c++ thread类:
class myFunc{ public: void operator()(string s){ print("functor", s, " "); s = "fgih"; } }; void test(){ myFunc fun; string s("abcd"); thread t4(fun, s); t4.join(); print(s); }
输出:
functor abcd abcd
Clion编译出错:
CMakeFiles/15_IPC.dir/main.cpp.o:在函数‘shm_mutex_test()’中: /home/enheng/CLionProjects/15_IPC/main.cpp:257:对‘pthread_mutexattr_init’未定义的引用 /home/enheng/CLionProjects/15_IPC/main.cpp:258:对‘pthread_mutexattr_setpshared’未定义的引用
解决办法:
在CMakeLists.txt添加:
find_package(Threads REQUIRED) target_link_libraries(15_IPC Threads::Threads)
将程序修改为引用:
void operator()(string &s){ print("functor", s, " "); s = "fgih"; }程序报错:
/usr/include/c++/6/functional: In instantiation of ‘struct std::_Bind_simple<myFunc(std::__cxx11::basic_string<char>)>’: /usr/include/c++/6/thread:138:26: required from ‘std::thread::thread(_Callable&&, _Args&& ...) [with _Callable = myFunc&; _Args = {std::__cxx11::basic_string<char, std::char_traits<char>, std::allocator<char> >&}]’ /home/enheng/CLionProjects/11_thread/main.cpp:353:21: required from here /usr/include/c++/6/functional:1365:61: error: no type named ‘type’ in ‘class std::result_of<myFunc(std::__cxx11::basic_string<char>)>’ typedef typename result_of<_Callable(_Args...)>::type result_type; ^~~~~~~~~~~ /usr/include/c++/6/functional:1386:9: error: no type named ‘type’ in ‘class std::result_of<myFunc(std::__cxx11::basic_string<char>)>’ _M_invoke(_Index_tuple<_Indices...>) ^~~~~~~~~
再修改:
class myFunc{ public: void operator()(string &s){ print("functor", s, " "); s = "fgih"; } }; void test(){ myFunc fun; string s("abcd"); thread t4(fun, ref(s)); t4.join(); print(s); }
输出:
functor abcd fgih
相关文章:Passing References to Deferred Function Calls with std::ref
"Note that template type deduction is (correctly) deducing the type of value to be int
, but this doesn’t match the type int&
that it sees in start_thread. "
模板推导值的类型是int, 但是不匹配接收类型int &.
扫描二维码关注公众号,回复:
1030080 查看本文章
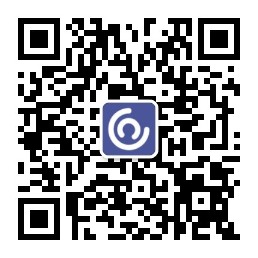
所以要用std::ref来传递引用.
(C++11)
|
可复制构造 (CopyConstructible ) 且可复制赋值 (CopyAssignable ) 的引用包装器 |