//1题
#include
double harmonic_mean(double, double);
int main()
{
using namespace std;
double a,b;
double har_m;
cout <<"请输入俩个数: “;
while (cin >> a >> b && a !=0 && b!=0)
{
while(cin.get() != ‘\n’)
continue;
har_m = harmonic_mean(a, b);
cout <<a <<” " << b <<"的调和平均数是: " << har_m << endl;
}
return 0;
}
double harmonic_mean(double a, double b)
{
double hn = 2.0 * a * b / (a + b);
return hn;
}
//2题
#include
const int Max = 10;
void in_put(double *);
void display(const double *);
double average(const double *);
using namespace std;
int main()
{
double aver;
double golf_scores[Max];
in_put(golf_scores);
display(golf_scores);
aver = average(golf_scores);
cout <<"\n平均成绩 = " << aver << endl;
return 0;
}
void in_put(double *pf)
{
int i = 0;
cout <<“请输入最多10个 高尔夫成绩(输入q结束) : \n”;
while (cin >> pf[i] && pf[i] != ‘q’)
{
i++;
if (i >= Max)
{
cout <<“数组已满!\n”;
break;
}
}
}
void display(const double * n)
{
cout <<"高尔夫成绩: “;
for (int i = 0; i < Max; i++)
{
if (n[i] == 0)
break;
else
cout <<n[i] <<” ";
}
}
double average(const double * n)
{
double totle = 0;
double aver = 0;
int count = 0;
for (int i = 0; i < Max; i++)
{
if (n[i] != 0)
++count;
totle += n[i];
}
aver = totle / count;
return aver;
}
//3题
#include
struct box {
char maker[40];
float height;
float width;
float length;
float volume;
};
void display(const struct box);
void product(struct box *);
int main()
{
struct box b = {
“wawawawa”,
4,
5,
6,
0
};
display(b);
product(&b);
display(b);
return 0;
}
void display(const struct box a)
{
std::cout <<"maker = " <<a.maker <<std::endl;
std::cout <<"height = " <<a.height <<std::endl;
std::cout <<"width = " << a.width << std::endl;
std::cout <<"length = " <<a.length << std::endl;
std::cout <<"volume = " <<a.volume << std::endl;
}
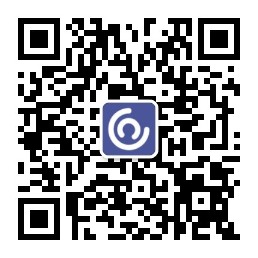
void product(struct box * a)
{
a->volume = a->height * a->width * a->length;
}
//4题
#include
long double probability(unsigned numbers, unsigned picks, unsigned numbers2,unsigned picks2);
int main()
{
using namespace std;
double total1, choices1,total2,choices2;
cout <<“Please enter the total number of the first selection and\n”
" the number that can be selected:\n";
while ((cin >> total1 >> choices1) && choices1 <= total1)
{
cout <<“Please enter the total number of the second selection\n”
" and the number that can be selected:\n";
cin >> total2 >> choices2;
if (total2 <= choices2)
{
cout <<“Please enter the correct number:\n”;
cin >> total2 >> choices2;
}
cout <<“You have one chance in “;
cout << probability(total1, choices1,total2,choices2);
cout <<” of winning.\n”;
cout <<"Next two numbers (q to quit): ";
}
cout <<“bye\n”;
return 0;
}
long double probability(unsigned numbers, unsigned picks, unsigned numbers2,unsigned picks2)
{
long double result1 = 1.0;
long double result2 = 1.0;
long double result = 1.0;
long double n;
unsigned p;
for (n = numbers, p = picks ; p > 0; n–, p–)
result1 = result1 * n / p;
for (n = numbers2, p = picks2; p > 0; n–, p–)
result2 = result2 * n / p;
result = result2 * result1;
return result;
}
//5题
#include
long double factorial(int n);
int main()
{
long double fac;
int n;
std::cout <<"请输入需要查询阶乘的整数: “;
std::cin >> n;
fac = factorial(n);
std::cout << n <<” 的阶乘 = " << fac << std::endl;
return 0;
}
long double factorial(int n)
{
long double fact;
if (n == 0)
return fact = 1;
fact = n * factorial(n - 1);
return fact;
}
//6题
#include
void Fill_array(double [], int n);
void Show_array(double [],int n);
void Reverse_array(double [], int n);
const int SIZE = 10;
using namespace std;
int main()
{
double darray[SIZE];
Fill_array(darray, SIZE);
Show_array(darray, SIZE);
Reverse_array(darray, SIZE);
Show_array(darray, SIZE);
return 0;
}
void Fill_array(double a[], int n)
{
cout <<“请输入 " << n <<” 个浮点数:\n";
for (int i = 0; i < n; i++)
{
if(!(cin >> a[i]))
break;
}
}
void Show_array(double a[], int n)
{
cout <<“查看的数组情况是:\n”;
for (int i = 0; i < n; i++)
cout << a[i] <<"\t";
cout <<"\n";
}
void Reverse_array(double a[], int n)
{
int i,j;
double num;
for (i = 1, j = n - 2; i < n / 2 - 1; i++, j–)
{
num = a[i] ;
a[i] = a[j];
a[j] = num;
}
}
//7题
#include
const int Max = 5;
double * fill_array(double *, double *);
void show_array(double *, double *);
void revalue(double b, double *,double *);
int main()
{
using namespace std;
double properties[Max];
double *pk, *pj; //开始k,结尾j
pk = properties; //定位指针指向数组开始
pj = &properties[Max-1]; //指针指向数组末尾
pj = fill_array(pk, pj);
show_array(pk, pj);
if (pj > pk)
{
cout <<"Enter revaluation factor: ";
double factor;
while (!(cin >> factor))
{
cin.clear();
while (cin.get() != ‘\n’)
continue;
cout <<"Bad input; please enter a number: ";
}
revalue(factor, pk, pj);
show_array(pk, pj);
}
cout <<“Done.\n”;
cin.get();
cin.get();
return 0;
}
double * fill_array(double *pk, double *pj)
{
using namespace std;
double temp;
int i = 1;
while (pk <= pj)
{
cout <<“Enter value #” << i <<": ";
cin >> temp;
if (!cin)
{
cin.clear();
while (cin.get() != ‘\n’)
continue;
cout <<“Bab input; input process terminated.”;
break;
}
else if (temp < 0)
break;
*pk = temp;
++pk;
++i;
}
pj = --pk;
return pj;
}
void show_array(double *pk, double *pj)
{
using namespace std;
int i = 1;
while (pk <= pj)
{
cout <<“Property #” << i++ <<": $";
cout <<*pk << endl;
++pk;
}
}
void revalue(double b, double *pk, double *pj)
{
while (pk <= pj)
{
*pk *= b;
++pk;
}
}
//8题
//a
#include
#include
const int Seasons = 4;
//存储指针的数组
const char *Snames[Seasons] = {“Spring”, “Summer”, “Fall”, “Winter”}; //
void fill(double pa[]);
void show(const double da[]);
int main()
{
double expenses[Seasons];
fill(expenses);
show(expenses);
return 0;
}
void fill(double pa[])
{
using namespace std;
for (int i = 0; i <Seasons; i++)
{
cout <<“Enter " << Snames[i] <<” expenses: ";
cin >> pa[i];
}
}
void show(const double da[])
{
using namespace std;
double total = 0.0;
cout <<“EXPENSES\n”;
for (int i = 0; i < Seasons; i++)
{
cout << Snames[i] <<": $" << da[i] << endl;
total += da[i];
}
cout <<“Total Expenses: $” << total << endl;
}
//b
#include
#include
const int Seasons = 4;
//存储指针的数组
const char *Snames[Seasons] = {“Spring”, “Summer”, “Fall”, “Winter”}; //
void fill(struct Expenses * pa);
void show(const struct Expenses * da);
struct Expenses {
double exp[Seasons];
};
int main()
{
struct Expenses expenses;
fill(&expenses);
show(&expenses);
return 0;
}
void fill(struct Expenses * pa)
{
using namespace std;
for (int i = 0; i <Seasons; i++)
{
cout <<“Enter " << Snames[i] <<” expenses: ";
cin >> pa->exp[i];
}
}
void show(const struct Expenses * da)
{
using namespace std;
double total = 0.0;
cout <<“EXPENSES\n”;
for (int i = 0; i < Seasons; i++)
{
cout << Snames[i] <<": $" << da->exp[i] << endl;
total += da->exp[i];
}
cout <<“Total Expenses: $” << total << endl;
}
//9题
#include
using namespace std;
const int SLEN = 30;
struct student {
char fullname[SLEN];
char hobby[SLEN];
int ooplevel;
};
int getinfo(student pa[], int n);
void display1(student st);
void display2(const student *st);
void display3(const student st[], int n);
int main()
{
cout <<"Enter class size: ";
int class_size;
cin >> class_size;
if (!cin)
{
cin.clear();
cout <<“Incorrect input!\n”;
return 0;
}
while (cin.get() != ‘\n’)
continue;
student *ptr_stu = new student[class_size];
int entered = getinfo(ptr_stu, class_size);
for (int i = 0; i < entered; i++)
{
display1(ptr_stu[i]);
display2(&ptr_stu[i]);
}
display3(ptr_stu, entered);
delete [] ptr_stu;
cout <<“Done\n”;
return 0;
}
int getinfo(student pa[], int n)
{
int num = 0;
for (int i = 0; i < n; i++)
{
cout <<“Please enter the full Student Name:\n”;
cin.getline(pa[i].fullname, SLEN);
cout <<“Please enter your hobbies:\n”;
cin.getline(pa[i].hobby, SLEN);
cout <<“Please enter ooplevel:\n”;
cin >> pa[i].ooplevel;
cin.get();
if(pa[i].fullname[0] == ‘\0’ || pa[i].hobby[0] == ‘\0’)
break;
num += 1;
}
return num;
}
void display1(student st)
{
cout <<"fullname: " << st.fullname << endl;
cout <<“hobby: " << st.hobby << endl;
cout <<“ooplevel: " << st.ooplevel << endl;
cout <<”\n\n”;
}
void display2(const student * st)
{
cout <<"fullname: " << st->fullname << endl;
cout <<“hobby: " << st->hobby << endl;
cout <<“ooplevel: " << st->ooplevel << endl;
cout <<”\n\n”;
}
void display3(const student st[], int n)
{
for (int i = 0; i < n; i++)
{
cout <<"fullname: " << st[i].fullname << endl;
cout <<“hobby: " << st[i].hobby << endl;
cout <<“ooplevel: " << st[i].ooplevel << endl;
cout <<”\n\n”;
}
}
//10题
#include
double calculate(double,double, double (*hp)(double, double));
double add1(double x, double y) { return x + y;};
double add2(double x, double y) { return x - y;};
double add3(double x, double y) { return x * y;};
double add4(double x, double y) { return x / y;};
double (*hp[4])(double, double) ={add1, add2, add3, add4};
int main()
{
using namespace std;
double a, b, result;
cout <<“请输入俩个浮点数 (q 退出): \n”;
while (cin >> a >> b)
{
for (int i = 0; i < 4; i++)
{
result = calculate(a, b, hp[i]);
if (0 == i)
cout << a <<" + " << b <<" = "<< result << endl;
else if (1 == i)
cout << a <<" - " << b <<" = "<< result << endl;
else if (2 == i)
cout << a <<" * " << b <<" = "<< result << endl;
else if(3 == i)
cout << a <<" / " << b <<" = "<< result << endl;
}
}
}
double calculate(double x, double y, double (*hp)(double, double))
{
double num;
num = (*hp)(x, y);
return num;
}
在这里插入代码片