概述: Spring 中bean的作用域有4种,默认为singleton.
类型 | 作用域范围 |
singleton | 默认类型单例,通过Spring容器获取的该对象是唯一的 |
prototype | 通过Spring容器获取对象时都会new一个新的对象出来 |
request | 表示在一个请求内有效 |
session | 表示在一个会话内有效 |
Sample:
Entity类:

1 package com.test.entity; 2 3 public class Students { 4 private int id; 5 6 private String name; 7 8 private int age; 9 10 private Classes classes; 11 12 public Classes getClasses() { 13 return classes; 14 } 15 16 public void setClasses(Classes classes) { 17 this.classes = classes; 18 } 19 20 public Students(int id, String name, int age) { 21 this.id = id; 22 this.name = name; 23 this.age = age; 24 } 25 26 public Students(int id, String name, int age, Classes classes) { 27 this.id = id; 28 this.name = name; 29 this.age = age; 30 this.classes = classes; 31 } 32 33 public Students(){ 34 35 } 36 37 public int getId() { 38 return id; 39 } 40 41 public void setId(int id) { 42 this.id = id; 43 } 44 45 public String getName() { 46 return name; 47 } 48 49 public void setName(String name) { 50 this.name = name; 51 } 52 53 public int getAge() { 54 return age; 55 } 56 57 public void setAge(int age) { 58 this.age = age; 59 } 60 61 @Override 62 public String toString() { 63 return "Students{" + 64 "id=" + id + 65 ", name='" + name + '\'' + 66 ", age=" + age + 67 ", classes=" + classes + 68 '}'; 69 } 70 }
Spring配置:

1 <bean id="stu1" class="com.test.entity.Students"></bean> 2 3 <bean id="stu2" class="com.test.entity.Students" scope="prototype"></bean>
测试类:
扫描二维码关注公众号,回复:
10680916 查看本文章
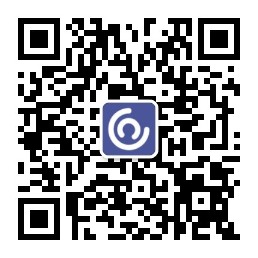

1 public static void main(String[] args) { 2 ApplicationContext applicationContext = new ClassPathXmlApplicationContext("Spring.xml"); 3 4 //bean scope test --- default 5 Students stuSingleton1 = (Students) applicationContext.getBean("stu1"); 6 7 Students stuSingleton2 = (Students) applicationContext.getBean("stu1"); 8 9 System.out.println(stuSingleton1 == stuSingleton2); 10 11 //bean scope test --- prototype 12 Students stuPrototype1 = (Students) applicationContext.getBean("stu2"); 13 14 Students stuPrototype2 = (Students) applicationContext.getBean("stu2"); 15 16 System.out.println(stuPrototype1 == stuPrototype2); 17 }
测试结果:

1 true 2 false
depends-on:

1 <bean id="car" class="com.test.entity.Car"> 2 <property name="id" value="1"></property> 3 <property name="name" value="car"></property> 4 </bean> 5 6 <bean id="dependsStu" class="com.test.entity.Students" depends-on="car"> 7 <property name="id" value="55"></property> 8 <property name="name" value="testDepends"></property> 9 <property name="age" value="25"></property> 10 </bean>