Java Virtual Machine architecture
Java’s extra run-time housekeeping– array bounds checking, type-safe reference casting, checking for null references, and garbage-collection – will cause your java program to be slower than an equivalent C++ program
Another speed hit, and one can be far more substantial, arises from the interpreted nature of Java programs, which are usually compiled to Java byte-codes, that are stored in class files. When Java program runs, a virtual machine loads the class files and executes the byte-codes they contain. When running on a virtual machine that interprets byte-codes, a Java program may be 10 to 30 times slower than an equivalent C++ program compiled to native machine code.
just-in-time compiling can speed up program execution 7 to 10 times over interpreting. A virtual machine can compile the byte-codes to native machine code the first time the method is invoked. The native machine code version of the method is then cached by the virtual machine, and re-used the next time the method is invoked by the program.
Java programs can run slower than an equivalent C++ program for many reasons:
- Interpreting byte-codes is 10 to 30 time slower than native execution
- Just-in-time compiling byte-codes can be 7 to 10 times faster than interpreting, but still not quite as fast as native execution.
- Java programs are dynamically linked
- The Java Virtual Machine may have to wait for class file to download across a network
- Array bounds are checked on each array access
- All objects are created on the heap(no objects are created on the stack)
- All uses of object reference are checked at run-time for null
- All reference casts are checked at run-time for type safety
- The garbage collector is likely less efficient(though often more effective) at managing the heap than you could be if you managed it directly as in C++
- Primitive types in Java are the same on every platform, rather than adjusting to the most efficient size on each platform as in C++
String in Java are always UNICODE. When you really need to manipulate just as ASCII string, a Java program will be slightly less efficient than an equivalent C++ program.
Three different things when you say “Java Virtual Machine”:
the abstract specification
- a concrete implementation
- a run-time instance
A Java Virtual Machine instance starts running its solitary application by invoking the main() method of some initial class.
Inside the Java Virtual machine, threads come in two flavors: daemon and non-daemon. A daemon thread is ordinarily a thread used by the virtual machine itself, such as a thread that performs garbage collection. The application, however, can mark any threads it creates as daemon thread. The initial thread of an application - the one that begins at main() - is a non-daemon thread. A Java application continues to execute as long as any non-daemon threads are still running. When all non-daemon threads of a Java application terminate, the virtual machine instance will exit. If permitted by the security manager, the application can also cause its own demise by invoking the exit() method of class Run-time of System
In the Java Virtual Machine specification, the behavior of a virtual machine instance is described in terms of subsystems(class loader system), memory areas, data types, and instructions. each Java Virtual Machine has a class loader subsystem: a mechanism for loading types(classes and interfaces) given fully qualified names. Each Java Virtual Machine also have a execution engine: a mechanism responsible for executing the instructions contained in the methods of loaded classes
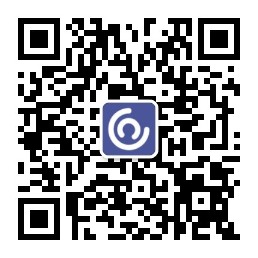
Each instance of the Java Virtual Machine has one method area and one heap. These areas are shared by all threads running inside the virtual machine. When the virtual machine loads a class file, it parses information about a type contained in the class file and places this type information into the method area. As the program runs, the virtual machine places all objects the program instantiates onto the heap.
As each new thread comes into existence, it gets its own pc register(program counter) and Java stack. If the thread is executing a Java method(not a native method), the value of the pc register indicates the next instruction to execute. A thread’s Java stack stores the state of java method invocations for the thread. The state of a Java method invocation includes its local variables, the parameters with which it was invoked, its return value, and intermediate calculations. The state of native method invocation is stored in an implementation-dependent way in native method stacks, as well as possibly in registers or other implementation-dependent memory areas.
the Java stack is composed of stack frames. A stack frame contains the state of one Java method invocation. When a thread invokes a method, the Java Virtual Machine pushes a new frame onto the thread’s Java stack. When the method completes, the virtual machine pops and discards the frame for that method.
In byte-codes, Java virtual machine uses ints or bytes to represent booleans. In the Java Virtual Machine, false is represented by integer zero and true by any non-zero integer. Operations involving boolean values use ints. Arrays of boolen are accessed as array of bytes, though they may be represented on the heap as arrays of byte or as bit fields
A special primitive type of Java Virtual Machine is: the returnValue type, which is unavailable to the Java programmer. This primitive type is used to implement finally clauses of java programs
Values of type reference come in three flavors: the class type, the interface type, and the array type. All three types have values that are references to dynamically created objects. The class type’s values are references to class instances. The array type’s values are references to arrays, which are full-fledged objects in the Java Virtual machine. The interface type’s values are references to class instances that implement an interface.
Inside a Java Virtual machine instance, information about loaded types is stored in a logical area of memory called the method area. Memory for class (static) variables declared in the class is also taken from the method area. All threads share the same method area, so access to the method area’s data structures must be designed to be thread-safe.
Type information
For each type is loads, a Java virtual machine must store the following kinds of information in the method area:
- The fully qualified name of the type
- the fully qualified name of the type’s direct superclass(unless the type is an interface or class java.lang.Object, neither of which have a superclass)
- Whether or not the type is a class or an interface
- The type’s modifiers(some subset to public, abstract, final)
- And ordered list of the fully qualified name of any direct super-interfaces
- The constant pool for the type
- Field information(including the order in which the fields are declared)
- the field’s name
- the field’s type
- the field’s modifiers(some subset of public, private, protected, static, final, volatile, transient)
Method information(including the order in which the methods are declared)
- the method’s name
- the method’s return type(or void)
- the number and types (in order) of the method’s paramters
- the method’s modifiers(some subset of public, private, protected, static, final, synchronized, native, abstract)
In addition to above items, the following information must be stored with each method that is not abstract or native:
-the method’s bytecodes - the size of the operand stack and local variables sections of the method’s stack frame
- An exception table
All class (static) variables declared in the type, except constants
- A reference to class ClassLoader
- A reference to class Class
Arrays are always stored on the heap. As=lso like objects, implementation designers can decide how the want to represent arrays on the heap. Arrays have a Class instance assoicated with their class. All arrays of the same dimension and type have the same class. For example, an array of three ints has the same class as an array of three hundred ints. The length of an array is considered part of its instance data. For example, the name class for an array of ints is “[I”, The class name for a three-dimensional array of bytes is “[[[B”, the class name for a two-dimensional array of Objects is “[[Ljava.lang.Object”
Each thread of a running program has its own pc register,or program counter, which is created when the thread is started. When a thread invokes a Java method, the virtual machine creates and pushes a new frame into the thread’s java stack. This new frame then becomes the current frame. As the method executes, it uses the frame to store parameters, local variables, intermediate computations, and other data. When a thread invokes a method, the method’s local variables are stored in a frame on the invoking thread’s java stack.
The stack frame has three parts: local variables, operand stack, and frame data. Compilers place the parameters into the local variable array first, in the order in which they are declared. The Java stack frame includes data to support constant pool resolution, normal method return, and exception dispatch. And this data is stored in the frame data portion of the Java stack frame.
The Java Virtual Machine uses the operand stack as a work space.