今天给大家演示的是一款由jsp+ssm框架(spring+springMVC+mybatis)+mysql实现的在线考试系统源码和开发教程,本系统配有完整的源码、视频开发教程、数据库文件、项目素材等详细材料。这里强调一点,本系统是在我们的万能脚手架系统的基础上开发的,如果你还没有学习脚手架系统开发教程的话请先去学习脚手架系统,如果你不学习脚手架系统的话这个系统你学不会哦!当然如果你只想要本系统的代码,并不想学习开发教程的话那你直接获取本项目资料就可以了,或者你基础能力很牛逼,那你也可以直接学习这个。本系统除了脚手架的基本功能外,实现的关于在线考试的功能有:用户前台:用户注册登录、查看考试信息、进行考试、查看考试成绩、查看历史考试记录、回顾已考试卷、修改密码、修改个人信息等,后台管理功能(脚手架功能不在这里列出),科目专业管理、考生管理、试题管理、考试管理、试卷管理、答题详情管理、考试成绩图表统计等,其中试题可以支持批量excel文件导入,试卷试题是随机生成的,每个人的试卷试题都不一样。
代码已经上传github,下载地址: https://github.com/21503882/exam
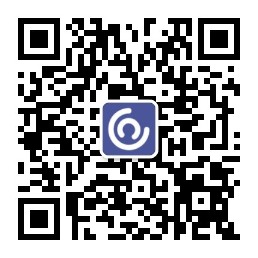
/**
* 学生Action类
* @author Administrator
*
*/
public class StudentAction extends ActionSupport implements ServletRequestAware{
/**
*
*/
private static final long serialVersionUID = 1L;
private StudentDao studentDao=new StudentDao();
private HttpServletRequest request;
private String mainPage;
private Student student;
private String error;
private String page;
private int total;
private String pageCode;
private List<Student> studentList;
private Student s_student;
private String id; // 学生编号
private String title; // 标题
public Student getStudent() {
return student;
}
public void setStudent(Student student) {
this.student = student;
}
public String getError() {
return error;
}
public void setError(String error) {
this.error = error;
}
public String getMainPage() {
return mainPage;
}
public void setMainPage(String mainPage) {
this.mainPage = mainPage;
}
public String getPageCode() {
return pageCode;
}
public void setPageCode(String pageCode) {
this.pageCode = pageCode;
}
public int getTotal() {
return total;
}
public void setTotal(int total) {
this.total = total;
}
public String getPage() {
return page;
}
public void setPage(String page) {
this.page = page;
}
public Student getS_student() {
return s_student;
}
public void setS_student(Student s_student) {
this.s_student = s_student;
}
public List<Student> getStudentList() {
return studentList;
}
public void setStudentList(List<Student> studentList) {
this.studentList = studentList;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
/**
* 登录验证
* @return
* @throws Exception
*/
public String login()throws Exception{
HttpSession session=request.getSession();
Student currentUser=studentDao.login(student);
if(currentUser==null){
error="准考证号或者密码错误!";
return ERROR;
}else{
session.setAttribute("currentUser", currentUser);
return SUCCESS;
}
}
/**
* 修改密码预操作
* @return
* @throws Exception
*/
public String preUpdatePassword()throws Exception{
mainPage="student/updatePassword.jsp";
return SUCCESS;
}
/**
* 修改密码
* @return
* @throws Exception
*/
public String updatePassword()throws Exception{
Student s=studentDao.getStudentById(student.getId());
s.setPassword(student.getPassword());
studentDao.saveStudent(s);
mainPage="student/updateSuccess.jsp";
return SUCCESS;
}
/**
* 查询学生信息
* @return
* @throws Exception
*/
public String list()throws Exception{
HttpSession session=request.getSession();
if(StringUtil.isEmpty(page)){
page="1";
}
if(s_student!=null){
session.setAttribute("s_student", s_student);
}else{
Object o=session.getAttribute("s_student");
if(o!=null){
s_student=(Student)o;
}else{
s_student=new Student();
}
}
PageBean pageBean=new PageBean(Integer.parseInt(page),Integer.parseInt(PropertiesUtil.getValue("pageSize")));
studentList=studentDao.getStudents(s_student,pageBean);
total=studentDao.studentCount(s_student);
pageCode=PageUtil.genPagation(request.getContextPath()+"/student!list",total, Integer.parseInt(page), Integer.parseInt(PropertiesUtil.getValue("pageSize")));
mainPage="student/studentList.jsp";
return SUCCESS;
}
/**
* 获取学生
* @return
* @throws Exception
*/
public String getStudentById()throws Exception{
student=studentDao.getStudent(id);
mainPage="student/studentSave.jsp";
return SUCCESS;
}
/**
* 保存学生
* @return
* @throws Exception
*/
public String saveStudent()throws Exception{
if(StringUtil.isEmpty(student.getId())){
student.setId("JS"+DateUtil.getCurrentDateStr());
}
studentDao.saveStudent(student);
return "save";
}
/**
* 删除学生
* @return
* @throws Exception
*/
public String deleteStudent()throws Exception{
student=studentDao.getStudent(id);
studentDao.studentDelete(student);
JSONObject resultJson=new JSONObject();
resultJson.put("success",true);
ResponseUtil.write(resultJson,ServletActionContext.getResponse());
return null;
}
/**
* 预添加操作
* @return
* @throws Exception
*/
public String preSave()throws Exception{
if(StringUtil.isNotEmpty(id)){
student=studentDao.getStudent(id);
title="修改学生信息";
}else{
title="添加学生信息";
}
mainPage="student/studentSave.jsp";
return SUCCESS;
}
/**
* 注销用户
* @throws Exception
*/
public String logout()throws Exception{
request.getSession().invalidate();
return "logout";
}
public void setServletRequest(HttpServletRequest request) {
this.request=request;
}
}
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>管理员登录考试系统</title>
<link href="${pageContext.request.contextPath}/style/studentInfo.css" rel="stylesheet">
<link href="${pageContext.request.contextPath}/bootstrap/css/bootstrap.css" rel="stylesheet">
<link href="${pageContext.request.contextPath}/bootstrap/css/bootstrap-responsive.css" rel="stylesheet">
<script src="${pageContext.request.contextPath}/bootstrap/js/jQuery.js"></script>
<script src="${pageContext.request.contextPath}/bootstrap/js/bootstrap.js"></script>
<script type="text/javascript">
function checkForm(){
var userName=document.getElementById("userName").value;
var password=document.getElementById("password").value;
if(userName==null||userName==""){
alert("用户名不能为空!");
return false;
}
if(password==null||password==""){
alert("密码不能为空!");
return false;
}
return true;
}
function resetValue(){
document.getElementById("userName").value="";
document.getElementById("password").value="";
}
</script>
</head>
<body >
<div align="center" style="padding-top: 20px;" >
<form action="manager!login" method="post" οnsubmit="return checkForm()">
<table width="1004" height="584" background="image/login_admin.jpg" >
<tr height="200">
<td colspan="4"></td>
</tr>
<tr height="10">
<td width="68%"></td>
<td width="10%"><label>用户名:</label></td>
<td><input type="text" id="userName" name="manager.userName" value="${manager.userName }" placeholder="请输入管理员用户名"/></td>
<td width="30%"></td>
</tr>
<tr height="10">
<td width="40%"></td>
<td width="10%"><label>密码:</label></td>
<td><input type="password" id="password" name="manager.password" value="${manager.password }" placeholder="请输入管理员密码"/></td>
<td width="30%"></td>
</tr>
<tr height="10">
<td width="40%"></td>
<td width="10%"><button class="btn btn-primary" type="submit">登录</button></td>
<td>
<button class="btn btn-primary" type="button" οnclick="resetValue()">重置</button>
<a class="btn btn-primary" style="margin-left:30px;" href="login.jsp">考生登录</a>
</td>
<td width="30%"></td>
</tr>
<tr>
<td></td>
</tr>
</table>
</form>
</div>
</body>
</html>