C++ primer Plus(第六版)课后编程题
第二章
2-1
#include<iostream>
using namespace std;
int main(){
cout<<"My name is Xin Tang,and I come from Zhangzhou,Henan."<<endl;
}
2-2
#include<iostream>
using namespace std;
double furtoyd(double furlong);
int main(void){
double furlong;
cout<<"Please enter the distance measured by furlong units: ";
cin>>furlong;
cout<<endl<<furlong<<" furlong is "<<furtoyd(furlong) <<" yd.";
return 0;
}
double furtoyd(double furlong){
return 220*furlong;
}
2-3
#include<iostream>
using namespace std;
void pri1(void);
void pri2(void);
int main(void){
pri1();
pri1();
pri2();
pri2();
return 0;
}
void pri1(void){
cout<<"Three blind mice"<<endl;
}
void pri2(void){
cout<<"See how they run"<<endl;
}
2-4
#include<iostream>
using namespace std;
int yeartomonth(int year);
int main(void){
int age;
cout<<"Please enter you age:";
cin>>age;
cout<<"Your age is "<<yeartomonth(age)<<" months.";
return 0;
}
int yeartomonth(int year){
return 12*year;
}
2-5
#include<iostream>
using namespace std;
double celtoFahren(double celsius);
int main(void){
double celsius;
cout<<"Please enter a Celsius value:";
cin>>celsius;
cout<<celsius<<"degrees Celsius is "<<celtoFahren(celsius)<<" degree Fahreheit.";
return 0;
}
double celtoFahren(double celsius){
return 1.8*celsius+32.0;
}
2-6
#include<iostream>
using namespace std;
double lightyeartoastro(double lightyear);
int main(void){
double lightyear;
cout<<"Please enter the number o light years : ";
cin>>lightyear;
cout<<lightyear<<" light years = "<<lightyeartoastro(lightyear)<<" astronimical units.";
return 0;
}
double lightyeartoastro(double lightyear){
return lightyear*63240;
}
2-7
#include<iostream>
using namespace std;
int main(void){
int hours;
int minutes;
cout<<"Enter the number of hours : ";
cin>>hours;
cout<<"Enter the number of minutes : ";
cin>>minutes;
cout<<"Time : "<<hours<<" : "<<minutes<<endl;
return 0;
}
第三章
3-1
#include<iostream>
//const 常量,1英尺=12英寸
const int inch_per_feet=12;
int main(void){
using namespace std;
int ht_inch;
cout<<"Enter your height_inch : ___\b\b\b ";
cin>>ht_inch;
cout<<"Your height_feet is : " <<ht_inch/inch_to_feet<<" feet and "<<ht_inch%inch_to_feet<<" inch.";
return 0;
}
3-2
#include<iostream>
//const 常量,1英尺=12英寸
const int inch_per_feet=12;
//1英寸=0.0254米
const double meter_per_inch=0.0254;
//1磅=2.2千克
const double pound_per_kilogram=2.2;
int main(void){
using namespace std;
int ht_inch,ht_feet;
double wt_pound;
cout<<"Enter your height : \n";
cout<<"First enter your height of feet part : _\b ";
cin>>ht_feet;
cout<<"First enter your height of inch part : _\b" ;
cin>>ht_inch;
cout<<"Then enter your weight in pound : ___\b\b\b";
cin>>wt_pound;
double ht_meter;
ht_meter=meter_per_inch*(ht_inch+ht_feet*inch_per_feet);
cout<<"身高为:"<<ht_meter<<" 米。\n";
double weight_kilo;
weight_kilo=pound_per_kilogram*wt_pound;
cout<<"体重为:"<<ht_meter<<" 千克。\n";
double BMI;
BMI=weight_kilo/(ht_meter*ht_meter);
cout<<"BMI为:"<<BMI;
return 0;
}
3-3
#include<iostream>
//1度=60分
const int minutes_per_degree=60;
//1分=60秒
const int seconds_per_minute=60;
int main(void){
using namespace std;
int degrees,minutes,seconds;
double degree_all;
cout<<"Enter a latitude in degrees ,minutes, and seconds: \n";
cout<<"First enter the degree: _\b ";
cin>>degrees;
cout<<"Next, enter the minutes of arc: _\b ";
cin>>minutes;
cout<<"Finally, enter the seconds of arc: _\b ";
cin>>seconds;
cout<<degrees<<" degrees ,"<<minutes<<" minutes,"<<seconds<<" seconds = "<<(double)degrees+(double)minutes/minutes_per_degree+(double)seconds_per_minute/minutes_per_degree/seconds_per_minute<<" degrees.";
return 0;
}
3-4
扫描二维码关注公众号,回复:
10588558 查看本文章
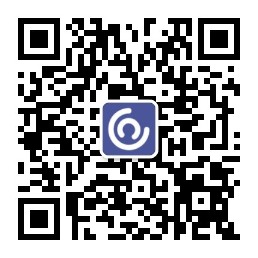
#include<iostream>
const int hours_per_day=24;
const int minutes_per_hours=60;
const int seconds_per_minute=60;
int main(void){
using namespace std;
int days,hours,minutes,seconds,second;
cout<<"Enter the number of seconds:_____\b\b\b\b\b ";
cin>>seconds;
days=seconds/seconds_per_minute/minutes_per_hours/hours_per_day;
hours=seconds/seconds_per_minute/minutes_per_hours%hours_per_day;
minutes=seconds/seconds_per_minute%minutes_per_hours;
second=seconds%seconds_per_minute;
cout<<seconds<<" seconds = "
<<days<<" days , "<<hours<<" hours , "<<minutes<<" minutes , "<<second<<" seconds .";
return 0;
}
3-5
#include<iostream>
int main(void){
using namespace std;
long long world_pop,Ame_pop;
double percentage;
cout<<"Enter the world's population:_____\b\b\b\b\b ";
cin>>world_pop;
cout<<"Enter the population of the US:_____\b\b\b\b\b ";
cin>>Ame_pop;
percentage=(double)Ame_pop/world_pop*100;
cout<<"The population of the US is "<<percentage<<" % of the world population. ";
return 0;
}
3-6
#include<iostream>
int main(void){
using namespace std;
double m_distance,m_gasoline,k_distance,k_gasoline;
cout<<"Enter the miles of distance you have driven:___\b\b\b ";
cin>>m_distance;
cout<<"Enter the gallons of gasoline you have used:___\b\b\b ";
cin>>m_gasoline;
cout<<"Your car can run "<<m_distance/m_gasoline<<" miles per gallon.\n";
cout<<"Enter the kilograms of distance you have driven:___\b\b\b ";
cin>>k_distance;
cout<<"Enter the petrol in liters you have used:___\b\b\b ";
cin>>k_gasoline;
cout<<"In European : Your car can run "<<100*k_gasoline/k_distance<<" liter of petrol per 100 kilometer.\n";
return 0;
}
3-7
#include<iostream>
int main(void){
using namespace std;
double u_style,m_style;
cout<<"Enter the fuel consumption in European(L/km):___\b\b\b ";
cin>>u_style;
cout<<"converts to US_Style(miles per gallon) "<<u_style<<" L/km = "<<3.875*62.14/u_style<<" mpg.\n";
return 0;
}
第四章
待更新