移动端事件
1.目标
- 掌握移动端 touch 事件使用
- 掌握 touch 事件和 mouse 事件的区别
- 掌握 touchEvent
- 实现移动端幻灯片
2.移动端touch事件
- touchstart
- touchmove
- touchend
- touch 事件 和 mouse 事件的区别
- 事件点透: mouse 事件的延迟问题
- 阻止默认事件
- 阻止 touchstart 事件带来的影响
- 阻止 touchmove 事件带来的影响
- 阻止 touchend 事件带来的影响
2.1touch事件
user-scale=no设置移动端不能缩放
- touchstart:手指触摸,手指在元素上按下
- touchmove:手指移动,手指在元素上按下后,在屏幕任意位置移动
- touchend:手指抬起,手指在元素按下后,在屏幕任意位置抬起
<style>
#box {
width: 200px;
height: 200px;
background: red;
}
</style>
</head>
<body>
<div id="box"></div>
<script>
{
let box = document.querySelector("#box");
box.addEventListener("touchstart",function(){
console.log("手指按下");
});
box.addEventListener("touchmove",function(){
console.log("手指移动");
});
box.addEventListener("touchend",function(){
console.log("手指抬起");
});
}
</script>
</body>
结果:
2.2touch 事件 和 mouse 事件的区别
同样使用mouse 事件时:
box.addEventListener("mousedown",function(){
console.log("鼠标按下");
});
box.addEventListener("mousemove",function(){
console.log("鼠标移动");
});
box.addEventListener("mouseup",function(){
console.log("鼠标抬起");
});
touch 事件 和 mouse 事件的区别:
- mousedown:鼠标按下 touchstart:手指触摸
- mousemove:鼠标移动 touchmove:手指移动
- moveup:鼠标抬起 touchend:手指抬起
- touch事件只能在移动端使用;mouse事件只能在PC端使用;
- touch事件的touchstart只能在元素内触发,touchmove和touchend可以在屏幕任意位置触发;mouse事件都只能在元素内触发;
- touch事件只有在touchstart触发后,才能触发touchmove和touchend事件;但mouse事件的mousemove只要在元素内没有鼠标按下也能触发
2.3事件点透(点击穿透)及解决方案
事件点透是移动端的一个bug。
事件点透:
移动端中,到底支不支持鼠标事件?移动端是兼容鼠标事件的,如点击事件。
移动端鼠标事件问题:移动端鼠标事件本身会有300ms左右的延迟。当在移动端中手指触碰元素后,会立即执行touch事件,然后会记录当前触发事件坐标,当延迟300ms后,会在该坐标位置查找元素,如果该元素有对应的鼠标事件,就会执行。如淘宝中基本没有使用a标签,就是以为事件点透。
事件点透解决方案:
扫描二维码关注公众号,回复:
10322799 查看本文章
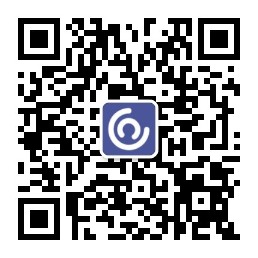
- 在移动端不要使用鼠标事件,包括a标签的href;
- 使用延时定时器,在300ms后再执行(不推荐);
- 阻止默认事件e.preventDefault()。(注意在最新Chrome下不能直接使用e.preventDefault(),需要加上passive:false允许阻止浏览器默认事件)
PC端下正常效果:在百度上只点击一次时,div消失,百度不会跳转
<style>
#box {
position: absolute;
left: 0;
top: 0;
width: 100px;
height: 100px;
background: red;
opacity: .2;
}
</style>
</head>
<body>
<a href="http://www.baidu.com">百度</a>
<div id="box"></div>
<script>
{
let box = document.querySelector("#box");
box.addEventListener("mousedown",()=>{
// console.log("鼠标按下");
box.style.display = "none";
});
}
</script>
</body>
移动端下效果(bug):在百度上只点击一次时,div消失,且直接跳转到了百度页面
let box = document.querySelector("#box");
box.addEventListener("touchend",()=>{
box.style.display = "none";
});
解决移动端下点击穿透:不使用鼠标事件(包括a标签的href);阻止浏览器默认事件e.preventDefault()
let box = document.querySelector("#box");
box.addEventListener("touchend",(e)=>{
box.style.display = "none";
e.preventDefault();
});
2.4移动端阻止默认事件后带来的问题
一般不建议在移动端阻止浏览器默认行为,会带来很多问题。
在移动端touchstart下阻止浏览器默认行为:
- 禁止多指操作时页面缩放;
- 禁止页面的橡皮筋效果(下拉);
- 阻止滚动条滚动(PC端模拟器下滚轮可用,但移动端没有滚轮);
- 阻止鼠标事件触发;
- 输入框不能进行输入;
- 阻止长按选中文字,弹出菜单;
在移动端touchmove下阻止浏览器默认行为:
- 禁止多指操作时页面缩放;
- 禁止页面的橡皮筋效果(下拉);
- 阻止滚动条滚动
在移动端touchmend下阻止浏览器默认行为:
- 阻止鼠标事件触发;
- 输入框不能进行输入;
- 阻止长按选中文字,弹出菜单;
移动端touchstart下阻止默认事件后:
<style>
#list {
padding: 0;
margin: 0;
}
#list li {
height: 30px;
}
</style>
</head>
<body>
<a href="http://www.baidu.com">百度</a><br>
<input type="text">
<ul id="list"></ul>
<script>
{
let list = document.querySelector("#list");
// 通过30个.创建30li
list.innerHTML += [...(".".repeat(30))].map((item,index)=>{
return `<li>这是第${index}个li</li>`;
}).join("");
document.addEventListener("touchstart",(e)=>{
e.preventDefault();
},{
//最新Chrome浏览器下,不允许设置阻止浏览器默认行为,只有设置了passive:false后才会被允许
passive:false
});
}
</script>
</body>
移动端touchmove下阻止浏览器默认行为:
document.addEventListener("touchmove",(e)=>{
e.preventDefault();
},{
passive:false
});
移动端touchend下阻止浏览器默认行为:
document.addEventListener("touchend",(e)=>{
e.preventDefault();
},{
passive:false
});
3.TouchEvent 对象详解
- touches 当前屏幕上的手指列表
- targetTouches 当前元素上的手指列表
- changedTouches 触发当前事件的手指列表
<style>
#box {
width: 300px;
height: 200px;
padding: 10px;
background: red;
font: 20px/40px "宋体";
color: #fff;
}
</style>
</head>
<body>
<div id="box"></div>
<script>
{
let box = document.querySelector("#box");
document.addEventListener("touchstart", function (e) {
//阻止浏览器默认行为,让多指在屏幕上时,不会有缩放等行为
e.preventDefault();
}, {
passive: false
});
box.addEventListener("touchmove", function (e) {
box.innerHTML = `
当前屏幕上的手指列表:${e.touches.length}<br/>
当前元素上的手指列表:${e.targetTouches.length}<br/>
触发当前事件的手指列表:${e.changedTouches.length}
`;
});
}
</script>
</body>
4.案例:移动端滑屏切换的幻灯片
5.下节内容
- 重力加速度
- 摇一摇实现
- 函数防抖与函数节流