 统一资源
URL url = new URL(urlPath);
// 连接类的父类,抽象类
URLConnection urlConnection = url.openConnection();
// http的连接类
HttpURLConnection httpURLConnection = (HttpURLConnection) urlConnection;
// 设定请求的方法,默认是GET
httpURLConnection.setRequestMethod("GET");
// 设置字符编码
httpURLConnection.setRequestProperty("Charset", "UTF-8");
// 打开到此 URL 引用的资源的通信链接(如果尚未建立这样的连接)。
httpURLConnection.connect();
// 文件大小
int fileLength = httpURLConnection.getContentLength();
// 文件名
//String filePathUrl = httpURLConnection.getURL().getFile();
//String fileFullName = filePathUrl.substring(filePathUrl.lastIndexOf(File.separatorChar) + 1);
System.out.println("file length---->" + fileLength);
URLConnection con = url.openConnection();
BufferedInputStream bin = new BufferedInputStream(httpURLConnection.getInputStream());
Calendar cal=Calendar.getInstance();
cal.add(Calendar.DATE,-1);
Date d=cal.getTime();
SimpleDateFormat sp=new SimpleDateFormat("yyyyMMdd");
String path = downloadDir +"/"+ sp.format(d)+"/"+ssh+".zip";
file = new File(path);
if (!file.getParentFile().exists()) {
file.getParentFile().mkdirs();
}
OutputStream out = new FileOutputStream(file);
int size = 0;
int len = 0;
byte[] buf = new byte[1024];
while ((size = bin.read(buf)) != -1) {
len += size;
out.write(buf, 0, size);
// 打印下载百分比
// System.out.println("下载了-------> " + len * 100 / fileLength +
// "%\n");
}
bin.close();
out.close();
} catch (MalformedURLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
return file;
}
}
// public static void main(String[] args) {
//
// // 下载文件测试
// downloadFile("http://localhost:8080/images/1467523487190.png", "D:/code/barcode");
//
// }
}